
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
tcpip.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Functions to sync with TCPIP thread 00004 */ 00005 00006 /* 00007 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * This file is part of the lwIP TCP/IP stack. 00033 * 00034 * Author: Adam Dunkels <adam@sics.se> 00035 * 00036 */ 00037 #ifndef LWIP_HDR_TCPIP_H 00038 #define LWIP_HDR_TCPIP_H 00039 00040 #include "lwip/opt.h" 00041 00042 #if !NO_SYS /* don't build if not configured for use in lwipopts.h */ 00043 00044 #include "lwip/err.h" 00045 #include "lwip/timeouts.h" 00046 #include "lwip/netif.h" 00047 00048 #ifdef __cplusplus 00049 extern "C" { 00050 #endif 00051 00052 #if LWIP_TCPIP_CORE_LOCKING 00053 /** The global semaphore to lock the stack. */ 00054 extern sys_mutex_t lock_tcpip_core; 00055 /** Lock lwIP core mutex (needs @ref LWIP_TCPIP_CORE_LOCKING 1) */ 00056 #define LOCK_TCPIP_CORE() sys_mutex_lock(&lock_tcpip_core) 00057 /** Unlock lwIP core mutex (needs @ref LWIP_TCPIP_CORE_LOCKING 1) */ 00058 #define UNLOCK_TCPIP_CORE() sys_mutex_unlock(&lock_tcpip_core) 00059 #else /* LWIP_TCPIP_CORE_LOCKING */ 00060 #define LOCK_TCPIP_CORE() 00061 #define UNLOCK_TCPIP_CORE() 00062 #endif /* LWIP_TCPIP_CORE_LOCKING */ 00063 00064 struct pbuf; 00065 struct netif; 00066 00067 /** Function prototype for the init_done function passed to tcpip_init */ 00068 typedef void (*tcpip_init_done_fn)(void *arg); 00069 /** Function prototype for functions passed to tcpip_callback() */ 00070 typedef void (*tcpip_callback_fn)(void *ctx); 00071 00072 /* Forward declarations */ 00073 struct tcpip_callback_msg; 00074 00075 void tcpip_init(tcpip_init_done_fn tcpip_init_done, void *arg); 00076 00077 err_t tcpip_inpkt(struct pbuf *p, struct netif *inp, netif_input_fn input_fn); 00078 err_t tcpip_input(struct pbuf *p, struct netif *inp); 00079 00080 err_t tcpip_callback_with_block(tcpip_callback_fn function, void *ctx, u8_t block); 00081 /** 00082 * @ingroup lwip_os 00083 * @see tcpip_callback_with_block 00084 */ 00085 #define tcpip_callback(f, ctx) tcpip_callback_with_block(f, ctx, 1) 00086 00087 struct tcpip_callback_msg* tcpip_callbackmsg_new(tcpip_callback_fn function, void *ctx); 00088 void tcpip_callbackmsg_delete(struct tcpip_callback_msg* msg); 00089 err_t tcpip_trycallback(struct tcpip_callback_msg* msg); 00090 00091 /* free pbufs or heap memory from another context without blocking */ 00092 err_t pbuf_free_callback(struct pbuf *p); 00093 err_t mem_free_callback(void *m); 00094 00095 #if LWIP_TCPIP_TIMEOUT && LWIP_TIMERS 00096 err_t tcpip_timeout(u32_t msecs, sys_timeout_handler h, void *arg); 00097 err_t tcpip_untimeout(sys_timeout_handler h, void *arg); 00098 #endif /* LWIP_TCPIP_TIMEOUT && LWIP_TIMERS */ 00099 00100 #ifdef __cplusplus 00101 } 00102 #endif 00103 00104 #endif /* !NO_SYS */ 00105 00106 #endif /* LWIP_HDR_TCPIP_H */
Generated on Tue Jul 12 2022 14:49:04 by
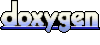