
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
ssl_srv.c
00001 /* 00002 * SSLv3/TLSv1 server-side functions 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 00022 #if !defined(MBEDTLS_CONFIG_FILE) 00023 #include "mbedtls/config.h" 00024 #else 00025 #include MBEDTLS_CONFIG_FILE 00026 #endif 00027 00028 #if defined(MBEDTLS_SSL_SRV_C) 00029 00030 #if defined(MBEDTLS_PLATFORM_C) 00031 #include "mbedtls/platform.h" 00032 #else 00033 #include <stdlib.h> 00034 #define mbedtls_calloc calloc 00035 #define mbedtls_free free 00036 #endif 00037 00038 #include "mbedtls/debug.h" 00039 #include "mbedtls/ssl.h" 00040 #include "mbedtls/ssl_internal.h" 00041 00042 #include <string.h> 00043 00044 #if defined(MBEDTLS_ECP_C) 00045 #include "mbedtls/ecp.h" 00046 #endif 00047 00048 #if defined(MBEDTLS_HAVE_TIME) 00049 #include "mbedtls/platform_time.h" 00050 #endif 00051 00052 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 00053 /* Implementation that should never be optimized out by the compiler */ 00054 static void mbedtls_zeroize( void *v, size_t n ) { 00055 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00056 } 00057 #endif 00058 00059 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) 00060 int mbedtls_ssl_set_client_transport_id( mbedtls_ssl_context *ssl, 00061 const unsigned char *info, 00062 size_t ilen ) 00063 { 00064 if( ssl->conf->endpoint != MBEDTLS_SSL_IS_SERVER ) 00065 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 00066 00067 mbedtls_free( ssl->cli_id ); 00068 00069 if( ( ssl->cli_id = mbedtls_calloc( 1, ilen ) ) == NULL ) 00070 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 00071 00072 memcpy( ssl->cli_id, info, ilen ); 00073 ssl->cli_id_len = ilen; 00074 00075 return( 0 ); 00076 } 00077 00078 void mbedtls_ssl_conf_dtls_cookies( mbedtls_ssl_config *conf, 00079 mbedtls_ssl_cookie_write_t *f_cookie_write, 00080 mbedtls_ssl_cookie_check_t *f_cookie_check, 00081 void *p_cookie ) 00082 { 00083 conf->f_cookie_write = f_cookie_write; 00084 conf->f_cookie_check = f_cookie_check; 00085 conf->p_cookie = p_cookie; 00086 } 00087 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY */ 00088 00089 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 00090 static int ssl_parse_servername_ext( mbedtls_ssl_context *ssl, 00091 const unsigned char *buf, 00092 size_t len ) 00093 { 00094 int ret; 00095 size_t servername_list_size, hostname_len; 00096 const unsigned char *p; 00097 00098 MBEDTLS_SSL_DEBUG_MSG( 3, ( "parse ServerName extension" ) ); 00099 00100 servername_list_size = ( ( buf[0] << 8 ) | ( buf[1] ) ); 00101 if( servername_list_size + 2 != len ) 00102 { 00103 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00104 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00105 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00106 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00107 } 00108 00109 p = buf + 2; 00110 while( servername_list_size > 0 ) 00111 { 00112 hostname_len = ( ( p[1] << 8 ) | p[2] ); 00113 if( hostname_len + 3 > servername_list_size ) 00114 { 00115 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00116 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00117 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00118 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00119 } 00120 00121 if( p[0] == MBEDTLS_TLS_EXT_SERVERNAME_HOSTNAME ) 00122 { 00123 ret = ssl->conf->f_sni( ssl->conf->p_sni, 00124 ssl, p + 3, hostname_len ); 00125 if( ret != 0 ) 00126 { 00127 MBEDTLS_SSL_DEBUG_RET( 1, "ssl_sni_wrapper", ret ); 00128 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00129 MBEDTLS_SSL_ALERT_MSG_UNRECOGNIZED_NAME ); 00130 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00131 } 00132 return( 0 ); 00133 } 00134 00135 servername_list_size -= hostname_len + 3; 00136 p += hostname_len + 3; 00137 } 00138 00139 if( servername_list_size != 0 ) 00140 { 00141 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00142 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00143 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 00144 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00145 } 00146 00147 return( 0 ); 00148 } 00149 #endif /* MBEDTLS_SSL_SERVER_NAME_INDICATION */ 00150 00151 static int ssl_parse_renegotiation_info( mbedtls_ssl_context *ssl, 00152 const unsigned char *buf, 00153 size_t len ) 00154 { 00155 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00156 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00157 { 00158 /* Check verify-data in constant-time. The length OTOH is no secret */ 00159 if( len != 1 + ssl->verify_data_len || 00160 buf[0] != ssl->verify_data_len || 00161 mbedtls_ssl_safer_memcmp( buf + 1, ssl->peer_verify_data, 00162 ssl->verify_data_len ) != 0 ) 00163 { 00164 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-matching renegotiation info" ) ); 00165 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00166 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 00167 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00168 } 00169 } 00170 else 00171 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 00172 { 00173 if( len != 1 || buf[0] != 0x0 ) 00174 { 00175 MBEDTLS_SSL_DEBUG_MSG( 1, ( "non-zero length renegotiation info" ) ); 00176 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00177 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 00178 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00179 } 00180 00181 ssl->secure_renegotiation = MBEDTLS_SSL_SECURE_RENEGOTIATION; 00182 } 00183 00184 return( 0 ); 00185 } 00186 00187 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 00188 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 00189 00190 /* 00191 * Status of the implementation of signature-algorithms extension: 00192 * 00193 * Currently, we are only considering the signature-algorithm extension 00194 * to pick a ciphersuite which allows us to send the ServerKeyExchange 00195 * message with a signature-hash combination that the user allows. 00196 * 00197 * We do *not* check whether all certificates in our certificate 00198 * chain are signed with an allowed signature-hash pair. 00199 * This needs to be done at a later stage. 00200 * 00201 */ 00202 static int ssl_parse_signature_algorithms_ext( mbedtls_ssl_context *ssl, 00203 const unsigned char *buf, 00204 size_t len ) 00205 { 00206 size_t sig_alg_list_size; 00207 00208 const unsigned char *p; 00209 const unsigned char *end = buf + len; 00210 00211 mbedtls_md_type_t md_cur; 00212 mbedtls_pk_type_t sig_cur; 00213 00214 sig_alg_list_size = ( ( buf[0] << 8 ) | ( buf[1] ) ); 00215 if( sig_alg_list_size + 2 != len || 00216 sig_alg_list_size % 2 != 0 ) 00217 { 00218 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00219 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00220 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00221 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00222 } 00223 00224 /* Currently we only guarantee signing the ServerKeyExchange message according 00225 * to the constraints specified in this extension (see above), so it suffices 00226 * to remember only one suitable hash for each possible signature algorithm. 00227 * 00228 * This will change when we also consider certificate signatures, 00229 * in which case we will need to remember the whole signature-hash 00230 * pair list from the extension. 00231 */ 00232 00233 for( p = buf + 2; p < end; p += 2 ) 00234 { 00235 /* Silently ignore unknown signature or hash algorithms. */ 00236 00237 if( ( sig_cur = mbedtls_ssl_pk_alg_from_sig( p[1] ) ) == MBEDTLS_PK_NONE ) 00238 { 00239 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, signature_algorithm ext" 00240 " unknown sig alg encoding %d", p[1] ) ); 00241 continue; 00242 } 00243 00244 /* Check if we support the hash the user proposes */ 00245 md_cur = mbedtls_ssl_md_alg_from_hash( p[0] ); 00246 if( md_cur == MBEDTLS_MD_NONE ) 00247 { 00248 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, signature_algorithm ext:" 00249 " unknown hash alg encoding %d", p[0] ) ); 00250 continue; 00251 } 00252 00253 if( mbedtls_ssl_check_sig_hash( ssl, md_cur ) == 0 ) 00254 { 00255 mbedtls_ssl_sig_hash_set_add( &ssl->handshake->hash_algs, sig_cur, md_cur ); 00256 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, signature_algorithm ext:" 00257 " match sig %d and hash %d", 00258 sig_cur, md_cur ) ); 00259 } 00260 else 00261 { 00262 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, signature_algorithm ext: " 00263 "hash alg %d not supported", md_cur ) ); 00264 } 00265 } 00266 00267 return( 0 ); 00268 } 00269 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 && 00270 MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 00271 00272 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 00273 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00274 static int ssl_parse_supported_elliptic_curves( mbedtls_ssl_context *ssl, 00275 const unsigned char *buf, 00276 size_t len ) 00277 { 00278 size_t list_size, our_size; 00279 const unsigned char *p; 00280 const mbedtls_ecp_curve_info *curve_info, **curves; 00281 00282 list_size = ( ( buf[0] << 8 ) | ( buf[1] ) ); 00283 if( list_size + 2 != len || 00284 list_size % 2 != 0 ) 00285 { 00286 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00287 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00288 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00289 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00290 } 00291 00292 /* Should never happen unless client duplicates the extension */ 00293 if( ssl->handshake->curves != NULL ) 00294 { 00295 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00296 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00297 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00298 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00299 } 00300 00301 /* Don't allow our peer to make us allocate too much memory, 00302 * and leave room for a final 0 */ 00303 our_size = list_size / 2 + 1; 00304 if( our_size > MBEDTLS_ECP_DP_MAX ) 00305 our_size = MBEDTLS_ECP_DP_MAX; 00306 00307 if( ( curves = mbedtls_calloc( our_size, sizeof( *curves ) ) ) == NULL ) 00308 { 00309 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00310 MBEDTLS_SSL_ALERT_MSG_INTERNAL_ERROR ); 00311 return( MBEDTLS_ERR_SSL_ALLOC_FAILED ); 00312 } 00313 00314 ssl->handshake->curves = curves; 00315 00316 p = buf + 2; 00317 while( list_size > 0 && our_size > 1 ) 00318 { 00319 curve_info = mbedtls_ecp_curve_info_from_tls_id( ( p[0] << 8 ) | p[1] ); 00320 00321 if( curve_info != NULL ) 00322 { 00323 *curves++ = curve_info; 00324 our_size--; 00325 } 00326 00327 list_size -= 2; 00328 p += 2; 00329 } 00330 00331 return( 0 ); 00332 } 00333 00334 static int ssl_parse_supported_point_formats( mbedtls_ssl_context *ssl, 00335 const unsigned char *buf, 00336 size_t len ) 00337 { 00338 size_t list_size; 00339 const unsigned char *p; 00340 00341 list_size = buf[0]; 00342 if( list_size + 1 != len ) 00343 { 00344 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00345 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00346 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00347 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00348 } 00349 00350 p = buf + 1; 00351 while( list_size > 0 ) 00352 { 00353 if( p[0] == MBEDTLS_ECP_PF_UNCOMPRESSED || 00354 p[0] == MBEDTLS_ECP_PF_COMPRESSED ) 00355 { 00356 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) 00357 ssl->handshake->ecdh_ctx.point_format = p[0]; 00358 #endif 00359 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00360 ssl->handshake->ecjpake_ctx.point_format = p[0]; 00361 #endif 00362 MBEDTLS_SSL_DEBUG_MSG( 4, ( "point format selected: %d", p[0] ) ); 00363 return( 0 ); 00364 } 00365 00366 list_size--; 00367 p++; 00368 } 00369 00370 return( 0 ); 00371 } 00372 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || 00373 MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 00374 00375 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00376 static int ssl_parse_ecjpake_kkpp( mbedtls_ssl_context *ssl, 00377 const unsigned char *buf, 00378 size_t len ) 00379 { 00380 int ret; 00381 00382 if( mbedtls_ecjpake_check( &ssl->handshake->ecjpake_ctx ) != 0 ) 00383 { 00384 MBEDTLS_SSL_DEBUG_MSG( 3, ( "skip ecjpake kkpp extension" ) ); 00385 return( 0 ); 00386 } 00387 00388 if( ( ret = mbedtls_ecjpake_read_round_one( &ssl->handshake->ecjpake_ctx, 00389 buf, len ) ) != 0 ) 00390 { 00391 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_read_round_one", ret ); 00392 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00393 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 00394 return( ret ); 00395 } 00396 00397 /* Only mark the extension as OK when we're sure it is */ 00398 ssl->handshake->cli_exts |= MBEDTLS_TLS_EXT_ECJPAKE_KKPP_OK; 00399 00400 return( 0 ); 00401 } 00402 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 00403 00404 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 00405 static int ssl_parse_max_fragment_length_ext( mbedtls_ssl_context *ssl, 00406 const unsigned char *buf, 00407 size_t len ) 00408 { 00409 if( len != 1 || buf[0] >= MBEDTLS_SSL_MAX_FRAG_LEN_INVALID ) 00410 { 00411 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00412 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00413 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 00414 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00415 } 00416 00417 ssl->session_negotiate->mfl_code = buf[0]; 00418 00419 return( 0 ); 00420 } 00421 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 00422 00423 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 00424 static int ssl_parse_truncated_hmac_ext( mbedtls_ssl_context *ssl, 00425 const unsigned char *buf, 00426 size_t len ) 00427 { 00428 if( len != 0 ) 00429 { 00430 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00431 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00432 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00433 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00434 } 00435 00436 ((void) buf); 00437 00438 if( ssl->conf->trunc_hmac == MBEDTLS_SSL_TRUNC_HMAC_ENABLED ) 00439 ssl->session_negotiate->trunc_hmac = MBEDTLS_SSL_TRUNC_HMAC_ENABLED; 00440 00441 return( 0 ); 00442 } 00443 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 00444 00445 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 00446 static int ssl_parse_encrypt_then_mac_ext( mbedtls_ssl_context *ssl, 00447 const unsigned char *buf, 00448 size_t len ) 00449 { 00450 if( len != 0 ) 00451 { 00452 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00453 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00454 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00455 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00456 } 00457 00458 ((void) buf); 00459 00460 if( ssl->conf->encrypt_then_mac == MBEDTLS_SSL_ETM_ENABLED && 00461 ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_0 ) 00462 { 00463 ssl->session_negotiate->encrypt_then_mac = MBEDTLS_SSL_ETM_ENABLED; 00464 } 00465 00466 return( 0 ); 00467 } 00468 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 00469 00470 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 00471 static int ssl_parse_extended_ms_ext( mbedtls_ssl_context *ssl, 00472 const unsigned char *buf, 00473 size_t len ) 00474 { 00475 if( len != 0 ) 00476 { 00477 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00478 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00479 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00480 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00481 } 00482 00483 ((void) buf); 00484 00485 if( ssl->conf->extended_ms == MBEDTLS_SSL_EXTENDED_MS_ENABLED && 00486 ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_0 ) 00487 { 00488 ssl->handshake->extended_ms = MBEDTLS_SSL_EXTENDED_MS_ENABLED; 00489 } 00490 00491 return( 0 ); 00492 } 00493 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 00494 00495 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 00496 static int ssl_parse_session_ticket_ext( mbedtls_ssl_context *ssl, 00497 unsigned char *buf, 00498 size_t len ) 00499 { 00500 int ret; 00501 mbedtls_ssl_session session; 00502 00503 mbedtls_ssl_session_init( &session ); 00504 00505 if( ssl->conf->f_ticket_parse == NULL || 00506 ssl->conf->f_ticket_write == NULL ) 00507 { 00508 return( 0 ); 00509 } 00510 00511 /* Remember the client asked us to send a new ticket */ 00512 ssl->handshake->new_session_ticket = 1; 00513 00514 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket length: %d", len ) ); 00515 00516 if( len == 0 ) 00517 return( 0 ); 00518 00519 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00520 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00521 { 00522 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket rejected: renegotiating" ) ); 00523 return( 0 ); 00524 } 00525 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 00526 00527 /* 00528 * Failures are ok: just ignore the ticket and proceed. 