
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
snmp.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * SNMP support API for implementing netifs and statitics for MIB2 00004 */ 00005 00006 /* 00007 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * This file is part of the lwIP TCP/IP stack. 00033 * 00034 * Author: Dirk Ziegelmeier <dziegel@gmx.de> 00035 * 00036 */ 00037 #ifndef LWIP_HDR_SNMP_H 00038 #define LWIP_HDR_SNMP_H 00039 00040 #include "lwip/opt.h" 00041 #include "lwip/ip_addr.h" 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 struct udp_pcb; 00048 struct netif; 00049 00050 /** 00051 * @defgroup netif_mib2 MIB2 statistics 00052 * @ingroup netif 00053 */ 00054 00055 /* MIB2 statistics functions */ 00056 #if MIB2_STATS /* don't build if not configured for use in lwipopts.h */ 00057 /** 00058 * @ingroup netif_mib2 00059 * @see RFC1213, "MIB-II, 6. Definitions" 00060 */ 00061 enum snmp_ifType { 00062 snmp_ifType_other=1, /* none of the following */ 00063 snmp_ifType_regular1822, 00064 snmp_ifType_hdh1822, 00065 snmp_ifType_ddn_x25, 00066 snmp_ifType_rfc877_x25, 00067 snmp_ifType_ethernet_csmacd, 00068 snmp_ifType_iso88023_csmacd, 00069 snmp_ifType_iso88024_tokenBus, 00070 snmp_ifType_iso88025_tokenRing, 00071 snmp_ifType_iso88026_man, 00072 snmp_ifType_starLan, 00073 snmp_ifType_proteon_10Mbit, 00074 snmp_ifType_proteon_80Mbit, 00075 snmp_ifType_hyperchannel, 00076 snmp_ifType_fddi, 00077 snmp_ifType_lapb, 00078 snmp_ifType_sdlc, 00079 snmp_ifType_ds1, /* T-1 */ 00080 snmp_ifType_e1, /* european equiv. of T-1 */ 00081 snmp_ifType_basicISDN, 00082 snmp_ifType_primaryISDN, /* proprietary serial */ 00083 snmp_ifType_propPointToPointSerial, 00084 snmp_ifType_ppp, 00085 snmp_ifType_softwareLoopback, 00086 snmp_ifType_eon, /* CLNP over IP [11] */ 00087 snmp_ifType_ethernet_3Mbit, 00088 snmp_ifType_nsip, /* XNS over IP */ 00089 snmp_ifType_slip, /* generic SLIP */ 00090 snmp_ifType_ultra, /* ULTRA technologies */ 00091 snmp_ifType_ds3, /* T-3 */ 00092 snmp_ifType_sip, /* SMDS */ 00093 snmp_ifType_frame_relay 00094 }; 00095 00096 /** This macro has a precision of ~49 days because sys_now returns u32_t. \#define your own if you want ~490 days. */ 00097 #ifndef MIB2_COPY_SYSUPTIME_TO 00098 #define MIB2_COPY_SYSUPTIME_TO(ptrToVal) (*(ptrToVal) = (sys_now() / 10)) 00099 #endif 00100 00101 /** 00102 * @ingroup netif_mib2 00103 * Increment stats member for SNMP MIB2 stats (struct stats_mib2_netif_ctrs) 00104 */ 00105 #define MIB2_STATS_NETIF_INC(n, x) do { ++(n)->mib2_counters.x; } while(0) 00106 /** 00107 * @ingroup netif_mib2 00108 * Add value to stats member for SNMP MIB2 stats (struct stats_mib2_netif_ctrs) 00109 */ 00110 #define MIB2_STATS_NETIF_ADD(n, x, val) do { (n)->mib2_counters.x += (val); } while(0) 00111 00112 /** 00113 * @ingroup netif_mib2 00114 * Init MIB2 statistic counters in netif 00115 * @param netif Netif to init 00116 * @param type one of enum @ref snmp_ifType 00117 * @param speed your link speed here (units: bits per second) 00118 */ 00119 #define MIB2_INIT_NETIF(netif, type, speed) do { \ 00120 (netif)->link_type = (type); \ 00121 (netif)->link_speed = (speed);\ 00122 (netif)->ts = 0; \ 00123 (netif)->mib2_counters.ifinoctets = 0; \ 00124 (netif)->mib2_counters.ifinucastpkts = 0; \ 00125 (netif)->mib2_counters.ifinnucastpkts = 0; \ 00126 (netif)->mib2_counters.ifindiscards = 0; \ 00127 (netif)->mib2_counters.ifinerrors = 0; \ 00128 (netif)->mib2_counters.ifinunknownprotos = 0; \ 00129 (netif)->mib2_counters.ifoutoctets = 0; \ 00130 (netif)->mib2_counters.ifoutucastpkts = 0; \ 00131 (netif)->mib2_counters.ifoutnucastpkts = 0; \ 00132 (netif)->mib2_counters.ifoutdiscards = 0; \ 00133 (netif)->mib2_counters.ifouterrors = 0; } while(0) 00134 #else /* MIB2_STATS */ 00135 #ifndef MIB2_COPY_SYSUPTIME_TO 00136 #define MIB2_COPY_SYSUPTIME_TO(ptrToVal) 00137 #endif 00138 #define MIB2_INIT_NETIF(netif, type, speed) 00139 #define MIB2_STATS_NETIF_INC(n, x) 00140 #define MIB2_STATS_NETIF_ADD(n, x, val) 00141 #endif /* MIB2_STATS */ 00142 00143 /* LWIP MIB2 callbacks */ 00144 #if LWIP_MIB2_CALLBACKS /* don't build if not configured for use in lwipopts.h */ 00145 /* network interface */ 00146 void mib2_netif_added(struct netif *ni); 00147 void mib2_netif_removed(struct netif *ni); 00148 00149 #if LWIP_IPV4 && LWIP_ARP 00150 /* ARP (for atTable and ipNetToMediaTable) */ 00151 void mib2_add_arp_entry(struct netif *ni, ip4_addr_t *ip); 00152 void mib2_remove_arp_entry(struct netif *ni, ip4_addr_t *ip); 00153 #else /* LWIP_IPV4 && LWIP_ARP */ 00154 #define mib2_add_arp_entry(ni,ip) 00155 #define mib2_remove_arp_entry(ni,ip) 00156 #endif /* LWIP_IPV4 && LWIP_ARP */ 00157 00158 /* IP */ 00159 #if LWIP_IPV4 00160 void mib2_add_ip4(struct netif *ni); 00161 void mib2_remove_ip4(struct netif *ni); 00162 void mib2_add_route_ip4(u8_t dflt, struct netif *ni); 00163 void mib2_remove_route_ip4(u8_t dflt, struct netif *ni); 00164 #endif /* LWIP_IPV4 */ 00165 00166 /* UDP */ 00167 #if LWIP_UDP 00168 void mib2_udp_bind(struct udp_pcb *pcb); 00169 void mib2_udp_unbind(struct udp_pcb *pcb); 00170 #endif /* LWIP_UDP */ 00171 00172 #else /* LWIP_MIB2_CALLBACKS */ 00173 /* LWIP_MIB2_CALLBACKS support not available */ 00174 /* define everything to be empty */ 00175 00176 /* network interface */ 00177 #define mib2_netif_added(ni) 00178 #define mib2_netif_removed(ni) 00179 00180 /* ARP */ 00181 #define mib2_add_arp_entry(ni,ip) 00182 #define mib2_remove_arp_entry(ni,ip) 00183 00184 /* IP */ 00185 #define mib2_add_ip4(ni) 00186 #define mib2_remove_ip4(ni) 00187 #define mib2_add_route_ip4(dflt, ni) 00188 #define mib2_remove_route_ip4(dflt, ni) 00189 00190 /* UDP */ 00191 #define mib2_udp_bind(pcb) 00192 #define mib2_udp_unbind(pcb) 00193 #endif /* LWIP_MIB2_CALLBACKS */ 00194 00195 /* for source-code compatibility reasons only, can be removed (not used internally) */ 00196 #define NETIF_INIT_SNMP MIB2_INIT_NETIF 00197 #define snmp_add_ifinoctets(ni,value) MIB2_STATS_NETIF_ADD(ni, ifinoctets, value) 00198 #define snmp_inc_ifinucastpkts(ni) MIB2_STATS_NETIF_INC(ni, ifinucastpkts) 00199 #define snmp_inc_ifinnucastpkts(ni) MIB2_STATS_NETIF_INC(ni, ifinnucastpkts) 00200 #define snmp_inc_ifindiscards(ni) MIB2_STATS_NETIF_INC(ni, ifindiscards) 00201 #define snmp_inc_ifinerrors(ni) MIB2_STATS_NETIF_INC(ni, ifinerrors) 00202 #define snmp_inc_ifinunknownprotos(ni) MIB2_STATS_NETIF_INC(ni, ifinunknownprotos) 00203 #define snmp_add_ifoutoctets(ni,value) MIB2_STATS_NETIF_ADD(ni, ifoutoctets, value) 00204 #define snmp_inc_ifoutucastpkts(ni) MIB2_STATS_NETIF_INC(ni, ifoutucastpkts) 00205 #define snmp_inc_ifoutnucastpkts(ni) MIB2_STATS_NETIF_INC(ni, ifoutnucastpkts) 00206 #define snmp_inc_ifoutdiscards(ni) MIB2_STATS_NETIF_INC(ni, ifoutdiscards) 00207 #define snmp_inc_ifouterrors(ni) MIB2_STATS_NETIF_INC(ni, ifouterrors) 00208 00209 #ifdef __cplusplus 00210 } 00211 #endif 00212 00213 #endif /* LWIP_HDR_SNMP_H */
Generated on Tue Jul 12 2022 14:48:58 by
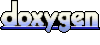