
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
ppp.h
00001 /***************************************************************************** 00002 * ppp.h - Network Point to Point Protocol header file. 00003 * 00004 * Copyright (c) 2003 by Marc Boucher, Services Informatiques (MBSI) inc. 00005 * portions Copyright (c) 1997 Global Election Systems Inc. 00006 * 00007 * The authors hereby grant permission to use, copy, modify, distribute, 00008 * and license this software and its documentation for any purpose, provided 00009 * that existing copyright notices are retained in all copies and that this 00010 * notice and the following disclaimer are included verbatim in any 00011 * distributions. No written agreement, license, or royalty fee is required 00012 * for any of the authorized uses. 00013 * 00014 * THIS SOFTWARE IS PROVIDED BY THE CONTRIBUTORS *AS IS* AND ANY EXPRESS OR 00015 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES 00016 * OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00017 * IN NO EVENT SHALL THE CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00018 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 00019 * NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00020 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00021 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00022 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF 00023 * THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00024 * 00025 ****************************************************************************** 00026 * REVISION HISTORY 00027 * 00028 * 03-01-01 Marc Boucher <marc@mbsi.ca> 00029 * Ported to lwIP. 00030 * 97-11-05 Guy Lancaster <glanca@gesn.com>, Global Election Systems Inc. 00031 * Original derived from BSD codes. 00032 *****************************************************************************/ 00033 00034 #include "netif/ppp/ppp_opts.h" 00035 #if PPP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00036 00037 #ifndef PPP_H 00038 #define PPP_H 00039 00040 #include "lwip/def.h" 00041 #include "lwip/stats.h" 00042 #include "lwip/mem.h" 00043 #include "lwip/netif.h" 00044 #include "lwip/sys.h" 00045 #include "lwip/timeouts.h" 00046 #if PPP_IPV6_SUPPORT 00047 #include "lwip/ip6_addr.h" 00048 #endif /* PPP_IPV6_SUPPORT */ 00049 00050 /* Disable non-working or rarely used PPP feature, so rarely that we don't want to bloat ppp_opts.h with them */ 00051 #ifndef PPP_OPTIONS 00052 #define PPP_OPTIONS 0 00053 #endif 00054 00055 #ifndef PPP_NOTIFY 00056 #define PPP_NOTIFY 0 00057 #endif 00058 00059 #ifndef PPP_REMOTENAME 00060 #define PPP_REMOTENAME 0 00061 #endif 00062 00063 #ifndef PPP_IDLETIMELIMIT 00064 #define PPP_IDLETIMELIMIT 0 00065 #endif 00066 00067 #ifndef PPP_LCP_ADAPTIVE 00068 #define PPP_LCP_ADAPTIVE 0 00069 #endif 00070 00071 #ifndef PPP_MAXCONNECT 00072 #define PPP_MAXCONNECT 0 00073 #endif 00074 00075 #ifndef PPP_ALLOWED_ADDRS 00076 #define PPP_ALLOWED_ADDRS 0 00077 #endif 00078 00079 #ifndef PPP_PROTOCOLNAME 00080 #define PPP_PROTOCOLNAME 0 00081 #endif 00082 00083 #ifndef PPP_STATS_SUPPORT 00084 #define PPP_STATS_SUPPORT 0 00085 #endif 00086 00087 #ifndef DEFLATE_SUPPORT 00088 #define DEFLATE_SUPPORT 0 00089 #endif 00090 00091 #ifndef BSDCOMPRESS_SUPPORT 00092 #define BSDCOMPRESS_SUPPORT 0 00093 #endif 00094 00095 #ifndef PREDICTOR_SUPPORT 00096 #define PREDICTOR_SUPPORT 0 00097 #endif 00098 00099 /************************* 00100 *** PUBLIC DEFINITIONS *** 00101 *************************/ 00102 00103 /* 00104 * The basic PPP frame. 