00529 */ 00530 if( ( ret = ssl->conf->f_ticket_parse( ssl->conf->p_ticket, &session, 00531 buf, len ) ) != 0 ) 00532 { 00533 mbedtls_ssl_session_free( &session ); 00534 00535 if( ret == MBEDTLS_ERR_SSL_INVALID_MAC ) 00536 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket is not authentic" ) ); 00537 else if( ret == MBEDTLS_ERR_SSL_SESSION_TICKET_EXPIRED ) 00538 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ticket is expired" ) ); 00539 else 00540 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_ticket_parse", ret ); 00541 00542 return( 0 ); 00543 } 00544 00545 /* 00546 * Keep the session ID sent by the client, since we MUST send it back to 00547 * inform them we're accepting the ticket (RFC 5077 section 3.4) 00548 */ 00549 session.id_len = ssl->session_negotiate->id_len; 00550 memcpy( &session.id, ssl->session_negotiate->id, session.id_len ); 00551 00552 mbedtls_ssl_session_free( ssl->session_negotiate ); 00553 memcpy( ssl->session_negotiate, &session, sizeof( mbedtls_ssl_session ) ); 00554 00555 /* Zeroize instead of free as we copied the content */ 00556 mbedtls_zeroize( &session, sizeof( mbedtls_ssl_session ) ); 00557 00558 MBEDTLS_SSL_DEBUG_MSG( 3, ( "session successfully restored from ticket" ) ); 00559 00560 ssl->handshake->resume = 1; 00561 00562 /* Don't send a new ticket after all, this one is OK */ 00563 ssl->handshake->new_session_ticket = 0; 00564 00565 return( 0 ); 00566 } 00567 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 00568 00569 #if defined(MBEDTLS_SSL_ALPN) 00570 static int ssl_parse_alpn_ext( mbedtls_ssl_context *ssl, 00571 const unsigned char *buf, size_t len ) 00572 { 00573 size_t list_len, cur_len, ours_len; 00574 const unsigned char *theirs, *start, *end; 00575 const char **ours; 00576 00577 /* If ALPN not configured, just ignore the extension */ 00578 if( ssl->conf->alpn_list == NULL ) 00579 return( 0 ); 00580 00581 /* 00582 * opaque ProtocolName<1..2^8-1>; 00583 * 00584 * struct { 00585 * ProtocolName protocol_name_list<2..2^16-1> 00586 * } ProtocolNameList; 00587 */ 00588 00589 /* Min length is 2 (list_len) + 1 (name_len) + 1 (name) */ 00590 if( len < 4 ) 00591 { 00592 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00593 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00594 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00595 } 00596 00597 list_len = ( buf[0] << 8 ) | buf[1]; 00598 if( list_len != len - 2 ) 00599 { 00600 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00601 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 00602 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00603 } 00604 00605 /* 00606 * Use our order of preference 00607 */ 00608 start = buf + 2; 00609 end = buf + len; 00610 for( ours = ssl->conf->alpn_list; *ours != NULL; ours++ ) 00611 { 00612 ours_len = strlen( *ours ); 00613 for( theirs = start; theirs != end; theirs += cur_len ) 00614 { 00615 /* If the list is well formed, we should get equality first */ 00616 if( theirs > end ) 00617 { 00618 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00619 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 00620 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00621 } 00622 00623 cur_len = *theirs++; 00624 00625 /* Empty strings MUST NOT be included */ 00626 if( cur_len == 0 ) 00627 { 00628 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00629 MBEDTLS_SSL_ALERT_MSG_ILLEGAL_PARAMETER ); 00630 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00631 } 00632 00633 if( cur_len == ours_len && 00634 memcmp( theirs, *ours, cur_len ) == 0 ) 00635 { 00636 ssl->alpn_chosen = *ours; 00637 return( 0 ); 00638 } 00639 } 00640 } 00641 00642 /* If we get there, no match was found */ 00643 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00644 MBEDTLS_SSL_ALERT_MSG_NO_APPLICATION_PROTOCOL ); 00645 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00646 } 00647 #endif /* MBEDTLS_SSL_ALPN */ 00648 00649 /* 00650 * Auxiliary functions for ServerHello parsing and related actions 00651 */ 00652 00653 #if defined(MBEDTLS_X509_CRT_PARSE_C) 00654 /* 00655 * Return 0 if the given key uses one of the acceptable curves, -1 otherwise 00656 */ 00657 #if defined(MBEDTLS_ECDSA_C) 00658 static int ssl_check_key_curve( mbedtls_pk_context *pk, 00659 const mbedtls_ecp_curve_info **curves ) 00660 { 00661 const mbedtls_ecp_curve_info **crv = curves; 00662 mbedtls_ecp_group_id grp_id = mbedtls_pk_ec( *pk )->grp .id ; 00663 00664 while( *crv != NULL ) 00665 { 00666 if( (*crv)->grp_id == grp_id ) 00667 return( 0 ); 00668 crv++; 00669 } 00670 00671 return( -1 ); 00672 } 00673 #endif /* MBEDTLS_ECDSA_C */ 00674 00675 /* 00676 * Try picking a certificate for this ciphersuite, 00677 * return 0 on success and -1 on failure. 00678 */ 00679 static int ssl_pick_cert( mbedtls_ssl_context *ssl, 00680 const mbedtls_ssl_ciphersuite_t * ciphersuite_info ) 00681 { 00682 mbedtls_ssl_key_cert *cur, *list, *fallback = NULL; 00683 mbedtls_pk_type_t pk_alg = 00684 mbedtls_ssl_get_ciphersuite_sig_pk_alg( ciphersuite_info ); 00685 uint32_t flags; 00686 00687 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 00688 if( ssl->handshake->sni_key_cert != NULL ) 00689 list = ssl->handshake->sni_key_cert; 00690 else 00691 #endif 00692 list = ssl->conf->key_cert; 00693 00694 if( pk_alg == MBEDTLS_PK_NONE ) 00695 return( 0 ); 00696 00697 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite requires certificate" ) ); 00698 00699 if( list == NULL ) 00700 { 00701 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server has no certificate" ) ); 00702 return( -1 ); 00703 } 00704 00705 for( cur = list; cur != NULL; cur = cur->next ) 00706 { 00707 MBEDTLS_SSL_DEBUG_CRT( 3, "candidate certificate chain, certificate", 00708 cur->cert ); 00709 00710 if( ! mbedtls_pk_can_do( cur->key, pk_alg ) ) 00711 { 00712 MBEDTLS_SSL_DEBUG_MSG( 3, ( "certificate mismatch: key type" ) ); 00713 continue; 00714 } 00715 00716 /* 00717 * This avoids sending the client a cert it'll reject based on 00718 * keyUsage or other extensions. 00719 * 00720 * It also allows the user to provision different certificates for 00721 * different uses based on keyUsage, eg if they want to avoid signing 00722 * and decrypting with the same RSA key. 00723 */ 00724 if( mbedtls_ssl_check_cert_usage( cur->cert, ciphersuite_info, 00725 MBEDTLS_SSL_IS_SERVER, &flags ) != 0 ) 00726 { 00727 MBEDTLS_SSL_DEBUG_MSG( 3, ( "certificate mismatch: " 00728 "(extended) key usage extension" ) ); 00729 continue; 00730 } 00731 00732 #if defined(MBEDTLS_ECDSA_C) 00733 if( pk_alg == MBEDTLS_PK_ECDSA && 00734 ssl_check_key_curve( cur->key, ssl->handshake->curves ) != 0 ) 00735 { 00736 MBEDTLS_SSL_DEBUG_MSG( 3, ( "certificate mismatch: elliptic curve" ) ); 00737 continue; 00738 } 00739 #endif 00740 00741 /* 00742 * Try to select a SHA-1 certificate for pre-1.2 clients, but still 00743 * present them a SHA-higher cert rather than failing if it's the only 00744 * one we got that satisfies the other conditions. 00745 */ 00746 if( ssl->minor_ver < MBEDTLS_SSL_MINOR_VERSION_3 && 00747 cur->cert->sig_md != MBEDTLS_MD_SHA1 ) 00748 { 00749 if( fallback == NULL ) 00750 fallback = cur; 00751 { 00752 MBEDTLS_SSL_DEBUG_MSG( 3, ( "certificate not preferred: " 00753 "sha-2 with pre-TLS 1.2 client" ) ); 00754 continue; 00755 } 00756 } 00757 00758 /* If we get there, we got a winner */ 00759 break; 00760 } 00761 00762 if( cur == NULL ) 00763 cur = fallback; 00764 00765 /* Do not update ssl->handshake->key_cert unless there is a match */ 00766 if( cur != NULL ) 00767 { 00768 ssl->handshake->key_cert = cur; 00769 MBEDTLS_SSL_DEBUG_CRT( 3, "selected certificate chain, certificate", 00770 ssl->handshake->key_cert->cert ); 00771 return( 0 ); 00772 } 00773 00774 return( -1 ); 00775 } 00776 #endif /* MBEDTLS_X509_CRT_PARSE_C */ 00777 00778 /* 00779 * Check if a given ciphersuite is suitable for use with our config/keys/etc 00780 * Sets ciphersuite_info only if the suite matches. 00781 */ 00782 static int ssl_ciphersuite_match( mbedtls_ssl_context *ssl, int suite_id, 00783 const mbedtls_ssl_ciphersuite_t **ciphersuite_info ) 00784 { 00785 const mbedtls_ssl_ciphersuite_t *suite_info; 00786 00787 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 00788 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 00789 mbedtls_pk_type_t sig_type; 00790 #endif 00791 00792 suite_info = mbedtls_ssl_ciphersuite_from_id( suite_id ); 00793 if( suite_info == NULL ) 00794 { 00795 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 00796 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 00797 } 00798 00799 MBEDTLS_SSL_DEBUG_MSG( 3, ( "trying ciphersuite: %s", suite_info->name ) ); 00800 00801 if( suite_info->min_minor_ver > ssl->minor_ver || 00802 suite_info->max_minor_ver < ssl->minor_ver ) 00803 { 00804 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: version" ) ); 00805 return( 0 ); 00806 } 00807 00808 #if defined(MBEDTLS_SSL_PROTO_DTLS) 00809 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 00810 ( suite_info->flags & MBEDTLS_CIPHERSUITE_NODTLS ) ) 00811 return( 0 ); 00812 #endif 00813 00814 #if defined(MBEDTLS_ARC4_C) 00815 if( ssl->conf->arc4_disabled == MBEDTLS_SSL_ARC4_DISABLED && 00816 suite_info->cipher == MBEDTLS_CIPHER_ARC4_128 ) 00817 { 00818 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: rc4" ) ); 00819 return( 0 ); 00820 } 00821 #endif 00822 00823 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 00824 if( suite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE && 00825 ( ssl->handshake->cli_exts & MBEDTLS_TLS_EXT_ECJPAKE_KKPP_OK ) == 0 ) 00826 { 00827 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: ecjpake " 00828 "not configured or ext missing" ) ); 00829 return( 0 ); 00830 } 00831 #endif 00832 00833 00834 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) 00835 if( mbedtls_ssl_ciphersuite_uses_ec( suite_info ) && 00836 ( ssl->handshake->curves == NULL || 00837 ssl->handshake->curves[0] == NULL ) ) 00838 { 00839 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: " 00840 "no common elliptic curve" ) ); 00841 return( 0 ); 00842 } 00843 #endif 00844 00845 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 00846 /* If the ciphersuite requires a pre-shared key and we don't 00847 * have one, skip it now rather than failing later */ 00848 if( mbedtls_ssl_ciphersuite_uses_psk( suite_info ) && 00849 ssl->conf->f_psk == NULL && 00850 ( ssl->conf->psk == NULL || ssl->conf->psk_identity == NULL || 00851 ssl->conf->psk_identity_len == 0 || ssl->conf->psk_len == 0 ) ) 00852 { 00853 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: no pre-shared key" ) ); 00854 return( 0 ); 00855 } 00856 #endif 00857 00858 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 00859 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 00860 /* If the ciphersuite requires signing, check whether 00861 * a suitable hash algorithm is present. */ 00862 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 00863 { 00864 sig_type = mbedtls_ssl_get_ciphersuite_sig_alg( suite_info ); 00865 if( sig_type != MBEDTLS_PK_NONE && 00866 mbedtls_ssl_sig_hash_set_find( &ssl->handshake->hash_algs, sig_type ) == MBEDTLS_MD_NONE ) 00867 { 00868 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: no suitable hash algorithm " 00869 "for signature algorithm %d", sig_type ) ); 00870 return( 0 ); 00871 } 00872 } 00873 00874 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 && 00875 MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 00876 00877 #if defined(MBEDTLS_X509_CRT_PARSE_C) 00878 /* 00879 * Final check: if ciphersuite requires us to have a 00880 * certificate/key of a particular type: 00881 * - select the appropriate certificate if we have one, or 00882 * - try the next ciphersuite if we don't 00883 * This must be done last since we modify the key_cert list. 00884 */ 00885 if( ssl_pick_cert( ssl, suite_info ) != 0 ) 00886 { 00887 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciphersuite mismatch: " 00888 "no suitable certificate" ) ); 00889 return( 0 ); 00890 } 00891 #endif 00892 00893 *ciphersuite_info = suite_info; 00894 return( 0 ); 00895 } 00896 00897 #if defined(MBEDTLS_SSL_SRV_SUPPORT_SSLV2_CLIENT_HELLO) 00898 static int ssl_parse_client_hello_v2( mbedtls_ssl_context *ssl ) 00899 { 00900 int ret, got_common_suite; 00901 unsigned int i, j; 00902 size_t n; 00903 unsigned int ciph_len, sess_len, chal_len; 00904 unsigned char *buf, *p; 00905 const int *ciphersuites; 00906 const mbedtls_ssl_ciphersuite_t *ciphersuite_info; 00907 00908 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse client hello v2" ) ); 00909 00910 #if defined(MBEDTLS_SSL_RENEGOTIATION) 00911 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 00912 { 00913 MBEDTLS_SSL_DEBUG_MSG( 1, ( "client hello v2 illegal for renegotiation" ) ); 00914 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00915 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 00916 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00917 } 00918 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 00919 00920 buf = ssl->in_hdr; 00921 00922 MBEDTLS_SSL_DEBUG_BUF( 4, "record header", buf, 5 ); 00923 00924 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v2, message type: %d", 00925 buf[2] ) ); 00926 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v2, message len.: %d", 00927 ( ( buf[0] & 0x7F ) << 8 ) | buf[1] ) ); 00928 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v2, max. version: [%d:%d]", 00929 buf[3], buf[4] ) ); 00930 00931 /* 00932 * SSLv2 Client Hello 00933 * 00934 * Record layer: 00935 * 0 . 1 message length 00936 * 00937 * SSL layer: 00938 * 2 . 2 message type 00939 * 3 . 4 protocol version 00940 */ 00941 if( buf[2] != MBEDTLS_SSL_HS_CLIENT_HELLO || 00942 buf[3] != MBEDTLS_SSL_MAJOR_VERSION_3 ) 00943 { 00944 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00945 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00946 } 00947 00948 n = ( ( buf[0] << 8 ) | buf[1] ) & 0x7FFF; 00949 00950 if( n < 17 || n > 512 ) 00951 { 00952 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 00953 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 00954 } 00955 00956 ssl->major_ver = MBEDTLS_SSL_MAJOR_VERSION_3; 00957 ssl->minor_ver = ( buf[4] <= ssl->conf->max_minor_ver ) 00958 ? buf[4] : ssl->conf->max_minor_ver; 00959 00960 if( ssl->minor_ver < ssl->conf->min_minor_ver ) 00961 { 00962 MBEDTLS_SSL_DEBUG_MSG( 1, ( "client only supports ssl smaller than minimum" 00963 " [%d:%d] < [%d:%d]", 00964 ssl->major_ver, ssl->minor_ver, 00965 ssl->conf->min_major_ver, ssl->conf->min_minor_ver ) ); 00966 00967 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 00968 MBEDTLS_SSL_ALERT_MSG_PROTOCOL_VERSION ); 00969 return( MBEDTLS_ERR_SSL_BAD_HS_PROTOCOL_VERSION ); 00970 } 00971 00972 ssl->handshake->max_major_ver = buf[3]; 00973 ssl->handshake->max_minor_ver = buf[4]; 00974 00975 if( ( ret = mbedtls_ssl_fetch_input( ssl, 2 + n ) ) != 0 ) 00976 { 00977 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_fetch_input", ret ); 00978 return( ret ); 00979 } 00980 00981 ssl->handshake->update_checksum( ssl, buf + 2, n ); 00982 00983 buf = ssl->in_msg; 00984 n = ssl->in_left - 5; 00985 00986 /* 00987 * 0 . 1 ciphersuitelist length 00988 * 2 . 3 session id length 00989 * 4 . 5 challenge length 00990 * 6 . .. ciphersuitelist 00991 * .. . .. session id 00992 * .. . .. challenge 00993 */ 00994 MBEDTLS_SSL_DEBUG_BUF( 4, "record contents", buf, n ); 00995 00996 ciph_len = ( buf[0] << 8 ) | buf[1]; 00997 sess_len = ( buf[2] << 8 ) | buf[3]; 00998 chal_len = ( buf[4] << 8 ) | buf[5]; 00999 01000 MBEDTLS_SSL_DEBUG_MSG( 3, ( "ciph_len: %d, sess_len: %d, chal_len: %d", 01001 ciph_len, sess_len, chal_len ) ); 01002 01003 /* 01004 * Make sure each parameter length is valid 01005 */ 01006 if( ciph_len < 3 || ( ciph_len % 3 ) != 0 ) 01007 { 01008 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01009 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01010 } 01011 01012 if( sess_len > 32 ) 01013 { 01014 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01015 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01016 } 01017 01018 if( chal_len < 8 || chal_len > 32 ) 01019 { 01020 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01021 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01022 } 01023 01024 if( n != 6 + ciph_len + sess_len + chal_len ) 01025 { 01026 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01027 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01028 } 01029 01030 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, ciphersuitelist", 01031 buf + 6, ciph_len ); 01032 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, session id", 01033 buf + 6 + ciph_len, sess_len ); 01034 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, challenge", 01035 buf + 6 + ciph_len + sess_len, chal_len ); 01036 01037 p = buf + 6 + ciph_len; 01038 ssl->session_negotiate->id_len = sess_len; 01039 memset( ssl->session_negotiate->id, 0, 01040 sizeof( ssl->session_negotiate->id ) ); 01041 memcpy( ssl->session_negotiate->id, p, ssl->session_negotiate->id_len ); 01042 01043 p += sess_len; 01044 memset( ssl->handshake->randbytes, 0, 64 ); 01045 memcpy( ssl->handshake->randbytes + 32 - chal_len, p, chal_len ); 01046 01047 /* 01048 * Check for TLS_EMPTY_RENEGOTIATION_INFO_SCSV 01049 */ 01050 for( i = 0, p = buf + 6; i < ciph_len; i += 3, p += 3 ) 01051 { 01052 if( p[0] == 0 && p[1] == 0 && p[2] == MBEDTLS_SSL_EMPTY_RENEGOTIATION_INFO ) 01053 { 01054 MBEDTLS_SSL_DEBUG_MSG( 3, ( "received TLS_EMPTY_RENEGOTIATION_INFO " ) ); 01055 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01056 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 01057 { 01058 MBEDTLS_SSL_DEBUG_MSG( 1, ( "received RENEGOTIATION SCSV " 01059 "during renegotiation" ) ); 01060 01061 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01062 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01063 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01064 } 01065 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 