
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
thread_border_router_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file thread_border_router_api.h 00017 * \brief Thread border router application interface. 00018 * 00019 * This file contains functions for managing Thread border router features. 00020 * Border routers can set up services in the Thread network including routes, 00021 * DHCPv6 servers, on-mesh prefixes and services. 00022 * 00023 */ 00024 00025 #ifndef THREAD_BORDER_ROUTER_API_H_ 00026 #define THREAD_BORDER_ROUTER_API_H_ 00027 00028 #include "ns_types.h" 00029 00030 /** 00031 * \brief Border router network data structure. 00032 */ 00033 typedef struct thread_border_router_info_t { 00034 unsigned Prf: 2; /**< Prefix preference, 01 = High, 00 = Default, 11 = Low, 10 = Reserved. */ 00035 bool P_preferred: 1; /**< Address is considered preferred address. */ 00036 bool P_slaac: 1; /**< Allowed to configure a new address */ 00037 bool P_dhcp: 1; /**< DHCPv6 server is available in the network. */ 00038 bool P_configure: 1; /**< DHCPv6 agent provides other configuration. */ 00039 bool P_default_route: 1; /**< This device provides the default route. */ 00040 bool P_on_mesh: 1; /**< This prefix is considered to be on-mesh */ 00041 bool P_nd_dns: 1; /**< this border router is able to provide DNS information */ 00042 bool stableData: 1; /**< This data is stable and expected to be available at least 48h. */ 00043 } thread_border_router_info_t; 00044 00045 /** 00046 * \brief Create local service that is provided to the Thread network. 00047 * If a prefix exists it is updated. For example, when removing SLAAC (Stateless Address Autoconfiguration) you should modify the prefix 00048 * first to remove the creation of new addresses and after a while, remove the prefix. 00049 * 00050 * When you have configured the services locally, you need to call 00051 * thread_border_router_publish to make the services available in the network. 00052 * 00053 * \param interface_id Network interface ID. 00054 * \param prefix_ptr Pointer prefix. 00055 * \param prefix_len Length of prefix. 00056 * \param prefix_info_ptr Prefix service structure configuring the published service. 00057 * 00058 * \return 0, Set OK. 00059 * \return <0 Set not OK. 00060 */ 00061 int thread_border_router_prefix_add(int8_t interface_id, uint8_t *prefix_ptr, uint8_t prefix_len, thread_border_router_info_t *prefix_info_ptr); 00062 00063 /** 00064 * \brief Delete local service. 00065 * 00066 * \param interface_id Network interface ID. 00067 * \param prefix_ptr Pointer prefix. 00068 * \param prefix_len Length of prefix. 00069 * 00070 * \return 0, Set OK. 00071 * \return <0 Set not OK. 00072 */ 00073 int thread_border_router_prefix_delete(int8_t interface_id, uint8_t *prefix_ptr, uint8_t prefix_len); 00074 00075 /** 00076 * \brief Add a new route to the Thread network. Other devices can use the route. 00077 * For example, 0::0/0 means that this device provides the default route. 00078 * For example, 2001::0/64 means that this device provides a more specific route. 00079 * 00080 * If a prefix exists it is updated. 00081 * 00082 * \param interface_id Network interface ID. 00083 * \param prefix_ptr Pointer prefix. Can be NULL for the default route. 00084 * \param prefix_len Length of prefix. 00085 * \param stable This data is stable and expected to be available at least 48h. 00086 * \param prf Route preference, 01 = High, 00 = Default, 11 = Low, 10 = Reserved. 00087 * 00088 * \return 0, Set OK. 00089 * \return <0 Set not OK. 00090 */ 00091 int thread_border_router_route_add(int8_t interface_id, uint8_t *prefix_ptr, uint8_t prefix_len, bool stable, int8_t prf); 00092 00093 /** 00094 * \brief Delete locally served route. 00095 * 00096 * \param interface_id Network interface ID. 00097 * \param prefix_ptr Pointer prefix. 00098 * \param prefix_len Length of prefix. 00099 * 00100 * \return 0, Set OK. 00101 * \return <0 Set not OK. 00102 */ 00103 int thread_border_router_route_delete(int8_t interface_id, uint8_t *prefix_ptr, uint8_t prefix_len); 00104 00105 /** 00106 * \brief Add or modify a local service. 00107 * 00108 * \param interface_id Network interface ID. 00109 * \param service_data Pointer to a byte string specifying the type of service. 00110 * \param service_len Length of service data. 00111 * \param sid Service Type ID, a value between 0 and 15, inclusive, assigned to this service data. 00112 * \param enterprise_number Enterprise number of the vendor that defined the type of the server. 00113 * \param server_data Pointer to a byte string containing server-specific information. 