00105 */ 00106 #define PPP_HDRLEN 4 /* octets for standard ppp header */ 00107 #define PPP_FCSLEN 2 /* octets for FCS */ 00108 00109 /* 00110 * Values for phase. 00111 */ 00112 #define PPP_PHASE_DEAD 0 00113 #define PPP_PHASE_MASTER 1 00114 #define PPP_PHASE_HOLDOFF 2 00115 #define PPP_PHASE_INITIALIZE 3 00116 #define PPP_PHASE_SERIALCONN 4 00117 #define PPP_PHASE_DORMANT 5 00118 #define PPP_PHASE_ESTABLISH 6 00119 #define PPP_PHASE_AUTHENTICATE 7 00120 #define PPP_PHASE_CALLBACK 8 00121 #define PPP_PHASE_NETWORK 9 00122 #define PPP_PHASE_RUNNING 10 00123 #define PPP_PHASE_TERMINATE 11 00124 #define PPP_PHASE_DISCONNECT 12 00125 00126 /* Error codes. */ 00127 #define PPPERR_NONE 0 /* No error. */ 00128 #define PPPERR_PARAM 1 /* Invalid parameter. */ 00129 #define PPPERR_OPEN 2 /* Unable to open PPP session. */ 00130 #define PPPERR_DEVICE 3 /* Invalid I/O device for PPP. */ 00131 #define PPPERR_ALLOC 4 /* Unable to allocate resources. */ 00132 #define PPPERR_USER 5 /* User interrupt. */ 00133 #define PPPERR_CONNECT 6 /* Connection lost. */ 00134 #define PPPERR_AUTHFAIL 7 /* Failed authentication challenge. */ 00135 #define PPPERR_PROTOCOL 8 /* Failed to meet protocol. */ 00136 #define PPPERR_PEERDEAD 9 /* Connection timeout */ 00137 #define PPPERR_IDLETIMEOUT 10 /* Idle Timeout */ 00138 #define PPPERR_CONNECTTIME 11 /* Max connect time reached */ 00139 #define PPPERR_LOOPBACK 12 /* Loopback detected */ 00140 00141 /* Whether auth support is enabled at all */ 00142 #define PPP_AUTH_SUPPORT (PAP_SUPPORT || CHAP_SUPPORT || EAP_SUPPORT) 00143 00144 /************************ 00145 *** PUBLIC DATA TYPES *** 00146 ************************/ 00147 00148 /* 00149 * Other headers require ppp_pcb definition for prototypes, but ppp_pcb 00150 * require some structure definition from other headers as well, we are 00151 * fixing the dependency loop here by declaring the ppp_pcb type then 00152 * by including headers containing necessary struct definition for ppp_pcb 00153 */ 00154 typedef struct ppp_pcb_s ppp_pcb; 00155 00156 /* Type definitions for BSD code. */ 00157 #ifndef __u_char_defined 00158 typedef unsigned long u_long; 00159 typedef unsigned int u_int; 00160 typedef unsigned short u_short; 00161 typedef unsigned char u_char; 00162 #endif 00163 00164 #include "fsm.h" 00165 #include "lcp.h" 00166 #if CCP_SUPPORT 00167 #include "ccp.h" 00168 #endif /* CCP_SUPPORT */ 00169 #if MPPE_SUPPORT 00170 #include "mppe.h" 00171 #endif /* MPPE_SUPPORT */ 00172 #if PPP_IPV4_SUPPORT 00173 #include "ipcp.h" 00174 #endif /* PPP_IPV4_SUPPORT */ 00175 #if PPP_IPV6_SUPPORT 00176 #include "ipv6cp.h" 00177 #endif /* PPP_IPV6_SUPPORT */ 00178 #if PAP_SUPPORT 00179 #include "upap.h" 00180 #endif /* PAP_SUPPORT */ 00181 #if CHAP_SUPPORT 00182 #include "chap-new.h" 00183 #endif /* CHAP_SUPPORT */ 00184 #if EAP_SUPPORT 00185 #include "eap.h" 00186 #endif /* EAP_SUPPORT */ 00187 #if VJ_SUPPORT 00188 #include "vj.h" 00189 #endif /* VJ_SUPPORT */ 00190 00191 /* Link status callback function prototype */ 00192 typedef void (*ppp_link_status_cb_fn)(ppp_pcb *pcb, int err_code, void *ctx); 00193 00194 /* 00195 * PPP configuration. 