01066 ssl->secure_renegotiation = MBEDTLS_SSL_SECURE_RENEGOTIATION; 01067 break; 01068 } 01069 } 01070 01071 #if defined(MBEDTLS_SSL_FALLBACK_SCSV) 01072 for( i = 0, p = buf + 6; i < ciph_len; i += 3, p += 3 ) 01073 { 01074 if( p[0] == 0 && 01075 p[1] == (unsigned char)( ( MBEDTLS_SSL_FALLBACK_SCSV_VALUE >> 8 ) & 0xff ) && 01076 p[2] == (unsigned char)( ( MBEDTLS_SSL_FALLBACK_SCSV_VALUE ) & 0xff ) ) 01077 { 01078 MBEDTLS_SSL_DEBUG_MSG( 3, ( "received FALLBACK_SCSV" ) ); 01079 01080 if( ssl->minor_ver < ssl->conf->max_minor_ver ) 01081 { 01082 MBEDTLS_SSL_DEBUG_MSG( 1, ( "inapropriate fallback" ) ); 01083 01084 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01085 MBEDTLS_SSL_ALERT_MSG_INAPROPRIATE_FALLBACK ); 01086 01087 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01088 } 01089 01090 break; 01091 } 01092 } 01093 #endif /* MBEDTLS_SSL_FALLBACK_SCSV */ 01094 01095 got_common_suite = 0; 01096 ciphersuites = ssl->conf->ciphersuite_list[ssl->minor_ver]; 01097 ciphersuite_info = NULL; 01098 #if defined(MBEDTLS_SSL_SRV_RESPECT_CLIENT_PREFERENCE) 01099 for( j = 0, p = buf + 6; j < ciph_len; j += 3, p += 3 ) 01100 for( i = 0; ciphersuites[i] != 0; i++ ) 01101 #else 01102 for( i = 0; ciphersuites[i] != 0; i++ ) 01103 for( j = 0, p = buf + 6; j < ciph_len; j += 3, p += 3 ) 01104 #endif 01105 { 01106 if( p[0] != 0 || 01107 p[1] != ( ( ciphersuites[i] >> 8 ) & 0xFF ) || 01108 p[2] != ( ( ciphersuites[i] ) & 0xFF ) ) 01109 continue; 01110 01111 got_common_suite = 1; 01112 01113 if( ( ret = ssl_ciphersuite_match( ssl, ciphersuites[i], 01114 &ciphersuite_info ) ) != 0 ) 01115 return( ret ); 01116 01117 if( ciphersuite_info != NULL ) 01118 goto have_ciphersuite_v2; 01119 } 01120 01121 if( got_common_suite ) 01122 { 01123 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got ciphersuites in common, " 01124 "but none of them usable" ) ); 01125 return( MBEDTLS_ERR_SSL_NO_USABLE_CIPHERSUITE ); 01126 } 01127 else 01128 { 01129 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no ciphersuites in common" ) ); 01130 return( MBEDTLS_ERR_SSL_NO_CIPHER_CHOSEN ); 01131 } 01132 01133 have_ciphersuite_v2: 01134 MBEDTLS_SSL_DEBUG_MSG( 2, ( "selected ciphersuite: %s", ciphersuite_info->name ) ); 01135 01136 ssl->session_negotiate->ciphersuite = ciphersuites[i]; 01137 ssl->transform_negotiate->ciphersuite_info = ciphersuite_info; 01138 01139 /* 01140 * SSLv2 Client Hello relevant renegotiation security checks 01141 */ 01142 if( ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01143 ssl->conf->allow_legacy_renegotiation == MBEDTLS_SSL_LEGACY_BREAK_HANDSHAKE ) 01144 { 01145 MBEDTLS_SSL_DEBUG_MSG( 1, ( "legacy renegotiation, breaking off handshake" ) ); 01146 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01147 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01148 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01149 } 01150 01151 ssl->in_left = 0; 01152 ssl->state++; 01153 01154 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse client hello v2" ) ); 01155 01156 return( 0 ); 01157 } 01158 #endif /* MBEDTLS_SSL_SRV_SUPPORT_SSLV2_CLIENT_HELLO */ 01159 01160 /* This function doesn't alert on errors that happen early during 01161 ClientHello parsing because they might indicate that the client is 01162 not talking SSL/TLS at all and would not understand our alert. */ 01163 static int ssl_parse_client_hello( mbedtls_ssl_context *ssl ) 01164 { 01165 int ret, got_common_suite; 01166 size_t i, j; 01167 size_t ciph_offset, comp_offset, ext_offset; 01168 size_t msg_len, ciph_len, sess_len, comp_len, ext_len; 01169 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01170 size_t cookie_offset, cookie_len; 01171 #endif 01172 unsigned char *buf, *p, *ext; 01173 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01174 int renegotiation_info_seen = 0; 01175 #endif 01176 int handshake_failure = 0; 01177 const int *ciphersuites; 01178 const mbedtls_ssl_ciphersuite_t *ciphersuite_info; 01179 int major, minor; 01180 01181 /* If there is no signature-algorithm extension present, 01182 * we need to fall back to the default values for allowed 01183 * signature-hash pairs. */ 01184 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 01185 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 01186 int sig_hash_alg_ext_present = 0; 01187 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 && 01188 MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 01189 01190 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse client hello" ) ); 01191 01192 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 01193 read_record_header: 01194 #endif 01195 /* 01196 * If renegotiating, then the input was read with mbedtls_ssl_read_record(), 01197 * otherwise read it ourselves manually in order to support SSLv2 01198 * ClientHello, which doesn't use the same record layer format. 01199 */ 01200 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01201 if( ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE ) 01202 #endif 01203 { 01204 if( ( ret = mbedtls_ssl_fetch_input( ssl, 5 ) ) != 0 ) 01205 { 01206 /* No alert on a read error. */ 01207 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_fetch_input", ret ); 01208 return( ret ); 01209 } 01210 } 01211 01212 buf = ssl->in_hdr; 01213 01214 #if defined(MBEDTLS_SSL_SRV_SUPPORT_SSLV2_CLIENT_HELLO) 01215 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01216 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_STREAM ) 01217 #endif 01218 if( ( buf[0] & 0x80 ) != 0 ) 01219 return( ssl_parse_client_hello_v2( ssl ) ); 01220 #endif 01221 01222 MBEDTLS_SSL_DEBUG_BUF( 4, "record header", buf, mbedtls_ssl_hdr_len( ssl ) ); 01223 01224 /* 01225 * SSLv3/TLS Client Hello 01226 * 01227 * Record layer: 01228 * 0 . 0 message type 01229 * 1 . 2 protocol version 01230 * 3 . 11 DTLS: epoch + record sequence number 01231 * 3 . 4 message length 01232 */ 01233 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, message type: %d", 01234 buf[0] ) ); 01235 01236 if( buf[0] != MBEDTLS_SSL_MSG_HANDSHAKE ) 01237 { 01238 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01239 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01240 } 01241 01242 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, message len.: %d", 01243 ( ssl->in_len[0] << 8 ) | ssl->in_len[1] ) ); 01244 01245 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, protocol version: [%d:%d]", 01246 buf[1], buf[2] ) ); 01247 01248 mbedtls_ssl_read_version( &major, &minor, ssl->conf->transport, buf + 1 ); 01249 01250 /* According to RFC 5246 Appendix E.1, the version here is typically 01251 * "{03,00}, the lowest version number supported by the client, [or] the 01252 * value of ClientHello.client_version", so the only meaningful check here 01253 * is the major version shouldn't be less than 3 */ 01254 if( major < MBEDTLS_SSL_MAJOR_VERSION_3 ) 01255 { 01256 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01257 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01258 } 01259 01260 /* For DTLS if this is the initial handshake, remember the client sequence 01261 * number to use it in our next message (RFC 6347 4.2.1) */ 01262 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01263 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM 01264 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01265 && ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE 01266 #endif 01267 ) 01268 { 01269 /* Epoch should be 0 for initial handshakes */ 01270 if( ssl->in_ctr[0] != 0 || ssl->in_ctr[1] != 0 ) 01271 { 01272 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01273 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01274 } 01275 01276 memcpy( ssl->out_ctr + 2, ssl->in_ctr + 2, 6 ); 01277 01278 #if defined(MBEDTLS_SSL_DTLS_ANTI_REPLAY) 01279 if( mbedtls_ssl_dtls_replay_check( ssl ) != 0 ) 01280 { 01281 MBEDTLS_SSL_DEBUG_MSG( 1, ( "replayed record, discarding" ) ); 01282 ssl->next_record_offset = 0; 01283 ssl->in_left = 0; 01284 goto read_record_header; 01285 } 01286 01287 /* No MAC to check yet, so we can update right now */ 01288 mbedtls_ssl_dtls_replay_update( ssl ); 01289 #endif 01290 } 01291 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 01292 01293 msg_len = ( ssl->in_len[0] << 8 ) | ssl->in_len[1]; 01294 01295 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01296 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 01297 { 01298 /* Set by mbedtls_ssl_read_record() */ 01299 msg_len = ssl->in_hslen; 01300 } 01301 else 01302 #endif 01303 { 01304 if( msg_len > MBEDTLS_SSL_MAX_CONTENT_LEN ) 01305 { 01306 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01307 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01308 } 01309 01310 if( ( ret = mbedtls_ssl_fetch_input( ssl, 01311 mbedtls_ssl_hdr_len( ssl ) + msg_len ) ) != 0 ) 01312 { 01313 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_fetch_input", ret ); 01314 return( ret ); 01315 } 01316 01317 /* Done reading this record, get ready for the next one */ 01318 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01319 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01320 ssl->next_record_offset = msg_len + mbedtls_ssl_hdr_len( ssl ); 01321 else 01322 #endif 01323 ssl->in_left = 0; 01324 } 01325 01326 buf = ssl->in_msg; 01327 01328 MBEDTLS_SSL_DEBUG_BUF( 4, "record contents", buf, msg_len ); 01329 01330 ssl->handshake->update_checksum( ssl, buf, msg_len ); 01331 01332 /* 01333 * Handshake layer: 01334 * 0 . 0 handshake type 01335 * 1 . 3 handshake length 01336 * 4 . 5 DTLS only: message seqence number 01337 * 6 . 8 DTLS only: fragment offset 01338 * 9 . 11 DTLS only: fragment length 01339 */ 01340 if( msg_len < mbedtls_ssl_hs_hdr_len( ssl ) ) 01341 { 01342 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01343 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01344 } 01345 01346 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, handshake type: %d", buf[0] ) ); 01347 01348 if( buf[0] != MBEDTLS_SSL_HS_CLIENT_HELLO ) 01349 { 01350 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01351 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01352 } 01353 01354 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, handshake len.: %d", 01355 ( buf[1] << 16 ) | ( buf[2] << 8 ) | buf[3] ) ); 01356 01357 /* We don't support fragmentation of ClientHello (yet?) */ 01358 if( buf[1] != 0 || 01359 msg_len != mbedtls_ssl_hs_hdr_len( ssl ) + ( ( buf[2] << 8 ) | buf[3] ) ) 01360 { 01361 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01362 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01363 } 01364 01365 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01366 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01367 { 01368 /* 01369 * Copy the client's handshake message_seq on initial handshakes, 01370 * check sequence number on renego. 01371 */ 01372 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01373 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 01374 { 01375 /* This couldn't be done in ssl_prepare_handshake_record() */ 01376 unsigned int cli_msg_seq = ( ssl->in_msg[4] << 8 ) | 01377 ssl->in_msg[5]; 01378 01379 if( cli_msg_seq != ssl->handshake->in_msg_seq ) 01380 { 01381 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message_seq: " 01382 "%d (expected %d)", cli_msg_seq, 01383 ssl->handshake->in_msg_seq ) ); 01384 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01385 } 01386 01387 ssl->handshake->in_msg_seq++; 01388 } 01389 else 01390 #endif 01391 { 01392 unsigned int cli_msg_seq = ( ssl->in_msg[4] << 8 ) | 01393 ssl->in_msg[5]; 01394 ssl->handshake->out_msg_seq = cli_msg_seq; 01395 ssl->handshake->in_msg_seq = cli_msg_seq + 1; 01396 } 01397 01398 /* 01399 * For now we don't support fragmentation, so make sure 01400 * fragment_offset == 0 and fragment_length == length 01401 */ 01402 if( ssl->in_msg[6] != 0 || ssl->in_msg[7] != 0 || ssl->in_msg[8] != 0 || 01403 memcmp( ssl->in_msg + 1, ssl->in_msg + 9, 3 ) != 0 ) 01404 { 01405 MBEDTLS_SSL_DEBUG_MSG( 1, ( "ClientHello fragmentation not supported" ) ); 01406 return( MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE ); 01407 } 01408 } 01409 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 01410 01411 buf += mbedtls_ssl_hs_hdr_len( ssl ); 01412 msg_len -= mbedtls_ssl_hs_hdr_len( ssl ); 01413 01414 /* 01415 * ClientHello layer: 01416 * 0 . 1 protocol version 01417 * 2 . 33 random bytes (starting with 4 bytes of Unix time) 01418 * 34 . 35 session id length (1 byte) 01419 * 35 . 34+x session id 01420 * 35+x . 35+x DTLS only: cookie length (1 byte) 01421 * 36+x . .. DTLS only: cookie 01422 * .. . .. ciphersuite list length (2 bytes) 01423 * .. . .. ciphersuite list 01424 * .. . .. compression alg. list length (1 byte) 01425 * .. . .. compression alg. list 01426 * .. . .. extensions length (2 bytes, optional) 01427 * .. . .. extensions (optional) 01428 */ 01429 01430 /* 01431 * Minimal length (with everything empty and extensions ommitted) is 01432 * 2 + 32 + 1 + 2 + 1 = 38 bytes. Check that first, so that we can 01433 * read at least up to session id length without worrying. 01434 */ 01435 if( msg_len < 38 ) 01436 { 01437 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01438 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01439 } 01440 01441 /* 01442 * Check and save the protocol version 01443 */ 01444 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, version", buf, 2 ); 01445 01446 mbedtls_ssl_read_version( &ssl->major_ver, &ssl->minor_ver, 01447 ssl->conf->transport, buf ); 01448 01449 ssl->handshake->max_major_ver = ssl->major_ver; 01450 ssl->handshake->max_minor_ver = ssl->minor_ver; 01451 01452 if( ssl->major_ver < ssl->conf->min_major_ver || 01453 ssl->minor_ver < ssl->conf->min_minor_ver ) 01454 { 01455 MBEDTLS_SSL_DEBUG_MSG( 1, ( "client only supports ssl smaller than minimum" 01456 " [%d:%d] < [%d:%d]", 01457 ssl->major_ver, ssl->minor_ver, 01458 ssl->conf->min_major_ver, ssl->conf->min_minor_ver ) ); 01459 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01460 MBEDTLS_SSL_ALERT_MSG_PROTOCOL_VERSION ); 01461 return( MBEDTLS_ERR_SSL_BAD_HS_PROTOCOL_VERSION ); 01462 } 01463 01464 if( ssl->major_ver > ssl->conf->max_major_ver ) 01465 { 01466 ssl->major_ver = ssl->conf->max_major_ver; 01467 ssl->minor_ver = ssl->conf->max_minor_ver; 01468 } 01469 else if( ssl->minor_ver > ssl->conf->max_minor_ver ) 01470 ssl->minor_ver = ssl->conf->max_minor_ver; 01471 01472 /* 01473 * Save client random (inc. Unix time) 01474 */ 01475 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, random bytes", buf + 2, 32 ); 01476 01477 memcpy( ssl->handshake->randbytes, buf + 2, 32 ); 01478 01479 /* 01480 * Check the session ID length and save session ID 01481 */ 01482 sess_len = buf[34]; 01483 01484 if( sess_len > sizeof( ssl->session_negotiate->id ) || 01485 sess_len + 34 + 2 > msg_len ) /* 2 for cipherlist length field */ 01486 { 01487 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01488 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01489 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01490 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01491 } 01492 01493 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, session id", buf + 35, sess_len ); 01494 01495 ssl->session_negotiate->id_len = sess_len; 01496 memset( ssl->session_negotiate->id, 0, 01497 sizeof( ssl->session_negotiate->id ) ); 01498 memcpy( ssl->session_negotiate->id, buf + 35, 01499 ssl->session_negotiate->id_len ); 01500 01501 /* 01502 * Check the cookie length and content 01503 */ 01504 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01505 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01506 { 01507 cookie_offset = 35 + sess_len; 01508 cookie_len = buf[cookie_offset]; 01509 01510 if( cookie_offset + 1 + cookie_len + 2 > msg_len ) 01511 { 01512 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01513 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01514 MBEDTLS_SSL_ALERT_MSG_PROTOCOL_VERSION ); 01515 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01516 } 01517 01518 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, cookie", 01519 buf + cookie_offset + 1, cookie_len ); 01520 01521 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) 01522 if( ssl->conf->f_cookie_check != NULL 01523 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01524 && ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE 01525 #endif 01526 ) 01527 { 01528 if( ssl->conf->f_cookie_check( ssl->conf->p_cookie, 01529 buf + cookie_offset + 1, cookie_len, 01530 ssl->cli_id, ssl->cli_id_len ) != 0 ) 01531 { 01532 MBEDTLS_SSL_DEBUG_MSG( 2, ( "cookie verification failed" ) ); 01533 ssl->handshake->verify_cookie_len = 1; 01534 } 01535 else 01536 { 01537 MBEDTLS_SSL_DEBUG_MSG( 2, ( "cookie verification passed" ) ); 01538 ssl->handshake->verify_cookie_len = 0; 01539 } 01540 } 01541 else 01542 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY */ 01543 { 01544 /* We know we didn't send a cookie, so it should be empty */ 01545 if( cookie_len != 0 ) 01546 { 01547 /* This may be an attacker's probe, so don't send an alert */ 01548 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01549 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01550 } 01551 01552 MBEDTLS_SSL_DEBUG_MSG( 2, ( "cookie verification skipped" ) ); 01553 } 01554 01555 /* 01556 * Check the ciphersuitelist length (will be parsed later) 01557 */ 01558 ciph_offset = cookie_offset + 1 + cookie_len; 01559 } 01560 else 01561 #endif /* MBEDTLS_SSL_PROTO_DTLS */ 01562 ciph_offset = 35 + sess_len; 01563 01564 ciph_len = ( buf[ciph_offset + 0] << 8 ) 01565 | ( buf[ciph_offset + 1] ); 01566 01567 if( ciph_len < 2 || 01568 ciph_len + 2 + ciph_offset + 1 > msg_len || /* 1 for comp. alg. len */ 01569 ( ciph_len % 2 ) != 0 ) 01570 { 01571 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01572 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01573 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01574 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01575 } 01576 01577 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, ciphersuitelist", 01578 buf + ciph_offset + 2, ciph_len ); 01579 01580 /* 01581 * Check the compression algorithms length and pick one 01582 */ 01583 comp_offset = ciph_offset + 2 + ciph_len; 01584 01585 comp_len = buf[comp_offset]; 01586 01587 if( comp_len < 1 || 01588 comp_len > 16 || 01589 comp_len + comp_offset + 1 > msg_len ) 01590 { 01591 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01592 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01593 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01594 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01595 } 01596 01597 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello, compression", 01598 buf + comp_offset + 1, comp_len ); 01599 01600 ssl->session_negotiate->compression = MBEDTLS_SSL_COMPRESS_NULL; 01601 #if defined(MBEDTLS_ZLIB_SUPPORT) 01602 for( i = 0; i < comp_len; ++i ) 01603 { 01604 if( buf[comp_offset + 1 + i] == MBEDTLS_SSL_COMPRESS_DEFLATE ) 01605 { 01606 ssl->session_negotiate->compression = MBEDTLS_SSL_COMPRESS_DEFLATE; 01607 break; 01608 } 01609 } 01610 #endif 01611 01612 /* See comments in ssl_write_client_hello() */ 01613 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01614 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01615 ssl->session_negotiate->compression = MBEDTLS_SSL_COMPRESS_NULL; 01616 #endif 01617 01618 /* Do not parse the extensions if the protocol is SSLv3 */ 01619 #if defined(MBEDTLS_SSL_PROTO_SSL3) 01620 if( ( ssl->major_ver != 3 ) || ( ssl->minor_ver != 0 ) ) 01621 { 01622 #endif 01623 /* 01624 * Check the extension length 01625 */ 01626 ext_offset = comp_offset + 1 + comp_len; 01627 if( msg_len > ext_offset ) 01628 { 01629 if( msg_len < ext_offset + 2 ) 01630 { 01631 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01632 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01633 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01634 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01635 } 01636 01637 ext_len = ( buf[ext_offset + 0] << 8 ) 01638 | ( buf[ext_offset + 1] ); 01639 01640 if( ( ext_len > 0 && ext_len < 4 ) || 01641 msg_len != ext_offset + 2 + ext_len ) 01642 { 01643 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01644 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01645 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01646 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01647 } 01648 } 01649 else 01650 ext_len = 0; 01651 01652 ext = buf + ext_offset + 2; 01653 MBEDTLS_SSL_DEBUG_BUF( 3, "client hello extensions", ext, ext_len ); 01654 01655 while( ext_len != 0 ) 01656 { 01657 unsigned int ext_id = ( ( ext[0] << 8 ) 01658 | ( ext[1] ) ); 01659 unsigned int ext_size = ( ( ext[2] << 8 ) 01660 | ( ext[3] ) ); 01661 01662 if( ext_size + 4 > ext_len ) 01663 { 01664 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01665 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01666 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01667 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01668 } 01669 switch( ext_id ) 01670 { 01671 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 01672 case MBEDTLS_TLS_EXT_SERVERNAME: 01673 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found ServerName extension" ) ); 01674 if( ssl->conf->f_sni == NULL ) 01675 break; 01676 01677 ret = ssl_parse_servername_ext( ssl, ext + 4, ext_size ); 01678 if( ret != 0 ) 01679 return( ret ); 01680 break; 01681 #endif /* MBEDTLS_SSL_SERVER_NAME_INDICATION */ 01682 01683 case MBEDTLS_TLS_EXT_RENEGOTIATION_INFO: 01684 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found renegotiation extension" ) ); 01685 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01686 renegotiation_info_seen = 1; 01687 #endif 01688 01689 ret = ssl_parse_renegotiation_info( ssl, ext + 4, ext_size ); 01690 if( ret != 0 ) 01691 return( ret ); 01692 break; 01693 01694 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 01695 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 01696 case MBEDTLS_TLS_EXT_SIG_ALG: 01697 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found signature_algorithms extension" ) ); 01698 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01699 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 01700 break; 01701 #endif 01702 ret = ssl_parse_signature_algorithms_ext( ssl, ext + 4, ext_size ); 01703 if( ret != 0 ) 01704 return( ret ); 01705 01706 sig_hash_alg_ext_present = 1; 01707 break; 01708 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 && 01709 MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 01710 01711 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 01712 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01713 case MBEDTLS_TLS_EXT_SUPPORTED_ELLIPTIC_CURVES: 01714 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found supported elliptic curves extension" ) ); 01715 01716 ret = ssl_parse_supported_elliptic_curves( ssl, ext + 4, ext_size ); 01717 if( ret != 0 ) 01718 return( ret ); 01719 break; 01720 01721 case MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS: 01722 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found supported point formats extension" ) ); 01723 ssl->handshake->cli_exts |= MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS_PRESENT; 01724 01725 ret = ssl_parse_supported_point_formats( ssl, ext + 4, ext_size ); 01726 if( ret != 0 ) 01727 return( ret ); 01728 break; 01729 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || 01730 MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 01731 01732 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 01733 case MBEDTLS_TLS_EXT_ECJPAKE_KKPP: 01734 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found ecjpake kkpp extension" ) ); 01735 01736 ret = ssl_parse_ecjpake_kkpp( ssl, ext + 4, ext_size ); 01737 if( ret != 0 ) 01738 return( ret ); 01739 break; 01740 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 01741 01742 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 01743 case MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH: 01744 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found max fragment length extension" ) ); 01745 01746 ret = ssl_parse_max_fragment_length_ext( ssl, ext + 4, ext_size ); 01747 if( ret != 0 ) 01748 return( ret ); 01749 break; 01750 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 01751 01752 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 01753 case MBEDTLS_TLS_EXT_TRUNCATED_HMAC: 01754 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found truncated hmac extension" ) ); 01755 01756 ret = ssl_parse_truncated_hmac_ext( ssl, ext + 4, ext_size ); 01757 if( ret != 0 ) 01758 return( ret ); 01759 break; 01760 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 01761 01762 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 01763 case MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC: 01764 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found encrypt then mac extension" ) ); 01765 01766 ret = ssl_parse_encrypt_then_mac_ext( ssl, ext + 4, ext_size ); 01767 if( ret != 0 ) 01768 return( ret ); 01769 break; 01770 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 01771 01772 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 01773 case MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET: 01774 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found extended master secret extension" ) ); 01775 01776 ret = ssl_parse_extended_ms_ext( ssl, ext + 4, ext_size ); 01777 if( ret != 0 ) 01778 return( ret ); 01779 break; 01780 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 01781 01782 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 01783 case MBEDTLS_TLS_EXT_SESSION_TICKET: 01784 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found session ticket extension" ) ); 01785 01786 ret = ssl_parse_session_ticket_ext( ssl, ext + 4, ext_size ); 01787 if( ret != 0 ) 01788 return( ret ); 01789 break; 01790 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 01791 01792 #if defined(MBEDTLS_SSL_ALPN) 01793 case MBEDTLS_TLS_EXT_ALPN: 01794 MBEDTLS_SSL_DEBUG_MSG( 3, ( "found alpn extension" ) ); 01795 01796 ret = ssl_parse_alpn_ext( ssl, ext + 4, ext_size ); 01797 if( ret != 0 ) 01798 return( ret ); 01799 break; 01800 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 01801 01802 default: 01803 MBEDTLS_SSL_DEBUG_MSG( 3, ( "unknown extension found: %d (ignoring)", 01804 ext_id ) ); 01805 } 01806 01807 ext_len -= 4 + ext_size; 01808 ext += 4 + ext_size; 01809 01810 if( ext_len > 0 && ext_len < 4 ) 01811 { 01812 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client hello message" ) ); 01813 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01814 MBEDTLS_SSL_ALERT_MSG_DECODE_ERROR ); 01815 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01816 } 01817 } 01818 #if defined(MBEDTLS_SSL_PROTO_SSL3) 01819 } 01820 #endif 01821 01822 #if defined(MBEDTLS_SSL_FALLBACK_SCSV) 01823 for( i = 0, p = buf + ciph_offset + 2; i < ciph_len; i += 2, p += 2 ) 01824 { 01825 if( p[0] == (unsigned char)( ( MBEDTLS_SSL_FALLBACK_SCSV_VALUE >> 8 ) & 0xff ) && 01826 p[1] == (unsigned char)( ( MBEDTLS_SSL_FALLBACK_SCSV_VALUE ) & 0xff ) ) 01827 { 01828 MBEDTLS_SSL_DEBUG_MSG( 2, ( "received FALLBACK_SCSV" ) ); 01829 01830 if( ssl->minor_ver < ssl->conf->max_minor_ver ) 01831 { 01832 MBEDTLS_SSL_DEBUG_MSG( 1, ( "inapropriate fallback" ) ); 01833 01834 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01835 MBEDTLS_SSL_ALERT_MSG_INAPROPRIATE_FALLBACK ); 01836 01837 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01838 } 01839 01840 break; 01841 } 01842 } 01843 #endif /* MBEDTLS_SSL_FALLBACK_SCSV */ 01844 01845 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 01846 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 01847 01848 /* 01849 * Try to fall back to default hash SHA1 if the client 01850 * hasn't provided any preferred signature-hash combinations. 01851 */ 01852 if( sig_hash_alg_ext_present == 0 ) 01853 { 01854 mbedtls_md_type_t md_default = MBEDTLS_MD_SHA1; 01855 01856 if( mbedtls_ssl_check_sig_hash( ssl, md_default ) != 0 ) 01857 md_default = MBEDTLS_MD_NONE; 01858 01859 mbedtls_ssl_sig_hash_set_const_hash( &ssl->handshake->hash_algs, md_default ); 01860 } 01861 01862 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 && 01863 MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED */ 01864 01865 /* 01866 * Check for TLS_EMPTY_RENEGOTIATION_INFO_SCSV 01867 */ 01868 for( i = 0, p = buf + ciph_offset + 2; i < ciph_len; i += 2, p += 2 ) 01869 { 01870 if( p[0] == 0 && p[1] == MBEDTLS_SSL_EMPTY_RENEGOTIATION_INFO ) 01871 { 01872 MBEDTLS_SSL_DEBUG_MSG( 3, ( "received TLS_EMPTY_RENEGOTIATION_INFO " ) ); 01873 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01874 if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS ) 01875 { 01876 MBEDTLS_SSL_DEBUG_MSG( 1, ( "received RENEGOTIATION SCSV " 01877 "during renegotiation" ) ); 01878 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01879 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01880 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01881 } 01882 #endif 01883 ssl->secure_renegotiation = MBEDTLS_SSL_SECURE_RENEGOTIATION; 01884 break; 01885 } 01886 } 01887 01888 /* 01889 * Renegotiation security checks 01890 */ 01891 if( ssl->secure_renegotiation != MBEDTLS_SSL_SECURE_RENEGOTIATION && 01892 ssl->conf->allow_legacy_renegotiation == MBEDTLS_SSL_LEGACY_BREAK_HANDSHAKE ) 01893 { 01894 MBEDTLS_SSL_DEBUG_MSG( 1, ( "legacy renegotiation, breaking off handshake" ) ); 01895 handshake_failure = 1; 01896 } 01897 #if defined(MBEDTLS_SSL_RENEGOTIATION) 01898 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01899 ssl->secure_renegotiation == MBEDTLS_SSL_SECURE_RENEGOTIATION && 01900 renegotiation_info_seen == 0 ) 01901 { 01902 MBEDTLS_SSL_DEBUG_MSG( 1, ( "renegotiation_info extension missing (secure)" ) ); 01903 handshake_failure = 1; 01904 } 01905 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01906 ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01907 ssl->conf->allow_legacy_renegotiation == MBEDTLS_SSL_LEGACY_NO_RENEGOTIATION ) 01908 { 01909 MBEDTLS_SSL_DEBUG_MSG( 1, ( "legacy renegotiation not allowed" ) ); 01910 handshake_failure = 1; 01911 } 01912 else if( ssl->renego_status == MBEDTLS_SSL_RENEGOTIATION_IN_PROGRESS && 01913 ssl->secure_renegotiation == MBEDTLS_SSL_LEGACY_RENEGOTIATION && 01914 renegotiation_info_seen == 1 ) 01915 { 01916 MBEDTLS_SSL_DEBUG_MSG( 1, ( "renegotiation_info extension present (legacy)" ) ); 01917 handshake_failure = 1; 01918 } 01919 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 01920 01921 if( handshake_failure == 1 ) 01922 { 01923 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01924 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01925 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_HELLO ); 01926 } 01927 01928 /* 01929 * Search for a matching ciphersuite 01930 * (At the end because we need information from the EC-based extensions 01931 * and certificate from the SNI callback triggered by the SNI extension.) 01932 */ 01933 got_common_suite = 0; 01934 ciphersuites = ssl->conf->ciphersuite_list[ssl->minor_ver]; 01935 ciphersuite_info = NULL; 01936 #if defined(MBEDTLS_SSL_SRV_RESPECT_CLIENT_PREFERENCE) 01937 for( j = 0, p = buf + ciph_offset + 2; j < ciph_len; j += 2, p += 2 ) 01938 for( i = 0; ciphersuites[i] != 0; i++ ) 01939 #else 01940 for( i = 0; ciphersuites[i] != 0; i++ ) 01941 for( j = 0, p = buf + ciph_offset + 2; j < ciph_len; j += 2, p += 2 ) 01942 #endif 01943 { 01944 if( p[0] != ( ( ciphersuites[i] >> 8 ) & 0xFF ) || 01945 p[1] != ( ( ciphersuites[i] ) & 0xFF ) ) 01946 continue; 01947 01948 got_common_suite = 1; 01949 01950 if( ( ret = ssl_ciphersuite_match( ssl, ciphersuites[i], 01951 &ciphersuite_info ) ) != 0 ) 01952 return( ret ); 01953 01954 if( ciphersuite_info != NULL ) 01955 goto have_ciphersuite; 01956 } 01957 01958 if( got_common_suite ) 01959 { 01960 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got ciphersuites in common, " 01961 "but none of them usable" ) ); 01962 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01963 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01964 return( MBEDTLS_ERR_SSL_NO_USABLE_CIPHERSUITE ); 01965 } 01966 else 01967 { 01968 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no ciphersuites in common" ) ); 01969 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 01970 MBEDTLS_SSL_ALERT_MSG_HANDSHAKE_FAILURE ); 01971 return( MBEDTLS_ERR_SSL_NO_CIPHER_CHOSEN ); 01972 } 01973 01974 have_ciphersuite: 01975 MBEDTLS_SSL_DEBUG_MSG( 2, ( "selected ciphersuite: %s", ciphersuite_info->name ) ); 01976 01977 ssl->session_negotiate->ciphersuite = ciphersuites[i]; 01978 ssl->transform_negotiate->ciphersuite_info = ciphersuite_info; 01979 01980 ssl->state++; 01981 01982 #if defined(MBEDTLS_SSL_PROTO_DTLS) 01983 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 01984 mbedtls_ssl_recv_flight_completed( ssl ); 01985 #endif 01986 01987 /* Debugging-only output for testsuite */ 01988 #if defined(MBEDTLS_DEBUG_C) && \ 01989 defined(MBEDTLS_SSL_PROTO_TLS1_2) && \ 01990 defined(MBEDTLS_KEY_EXCHANGE__WITH_CERT__ENABLED) 01991 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 01992 { 01993 mbedtls_pk_type_t sig_alg = mbedtls_ssl_get_ciphersuite_sig_alg( ciphersuite_info ); 01994 if( sig_alg != MBEDTLS_PK_NONE ) 01995 { 01996 mbedtls_md_type_t md_alg = mbedtls_ssl_sig_hash_set_find( &ssl->handshake->hash_algs, 01997 sig_alg ); 01998 MBEDTLS_SSL_DEBUG_MSG( 3, ( "client hello v3, signature_algorithm ext: %d", 01999 mbedtls_ssl_hash_from_md_alg( md_alg ) ) ); 02000 } 02001 else 02002 { 02003 MBEDTLS_SSL_DEBUG_MSG( 3, ( "no hash algorithm for signature algorithm " 02004 "%d - should not happen", sig_alg ) ); 02005 } 02006 } 02007 #endif 02008 02009 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse client hello" ) ); 02010 02011 return( 0 ); 02012 } 02013 02014 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 02015 static void ssl_write_truncated_hmac_ext( mbedtls_ssl_context *ssl, 02016 unsigned char *buf, 02017 size_t *olen ) 02018 { 02019 unsigned char *p = buf; 02020 02021 if( ssl->session_negotiate->trunc_hmac == MBEDTLS_SSL_TRUNC_HMAC_DISABLED ) 02022 { 02023 *olen = 0; 02024 return; 02025 } 02026 02027 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding truncated hmac extension" ) ); 02028 02029 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_TRUNCATED_HMAC >> 8 ) & 0xFF ); 02030 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_TRUNCATED_HMAC ) & 0xFF ); 02031 02032 *p++ = 0x00; 02033 *p++ = 0x00; 02034 02035 *olen = 4; 02036 } 02037 #endif /* MBEDTLS_SSL_TRUNCATED_HMAC */ 02038 02039 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 02040 static void ssl_write_encrypt_then_mac_ext( mbedtls_ssl_context *ssl, 02041 unsigned char *buf, 02042 size_t *olen ) 02043 { 02044 unsigned char *p = buf; 02045 const mbedtls_ssl_ciphersuite_t *suite = NULL; 02046 const mbedtls_cipher_info_t *cipher = NULL; 02047 02048 if( ssl->session_negotiate->encrypt_then_mac == MBEDTLS_SSL_EXTENDED_MS_DISABLED || 02049 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 02050 { 02051 *olen = 0; 02052 return; 02053 } 02054 02055 /* 02056 * RFC 7366: "If a server receives an encrypt-then-MAC request extension 02057 * from a client and then selects a stream or Authenticated Encryption 02058 * with Associated Data (AEAD) ciphersuite, it MUST NOT send an 02059 * encrypt-then-MAC response extension back to the client." 