00114 * \param server_data_len Length of server data. 00115 * \param stable This data is stable and expected to be available at least 48h. 00116 * 00117 * \return 0, Addition OK. 00118 * \return <0 Addition not OK. 00119 */ 00120 int thread_border_router_service_add(int8_t interface_id, uint8_t *service_data, uint8_t service_len, uint8_t sid, uint32_t enterprise_number, uint8_t *server_data, uint8_t server_data_len, bool stable); 00121 00122 /** 00123 * \brief Delete local service by service data and enterprise number. 00124 * 00125 * \param interface_id Network interface ID. 00126 * \param service_data Pointer to a byte string specifying the type of service. 00127 * \param service_len Length of service. 00128 * \param enterprise_number Enterprise number of the vendor that defined the type of the server. 00129 * 00130 * \return 0, Delete OK. 00131 * \return <0 Delete not OK. 00132 */ 00133 int thread_border_router_service_delete(int8_t interface_id, uint8_t *service_data, uint8_t service_len, uint32_t enterprise_number); 00134 00135 /** 00136 * \brief Publish local services to Thread network. 00137 * 00138 * If local services are deleted before calling this, all services are deregistered from the network. 00139 * 00140 * \param interface_id Network interface ID. 00141 * 00142 * \return 0, Push OK. 00143 * \return <0 Push not OK. 00144 */ 00145 int thread_border_router_publish(int8_t interface_id); 00146 00147 /** 00148 * \brief Clear the local service list. 00149 * 00150 * \param interface_id Network interface ID. 00151 * 00152 * \return 0, Push OK. 00153 * \return <0 Push not OK. 00154 */ 00155 int thread_border_router_delete_all(int8_t interface_id); 00156 00157 /** 00158 * \brief Set Recursive DNS server (RDNSS) option that is encoded according to RFC6106. 00159 * Setting a new RDNSS will overwrite previous RDNSS option. Set RNDDS will be used 00160 * until it is cleared. 00161 * 00162 * \param interface_id Network interface ID. 00163 * \param recursive_dns_server_option Recursive DNS server option encoded according to rfc6106, can be NULL to clear existing RDNSS. 00164 * \param recursive_dns_server_option_len Length of the recursive_dns_server_option in bytes. 00165 * 00166 * \return 0, Option saved OK. 00167 * \return <0 when error occurs during option processing. 00168 */ 00169 int thread_border_router_recursive_dns_server_option_set(int8_t interface_id, uint8_t *recursive_dns_server_option, uint16_t recursive_dns_server_option_len); 00170 00171 /** 00172 * \brief Set DNS server search list (DNSSL) option that is encoded according to RFC6106. 00173 * Setting a new DNSSL will overwrite previous DNSSL option. Set DNSSL will be used 00174 * until it is cleared. 00175 * 00176 * \param interface_id Network interface ID. 00177 * \param dns_search_list_option DNS search list option encoded according to rfc6106, can be NULL to clear existing DNSSL. 00178 * \param search_list_option_len Length of the dns_search_list_option in bytes. 00179 * 00180 * \return 0, Option saved OK. 00181 * \return <0 when error occurs during option processing. 00182 */ 00183 int thread_border_router_dns_search_list_option_set(int8_t interface_id, uint8_t *dns_search_list_option, uint16_t search_list_option_len); 00184 00185 /** 00186 * \brief Callback type for Thread network data TLV registration 00187 * 00188 * \param interface_id Network interface ID. 00189 * \param network_data_tlv Thread Network data TLV as specified in Thread specification. 00190 * \param network_data_tlv_length length of the network data TLV. 00191 */ 00192 typedef void (thread_network_data_tlv_cb)(int8_t interface_id, uint8_t *network_data_tlv, uint16_t network_data_tlv_length); 00193 00194 /** 00195 * \brief Register callback function to receive thread network data TLV in byte array. For the first time the callback 00196 * will be called before returning from the function. Afterwards the callback will be called when there is a change 00197 * to the network data or when the network data is advertised. Application is not allowed to block the callback execution 00198 * and must make a copy of the network data if it is needed afterwards. 00199 * 00200 * Setting nwk_data_cb to NULL will prevent further calls to the callback function. 00201 * 00202 * \param interface_id Network interface ID. 00203 * \param nwk_data_cb callback function to receive network data TLV in byte array as specified in the Thread specification. 00204 * 00205 * \return 0, Callback function registered successfully. 00206 * \return <0 when error occurs during registering the callback function. 00207 */ 00208 int thread_border_router_network_data_callback_register(int8_t interface_id, thread_network_data_tlv_cb* nwk_data_cb); 00209 00210 /** 00211 * Find Prefix TLV from the Network Data TLV byte array. 00212 * 00213 * \param network_data_tlv [IN] Network data TLV in byte array. 