00196 */ 00197 typedef struct ppp_settings_s { 00198 00199 #if PPP_SERVER && PPP_AUTH_SUPPORT 00200 unsigned int auth_required :1; /* Peer is required to authenticate */ 00201 unsigned int null_login :1; /* Username of "" and a password of "" are acceptable */ 00202 #endif /* PPP_SERVER && PPP_AUTH_SUPPORT */ 00203 #if PPP_REMOTENAME 00204 unsigned int explicit_remote :1; /* remote_name specified with remotename opt */ 00205 #endif /* PPP_REMOTENAME */ 00206 #if PAP_SUPPORT 00207 unsigned int refuse_pap :1; /* Don't proceed auth. with PAP */ 00208 #endif /* PAP_SUPPORT */ 00209 #if CHAP_SUPPORT 00210 unsigned int refuse_chap :1; /* Don't proceed auth. with CHAP */ 00211 #endif /* CHAP_SUPPORT */ 00212 #if MSCHAP_SUPPORT 00213 unsigned int refuse_mschap :1; /* Don't proceed auth. with MS-CHAP */ 00214 unsigned int refuse_mschap_v2 :1; /* Don't proceed auth. with MS-CHAPv2 */ 00215 #endif /* MSCHAP_SUPPORT */ 00216 #if EAP_SUPPORT 00217 unsigned int refuse_eap :1; /* Don't proceed auth. with EAP */ 00218 #endif /* EAP_SUPPORT */ 00219 #if LWIP_DNS 00220 unsigned int usepeerdns :1; /* Ask peer for DNS adds */ 00221 #endif /* LWIP_DNS */ 00222 unsigned int persist :1; /* Persist mode, always try to open the connection */ 00223 #if PRINTPKT_SUPPORT 00224 unsigned int hide_password :1; /* Hide password in dumped packets */ 00225 #endif /* PRINTPKT_SUPPORT */ 00226 unsigned int noremoteip :1; /* Let him have no IP address */ 00227 unsigned int lax_recv :1; /* accept control chars in asyncmap */ 00228 unsigned int noendpoint :1; /* don't send/accept endpoint discriminator */ 00229 #if PPP_LCP_ADAPTIVE 00230 unsigned int lcp_echo_adaptive :1; /* request echo only if the link was idle */ 00231 #endif /* PPP_LCP_ADAPTIVE */ 00232 #if MPPE_SUPPORT 00233 unsigned int require_mppe :1; /* Require MPPE (Microsoft Point to Point Encryption) */ 00234 unsigned int refuse_mppe_40 :1; /* Allow MPPE 40-bit mode? */ 00235 unsigned int refuse_mppe_128 :1; /* Allow MPPE 128-bit mode? */ 00236 unsigned int refuse_mppe_stateful :1; /* Allow MPPE stateful mode? */ 00237 #endif /* MPPE_SUPPORT */ 00238 00239 u16_t listen_time; /* time to listen first (ms), waiting for peer to send LCP packet */ 00240 00241 #if PPP_IDLETIMELIMIT 00242 u16_t idle_time_limit; /* Disconnect if idle for this many seconds */ 00243 #endif /* PPP_IDLETIMELIMIT */ 00244 #if PPP_MAXCONNECT 00245 u32_t maxconnect; /* Maximum connect time (seconds) */ 00246 #endif /* PPP_MAXCONNECT */ 00247 00248 #if PPP_AUTH_SUPPORT 00249 /* auth data */ 00250 const char *user; /* Username for PAP */ 00251 const char *passwd; /* Password for PAP, secret for CHAP */ 00252 #if PPP_REMOTENAME 00253 char remote_name[MAXNAMELEN + 1]; /* Peer's name for authentication */ 00254 #endif /* PPP_REMOTENAME */ 00255 00256 #if PAP_SUPPORT 00257 u8_t pap_timeout_time; /* Timeout (seconds) for auth-req retrans. */ 00258 u8_t pap_max_transmits; /* Number of auth-reqs sent */ 00259 #if PPP_SERVER 00260 u8_t pap_req_timeout; /* Time to wait for auth-req from peer */ 00261 #endif /* PPP_SERVER */ 00262 #endif /* PAP_SUPPPORT */ 00263 00264 #if CHAP_SUPPORT 00265 u8_t chap_timeout_time; /* Timeout (seconds) for retransmitting req */ 00266 u8_t chap_max_transmits; /* max # times to send challenge */ 00267 #if PPP_SERVER 00268 u8_t chap_rechallenge_time; /* Time to wait for auth-req from peer */ 00269 #endif /* PPP_SERVER */ 00270 #endif /* CHAP_SUPPPORT */ 00271 00272 #if EAP_SUPPORT 00273 u8_t eap_req_time; /* Time to wait (for retransmit/fail) */ 00274 u8_t eap_allow_req; /* Max