02060 */ 02061 if( ( suite = mbedtls_ssl_ciphersuite_from_id( 02062 ssl->session_negotiate->ciphersuite ) ) == NULL || 02063 ( cipher = mbedtls_cipher_info_from_type( suite->cipher ) ) == NULL || 02064 cipher->mode != MBEDTLS_MODE_CBC ) 02065 { 02066 *olen = 0; 02067 return; 02068 } 02069 02070 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding encrypt then mac extension" ) ); 02071 02072 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC >> 8 ) & 0xFF ); 02073 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ENCRYPT_THEN_MAC ) & 0xFF ); 02074 02075 *p++ = 0x00; 02076 *p++ = 0x00; 02077 02078 *olen = 4; 02079 } 02080 #endif /* MBEDTLS_SSL_ENCRYPT_THEN_MAC */ 02081 02082 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 02083 static void ssl_write_extended_ms_ext( mbedtls_ssl_context *ssl, 02084 unsigned char *buf, 02085 size_t *olen ) 02086 { 02087 unsigned char *p = buf; 02088 02089 if( ssl->handshake->extended_ms == MBEDTLS_SSL_EXTENDED_MS_DISABLED || 02090 ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_0 ) 02091 { 02092 *olen = 0; 02093 return; 02094 } 02095 02096 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding extended master secret " 02097 "extension" ) ); 02098 02099 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET >> 8 ) & 0xFF ); 02100 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_EXTENDED_MASTER_SECRET ) & 0xFF ); 02101 02102 *p++ = 0x00; 02103 *p++ = 0x00; 02104 02105 *olen = 4; 02106 } 02107 #endif /* MBEDTLS_SSL_EXTENDED_MASTER_SECRET */ 02108 02109 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 02110 static void ssl_write_session_ticket_ext( mbedtls_ssl_context *ssl, 02111 unsigned char *buf, 02112 size_t *olen ) 02113 { 02114 unsigned char *p = buf; 02115 02116 if( ssl->handshake->new_session_ticket == 0 ) 02117 { 02118 *olen = 0; 02119 return; 02120 } 02121 02122 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding session ticket extension" ) ); 02123 02124 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SESSION_TICKET >> 8 ) & 0xFF ); 02125 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SESSION_TICKET ) & 0xFF ); 02126 02127 *p++ = 0x00; 02128 *p++ = 0x00; 02129 02130 *olen = 4; 02131 } 02132 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 02133 02134 static void ssl_write_renegotiation_ext( mbedtls_ssl_context *ssl, 02135 unsigned char *buf, 02136 size_t *olen ) 02137 { 02138 unsigned char *p = buf; 02139 02140 if( ssl->secure_renegotiation != MBEDTLS_SSL_SECURE_RENEGOTIATION ) 02141 { 02142 *olen = 0; 02143 return; 02144 } 02145 02146 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, secure renegotiation extension" ) ); 02147 02148 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_RENEGOTIATION_INFO >> 8 ) & 0xFF ); 02149 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_RENEGOTIATION_INFO ) & 0xFF ); 02150 02151 #if defined(MBEDTLS_SSL_RENEGOTIATION) 02152 if( ssl->renego_status != MBEDTLS_SSL_INITIAL_HANDSHAKE ) 02153 { 02154 *p++ = 0x00; 02155 *p++ = ( ssl->verify_data_len * 2 + 1 ) & 0xFF; 02156 *p++ = ssl->verify_data_len * 2 & 0xFF; 02157 02158 memcpy( p, ssl->peer_verify_data, ssl->verify_data_len ); 02159 p += ssl->verify_data_len; 02160 memcpy( p, ssl->own_verify_data, ssl->verify_data_len ); 02161 p += ssl->verify_data_len; 02162 } 02163 else 02164 #endif /* MBEDTLS_SSL_RENEGOTIATION */ 02165 { 02166 *p++ = 0x00; 02167 *p++ = 0x01; 02168 *p++ = 0x00; 02169 } 02170 02171 *olen = p - buf; 02172 } 02173 02174 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 02175 static void ssl_write_max_fragment_length_ext( mbedtls_ssl_context *ssl, 02176 unsigned char *buf, 02177 size_t *olen ) 02178 { 02179 unsigned char *p = buf; 02180 02181 if( ssl->session_negotiate->mfl_code == MBEDTLS_SSL_MAX_FRAG_LEN_NONE ) 02182 { 02183 *olen = 0; 02184 return; 02185 } 02186 02187 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, max_fragment_length extension" ) ); 02188 02189 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH >> 8 ) & 0xFF ); 02190 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_MAX_FRAGMENT_LENGTH ) & 0xFF ); 02191 02192 *p++ = 0x00; 02193 *p++ = 1; 02194 02195 *p++ = ssl->session_negotiate->mfl_code; 02196 02197 *olen = 5; 02198 } 02199 #endif /* MBEDTLS_SSL_MAX_FRAGMENT_LENGTH */ 02200 02201 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 02202 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02203 static void ssl_write_supported_point_formats_ext( mbedtls_ssl_context *ssl, 02204 unsigned char *buf, 02205 size_t *olen ) 02206 { 02207 unsigned char *p = buf; 02208 ((void) ssl); 02209 02210 if( ( ssl->handshake->cli_exts & 02211 MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS_PRESENT ) == 0 ) 02212 { 02213 *olen = 0; 02214 return; 02215 } 02216 02217 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, supported_point_formats extension" ) ); 02218 02219 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS >> 8 ) & 0xFF ); 02220 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_SUPPORTED_POINT_FORMATS ) & 0xFF ); 02221 02222 *p++ = 0x00; 02223 *p++ = 2; 02224 02225 *p++ = 1; 02226 *p++ = MBEDTLS_ECP_PF_UNCOMPRESSED; 02227 02228 *olen = 6; 02229 } 02230 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C || MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 02231 02232 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02233 static void ssl_write_ecjpake_kkpp_ext( mbedtls_ssl_context *ssl, 02234 unsigned char *buf, 02235 size_t *olen ) 02236 { 02237 int ret; 02238 unsigned char *p = buf; 02239 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 02240 size_t kkpp_len; 02241 02242 *olen = 0; 02243 02244 /* Skip costly computation if not needed */ 02245 if( ssl->transform_negotiate->ciphersuite_info->key_exchange != 02246 MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 02247 return; 02248 02249 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, ecjpake kkpp extension" ) ); 02250 02251 if( end - p < 4 ) 02252 { 02253 MBEDTLS_SSL_DEBUG_MSG( 1, ( "buffer too small" ) ); 02254 return; 02255 } 02256 02257 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ECJPAKE_KKPP >> 8 ) & 0xFF ); 02258 *p++ = (unsigned char)( ( MBEDTLS_TLS_EXT_ECJPAKE_KKPP ) & 0xFF ); 02259 02260 ret = mbedtls_ecjpake_write_round_one( &ssl->handshake->ecjpake_ctx, 02261 p + 2, end - p - 2, &kkpp_len, 02262 ssl->conf->f_rng, ssl->conf->p_rng ); 02263 if( ret != 0 ) 02264 { 02265 MBEDTLS_SSL_DEBUG_RET( 1 , "mbedtls_ecjpake_write_round_one", ret ); 02266 return; 02267 } 02268 02269 *p++ = (unsigned char)( ( kkpp_len >> 8 ) & 0xFF ); 02270 *p++ = (unsigned char)( ( kkpp_len ) & 0xFF ); 02271 02272 *olen = kkpp_len + 4; 02273 } 02274 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 02275 02276 #if defined(MBEDTLS_SSL_ALPN ) 02277 static void ssl_write_alpn_ext( mbedtls_ssl_context *ssl, 02278 unsigned char *buf, size_t *olen ) 02279 { 02280 if( ssl->alpn_chosen == NULL ) 02281 { 02282 *olen = 0; 02283 return; 02284 } 02285 02286 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, adding alpn extension" ) ); 02287 02288 /* 02289 * 0 . 1 ext identifier 02290 * 2 . 3 ext length 02291 * 4 . 5 protocol list length 02292 * 6 . 6 protocol name length 02293 * 7 . 7+n protocol name 02294 */ 02295 buf[0] = (unsigned char)( ( MBEDTLS_TLS_EXT_ALPN >> 8 ) & 0xFF ); 02296 buf[1] = (unsigned char)( ( MBEDTLS_TLS_EXT_ALPN ) & 0xFF ); 02297 02298 *olen = 7 + strlen( ssl->alpn_chosen ); 02299 02300 buf[2] = (unsigned char)( ( ( *olen - 4 ) >> 8 ) & 0xFF ); 02301 buf[3] = (unsigned char)( ( ( *olen - 4 ) ) & 0xFF ); 02302 02303 buf[4] = (unsigned char)( ( ( *olen - 6 ) >> 8 ) & 0xFF ); 02304 buf[5] = (unsigned char)( ( ( *olen - 6 ) ) & 0xFF ); 02305 02306 buf[6] = (unsigned char)( ( ( *olen - 7 ) ) & 0xFF ); 02307 02308 memcpy( buf + 7, ssl->alpn_chosen, *olen - 7 ); 02309 } 02310 #endif /* MBEDTLS_ECDH_C || MBEDTLS_ECDSA_C */ 02311 02312 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) 02313 static int ssl_write_hello_verify_request( mbedtls_ssl_context *ssl ) 02314 { 02315 int ret; 02316 unsigned char *p = ssl->out_msg + 4; 02317 unsigned char *cookie_len_byte; 02318 02319 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write hello verify request" ) ); 02320 02321 /* 02322 * struct { 02323 * ProtocolVersion server_version; 02324 * opaque cookie<0..2^8-1>; 02325 * } HelloVerifyRequest; 02326 */ 02327 02328 /* The RFC is not clear on this point, but sending the actual negotiated 02329 * version looks like the most interoperable thing to do. */ 02330 mbedtls_ssl_write_version( ssl->major_ver, ssl->minor_ver, 02331 ssl->conf->transport, p ); 02332 MBEDTLS_SSL_DEBUG_BUF( 3, "server version", p, 2 ); 02333 p += 2; 02334 02335 /* If we get here, f_cookie_check is not null */ 02336 if( ssl->conf->f_cookie_write == NULL ) 02337 { 02338 MBEDTLS_SSL_DEBUG_MSG( 1, ( "inconsistent cookie callbacks" ) ); 02339 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02340 } 02341 02342 /* Skip length byte until we know the length */ 02343 cookie_len_byte = p++; 02344 02345 if( ( ret = ssl->conf->f_cookie_write( ssl->conf->p_cookie, 02346 &p, ssl->out_buf + MBEDTLS_SSL_BUFFER_LEN, 02347 ssl->cli_id, ssl->cli_id_len ) ) != 0 ) 02348 { 02349 MBEDTLS_SSL_DEBUG_RET( 1, "f_cookie_write", ret ); 02350 return( ret ); 02351 } 02352 02353 *cookie_len_byte = (unsigned char)( p - ( cookie_len_byte + 1 ) ); 02354 02355 MBEDTLS_SSL_DEBUG_BUF( 3, "cookie sent", cookie_len_byte + 1, *cookie_len_byte ); 02356 02357 ssl->out_msglen = p - ssl->out_msg; 02358 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 02359 ssl->out_msg[0] = MBEDTLS_SSL_HS_HELLO_VERIFY_REQUEST; 02360 02361 ssl->state = MBEDTLS_SSL_SERVER_HELLO_VERIFY_REQUEST_SENT; 02362 02363 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 02364 { 02365 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 02366 return( ret ); 02367 } 02368 02369 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write hello verify request" ) ); 02370 02371 return( 0 ); 02372 } 02373 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY */ 02374 02375 static int ssl_write_server_hello( mbedtls_ssl_context *ssl ) 02376 { 02377 #if defined(MBEDTLS_HAVE_TIME) 02378 mbedtls_time_t t; 02379 #endif 02380 int ret; 02381 size_t olen, ext_len = 0, n; 02382 unsigned char *buf, *p; 02383 02384 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write server hello" ) ); 02385 02386 #if defined(MBEDTLS_SSL_DTLS_HELLO_VERIFY) 02387 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 02388 ssl->handshake->verify_cookie_len != 0 ) 02389 { 02390 MBEDTLS_SSL_DEBUG_MSG( 2, ( "client hello was not authenticated" ) ); 02391 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write server hello" ) ); 02392 02393 return( ssl_write_hello_verify_request( ssl ) ); 02394 } 02395 #endif /* MBEDTLS_SSL_DTLS_HELLO_VERIFY */ 02396 02397 if( ssl->conf->f_rng == NULL ) 02398 { 02399 MBEDTLS_SSL_DEBUG_MSG( 1, ( "no RNG provided") ); 02400 return( MBEDTLS_ERR_SSL_NO_RNG ); 02401 } 02402 02403 /* 02404 * 0 . 0 handshake type 02405 * 1 . 3 handshake length 02406 * 4 . 5 protocol version 02407 * 6 . 9 UNIX time() 02408 * 10 . 37 random bytes 02409 */ 02410 buf = ssl->out_msg; 02411 p = buf + 4; 02412 02413 mbedtls_ssl_write_version( ssl->major_ver, ssl->minor_ver, 02414 ssl->conf->transport, p ); 02415 p += 2; 02416 02417 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, chosen version: [%d:%d]", 02418 buf[4], buf[5] ) ); 02419 02420 #if defined(MBEDTLS_HAVE_TIME) 02421 t = mbedtls_time( NULL ); 02422 *p++ = (unsigned char)( t >> 24 ); 02423 *p++ = (unsigned char)( t >> 16 ); 02424 *p++ = (unsigned char)( t >> 8 ); 02425 *p++ = (unsigned char)( t ); 02426 02427 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, current time: %lu", t ) ); 02428 #else 02429 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, p, 4 ) ) != 0 ) 02430 return( ret ); 02431 02432 p += 4; 02433 #endif /* MBEDTLS_HAVE_TIME */ 02434 02435 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, p, 28 ) ) != 0 ) 02436 return( ret ); 02437 02438 p += 28; 02439 02440 memcpy( ssl->handshake->randbytes + 32, buf + 6, 32 ); 02441 02442 MBEDTLS_SSL_DEBUG_BUF( 3, "server hello, random bytes", buf + 6, 32 ); 02443 02444 /* 02445 * Resume is 0 by default, see ssl_handshake_init(). 02446 * It may be already set to 1 by ssl_parse_session_ticket_ext(). 02447 * If not, try looking up session ID in our cache. 02448 */ 02449 if( ssl->handshake->resume == 0 && 02450 #if defined(MBEDTLS_SSL_RENEGOTIATION) 02451 ssl->renego_status == MBEDTLS_SSL_INITIAL_HANDSHAKE && 02452 #endif 02453 ssl->session_negotiate->id_len != 0 && 02454 ssl->conf->f_get_cache != NULL && 02455 ssl->conf->f_get_cache( ssl->conf->p_cache, ssl->session_negotiate ) == 0 ) 02456 { 02457 MBEDTLS_SSL_DEBUG_MSG( 3, ( "session successfully restored from cache" ) ); 02458 ssl->handshake->resume = 1; 02459 } 02460 02461 if( ssl->handshake->resume == 0 ) 02462 { 02463 /* 02464 * New session, create a new session id, 02465 * unless we're about to issue a session ticket 02466 */ 02467 ssl->state++; 02468 02469 #if defined(MBEDTLS_HAVE_TIME) 02470 ssl->session_negotiate->start = mbedtls_time( NULL ); 02471 #endif 02472 02473 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 02474 if( ssl->handshake->new_session_ticket != 0 ) 02475 { 02476 ssl->session_negotiate->id_len = n = 0; 02477 memset( ssl->session_negotiate->id, 0, 32 ); 02478 } 02479 else 02480 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 02481 { 02482 ssl->session_negotiate->id_len = n = 32; 02483 if( ( ret = ssl->conf->f_rng( ssl->conf->p_rng, ssl->session_negotiate->id, 02484 n ) ) != 0 ) 02485 return( ret ); 02486 } 02487 } 02488 else 02489 { 02490 /* 02491 * Resuming a session 02492 */ 02493 n = ssl->session_negotiate->id_len; 02494 ssl->state = MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC; 02495 02496 if( ( ret = mbedtls_ssl_derive_keys( ssl ) ) != 0 ) 02497 { 02498 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_derive_keys", ret ); 02499 return( ret ); 02500 } 02501 } 02502 02503 /* 02504 * 38 . 38 session id length 02505 * 39 . 38+n session id 02506 * 39+n . 40+n chosen ciphersuite 02507 * 41+n . 41+n chosen compression alg. 02508 * 42+n . 43+n extensions length 02509 * 44+n . 43+n+m extensions 02510 */ 02511 *p++ = (unsigned char) ssl->session_negotiate->id_len; 02512 memcpy( p, ssl->session_negotiate->id, ssl->session_negotiate->id_len ); 02513 p += ssl->session_negotiate->id_len; 02514 02515 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, session id len.: %d", n ) ); 02516 MBEDTLS_SSL_DEBUG_BUF( 3, "server hello, session id", buf + 39, n ); 02517 MBEDTLS_SSL_DEBUG_MSG( 3, ( "%s session has been resumed", 02518 ssl->handshake->resume ? "a" : "no" ) ); 02519 02520 *p++ = (unsigned char)( ssl->session_negotiate->ciphersuite >> 8 ); 02521 *p++ = (unsigned char)( ssl->session_negotiate->ciphersuite ); 02522 *p++ = (unsigned char)( ssl->session_negotiate->compression ); 02523 02524 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, chosen ciphersuite: %s", 02525 mbedtls_ssl_get_ciphersuite_name( ssl->session_negotiate->ciphersuite ) ) ); 02526 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, compress alg.: 0x%02X", 02527 ssl->session_negotiate->compression ) ); 02528 02529 /* Do not write the extensions if the protocol is SSLv3 */ 02530 #if defined(MBEDTLS_SSL_PROTO_SSL3) 02531 if( ( ssl->major_ver != 3 ) || ( ssl->minor_ver != 0 ) ) 02532 { 02533 #endif 02534 02535 /* 02536 * First write extensions, then the total length 02537 */ 02538 ssl_write_renegotiation_ext( ssl, p + 2 + ext_len, &olen ); 02539 ext_len += olen; 02540 02541 #if defined(MBEDTLS_SSL_MAX_FRAGMENT_LENGTH) 02542 ssl_write_max_fragment_length_ext( ssl, p + 2 + ext_len, &olen ); 02543 ext_len += olen; 02544 #endif 02545 02546 #if defined(MBEDTLS_SSL_TRUNCATED_HMAC) 02547 ssl_write_truncated_hmac_ext( ssl, p + 2 + ext_len, &olen ); 02548 ext_len += olen; 02549 #endif 02550 02551 #if defined(MBEDTLS_SSL_ENCRYPT_THEN_MAC) 02552 ssl_write_encrypt_then_mac_ext( ssl, p + 2 + ext_len, &olen ); 02553 ext_len += olen; 02554 #endif 02555 02556 #if defined(MBEDTLS_SSL_EXTENDED_MASTER_SECRET) 02557 ssl_write_extended_ms_ext( ssl, p + 2 + ext_len, &olen ); 02558 ext_len += olen; 02559 #endif 02560 02561 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 02562 ssl_write_session_ticket_ext( ssl, p + 2 + ext_len, &olen ); 02563 ext_len += olen; 02564 #endif 02565 02566 #if defined(MBEDTLS_ECDH_C) || defined(MBEDTLS_ECDSA_C) || \ 02567 defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02568 ssl_write_supported_point_formats_ext( ssl, p + 2 + ext_len, &olen ); 02569 ext_len += olen; 02570 #endif 02571 02572 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02573 ssl_write_ecjpake_kkpp_ext( ssl, p + 2 + ext_len, &olen ); 02574 ext_len += olen; 02575 #endif 02576 02577 #if defined(MBEDTLS_SSL_ALPN) 