
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
pppos.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Network Point to Point Protocol over Serial header file. 00004 * 00005 */ 00006 00007 /* 00008 * Redistribution and use in source and binary forms, with or without modification, 00009 * are permitted provided that the following conditions are met: 00010 * 00011 * 1. Redistributions of source code must retain the above copyright notice, 00012 * this list of conditions and the following disclaimer. 00013 * 2. Redistributions in binary form must reproduce the above copyright notice, 00014 * this list of conditions and the following disclaimer in the documentation 00015 * and/or other materials provided with the distribution. 00016 * 3. The name of the author may not be used to endorse or promote products 00017 * derived from this software without specific prior written permission. 00018 * 00019 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00020 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00021 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00022 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00023 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00024 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00025 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00026 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00027 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00028 * OF SUCH DAMAGE. 00029 * 00030 * This file is part of the lwIP TCP/IP stack. 00031 * 00032 */ 00033 00034 #include "netif/ppp/ppp_opts.h" 00035 #if PPP_SUPPORT && PPPOS_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00036 00037 #ifndef PPPOS_H 00038 #define PPPOS_H 00039 00040 #include "lwip/sys.h" 00041 00042 #include "ppp.h" 00043 #include "vj.h" 00044 00045 /* PPP packet parser states. Current state indicates operation yet to be 00046 * completed. */ 00047 enum { 00048 PDIDLE = 0, /* Idle state - waiting. */ 00049 PDSTART, /* Process start flag. */ 00050 PDADDRESS, /* Process address field. */ 00051 PDCONTROL, /* Process control field. */ 00052 PDPROTOCOL1, /* Process protocol field 1. */ 00053 PDPROTOCOL2, /* Process protocol field 2. */ 00054 PDDATA /* Process data byte. */ 00055 }; 00056 00057 /* PPPoS serial output callback function prototype */ 00058 typedef u32_t (*pppos_output_cb_fn)(ppp_pcb *pcb, u8_t *data, u32_t len, void *ctx); 00059 00060 /* 00061 * Extended asyncmap - allows any character to be escaped. 00062 */ 00063 typedef u8_t ext_accm[32]; 00064 00065 /* 00066 * PPPoS interface control block. 00067 */ 00068 typedef struct pppos_pcb_s pppos_pcb; 00069 struct pppos_pcb_s { 00070 /* -- below are data that will NOT be cleared between two sessions */ 00071 ppp_pcb *ppp; /* PPP PCB */ 00072 pppos_output_cb_fn output_cb; /* PPP serial output callback */ 00073 00074 /* -- below are data that will be cleared between two sessions 00075 * 00076 * last_xmit must be the first member of cleared members, because it is 00077 * used to know which part must not be cleared. 00078 */ 00079 u32_t last_xmit; /* Time of last transmission. */ 00080 ext_accm out_accm; /* Async-Ctl-Char-Map for output. */ 00081 00082 /* flags */ 00083 unsigned int open :1; /* Set if PPPoS is open */ 00084 unsigned int pcomp :1; /* Does peer accept protocol compression? */ 00085 unsigned int accomp :1; /* Does peer accept addr/ctl compression? */ 00086 00087 /* PPPoS rx */ 00088 ext_accm in_accm; /* Async-Ctl-Char-Map for input. */ 00089 struct pbuf *in_head, *in_tail; /* The input packet. */ 00090 u16_t in_protocol; /* The input protocol code. */ 00091 u16_t in_fcs; /* Input Frame Check Sequence value. */ 00092 u8_t in_state; /* The input process state. */ 00093 u8_t in_escaped; /* Escape next character. */ 00094 }; 00095 00096 /* Create a new PPPoS session. */ 00097 ppp_pcb *pppos_create(struct netif *pppif, pppos_output_cb_fn output_cb, 00098 ppp_link_status_cb_fn link_status_cb, void *ctx_cb); 00099 00100 #if !NO_SYS && !PPP_INPROC_IRQ_SAFE 00101 /* Pass received raw characters to PPPoS to be decoded through lwIP TCPIP thread. */ 00102 err_t pppos_input_tcpip(ppp_pcb *ppp, u8_t *s, int l); 00103 #endif /* !NO_SYS && !PPP_INPROC_IRQ_SAFE */ 00104 00105 /* PPP over Serial: this is the input function to be called for received data. */ 00106 void pppos_input(ppp_pcb *ppp, u8_t* data, int len); 00107 00108 00109 /* 00110 * Functions called from lwIP 00111 * DO NOT CALL FROM lwIP USER APPLICATION. 00112 */ 00113 #if !NO_SYS && !PPP_INPROC_IRQ_SAFE 00114 err_t pppos_input_sys(struct pbuf *p, struct netif *inp); 00115 #endif /* !NO_SYS && !PPP_INPROC_IRQ_SAFE */ 00116 00117 #endif /* PPPOS_H */ 00118 #endif /* PPP_SUPPORT && PPPOL2TP_SUPPORT */
Generated on Tue Jul 12 2022 14:48:57 by
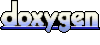