Requests allowed */ 00275 #if PPP_SERVER 00276 u8_t eap_timeout_time; /* Time to wait (for retransmit/fail) */ 00277 u8_t eap_max_transmits; /* Max Requests allowed */ 00278 #endif /* PPP_SERVER */ 00279 #endif /* EAP_SUPPORT */ 00280 00281 #endif /* PPP_AUTH_SUPPORT */ 00282 00283 u8_t fsm_timeout_time; /* Timeout time in seconds */ 00284 u8_t fsm_max_conf_req_transmits; /* Maximum Configure-Request transmissions */ 00285 u8_t fsm_max_term_transmits; /* Maximum Terminate-Request transmissions */ 00286 u8_t fsm_max_nak_loops; /* Maximum number of nak loops tolerated */ 00287 00288 u8_t lcp_loopbackfail; /* Number of times we receive our magic number from the peer 00289 before deciding the link is looped-back. */ 00290 u8_t lcp_echo_interval; /* Interval between LCP echo-requests */ 00291 u8_t lcp_echo_fails; /* Tolerance to unanswered echo-requests */ 00292 00293 } ppp_settings; 00294 00295 #if PPP_SERVER 00296 struct ppp_addrs { 00297 #if PPP_IPV4_SUPPORT 00298 ip4_addr_t our_ipaddr, his_ipaddr, netmask; 00299 #if LWIP_DNS 00300 ip4_addr_t dns1, dns2; 00301 #endif /* LWIP_DNS */ 00302 #endif /* PPP_IPV4_SUPPORT */ 00303 #if PPP_IPV6_SUPPORT 00304 ip6_addr_t our6_ipaddr, his6_ipaddr; 00305 #endif /* PPP_IPV6_SUPPORT */ 00306 }; 00307 #endif /* PPP_SERVER */ 00308 00309 /* 00310 * PPP interface control block. 00311 */ 00312 struct ppp_pcb_s { 00313 ppp_settings settings; 00314 const struct link_callbacks *link_cb; 00315 void *link_ctx_cb; 00316 void (*link_status_cb)(ppp_pcb *pcb, int err_code, void *ctx); /* Status change callback */ 00317 #if PPP_NOTIFY_PHASE 00318 void (*notify_phase_cb)(ppp_pcb *pcb, u8_t phase, void *ctx); /* Notify phase callback */ 00319 #endif /* PPP_NOTIFY_PHASE */ 00320 void *ctx_cb; /* Callbacks optional pointer */ 00321 struct netif *netif; /* PPP interface */ 00322 u8_t phase; /* where the link is at */ 00323 u8_t err_code; /* Code indicating why interface is down. */ 00324 00325 /* flags */ 00326 #if PPP_IPV4_SUPPORT 00327 unsigned int ask_for_local :1; /* request our address from peer */ 00328 unsigned int ipcp_is_open :1; /* haven't called np_finished() */ 00329 unsigned int ipcp_is_up :1; /* have called ipcp_up() */ 00330 unsigned int if4_up :1; /* True when the IPv4 interface is up. */ 00331 #if 0 /* UNUSED - PROXY ARP */ 00332 unsigned int proxy_arp_set :1; /* Have created proxy arp entry */ 00333 #endif /* UNUSED - PROXY ARP */ 00334 #endif /* PPP_IPV4_SUPPORT */ 00335 #if PPP_IPV6_SUPPORT 00336 unsigned int ipv6cp_is_up :1; /* have called ip6cp_up() */ 00337 unsigned int if6_up :1; /* True when the IPv6 interface is up. */ 00338 #endif /* PPP_IPV6_SUPPORT */ 00339 #if PPP_IPV4_SUPPORT && PPP_IPV6_SUPPORT 00340 unsigned int ipcp_disabled :1; /* disable ipcp */ 00341 unsigned int ipv6cp_disabled :1; /* disable ipv6cp */ 00342 #endif 00343 unsigned int lcp_echo_timer_running :1; /* set if a timer is running */ 00344 #if VJ_SUPPORT 00345 unsigned int vj_enabled :1; /* Flag indicating VJ compression enabled. */ 00346 #endif /* VJ_SUPPORT */ 00347 #if CCP_SUPPORT 00348 unsigned int ccp_all_rejected :1; /* we rejected all peer's options */ 00349 #endif /* CCP_SUPPORT */ 00350 #if MPPE_SUPPORT 00351 unsigned int mppe_keys_set :1; /* Have the MPPE keys been set? */ 00352 #endif /* MPPE_SUPPORT */ 00353 00354 #if PPP_AUTH_SUPPORT 00355 /* auth data */ 00356 #if PPP_SERVER && defined(HAVE_MULTILINK) 00357 char peer_authname[MAXNAMELEN + 1]; /* The name by which the peer authenticated itself to us. */ 00358 #endif /* PPP_SERVER && defined(HAVE_MULTILINK) */ 00359 u16_t auth_pending; /* Records which authentication operations haven't completed yet. */ 00360 u16_t auth_done; /* Records which authentication operations have been completed. */ 00361 00362 #if PAP_SUPPORT 00363 upap_state upap; /* PAP data */ 00364 #endif /* PAP_SUPPORT */ 00365 00366 #if CHAP_SUPPORT 00367 chap_client_state chap_client; /* CHAP client data */ 00368 #if PPP_SERVER 00369 chap_server_state chap_server; /* CHAP server data */ 00370 #endif /* PPP_SERVER */ 00371 #endif /* CHAP_SUPPORT */ 00372 00373 #if EAP_SUPPORT 00374 eap_state eap; /* EAP data */ 00375 #endif /* EAP_SUPPORT */ 00376 #endif /* PPP_AUTH_SUPPORT */ 00377 00378 fsm lcp_fsm; /* LCP fsm structure */ 00379 lcp_options lcp_wantoptions; /* Options that we want to request */ 00380 lcp_options lcp_gotoptions; /* Options that peer ack'd */ 00381 lcp_options lcp_allowoptions; /* Options we allow peer to request */ 00382 lcp_options lcp_hisoptions; /* Options that we ack'd */ 00383 u16_t peer_mru; /* currently negotiated peer MRU */ 00384 u8_t lcp_echos_pending; /* Number of outstanding echo msgs */ 00385 u8_t lcp_echo_number; /* ID number of next echo frame */ 00386 00387 u8_t num_np_open; /* Number of network protocols which we have opened. */ 00388 u8_t num_np_up; /* Number of network protocols which have come up. */ 00389 00390 #if VJ_SUPPORT 00391 struct vjcompress vj_comp; /* Van Jacobson compression header. */ 00392 #endif /* VJ_SUPPORT */ 00393 00394 #if CCP_SUPPORT 00395 fsm ccp_fsm; /* CCP fsm structure */ 00396 ccp_options ccp_wantoptions; /* what to request the peer to use */ 00397 ccp_options ccp_gotoptions; /* what the peer agreed to do */ 00398 ccp_options ccp_allowoptions; /* what we'll agree to do */ 00399 ccp_options ccp_hisoptions; /* what we agreed to do */ 00400 u8_t ccp_localstate; /* Local state (mainly for handling reset-reqs and reset-acks). */ 00401 u8_t ccp_receive_method; /* Method chosen on receive path */ 00402 u8_t ccp_transmit_method; /* Method chosen on transmit path */ 00403 #if MPPE_SUPPORT 00404 ppp_mppe_state mppe_comp; /* MPPE "compressor" structure */ 00405 ppp_mppe_state mppe_decomp; /* MPPE "decompressor" structure */ 00406 #endif /* MPPE_SUPPORT */ 00407 #endif /* CCP_SUPPORT */ 00408 00409 #if PPP_IPV4_SUPPORT 00410 fsm ipcp_fsm; /* IPCP fsm structure */ 00411 ipcp_options ipcp_wantoptions; /* Options that we want to request */ 00412 ipcp_options ipcp_gotoptions; /* Options that peer ack'd */ 00413 ipcp_options ipcp_allowoptions; /* Options we allow peer to request */ 00414 ipcp_options ipcp_hisoptions; /* Options that we ack'd */ 00415 #endif /* PPP_IPV4_SUPPORT */ 00416 00417 #if PPP_IPV6_SUPPORT 00418 fsm ipv6cp_fsm; /* IPV6CP fsm structure */ 00419 ipv6cp_options ipv6cp_wantoptions; /* Options that we want to request */ 00420 ipv6cp_options ipv6cp_gotoptions; /* Options that peer ack'd */ 00421 ipv6cp_options ipv6cp_allowoptions; /* Options we allow peer to request */ 00422 ipv6cp_options ipv6cp_hisoptions; /* Options that we ack'd */ 00423 #endif /* PPP_IPV6_SUPPORT */ 00424 }; 00425 00426 /************************ 00427 *** PUBLIC FUNCTIONS *** 00428 ************************/ 00429 00430 /* 00431 * WARNING: For multi-threads environment, all ppp_set_* functions most 00432 * only be called while the PPP is in the dead phase (i.e. disconnected). 