00214 * \param network_data_tlv_length [IN] Length of the network data TLV byte array in bytes. 00215 * \param prefix_tlv [IN] pointer to the previous prefix_tlv, NULL if previous TLV not known. 00216 * [OUT] Pointer to the prefix TLV found. 00217 * \param stable [OUT] value set to true if found TLV is stable, false otherwise. 00218 * 00219 * \return Length of the found Prefix TLV 00220 * \return 0 if TLV is empty or no Prefix TLV found. 00221 * \return negative value indicates error in input parameters. 00222 */ 00223 int thread_border_router_prefix_tlv_find(uint8_t* network_data_tlv, uint16_t network_data_tlv_length, uint8_t** prefix_tlv, bool* stable); 00224 00225 /** 00226 * Find Border router TLV from the Network Data TLV (under Prefix TLV) byte array. 00227 * 00228 * \param prefix_tlv [IN] Network data TLV in byte array. 00229 * \param prefix_tlv_length [IN] Length of the Network data TLV byte array in bytes. 00230 * \param border_router_tlv [IN] pointer to the previous Border Router TLV, NULL if not known. 00231 * [OUT] Pointer to the Border Router TLV found. 00232 * \param stable [OUT] value set to true if found TLV is stable, false otherwise 00233 * 00234 * \return Length of the Prefix found 00235 * \return 0 if TLV is empty or no TLV found. 00236 * \return negative value indicates error in input parameters. 00237 */ 00238 int thread_border_router_tlv_find(uint8_t* prefix_tlv, uint16_t prefix_tlv_length, uint8_t** border_router_tlv, bool* stable); 00239 00240 /** 00241 * Find Service TLV from the Network Data TLV byte array. 00242 * 00243 * \param network_data_tlv [IN] Network data TLV in byte array. 00244 * \param network_data_tlv_length [IN] Length of the network data TLV byte array in bytes. 00245 * \param service_tlv [IN] pointer to the previous Service TLV, NULL if previous TLV not known. 00246 * [OUT] Pointer to the Service TLV found. 00247 * \param stable [OUT] value set to true if found TLV is stable, false otherwise. 00248 * 00249 * \return Length of the found Service TLV 00250 * \return 0 if TLV is empty or no Service TLV found. 00251 * \return negative value indicates error in input parameters. 00252 */ 00253 int thread_border_router_service_tlv_find(uint8_t* network_data_tlv, uint16_t network_data_tlv_length, uint8_t** service_tlv, bool* stable); 00254 00255 /** 00256 * Find Server TLV from the Network Data TLV (under Service TLV) byte array. 00257 * 00258 * \param service_tlv [IN] Network data TLV in byte array. 00259 * \param service_tlv_length [IN] Length of the Network data TLV byte array in bytes. 00260 * \param server_tlv [IN] pointer to the previous Server TLV, NULL if not known. 00261 * [OUT] Pointer to the Server TLV found. 00262 * \param stable [OUT] value set to true if found TLV is stable, false otherwise 00263 * 00264 * \return Length of the Prefix found 00265 * \return 0 if TLV is empty or no TLV found. 00266 * \return negative value indicates error in input parameters. 00267 */ 00268 int thread_border_router_server_tlv_find(uint8_t* service_tlv, uint16_t service_tlv_length, uint8_t** server_tlv, bool* stable); 00269 00270 /** 00271 * Determine context ID from the Network Data TLV (under Prefix TLV) byte array. 00272 * 00273 * \param prefix_tlv [IN] Prefix TLV in byte array. 00274 * \param prefix_tlv_length [IN] Length of the Prefix TLV byte array in bytes. 00275 * 00276 * \return The context ID value found 00277 * \return -1 if error in input parameters. 00278 * \return -2 if no context ID value found. 00279 */ 00280 int thread_border_router_prefix_context_id(uint8_t *prefix_tlv, uint16_t prefix_tlv_length); 00281 00282 /** 00283 * Start mDNS responder service. The responder will respond to DNS-SD queries and send announcement when 00284 * Thread network data is updated. 00285 * 00286 * The mDNS responder can be closed by calling thread_border_router_mdns_responder_stop(). Closing the Thread 00287 * network interface will stop the mDNS responder automatically. 00288 * 00289 * \param interface_id interface ID of the Thread network 00290 * \param interface_id_mdns interface where mDNS messaging occurs 00291 * \param service_name mDNS instance name 00292 * 00293 * \return 0 on success 00294 * \return <0 in case of errors 00295 * 00296 */ 00297 int thread_border_router_mdns_responder_start(int8_t interface_id, int8_t interface_id_mdns, const char *service_name); 00298 00299 /** 00300 * Stop mDNS responder service 00301 * 00302 * 00303 * \return 0 on success 00304 * \return <0 in case of errors 00305 * 00306 */ 00307 int thread_border_router_mdns_responder_stop(void); 00308 00309 #endif /* THREAD_BORDER_ROUTER_API_H_ */
Generated on Tue Jul 12 2022 14:49:05 by
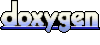