02578 ssl_write_alpn_ext( ssl, p + 2 + ext_len, &olen ); 02579 ext_len += olen; 02580 #endif 02581 02582 MBEDTLS_SSL_DEBUG_MSG( 3, ( "server hello, total extension length: %d", ext_len ) ); 02583 02584 if( ext_len > 0 ) 02585 { 02586 *p++ = (unsigned char)( ( ext_len >> 8 ) & 0xFF ); 02587 *p++ = (unsigned char)( ( ext_len ) & 0xFF ); 02588 p += ext_len; 02589 } 02590 02591 #if defined(MBEDTLS_SSL_PROTO_SSL3) 02592 } 02593 #endif 02594 02595 ssl->out_msglen = p - buf; 02596 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 02597 ssl->out_msg[0] = MBEDTLS_SSL_HS_SERVER_HELLO; 02598 02599 ret = mbedtls_ssl_write_record( ssl ); 02600 02601 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write server hello" ) ); 02602 02603 return( ret ); 02604 } 02605 02606 #if !defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) && \ 02607 !defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) && \ 02608 !defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) && \ 02609 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) && \ 02610 !defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED)&& \ 02611 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) 02612 static int ssl_write_certificate_request( mbedtls_ssl_context *ssl ) 02613 { 02614 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 02615 ssl->transform_negotiate->ciphersuite_info; 02616 02617 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write certificate request" ) ); 02618 02619 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 02620 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 02621 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 02622 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 02623 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 02624 { 02625 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate request" ) ); 02626 ssl->state++; 02627 return( 0 ); 02628 } 02629 02630 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 02631 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 02632 } 02633 #else 02634 static int ssl_write_certificate_request( mbedtls_ssl_context *ssl ) 02635 { 02636 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 02637 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 02638 ssl->transform_negotiate->ciphersuite_info; 02639 size_t dn_size, total_dn_size; /* excluding length bytes */ 02640 size_t ct_len, sa_len; /* including length bytes */ 02641 unsigned char *buf, *p; 02642 const unsigned char * const end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 02643 const mbedtls_x509_crt *crt; 02644 int authmode; 02645 02646 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write certificate request" ) ); 02647 02648 ssl->state++; 02649 02650 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 02651 if( ssl->handshake->sni_authmode != MBEDTLS_SSL_VERIFY_UNSET ) 02652 authmode = ssl->handshake->sni_authmode; 02653 else 02654 #endif 02655 authmode = ssl->conf->authmode; 02656 02657 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 02658 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 02659 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 02660 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 02661 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE || 02662 authmode == MBEDTLS_SSL_VERIFY_NONE ) 02663 { 02664 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write certificate request" ) ); 02665 return( 0 ); 02666 } 02667 02668 /* 02669 * 0 . 0 handshake type 02670 * 1 . 3 handshake length 02671 * 4 . 4 cert type count 02672 * 5 .. m-1 cert types 02673 * m .. m+1 sig alg length (TLS 1.2 only) 02674 * m+1 .. n-1 SignatureAndHashAlgorithms (TLS 1.2 only) 02675 * n .. n+1 length of all DNs 02676 * n+2 .. n+3 length of DN 1 02677 * n+4 .. ... Distinguished Name #1 02678 * ... .. ... length of DN 2, etc. 02679 */ 02680 buf = ssl->out_msg; 02681 p = buf + 4; 02682 02683 /* 02684 * Supported certificate types 02685 * 02686 * ClientCertificateType certificate_types<1..2^8-1>; 02687 * enum { (255) } ClientCertificateType; 02688 */ 02689 ct_len = 0; 02690 02691 #if defined(MBEDTLS_RSA_C) 02692 p[1 + ct_len++] = MBEDTLS_SSL_CERT_TYPE_RSA_SIGN; 02693 #endif 02694 #if defined(MBEDTLS_ECDSA_C) 02695 p[1 + ct_len++] = MBEDTLS_SSL_CERT_TYPE_ECDSA_SIGN; 02696 #endif 02697 02698 p[0] = (unsigned char) ct_len++; 02699 p += ct_len; 02700 02701 sa_len = 0; 02702 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 02703 /* 02704 * Add signature_algorithms for verify (TLS 1.2) 02705 * 02706 * SignatureAndHashAlgorithm supported_signature_algorithms<2..2^16-2>; 02707 * 02708 * struct { 02709 * HashAlgorithm hash; 02710 * SignatureAlgorithm signature; 02711 * } SignatureAndHashAlgorithm; 02712 * 02713 * enum { (255) } HashAlgorithm; 02714 * enum { (255) } SignatureAlgorithm; 02715 */ 02716 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 02717 { 02718 const int *cur; 02719 02720 /* 02721 * Supported signature algorithms 02722 */ 02723 for( cur = ssl->conf->sig_hashes; *cur != MBEDTLS_MD_NONE; cur++ ) 02724 { 02725 unsigned char hash = mbedtls_ssl_hash_from_md_alg( *cur ); 02726 02727 if( MBEDTLS_SSL_HASH_NONE == hash || mbedtls_ssl_set_calc_verify_md( ssl, hash ) ) 02728 continue; 02729 02730 #if defined(MBEDTLS_RSA_C) 02731 p[2 + sa_len++] = hash; 02732 p[2 + sa_len++] = MBEDTLS_SSL_SIG_RSA; 02733 #endif 02734 #if defined(MBEDTLS_ECDSA_C) 02735 p[2 + sa_len++] = hash; 02736 p[2 + sa_len++] = MBEDTLS_SSL_SIG_ECDSA; 02737 #endif 02738 } 02739 02740 p[0] = (unsigned char)( sa_len >> 8 ); 02741 p[1] = (unsigned char)( sa_len ); 02742 sa_len += 2; 02743 p += sa_len; 02744 } 02745 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 02746 02747 /* 02748 * DistinguishedName certificate_authorities<0..2^16-1>; 02749 * opaque DistinguishedName<1..2^16-1>; 02750 */ 02751 p += 2; 02752 02753 total_dn_size = 0; 02754 02755 if( ssl->conf->cert_req_ca_list == MBEDTLS_SSL_CERT_REQ_CA_LIST_ENABLED ) 02756 { 02757 #if defined(MBEDTLS_SSL_SERVER_NAME_INDICATION) 02758 if( ssl->handshake->sni_ca_chain != NULL ) 02759 crt = ssl->handshake->sni_ca_chain; 02760 else 02761 #endif 02762 crt = ssl->conf->ca_chain; 02763 02764 while( crt != NULL && crt->version != 0 ) 02765 { 02766 dn_size = crt->subject_raw.len; 02767 02768 if( end < p || 02769 (size_t)( end - p ) < dn_size || 02770 (size_t)( end - p ) < 2 + dn_size ) 02771 { 02772 MBEDTLS_SSL_DEBUG_MSG( 1, ( "skipping CAs: buffer too short" ) ); 02773 break; 02774 } 02775 02776 *p++ = (unsigned char)( dn_size >> 8 ); 02777 *p++ = (unsigned char)( dn_size ); 02778 memcpy( p, crt->subject_raw.p, dn_size ); 02779 p += dn_size; 02780 02781 MBEDTLS_SSL_DEBUG_BUF( 3, "requested DN", p - dn_size, dn_size ); 02782 02783 total_dn_size += 2 + dn_size; 02784 crt = crt->next; 02785 } 02786 } 02787 02788 ssl->out_msglen = p - buf; 02789 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 02790 ssl->out_msg[0] = MBEDTLS_SSL_HS_CERTIFICATE_REQUEST; 02791 ssl->out_msg[4 + ct_len + sa_len] = (unsigned char)( total_dn_size >> 8 ); 02792 ssl->out_msg[5 + ct_len + sa_len] = (unsigned char)( total_dn_size ); 02793 02794 ret = mbedtls_ssl_write_record( ssl ); 02795 02796 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write certificate request" ) ); 02797 02798 return( ret ); 02799 } 02800 #endif /* !MBEDTLS_KEY_EXCHANGE_RSA_ENABLED && 02801 !MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED && 02802 !MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED && 02803 !MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED && 02804 !MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED && 02805 !MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED */ 02806 02807 #if defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 02808 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 02809 static int ssl_get_ecdh_params_from_cert( mbedtls_ssl_context *ssl ) 02810 { 02811 int ret; 02812 02813 if( ! mbedtls_pk_can_do( mbedtls_ssl_own_key( ssl ), MBEDTLS_PK_ECKEY ) ) 02814 { 02815 MBEDTLS_SSL_DEBUG_MSG( 1, ( "server key not ECDH capable" ) ); 02816 return( MBEDTLS_ERR_SSL_PK_TYPE_MISMATCH ); 02817 } 02818 02819 if( ( ret = mbedtls_ecdh_get_params( &ssl->handshake->ecdh_ctx, 02820 mbedtls_pk_ec( *mbedtls_ssl_own_key( ssl ) ), 02821 MBEDTLS_ECDH_OURS ) ) != 0 ) 02822 { 02823 MBEDTLS_SSL_DEBUG_RET( 1, ( "mbedtls_ecdh_get_params" ), ret ); 02824 return( ret ); 02825 } 02826 02827 return( 0 ); 02828 } 02829 #endif /* MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || 02830 MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 02831 02832 static int ssl_write_server_key_exchange( mbedtls_ssl_context *ssl ) 02833 { 02834 int ret; 02835 size_t n = 0; 02836 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 02837 ssl->transform_negotiate->ciphersuite_info; 02838 02839 #if defined(MBEDTLS_KEY_EXCHANGE__SOME_PFS__ENABLED) 02840 unsigned char *p = ssl->out_msg + 4; 02841 size_t len; 02842 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_SERVER_SIGNATURE__ENABLED) 02843 unsigned char *dig_signed = p; 02844 size_t dig_signed_len = 0; 02845 #endif /* MBEDTLS_KEY_EXCHANGE__WITH_SERVER_SIGNATURE__ENABLED */ 02846 #endif /* MBEDTLS_KEY_EXCHANGE__SOME_PFS__ENABLED */ 02847 02848 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write server key exchange" ) ); 02849 02850 /* 02851 * 02852 * Part 1: Extract static ECDH parameters and abort 02853 * if ServerKeyExchange not needed. 02854 * 02855 */ 02856 02857 /* For suites involving ECDH, extract DH parameters 02858 * from certificate at this point. */ 02859 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__ECDH_ENABLED) 02860 if( mbedtls_ssl_ciphersuite_uses_ecdh( ciphersuite_info ) ) 02861 { 02862 ssl_get_ecdh_params_from_cert( ssl ); 02863 } 02864 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__ECDH_ENABLED */ 02865 02866 /* Key exchanges not involving ephemeral keys don't use 02867 * ServerKeyExchange, so end here. */ 02868 #if defined(MBEDTLS_KEY_EXCHANGE__SOME_NON_PFS__ENABLED) 02869 if( mbedtls_ssl_ciphersuite_no_pfs( ciphersuite_info ) ) 02870 { 02871 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip write server key exchange" ) ); 02872 ssl->state++; 02873 return( 0 ); 02874 } 02875 #endif /* MBEDTLS_KEY_EXCHANGE__NON_PFS__ENABLED */ 02876 02877 /* 02878 * 02879 * Part 2: Provide key exchange parameters for chosen ciphersuite. 02880 * 02881 */ 02882 02883 /* 02884 * - ECJPAKE key exchanges 02885 */ 02886 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 02887 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 02888 { 02889 const unsigned char *end = ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN; 02890 02891 ret = mbedtls_ecjpake_write_round_two( &ssl->handshake->ecjpake_ctx, 02892 p, end - p, &len, ssl->conf->f_rng, ssl->conf->p_rng ); 02893 if( ret != 0 ) 02894 { 02895 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_write_round_two", ret ); 02896 return( ret ); 02897 } 02898 02899 p += len; 02900 n += len; 02901 } 02902 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 02903 02904 /* 02905 * For (EC)DHE key exchanges with PSK, parameters are prefixed by support 02906 * identity hint (RFC 4279, Sec. 3). Until someone needs this feature, 02907 * we use empty support identity hints here. 02908 **/ 02909 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) || \ 02910 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 02911 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 02912 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK ) 02913 { 02914 *(p++) = 0x00; 02915 *(p++) = 0x00; 02916 02917 n += 2; 02918 } 02919 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED || 02920 MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED */ 02921 02922 /* 02923 * - DHE key exchanges 02924 */ 02925 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__DHE_ENABLED) 02926 if( mbedtls_ssl_ciphersuite_uses_dhe( ciphersuite_info ) ) 02927 { 02928 if( ssl->conf->dhm_P.p == NULL || ssl->conf->dhm_G.p == NULL ) 02929 { 02930 MBEDTLS_SSL_DEBUG_MSG( 1, ( "no DH parameters set" ) ); 02931 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 02932 } 02933 02934 /* 02935 * Ephemeral DH parameters: 02936 * 02937 * struct { 02938 * opaque dh_p<1..2^16-1>; 02939 * opaque dh_g<1..2^16-1>; 02940 * opaque dh_Ys<1..2^16-1>; 02941 * } ServerDHParams; 02942 */ 02943 if( ( ret = mbedtls_mpi_copy( &ssl->handshake->dhm_ctx.P, &ssl->conf->dhm_P ) ) != 0 || 02944 ( ret = mbedtls_mpi_copy( &ssl->handshake->dhm_ctx.G, &ssl->conf->dhm_G ) ) != 0 ) 02945 { 02946 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_mpi_copy", ret ); 02947 return( ret ); 02948 } 02949 02950 if( ( ret = mbedtls_dhm_make_params( &ssl->handshake->dhm_ctx, 02951 (int) mbedtls_mpi_size( &ssl->handshake->dhm_ctx.P ), 02952 p, &len, ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 02953 { 02954 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_make_params", ret ); 02955 return( ret ); 02956 } 02957 02958 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_SERVER_SIGNATURE__ENABLED) 02959 dig_signed = p; 02960 dig_signed_len = len; 02961 #endif 02962 02963 p += len; 02964 n += len; 02965 02966 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: X ", &ssl->handshake->dhm_ctx.X ); 02967 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: P ", &ssl->handshake->dhm_ctx.P ); 02968 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: G ", &ssl->handshake->dhm_ctx.G ); 02969 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: GX", &ssl->handshake->dhm_ctx.GX ); 02970 } 02971 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__DHE_ENABLED */ 02972 02973 /* 02974 * - ECDHE key exchanges 02975 */ 02976 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__ECDHE_ENABLED) 02977 if( mbedtls_ssl_ciphersuite_uses_ecdhe( ciphersuite_info ) ) 02978 { 02979 /* 02980 * Ephemeral ECDH parameters: 02981 * 02982 * struct { 02983 * ECParameters curve_params; 02984 * ECPoint public; 02985 * } ServerECDHParams; 02986 */ 02987 const mbedtls_ecp_curve_info **curve = NULL; 02988 const mbedtls_ecp_group_id *gid; 02989 02990 /* Match our preference list against the offered curves */ 02991 for( gid = ssl->conf->curve_list; *gid != MBEDTLS_ECP_DP_NONE; gid++ ) 02992 for( curve = ssl->handshake->curves; *curve != NULL; curve++ ) 02993 if( (*curve)->grp_id == *gid ) 02994 goto curve_matching_done; 02995 02996 curve_matching_done: 02997 if( curve == NULL || *curve == NULL ) 02998 { 02999 MBEDTLS_SSL_DEBUG_MSG( 1, ( "no matching curve for ECDHE" ) ); 03000 return( MBEDTLS_ERR_SSL_NO_CIPHER_CHOSEN ); 03001 } 03002 03003 MBEDTLS_SSL_DEBUG_MSG( 2, ( "ECDHE curve: %s", (*curve)->name ) ); 03004 03005 if( ( ret = mbedtls_ecp_group_load( &ssl->handshake->ecdh_ctx.grp, 03006 (*curve)->grp_id ) ) != 0 ) 03007 { 03008 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecp_group_load", ret ); 03009 return( ret ); 03010 } 03011 03012 if( ( ret = mbedtls_ecdh_make_params( &ssl->handshake->ecdh_ctx, &len, 03013 p, MBEDTLS_SSL_MAX_CONTENT_LEN - n, 03014 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 03015 { 03016 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_make_params", ret ); 03017 return( ret ); 03018 } 03019 03020 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_SERVER_SIGNATURE__ENABLED) 03021 dig_signed = p; 03022 dig_signed_len = len; 03023 #endif 03024 03025 p += len; 03026 n += len; 03027 03028 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Q ", &ssl->handshake->ecdh_ctx.Q ); 03029 } 03030 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__ECDHE_ENABLED */ 03031 03032 /* 03033 * 03034 * Part 3: For key exchanges involving the server signing the 03035 * exchange parameters, compute and add the signature here. 03036 * 03037 */ 03038 #if defined(MBEDTLS_KEY_EXCHANGE__WITH_SERVER_SIGNATURE__ENABLED) 03039 if( mbedtls_ssl_ciphersuite_uses_server_signature( ciphersuite_info ) ) 03040 { 03041 size_t signature_len = 0; 03042 unsigned int hashlen = 0; 03043 unsigned char hash[64]; 03044 03045 /* 03046 * 3.1: Choose hash algorithm: 03047 * A: For TLS 1.2, obey signature-hash-algorithm extension 03048 * to choose appropriate hash. 03049 * B: For SSL3, TLS1.0, TLS1.1 and ECDHE_ECDSA, use SHA1 03050 * (RFC 4492, Sec. 5.4) 03051 * C: Otherwise, use MD5 + SHA1 (RFC 4346, Sec. 7.4.3) 03052 */ 03053 03054 mbedtls_md_type_t md_alg; 03055 03056 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 03057 mbedtls_pk_type_t sig_alg = 03058 mbedtls_ssl_get_ciphersuite_sig_pk_alg( ciphersuite_info ); 03059 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 03060 { 03061 /* A: For TLS 1.2, obey signature-hash-algorithm extension 03062 * (RFC 5246, Sec. 7.4.1.4.1). */ 03063 if( sig_alg == MBEDTLS_PK_NONE || 03064 ( md_alg = mbedtls_ssl_sig_hash_set_find( &ssl->handshake->hash_algs, 03065 sig_alg ) ) == MBEDTLS_MD_NONE ) 03066 { 03067 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03068 /* (... because we choose a cipher suite 03069 * only if there is a matching hash.) */ 03070 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03071 } 03072 } 03073 else 03074 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 03075 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 03076 defined(MBEDTLS_SSL_PROTO_TLS1_1) 03077 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA ) 03078 { 03079 /* B: Default hash SHA1 */ 03080 md_alg = MBEDTLS_MD_SHA1; 03081 } 03082 else 03083 #endif /* MBEDTLS_SSL_PROTO_SSL3 || MBEDTLS_SSL_PROTO_TLS1 || \ 