00433 */ 00434 00435 #if PPP_AUTH_SUPPORT 00436 /* 00437 * Set PPP authentication. 00438 * 00439 * Warning: Using PPPAUTHTYPE_ANY might have security consequences. 00440 * RFC 1994 says: 00441 * 00442 * In practice, within or associated with each PPP server, there is a 00443 * database which associates "user" names with authentication 00444 * information ("secrets"). It is not anticipated that a particular 00445 * named user would be authenticated by multiple methods. This would 00446 * make the user vulnerable to attacks which negotiate the least secure 00447 * method from among a set (such as PAP rather than CHAP). If the same 00448 * secret was used, PAP would reveal the secret to be used later with 00449 * CHAP. 00450 * 00451 * Instead, for each user name there should be an indication of exactly 00452 * one method used to authenticate that user name. If a user needs to 00453 * make use of different authentication methods under different 00454 * circumstances, then distinct user names SHOULD be employed, each of 00455 * which identifies exactly one authentication method. 00456 * 00457 * Default is none auth type, unset (NULL) user and passwd. 00458 */ 00459 #define PPPAUTHTYPE_NONE 0x00 00460 #define PPPAUTHTYPE_PAP 0x01 00461 #define PPPAUTHTYPE_CHAP 0x02 00462 #define PPPAUTHTYPE_MSCHAP 0x04 00463 #define PPPAUTHTYPE_MSCHAP_V2 0x08 00464 #define PPPAUTHTYPE_EAP 0x10 00465 #define PPPAUTHTYPE_ANY 0xff 00466 void ppp_set_auth(ppp_pcb *pcb, u8_t authtype, const char *user, const char *passwd); 00467 00468 /* 00469 * If set, peer is required to authenticate. This is mostly necessary for PPP server support. 00470 * 00471 * Default is false. 00472 */ 00473 #define ppp_set_auth_required(ppp, boolval) (ppp->settings.auth_required = boolval) 00474 #endif /* PPP_AUTH_SUPPORT */ 00475 00476 #if PPP_IPV4_SUPPORT 00477 /* 00478 * Set PPP interface "our" and "his" IPv4 addresses. This is mostly necessary for PPP server 00479 * support but it can also be used on a PPP link where each side choose its own IP address. 00480 * 00481 * Default is unset (0.0.0.0). 00482 */ 00483 #define ppp_set_ipcp_ouraddr(ppp, addr) do { ppp->ipcp_wantoptions.ouraddr = ip4_addr_get_u32(addr); \ 00484 ppp->ask_for_local = ppp->ipcp_wantoptions.ouraddr != 0; } while(0) 00485 #define ppp_set_ipcp_hisaddr(ppp, addr) (ppp->ipcp_wantoptions.hisaddr = ip4_addr_get_u32(addr)) 00486 #if LWIP_DNS 00487 /* 00488 * Set DNS server addresses that are sent if the peer asks for them. This is mostly necessary 00489 * for PPP server support. 00490 * 00491 * Default is unset (0.0.0.0). 00492 */ 00493 #define ppp_set_ipcp_dnsaddr(ppp, index, addr) (ppp->ipcp_allowoptions.dnsaddr[index] = ip4_addr_get_u32(addr)) 00494 00495 /* 00496 * If set, we ask the peer for up to 2 DNS server addresses. Received DNS server addresses are 00497 * registered using the dns_setserver() function. 00498 * 00499 * Default is false. 00500 */ 00501 #define ppp_set_usepeerdns(ppp, boolval) (ppp->settings.usepeerdns = boolval) 00502 #endif /* LWIP_DNS */ 00503 #endif /* PPP_IPV4_SUPPORT */ 00504 00505 #if MPPE_SUPPORT 00506 /* Disable MPPE (Microsoft Point to Point Encryption). This parameter is exclusive. */ 00507 #define PPP_MPPE_DISABLE 0x00 00508 /* Require the use of MPPE (Microsoft Point to Point Encryption). */ 00509 #define PPP_MPPE_ENABLE 0x01 00510 /* Allow MPPE to use stateful mode. Stateless mode is still attempted first. */ 00511 #define PPP_MPPE_ALLOW_STATEFUL 0x02 00512 /* Refuse the use of MPPE with 40-bit encryption. Conflict with PPP_MPPE_REFUSE_128. */ 00513 #define PPP_MPPE_REFUSE_40 0x04 00514 /* Refuse the use of MPPE with 128-bit encryption. Conflict with PPP_MPPE_REFUSE_40. */ 00515 #define PPP_MPPE_REFUSE_128 0x08 00516 /* 00517 * Set MPPE configuration 00518 * 00519 * Default is disabled. 