
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
nd6.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * 00004 * Neighbor discovery and stateless address autoconfiguration for IPv6. 00005 * Aims to be compliant with RFC 4861 (Neighbor discovery) and RFC 4862 00006 * (Address autoconfiguration). 00007 */ 00008 00009 /* 00010 * Copyright (c) 2010 Inico Technologies Ltd. 00011 * All rights reserved. 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. The name of the author may not be used to endorse or promote products 00022 * derived from this software without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00025 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00026 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00027 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00028 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00029 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00030 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00031 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00032 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00033 * OF SUCH DAMAGE. 00034 * 00035 * This file is part of the lwIP TCP/IP stack. 00036 * 00037 * Author: Ivan Delamer <delamer@inicotech.com> 00038 * 00039 * 00040 * Please coordinate changes and requests with Ivan Delamer 00041 * <delamer@inicotech.com> 00042 */ 00043 00044 #ifndef LWIP_HDR_ND6_H 00045 #define LWIP_HDR_ND6_H 00046 00047 #include "lwip/opt.h" 00048 00049 #if LWIP_IPV6 /* don't build if not configured for use in lwipopts.h */ 00050 00051 #include "lwip/ip6_addr.h" 00052 #include "lwip/err.h" 00053 00054 #ifdef __cplusplus 00055 extern "C" { 00056 #endif 00057 00058 /** 1 second period */ 00059 #define ND6_TMR_INTERVAL 1000 00060 00061 struct pbuf; 00062 struct netif; 00063 00064 void nd6_tmr(void); 00065 void nd6_input(struct pbuf *p, struct netif *inp); 00066 void nd6_clear_destination_cache(void); 00067 struct netif *nd6_find_route(const ip6_addr_t *ip6addr); 00068 err_t nd6_get_next_hop_addr_or_queue(struct netif *netif, struct pbuf *q, const ip6_addr_t *ip6addr, const u8_t **hwaddrp); 00069 u16_t nd6_get_destination_mtu(const ip6_addr_t *ip6addr, struct netif *netif); 00070 #if LWIP_ND6_TCP_REACHABILITY_HINTS 00071 void nd6_reachability_hint(const ip6_addr_t *ip6addr); 00072 #endif /* LWIP_ND6_TCP_REACHABILITY_HINTS */ 00073 void nd6_cleanup_netif(struct netif *netif); 00074 #if LWIP_IPV6_MLD 00075 void nd6_adjust_mld_membership(struct netif *netif, s8_t addr_idx, u8_t new_state); 00076 #endif /* LWIP_IPV6_MLD */ 00077 00078 #ifdef __cplusplus 00079 } 00080 #endif 00081 00082 #endif /* LWIP_IPV6 */ 00083 00084 #endif /* LWIP_HDR_ND6_H */
Generated on Tue Jul 12 2022 14:48:54 by
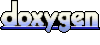