03084 MBEDTLS_SSL_PROTO_TLS1_1 */ 03085 { 03086 /* C: MD5 + SHA1 */ 03087 md_alg = MBEDTLS_MD_NONE; 03088 } 03089 03090 MBEDTLS_SSL_DEBUG_MSG( 3, ( "pick hash algorithm %d for signing", md_alg ) ); 03091 03092 /* 03093 * 3.2: Compute the hash to be signed 03094 */ 03095 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 03096 defined(MBEDTLS_SSL_PROTO_TLS1_1) 03097 if( md_alg == MBEDTLS_MD_NONE ) 03098 { 03099 mbedtls_md5_context mbedtls_md5; 03100 mbedtls_sha1_context mbedtls_sha1; 03101 03102 mbedtls_md5_init( &mbedtls_md5 ); 03103 mbedtls_sha1_init( &mbedtls_sha1 ); 03104 03105 /* 03106 * digitally-signed struct { 03107 * opaque md5_hash[16]; 03108 * opaque sha_hash[20]; 03109 * }; 03110 * 03111 * md5_hash 03112 * MD5(ClientHello.random + ServerHello.random 03113 * + ServerParams); 03114 * sha_hash 03115 * SHA(ClientHello.random + ServerHello.random 03116 * + ServerParams); 03117 */ 03118 03119 mbedtls_md5_starts( &mbedtls_md5 ); 03120 mbedtls_md5_update( &mbedtls_md5, ssl->handshake->randbytes, 64 ); 03121 mbedtls_md5_update( &mbedtls_md5, dig_signed, dig_signed_len ); 03122 mbedtls_md5_finish( &mbedtls_md5, hash ); 03123 03124 mbedtls_sha1_starts( &mbedtls_sha1 ); 03125 mbedtls_sha1_update( &mbedtls_sha1, ssl->handshake->randbytes, 64 ); 03126 mbedtls_sha1_update( &mbedtls_sha1, dig_signed, dig_signed_len ); 03127 mbedtls_sha1_finish( &mbedtls_sha1, hash + 16 ); 03128 03129 hashlen = 36; 03130 03131 mbedtls_md5_free( &mbedtls_md5 ); 03132 mbedtls_sha1_free( &mbedtls_sha1 ); 03133 } 03134 else 03135 #endif /* MBEDTLS_SSL_PROTO_SSL3 || MBEDTLS_SSL_PROTO_TLS1 || \ 03136 MBEDTLS_SSL_PROTO_TLS1_1 */ 03137 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 03138 defined(MBEDTLS_SSL_PROTO_TLS1_2) 03139 if( md_alg != MBEDTLS_MD_NONE ) 03140 { 03141 mbedtls_md_context_t ctx; 03142 const mbedtls_md_info_t *md_info = mbedtls_md_info_from_type( md_alg ); 03143 03144 mbedtls_md_init( &ctx ); 03145 03146 /* Info from md_alg will be used instead */ 03147 hashlen = 0; 03148 03149 /* 03150 * digitally-signed struct { 03151 * opaque client_random[32]; 03152 * opaque server_random[32]; 03153 * ServerDHParams params; 03154 * }; 03155 */ 03156 if( ( ret = mbedtls_md_setup( &ctx, md_info, 0 ) ) != 0 ) 03157 { 03158 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_md_setup", ret ); 03159 return( ret ); 03160 } 03161 03162 mbedtls_md_starts( &ctx ); 03163 mbedtls_md_update( &ctx, ssl->handshake->randbytes, 64 ); 03164 mbedtls_md_update( &ctx, dig_signed, dig_signed_len ); 03165 mbedtls_md_finish( &ctx, hash ); 03166 mbedtls_md_free( &ctx ); 03167 } 03168 else 03169 #endif /* MBEDTLS_SSL_PROTO_TLS1 || MBEDTLS_SSL_PROTO_TLS1_1 || \ 03170 MBEDTLS_SSL_PROTO_TLS1_2 */ 03171 { 03172 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03173 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03174 } 03175 03176 MBEDTLS_SSL_DEBUG_BUF( 3, "parameters hash", hash, hashlen != 0 ? hashlen : 03177 (unsigned int) ( mbedtls_md_get_size( mbedtls_md_info_from_type( md_alg ) ) ) ); 03178 03179 /* 03180 * 3.3: Compute and add the signature 03181 */ 03182 if( mbedtls_ssl_own_key( ssl ) == NULL ) 03183 { 03184 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no private key" ) ); 03185 return( MBEDTLS_ERR_SSL_PRIVATE_KEY_REQUIRED ); 03186 } 03187 03188 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 03189 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 03190 { 03191 /* 03192 * For TLS 1.2, we need to specify signature and hash algorithm 03193 * explicitly through a prefix to the signature. 03194 * 03195 * struct { 03196 * HashAlgorithm hash; 03197 * SignatureAlgorithm signature; 03198 * } SignatureAndHashAlgorithm; 03199 * 03200 * struct { 03201 * SignatureAndHashAlgorithm algorithm; 03202 * opaque signature<0..2^16-1>; 03203 * } DigitallySigned; 03204 * 03205 */ 03206 03207 *(p++) = mbedtls_ssl_hash_from_md_alg( md_alg ); 03208 *(p++) = mbedtls_ssl_sig_from_pk_alg( sig_alg ); 03209 03210 n += 2; 03211 } 03212 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 03213 03214 if( ( ret = mbedtls_pk_sign( mbedtls_ssl_own_key( ssl ), md_alg, hash, hashlen, 03215 p + 2 , &signature_len, ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 03216 { 03217 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_pk_sign", ret ); 03218 return( ret ); 03219 } 03220 03221 *(p++) = (unsigned char)( signature_len >> 8 ); 03222 *(p++) = (unsigned char)( signature_len ); 03223 n += 2; 03224 03225 MBEDTLS_SSL_DEBUG_BUF( 3, "my signature", p, signature_len ); 03226 03227 n += signature_len; 03228 } 03229 #endif /* MBEDTLS_KEY_EXCHANGE__WITH_SERVER_SIGNATURE__ENABLED */ 03230 03231 /* Done with actual work; add header and send. */ 03232 03233 ssl->out_msglen = 4 + n; 03234 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 03235 ssl->out_msg[0] = MBEDTLS_SSL_HS_SERVER_KEY_EXCHANGE; 03236 03237 ssl->state++; 03238 03239 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03240 { 03241 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03242 return( ret ); 03243 } 03244 03245 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write server key exchange" ) ); 03246 03247 return( 0 ); 03248 } 03249 03250 static int ssl_write_server_hello_done( mbedtls_ssl_context *ssl ) 03251 { 03252 int ret; 03253 03254 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write server hello done" ) ); 03255 03256 ssl->out_msglen = 4; 03257 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 03258 ssl->out_msg[0] = MBEDTLS_SSL_HS_SERVER_HELLO_DONE; 03259 03260 ssl->state++; 03261 03262 #if defined(MBEDTLS_SSL_PROTO_DTLS) 03263 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM ) 03264 mbedtls_ssl_send_flight_completed( ssl ); 03265 #endif 03266 03267 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03268 { 03269 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03270 return( ret ); 03271 } 03272 03273 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write server hello done" ) ); 03274 03275 return( 0 ); 03276 } 03277 03278 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) || \ 03279 defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 03280 static int ssl_parse_client_dh_public( mbedtls_ssl_context *ssl, unsigned char **p, 03281 const unsigned char *end ) 03282 { 03283 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 03284 size_t n; 03285 03286 /* 03287 * Receive G^Y mod P, premaster = (G^Y)^X mod P 03288 */ 03289 if( *p + 2 > end ) 03290 { 03291 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03292 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03293 } 03294 03295 n = ( (*p)[0] << 8 ) | (*p)[1]; 03296 *p += 2; 03297 03298 if( *p + n > end ) 03299 { 03300 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03301 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03302 } 03303 03304 if( ( ret = mbedtls_dhm_read_public( &ssl->handshake->dhm_ctx, *p, n ) ) != 0 ) 03305 { 03306 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_read_public", ret ); 03307 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_RP ); 03308 } 03309 03310 *p += n; 03311 03312 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: GY", &ssl->handshake->dhm_ctx.GY ); 03313 03314 return( ret ); 03315 } 03316 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED || 03317 MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 03318 03319 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) || \ 03320 defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 03321 static int ssl_parse_encrypted_pms( mbedtls_ssl_context *ssl, 03322 const unsigned char *p, 03323 const unsigned char *end, 03324 size_t pms_offset ) 03325 { 03326 int ret; 03327 size_t len = mbedtls_pk_get_len( mbedtls_ssl_own_key( ssl ) ); 03328 unsigned char *pms = ssl->handshake->premaster + pms_offset; 03329 unsigned char ver[2]; 03330 unsigned char fake_pms[48], peer_pms[48]; 03331 unsigned char mask; 03332 size_t i, peer_pmslen; 03333 unsigned int diff; 03334 03335 if( ! mbedtls_pk_can_do( mbedtls_ssl_own_key( ssl ), MBEDTLS_PK_RSA ) ) 03336 { 03337 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no RSA private key" ) ); 03338 return( MBEDTLS_ERR_SSL_PRIVATE_KEY_REQUIRED ); 03339 } 03340 03341 /* 03342 * Decrypt the premaster using own private RSA key 03343 */ 03344 #if defined(MBEDTLS_SSL_PROTO_TLS1) || defined(MBEDTLS_SSL_PROTO_TLS1_1) || \ 03345 defined(MBEDTLS_SSL_PROTO_TLS1_2) 03346 if( ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_0 ) 03347 { 03348 if( *p++ != ( ( len >> 8 ) & 0xFF ) || 03349 *p++ != ( ( len ) & 0xFF ) ) 03350 { 03351 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03352 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03353 } 03354 } 03355 #endif 03356 03357 if( p + len != end ) 03358 { 03359 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03360 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03361 } 03362 03363 mbedtls_ssl_write_version( ssl->handshake->max_major_ver, 03364 ssl->handshake->max_minor_ver, 03365 ssl->conf->transport, ver ); 03366 03367 /* 03368 * Protection against Bleichenbacher's attack: invalid PKCS#1 v1.5 padding 03369 * must not cause the connection to end immediately; instead, send a 03370 * bad_record_mac later in the handshake. 03371 * Also, avoid data-dependant branches here to protect against 03372 * timing-based variants. 03373 */ 03374 ret = ssl->conf->f_rng( ssl->conf->p_rng, fake_pms, sizeof( fake_pms ) ); 03375 if( ret != 0 ) 03376 return( ret ); 03377 03378 ret = mbedtls_pk_decrypt( mbedtls_ssl_own_key( ssl ), p, len, 03379 peer_pms, &peer_pmslen, 03380 sizeof( peer_pms ), 03381 ssl->conf->f_rng, ssl->conf->p_rng ); 03382 03383 diff = (unsigned int) ret; 03384 diff |= peer_pmslen ^ 48; 03385 diff |= peer_pms[0] ^ ver[0]; 03386 diff |= peer_pms[1] ^ ver[1]; 03387 03388 #if defined(MBEDTLS_SSL_DEBUG_ALL) 03389 if( diff != 0 ) 03390 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03391 #endif 03392 03393 if( sizeof( ssl->handshake->premaster ) < pms_offset || 03394 sizeof( ssl->handshake->premaster ) - pms_offset < 48 ) 03395 { 03396 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03397 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03398 } 03399 ssl->handshake->pmslen = 48; 03400 03401 /* mask = diff ? 0xff : 0x00 using bit operations to avoid branches */ 03402 /* MSVC has a warning about unary minus on unsigned, but this is 03403 * well-defined and precisely what we want to do here */ 03404 #if defined(_MSC_VER) 03405 #pragma warning( push ) 03406 #pragma warning( disable : 4146 ) 03407 #endif 03408 mask = - ( ( diff | - diff ) >> ( sizeof( unsigned int ) * 8 - 1 ) ); 03409 #if defined(_MSC_VER) 03410 #pragma warning( pop ) 03411 #endif 03412 03413 for( i = 0; i < ssl->handshake->pmslen; i++ ) 03414 pms[i] = ( mask & fake_pms[i] ) | ( (~mask) & peer_pms[i] ); 03415 03416 return( 0 ); 03417 } 03418 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_ENABLED || 03419 MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED */ 03420 03421 #if defined(MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED) 03422 static int ssl_parse_client_psk_identity( mbedtls_ssl_context *ssl, unsigned char **p, 03423 const unsigned char *end ) 03424 { 03425 int ret = 0; 03426 size_t n; 03427 03428 if( ssl->conf->f_psk == NULL && 03429 ( ssl->conf->psk == NULL || ssl->conf->psk_identity == NULL || 03430 ssl->conf->psk_identity_len == 0 || ssl->conf->psk_len == 0 ) ) 03431 { 03432 MBEDTLS_SSL_DEBUG_MSG( 1, ( "got no pre-shared key" ) ); 03433 return( MBEDTLS_ERR_SSL_PRIVATE_KEY_REQUIRED ); 03434 } 03435 03436 /* 03437 * Receive client pre-shared key identity name 03438 */ 03439 if( *p + 2 > end ) 03440 { 03441 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03442 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03443 } 03444 03445 n = ( (*p)[0] << 8 ) | (*p)[1]; 03446 *p += 2; 03447 03448 if( n < 1 || n > 65535 || *p + n > end ) 03449 { 03450 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03451 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03452 } 03453 03454 if( ssl->conf->f_psk != NULL ) 03455 { 03456 if( ssl->conf->f_psk( ssl->conf->p_psk, ssl, *p, n ) != 0 ) 03457 ret = MBEDTLS_ERR_SSL_UNKNOWN_IDENTITY; 03458 } 03459 else 03460 { 03461 /* Identity is not a big secret since clients send it in the clear, 03462 * but treat it carefully anyway, just in case */ 03463 if( n != ssl->conf->psk_identity_len || 03464 mbedtls_ssl_safer_memcmp( ssl->conf->psk_identity, *p, n ) != 0 ) 03465 { 03466 ret = MBEDTLS_ERR_SSL_UNKNOWN_IDENTITY; 03467 } 03468 } 03469 03470 if( ret == MBEDTLS_ERR_SSL_UNKNOWN_IDENTITY ) 03471 { 03472 MBEDTLS_SSL_DEBUG_BUF( 3, "Unknown PSK identity", *p, n ); 03473 mbedtls_ssl_send_alert_message( ssl, MBEDTLS_SSL_ALERT_LEVEL_FATAL, 03474 MBEDTLS_SSL_ALERT_MSG_UNKNOWN_PSK_IDENTITY ); 03475 return( MBEDTLS_ERR_SSL_UNKNOWN_IDENTITY ); 03476 } 03477 03478 *p += n; 03479 03480 return( 0 ); 03481 } 03482 #endif /* MBEDTLS_KEY_EXCHANGE__SOME__PSK_ENABLED */ 03483 03484 static int ssl_parse_client_key_exchange( mbedtls_ssl_context *ssl ) 03485 { 03486 int ret; 03487 const mbedtls_ssl_ciphersuite_t *ciphersuite_info; 03488 unsigned char *p, *end; 03489 03490 ciphersuite_info = ssl->transform_negotiate->ciphersuite_info; 03491 03492 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse client key exchange" ) ); 03493 03494 if( ( ret = mbedtls_ssl_read_record( ssl ) ) != 0 ) 03495 { 03496 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_read_record", ret ); 03497 return( ret ); 03498 } 03499 03500 p = ssl->in_msg + mbedtls_ssl_hs_hdr_len( ssl ); 03501 end = ssl->in_msg + ssl->in_hslen; 03502 03503 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE ) 03504 { 03505 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03506 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03507 } 03508 03509 if( ssl->in_msg[0] != MBEDTLS_SSL_HS_CLIENT_KEY_EXCHANGE ) 03510 { 03511 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange message" ) ); 03512 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03513 } 03514 03515 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) 03516 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_RSA ) 03517 { 03518 if( ( ret = ssl_parse_client_dh_public( ssl, &p, end ) ) != 0 ) 03519 { 03520 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_dh_public" ), ret ); 03521 return( ret ); 03522 } 03523 03524 if( p != end ) 03525 { 03526 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange" ) ); 03527 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03528 } 03529 03530 if( ( ret = mbedtls_dhm_calc_secret( &ssl->handshake->dhm_ctx, 03531 ssl->handshake->premaster, 03532 MBEDTLS_PREMASTER_SIZE, 03533 &ssl->handshake->pmslen, 03534 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 03535 { 03536 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_dhm_calc_secret", ret ); 03537 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_CS ); 03538 } 03539 03540 MBEDTLS_SSL_DEBUG_MPI( 3, "DHM: K ", &ssl->handshake->dhm_ctx.K ); 03541 } 03542 else 03543 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED */ 03544 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) || \ 03545 defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) || \ 03546 defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) || \ 03547 defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED) 03548 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_RSA || 03549 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA || 03550 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_RSA || 03551 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA ) 03552 { 03553 if( ( ret = mbedtls_ecdh_read_public( &ssl->handshake->ecdh_ctx, 03554 p, end - p) ) != 0 ) 03555 { 03556 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_read_public", ret ); 03557 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_RP ); 03558 } 03559 03560 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Qp ", &ssl->handshake->ecdh_ctx.Qp ); 03561 03562 if( ( ret = mbedtls_ecdh_calc_secret( &ssl->handshake->ecdh_ctx, 03563 &ssl->handshake->pmslen, 03564 ssl->handshake->premaster, 03565 MBEDTLS_MPI_MAX_SIZE, 03566 ssl->conf->f_rng, ssl->conf->p_rng ) ) != 0 ) 03567 { 03568 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_calc_secret", ret ); 03569 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_CS ); 03570 } 03571 03572 MBEDTLS_SSL_DEBUG_MPI( 3, "ECDH: z ", &ssl->handshake->ecdh_ctx.z ); 03573 } 03574 else 03575 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED || 03576 MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED || 03577 MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED || 03578 MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED */ 03579 #if defined(MBEDTLS_KEY_EXCHANGE_PSK_ENABLED) 03580 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK ) 03581 { 03582 if( ( ret = ssl_parse_client_psk_identity( ssl, &p, end ) ) != 0 ) 03583 { 03584 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_psk_identity" ), ret ); 03585 return( ret ); 03586 } 03587 03588 if( p != end ) 03589 { 03590 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange" ) ); 03591 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03592 } 03593 03594 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 03595 ciphersuite_info->key_exchange ) ) != 0 ) 03596 { 03597 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 03598 return( ret ); 03599 } 03600 } 03601 else 03602 #endif /* MBEDTLS_KEY_EXCHANGE_PSK_ENABLED */ 03603 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED) 03604 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK ) 03605 { 03606 if( ( ret = ssl_parse_client_psk_identity( ssl, &p, end ) ) != 0 ) 03607 { 03608 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_psk_identity" ), ret ); 03609 return( ret ); 03610 } 03611 03612 if( ( ret = ssl_parse_encrypted_pms( ssl, p, end, 2 ) ) != 0 ) 03613 { 03614 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_encrypted_pms" ), ret ); 03615 return( ret ); 03616 } 03617 03618 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 03619 ciphersuite_info->key_exchange ) ) != 0 ) 03620 { 03621 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 03622 return( ret ); 03623 } 03624 } 03625 else 03626 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED */ 03627 #if defined(MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED) 03628 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK ) 03629 { 03630 if( ( ret = ssl_parse_client_psk_identity( ssl, &p, end ) ) != 0 ) 03631 { 03632 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_psk_identity" ), ret ); 03633 return( ret ); 03634 } 03635 if( ( ret = ssl_parse_client_dh_public( ssl, &p, end ) ) != 0 ) 03636 { 03637 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_dh_public" ), ret ); 03638 return( ret ); 03639 } 03640 03641 if( p != end ) 03642 { 03643 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad client key exchange" ) ); 03644 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE ); 03645 } 03646 03647 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 03648 ciphersuite_info->key_exchange ) ) != 0 ) 03649 { 03650 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 03651 return( ret ); 03652 } 03653 } 03654 else 03655 #endif /* MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED */ 03656 #if defined(MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED) 03657 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK ) 03658 { 03659 if( ( ret = ssl_parse_client_psk_identity( ssl, &p, end ) ) != 0 ) 03660 { 03661 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_client_psk_identity" ), ret ); 03662 return( ret ); 03663 } 03664 03665 if( ( ret = mbedtls_ecdh_read_public( &ssl->handshake->ecdh_ctx, 03666 p, end - p ) ) != 0 ) 03667 { 03668 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecdh_read_public", ret ); 03669 return( MBEDTLS_ERR_SSL_BAD_HS_CLIENT_KEY_EXCHANGE_RP ); 03670 } 03671 03672 MBEDTLS_SSL_DEBUG_ECP( 3, "ECDH: Qp ", &ssl->handshake->ecdh_ctx.Qp ); 03673 03674 if( ( ret = mbedtls_ssl_psk_derive_premaster( ssl, 03675 ciphersuite_info->key_exchange ) ) != 0 ) 03676 { 03677 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_psk_derive_premaster", ret ); 03678 return( ret ); 03679 } 03680 } 03681 else 03682 #endif /* MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED */ 03683 #if defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) 03684 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA ) 03685 { 03686 if( ( ret = ssl_parse_encrypted_pms( ssl, p, end, 0 ) ) != 0 ) 03687 { 03688 MBEDTLS_SSL_DEBUG_RET( 1, ( "ssl_parse_parse_encrypted_pms_secret" ), ret ); 03689 return( ret ); 03690 } 03691 } 03692 else 03693 #endif /* MBEDTLS_KEY_EXCHANGE_RSA_ENABLED */ 03694 #if defined(MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED) 03695 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 03696 { 03697 ret = mbedtls_ecjpake_read_round_two( &ssl->handshake->ecjpake_ctx, 03698 p, end - p ); 03699 if( ret != 0 ) 03700 { 03701 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_read_round_two", ret ); 03702 return( MBEDTLS_ERR_SSL_BAD_HS_SERVER_KEY_EXCHANGE ); 03703 } 03704 03705 ret = mbedtls_ecjpake_derive_secret( &ssl->handshake->ecjpake_ctx, 03706 ssl->handshake->premaster, 32, &ssl->handshake->pmslen, 03707 ssl->conf->f_rng, ssl->conf->p_rng ); 03708 if( ret != 0 ) 03709 { 03710 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ecjpake_derive_secret", ret ); 03711 return( ret ); 03712 } 03713 } 03714 else 03715 #endif /* MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED */ 03716 { 03717 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03718 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03719 } 03720 03721 if( ( ret = mbedtls_ssl_derive_keys( ssl ) ) != 0 ) 03722 { 03723 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_derive_keys", ret ); 03724 return( ret ); 03725 } 03726 03727 ssl->state++; 03728 03729 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse client key exchange" ) ); 03730 03731 return( 0 ); 03732 } 03733 03734 #if !defined(MBEDTLS_KEY_EXCHANGE_RSA_ENABLED) && \ 03735 !defined(MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED) && \ 03736 !defined(MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED) && \ 03737 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED) && \ 03738 !defined(MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED)&& \ 03739 !defined(MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED) 03740 static int ssl_parse_certificate_verify( mbedtls_ssl_context *ssl ) 03741 { 03742 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 03743 ssl->transform_negotiate->ciphersuite_info; 03744 03745 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse certificate verify" ) ); 03746 03747 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 03748 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 03749 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 03750 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 03751 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE ) 03752 { 03753 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate verify" ) ); 03754 ssl->state++; 03755 return( 0 ); 03756 } 03757 03758 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03759 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03760 } 03761 #else 03762 static int ssl_parse_certificate_verify( mbedtls_ssl_context *ssl ) 03763 { 03764 int ret = MBEDTLS_ERR_SSL_FEATURE_UNAVAILABLE; 03765 size_t i, sig_len; 03766 unsigned char hash[48]; 03767 unsigned char *hash_start = hash; 03768 size_t hashlen; 03769 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 03770 mbedtls_pk_type_t pk_alg; 03771 #endif 03772 mbedtls_md_type_t md_alg; 03773 const mbedtls_ssl_ciphersuite_t *ciphersuite_info = 03774 ssl->transform_negotiate->ciphersuite_info; 03775 03776 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> parse certificate verify" ) ); 03777 03778 if( ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_PSK || 03779 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_RSA_PSK || 03780 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECDHE_PSK || 03781 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_DHE_PSK || 03782 ciphersuite_info->key_exchange == MBEDTLS_KEY_EXCHANGE_ECJPAKE || 03783 ssl->session_negotiate->peer_cert == NULL ) 03784 { 03785 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= skip parse certificate verify" ) ); 03786 ssl->state++; 03787 return( 0 ); 03788 } 03789 03790 /* Read the message without adding it to the checksum */ 03791 do { 03792 03793 if( ( ret = mbedtls_ssl_read_record_layer( ssl ) ) != 0 ) 03794 { 03795 MBEDTLS_SSL_DEBUG_RET( 1, ( "mbedtls_ssl_read_record_layer" ), ret ); 03796 return( ret ); 03797 } 03798 03799 ret = mbedtls_ssl_handle_message_type( ssl ); 03800 03801 } while( MBEDTLS_ERR_SSL_NON_FATAL == ret ); 03802 03803 if( 0 != ret ) 03804 { 03805 MBEDTLS_SSL_DEBUG_RET( 1, ( "mbedtls_ssl_handle_message_type" ), ret ); 03806 return( ret ); 03807 } 03808 03809 ssl->state++; 03810 03811 /* Process the message contents */ 03812 if( ssl->in_msgtype != MBEDTLS_SSL_MSG_HANDSHAKE || 03813 ssl->in_msg[0] != MBEDTLS_SSL_HS_CERTIFICATE_VERIFY ) 03814 { 03815 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate verify message" ) ); 03816 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03817 } 03818 03819 i = mbedtls_ssl_hs_hdr_len( ssl ); 03820 03821 /* 03822 * struct { 03823 * SignatureAndHashAlgorithm algorithm; -- TLS 1.2 only 03824 * opaque signature<0..2^16-1>; 03825 * } DigitallySigned; 03826 */ 03827 #if defined(MBEDTLS_SSL_PROTO_SSL3) || defined(MBEDTLS_SSL_PROTO_TLS1) || \ 03828 defined(MBEDTLS_SSL_PROTO_TLS1_1) 03829 if( ssl->minor_ver != MBEDTLS_SSL_MINOR_VERSION_3 ) 03830 { 03831 md_alg = MBEDTLS_MD_NONE; 03832 hashlen = 36; 03833 03834 /* For ECDSA, use SHA-1, not MD-5 + SHA-1 */ 03835 if( mbedtls_pk_can_do( &ssl->session_negotiate->peer_cert->pk, 03836 MBEDTLS_PK_ECDSA ) ) 03837 { 03838 hash_start += 16; 03839 hashlen -= 16; 03840 md_alg = MBEDTLS_MD_SHA1; 03841 } 03842 } 03843 else 03844 #endif /* MBEDTLS_SSL_PROTO_SSL3 || MBEDTLS_SSL_PROTO_TLS1 || 03845 MBEDTLS_SSL_PROTO_TLS1_1 */ 03846 #if defined(MBEDTLS_SSL_PROTO_TLS1_2) 03847 if( ssl->minor_ver == MBEDTLS_SSL_MINOR_VERSION_3 ) 03848 { 03849 if( i + 2 > ssl->in_hslen ) 03850 { 03851 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate verify message" ) ); 03852 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03853 } 03854 03855 /* 03856 * Hash 03857 */ 03858 md_alg = mbedtls_ssl_md_alg_from_hash( ssl->in_msg[i] ); 03859 03860 if( md_alg == MBEDTLS_MD_NONE || mbedtls_ssl_set_calc_verify_md( ssl, ssl->in_msg[i] ) ) 03861 { 03862 MBEDTLS_SSL_DEBUG_MSG( 1, ( "peer not adhering to requested sig_alg" 03863 " for verify message" ) ); 03864 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03865 } 03866 03867 #if !defined(MBEDTLS_MD_SHA1) 03868 if( MBEDTLS_MD_SHA1 == md_alg ) 03869 hash_start += 16; 03870 #endif 03871 03872 /* Info from md_alg will be used instead */ 03873 hashlen = 0; 03874 03875 i++; 03876 03877 /* 03878 * Signature 03879 */ 03880 if( ( pk_alg = mbedtls_ssl_pk_alg_from_sig( ssl->in_msg[i] ) ) 03881 == MBEDTLS_PK_NONE ) 03882 { 03883 MBEDTLS_SSL_DEBUG_MSG( 1, ( "peer not adhering to requested sig_alg" 03884 " for verify message" ) ); 03885 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03886 } 03887 03888 /* 03889 * Check the certificate's key type matches the signature alg 03890 */ 03891 if( ! mbedtls_pk_can_do( &ssl->session_negotiate->peer_cert->pk, pk_alg ) ) 03892 { 03893 MBEDTLS_SSL_DEBUG_MSG( 1, ( "sig_alg doesn't match cert key" ) ); 03894 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03895 } 03896 03897 i++; 03898 } 03899 else 03900 #endif /* MBEDTLS_SSL_PROTO_TLS1_2 */ 03901 { 03902 MBEDTLS_SSL_DEBUG_MSG( 1, ( "should never happen" ) ); 03903 return( MBEDTLS_ERR_SSL_INTERNAL_ERROR ); 03904 } 03905 03906 if( i + 2 > ssl->in_hslen ) 03907 { 03908 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate verify message" ) ); 03909 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03910 } 03911 03912 sig_len = ( ssl->in_msg[i] << 8 ) | ssl->in_msg[i+1]; 03913 i += 2; 03914 03915 if( i + sig_len != ssl->in_hslen ) 03916 { 03917 MBEDTLS_SSL_DEBUG_MSG( 1, ( "bad certificate verify message" ) ); 03918 return( MBEDTLS_ERR_SSL_BAD_HS_CERTIFICATE_VERIFY ); 03919 } 03920 03921 /* Calculate hash and verify signature */ 03922 ssl->handshake->calc_verify( ssl, hash ); 03923 03924 if( ( ret = mbedtls_pk_verify( &ssl->session_negotiate->peer_cert->pk, 03925 md_alg, hash_start, hashlen, 03926 ssl->in_msg + i, sig_len ) ) != 0 ) 03927 { 03928 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_pk_verify", ret ); 03929 return( ret ); 03930 } 03931 03932 mbedtls_ssl_update_handshake_status( ssl ); 03933 03934 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= parse certificate verify" ) ); 03935 03936 return( ret ); 03937 } 03938 #endif /* !MBEDTLS_KEY_EXCHANGE_RSA_ENABLED && 03939 !MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED && 03940 !MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED && 03941 !MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED && 03942 !MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED && 03943 !MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED */ 03944 03945 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 03946 static int ssl_write_new_session_ticket( mbedtls_ssl_context *ssl ) 03947 { 03948 int ret; 03949 size_t tlen; 03950 uint32_t lifetime; 03951 03952 MBEDTLS_SSL_DEBUG_MSG( 2, ( "=> write new session ticket" ) ); 03953 03954 ssl->out_msgtype = MBEDTLS_SSL_MSG_HANDSHAKE; 03955 ssl->out_msg[0] = MBEDTLS_SSL_HS_NEW_SESSION_TICKET; 03956 03957 /* 03958 * struct { 03959 * uint32 ticket_lifetime_hint; 03960 * opaque ticket<0..2^16-1>; 03961 * } NewSessionTicket; 03962 * 03963 * 4 . 7 ticket_lifetime_hint (0 = unspecified) 03964 * 8 . 9 ticket_len (n) 03965 * 10 . 9+n ticket content 03966 */ 03967 03968 if( ( ret = ssl->conf->f_ticket_write( ssl->conf->p_ticket, 03969 ssl->session_negotiate, 03970 ssl->out_msg + 10, 03971 ssl->out_msg + MBEDTLS_SSL_MAX_CONTENT_LEN, 03972 &tlen, &lifetime ) ) != 0 ) 03973 { 03974 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_ticket_write", ret ); 03975 tlen = 0; 03976 } 03977 03978 ssl->out_msg[4] = ( lifetime >> 24 ) & 0xFF; 03979 ssl->out_msg[5] = ( lifetime >> 16 ) & 0xFF; 03980 ssl->out_msg[6] = ( lifetime >> 8 ) & 0xFF; 03981 ssl->out_msg[7] = ( lifetime ) & 0xFF; 03982 03983 ssl->out_msg[8] = (unsigned char)( ( tlen >> 8 ) & 0xFF ); 03984 ssl->out_msg[9] = (unsigned char)( ( tlen ) & 0xFF ); 03985 03986 ssl->out_msglen = 10 + tlen; 03987 03988 /* 03989 * Morally equivalent to updating ssl->state, but NewSessionTicket and 03990 * ChangeCipherSpec share the same state. 03991 */ 03992 ssl->handshake->new_session_ticket = 0; 03993 03994 if( ( ret = mbedtls_ssl_write_record( ssl ) ) != 0 ) 03995 { 03996 MBEDTLS_SSL_DEBUG_RET( 1, "mbedtls_ssl_write_record", ret ); 03997 return( ret ); 03998 } 03999 04000 MBEDTLS_SSL_DEBUG_MSG( 2, ( "<= write new session ticket" ) ); 04001 04002 return( 0 ); 04003 } 04004 #endif /* MBEDTLS_SSL_SESSION_TICKETS */ 04005 04006 /* 04007 * SSL handshake -- server side -- single step 04008 */ 04009 int mbedtls_ssl_handshake_server_step( mbedtls_ssl_context *ssl ) 04010 { 04011 int ret = 0; 04012 04013 if( ssl->state == MBEDTLS_SSL_HANDSHAKE_OVER || ssl->handshake == NULL ) 04014 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 04015 04016 MBEDTLS_SSL_DEBUG_MSG( 2, ( "server state: %d", ssl->state ) ); 04017 04018 if( ( ret = mbedtls_ssl_flush_output( ssl ) ) != 0 ) 04019 return( ret ); 04020 04021 #if defined(MBEDTLS_SSL_PROTO_DTLS) 04022 if( ssl->conf->transport == MBEDTLS_SSL_TRANSPORT_DATAGRAM && 04023 ssl->handshake->retransmit_state == MBEDTLS_SSL_RETRANS_SENDING ) 04024 { 04025 if( ( ret = mbedtls_ssl_resend( ssl ) ) != 0 ) 04026 return( ret ); 04027 } 04028 #endif 04029 04030 switch( ssl->state ) 04031 { 04032 case MBEDTLS_SSL_HELLO_REQUEST: 04033 ssl->state = MBEDTLS_SSL_CLIENT_HELLO; 04034 break; 04035 04036 /* 04037 * <== ClientHello 04038 */ 04039 case MBEDTLS_SSL_CLIENT_HELLO: 04040 ret = ssl_parse_client_hello( ssl ); 04041 break; 04042 04043 #if defined(MBEDTLS_SSL_PROTO_DTLS) 04044 case MBEDTLS_SSL_SERVER_HELLO_VERIFY_REQUEST_SENT: 04045 return( MBEDTLS_ERR_SSL_HELLO_VERIFY_REQUIRED ); 04046 #endif 04047 04048 /* 04049 * ==> ServerHello 04050 * Certificate 04051 * ( ServerKeyExchange ) 04052 * ( CertificateRequest ) 04053 * ServerHelloDone 04054 */ 04055 case MBEDTLS_SSL_SERVER_HELLO: 04056 ret = ssl_write_server_hello( ssl ); 04057 break; 04058 04059 case MBEDTLS_SSL_SERVER_CERTIFICATE: 04060 ret = mbedtls_ssl_write_certificate( ssl ); 04061 break; 04062 04063 case MBEDTLS_SSL_SERVER_KEY_EXCHANGE: 04064 ret = ssl_write_server_key_exchange( ssl ); 04065 break; 04066 04067 case MBEDTLS_SSL_CERTIFICATE_REQUEST: 04068 ret = ssl_write_certificate_request( ssl ); 04069 break; 04070 04071 case MBEDTLS_SSL_SERVER_HELLO_DONE: 04072 ret = ssl_write_server_hello_done( ssl ); 04073 break; 04074 04075 /* 04076 * <== ( Certificate/Alert ) 04077 * ClientKeyExchange 04078 * ( CertificateVerify ) 04079 * ChangeCipherSpec 04080 * Finished 04081 */ 04082 case MBEDTLS_SSL_CLIENT_CERTIFICATE: 04083 ret = mbedtls_ssl_parse_certificate( ssl ); 04084 break; 04085 04086 case MBEDTLS_SSL_CLIENT_KEY_EXCHANGE: 04087 ret = ssl_parse_client_key_exchange( ssl ); 04088 break; 04089 04090 case MBEDTLS_SSL_CERTIFICATE_VERIFY: 04091 ret = ssl_parse_certificate_verify( ssl ); 04092 break; 04093 04094 case MBEDTLS_SSL_CLIENT_CHANGE_CIPHER_SPEC: 04095 ret = mbedtls_ssl_parse_change_cipher_spec( ssl ); 04096 break; 04097 04098 case MBEDTLS_SSL_CLIENT_FINISHED: 04099 ret = mbedtls_ssl_parse_finished( ssl ); 04100 break; 04101 04102 /* 04103 * ==> ( NewSessionTicket ) 04104 * ChangeCipherSpec 04105 * Finished 04106 */ 04107 case MBEDTLS_SSL_SERVER_CHANGE_CIPHER_SPEC: 04108 #if defined(MBEDTLS_SSL_SESSION_TICKETS) 04109 if( ssl->handshake->new_session_ticket != 0 ) 04110 ret = ssl_write_new_session_ticket( ssl ); 04111 else 04112 #endif 04113 ret = mbedtls_ssl_write_change_cipher_spec( ssl ); 04114 break; 04115 04116 case MBEDTLS_SSL_SERVER_FINISHED: 04117 ret = mbedtls_ssl_write_finished( ssl ); 04118 break; 04119 04120 case MBEDTLS_SSL_FLUSH_BUFFERS: 04121 MBEDTLS_SSL_DEBUG_MSG( 2, ( "handshake: done" ) ); 04122 ssl->state = MBEDTLS_SSL_HANDSHAKE_WRAPUP; 04123 break; 04124 04125 case MBEDTLS_SSL_HANDSHAKE_WRAPUP: 04126 mbedtls_ssl_handshake_wrapup( ssl ); 04127 break; 04128 04129 default: 04130 MBEDTLS_SSL_DEBUG_MSG( 1, ( "invalid state %d", ssl->state ) ); 04131 return( MBEDTLS_ERR_SSL_BAD_INPUT_DATA ); 04132 } 04133 04134 return( ret ); 04135 } 04136 #endif /* MBEDTLS_SSL_SRV_C */
Generated on Tue Jul 12 2022 14:49:00 by
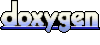