00520 */ 00521 void ppp_set_mppe(ppp_pcb *pcb, u8_t flags); 00522 #endif /* MPPE_SUPPORT */ 00523 00524 /* 00525 * Wait for up to intval milliseconds for a valid PPP packet from the peer. 00526 * At the end of this time, or when a valid PPP packet is received from the 00527 * peer, we commence negotiation by sending our first LCP packet. 00528 * 00529 * Default is 0. 00530 */ 00531 #define ppp_set_listen_time(ppp, intval) (ppp->settings.listen_time = intval) 00532 00533 /* 00534 * If set, we will attempt to initiate a connection but if no reply is received from 00535 * the peer, we will then just wait passively for a valid LCP packet from the peer. 00536 * 00537 * Default is false. 00538 */ 00539 #define ppp_set_passive(ppp, boolval) (ppp->lcp_wantoptions.passive = boolval) 00540 00541 /* 00542 * If set, we will not transmit LCP packets to initiate a connection until a valid 00543 * LCP packet is received from the peer. This is what we usually call the server mode. 00544 * 00545 * Default is false. 00546 */ 00547 #define ppp_set_silent(ppp, boolval) (ppp->lcp_wantoptions.silent = boolval) 00548 00549 /* 00550 * If set, enable protocol field compression negotiation in both the receive and 00551 * the transmit direction. 00552 * 00553 * Default is true. 00554 */ 00555 #define ppp_set_neg_pcomp(ppp, boolval) (ppp->lcp_wantoptions.neg_pcompression = \ 00556 ppp->lcp_allowoptions.neg_pcompression = boolval) 00557 00558 /* 00559 * If set, enable Address/Control compression in both the receive and the transmit 00560 * direction. 00561 * 00562 * Default is true. 00563 */ 00564 #define ppp_set_neg_accomp(ppp, boolval) (ppp->lcp_wantoptions.neg_accompression = \ 00565 ppp->lcp_allowoptions.neg_accompression = boolval) 00566 00567 /* 00568 * If set, enable asyncmap negotiation. Otherwise forcing all control characters to 00569 * be escaped for both the transmit and the receive direction. 00570 * 00571 * Default is true. 00572 */ 00573 #define ppp_set_neg_asyncmap(ppp, boolval) (ppp->lcp_wantoptions.neg_asyncmap = \ 00574 ppp->lcp_allowoptions.neg_asyncmap = boolval) 00575 00576 /* 00577 * This option sets the Async-Control-Character-Map (ACCM) for this end of the link. 00578 * The ACCM is a set of 32 bits, one for each of the ASCII control characters with 00579 * values from 0 to 31, where a 1 bit indicates that the corresponding control 00580 * character should not be used in PPP packets sent to this system. The map is 00581 * an unsigned 32 bits integer where the least significant bit (00000001) represents 00582 * character 0 and the most significant bit (80000000) represents character 31. 00583 * We will then ask the peer to send these characters as a 2-byte escape sequence. 00584 * 00585 * Default is 0. 00586 */ 00587 #define ppp_set_asyncmap(ppp, intval) (ppp->lcp_wantoptions.asyncmap = intval) 00588 00589 /* 00590 * Set a PPP interface as the default network interface 00591 * (used to output all packets for which no specific route is found). 00592 */ 00593 #define ppp_set_default(ppp) netif_set_default(ppp->netif) 00594 00595 #if PPP_NOTIFY_PHASE 00596 /* 00597 * Set a PPP notify phase callback. 00598 * 00599 * This can be used for example to set a LED pattern depending on the 00600 * current phase of the PPP session. 00601 */ 00602 typedef void (*ppp_notify_phase_cb_fn)(ppp_pcb *pcb, u8_t phase, void *ctx); 00603 void ppp_set_notify_phase_callback(ppp_pcb *pcb, ppp_notify_phase_cb_fn notify_phase_cb); 00604 #endif /* PPP_NOTIFY_PHASE */ 00605 00606 /* 00607 * Initiate a PPP connection. 00608 * 00609 * This can only be called if PPP is in the dead phase. 00610 * 00611 * Holdoff is the time to wait (in seconds) before initiating 00612 * the connection. 00613 * 00614 * If this port connects to a modem, the modem connection must be 00615 * established before calling this. 00616 */ 00617 err_t ppp_connect(ppp_pcb *pcb, u16_t holdoff); 00618 00619 #if PPP_SERVER 00620 /* 00621 * Listen for an incoming PPP connection. 00622 * 00623 * This can only be called if PPP is in the dead phase. 00624 * 00625 * If this port connects to a modem, the modem connection must be 00626 * established before calling this. 00627 */ 00628 err_t ppp_listen(ppp_pcb *pcb); 00629 #endif /* PPP_SERVER */ 00630 00631 /* 00632 * Initiate the end of a PPP connection. 00633 * Any outstanding packets in the queues are dropped. 00634 * 00635 * Setting nocarrier to 1 close the PPP connection without initiating the 00636 * shutdown procedure. Always using nocarrier = 0 is still recommended, 00637 * this is going to take a little longer time if your link is down, but 00638 * is a safer choice for the PPP state machine. 00639 * 00640 * Return 0 on success, an error code on failure. 00641 */ 00642 err_t ppp_close(ppp_pcb *pcb, u8_t nocarrier); 00643 00644 /* 00645 * Release the control block. 00646 * 00647 * This can only be called if PPP is in the dead phase. 00648 * 00649 * You must use ppp_close() before if you wish to terminate 00650 * an established PPP session. 00651 * 00652 * Return 0 on success, an error code on failure. 00653 */ 00654 err_t ppp_free(ppp_pcb *pcb); 00655 00656 /* 00657 * PPP IOCTL commands. 00658 * 00659 * Get the up status - 0 for down, non-zero for up. The argument must 00660 * point to an int. 00661 */ 00662 #define PPPCTLG_UPSTATUS 0 00663 00664 /* 00665 * Get the PPP error code. The argument must point to an int. 00666 * Returns a PPPERR_* value. 00667 */ 00668 #define PPPCTLG_ERRCODE 1 00669 00670 /* 00671 * Get the fd associated with a PPP over serial 00672 */ 00673 #define PPPCTLG_FD 2 00674 00675 /* 00676 * Get and set parameters for the given connection. 00677 * Return 0 on success, an error code on failure. 00678 */ 00679 err_t ppp_ioctl(ppp_pcb *pcb, u8_t cmd, void *arg); 00680 00681 /* Get the PPP netif interface */ 00682 #define ppp_netif(ppp) (ppp->netif) 00683 00684 /* Set an lwIP-style status-callback for the selected PPP device */ 00685 #define ppp_set_netif_statuscallback(ppp, status_cb) \ 00686 netif_set_status_callback(ppp->netif, status_cb); 00687 00688 /* Set an lwIP-style link-callback for the selected PPP device */ 00689 #define ppp_set_netif_linkcallback(ppp, link_cb) \ 00690 netif_set_link_callback(ppp->netif, link_cb); 00691 00692 #endif /* PPP_H */ 00693 00694 #endif /* PPP_SUPPORT */
Generated on Tue Jul 12 2022 14:48:56 by
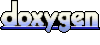