
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
memp.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * Memory pool API 00004 */ 00005 00006 /* 00007 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * This file is part of the lwIP TCP/IP stack. 00033 * 00034 * Author: Adam Dunkels <adam@sics.se> 00035 * 00036 */ 00037 00038 #ifndef LWIP_HDR_MEMP_H 00039 #define LWIP_HDR_MEMP_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* run once with empty definition to handle all custom includes in lwippools.h */ 00046 #define LWIP_MEMPOOL(name,num,size,desc) 00047 #include "lwip/priv/memp_std.h" 00048 00049 /** Create the list of all memory pools managed by memp. MEMP_MAX represents a NULL pool at the end */ 00050 typedef enum { 00051 #define LWIP_MEMPOOL(name,num,size,desc) MEMP_##name, 00052 #include "lwip/priv/memp_std.h" 00053 MEMP_MAX 00054 } memp_t; 00055 00056 #include "lwip/priv/memp_priv.h" 00057 #include "lwip/stats.h" 00058 00059 extern const struct memp_desc* const memp_pools[MEMP_MAX]; 00060 00061 /** 00062 * @ingroup mempool 00063 * Declare prototype for private memory pool if it is used in multiple files 00064 */ 00065 #define LWIP_MEMPOOL_PROTOTYPE(name) extern const struct memp_desc memp_ ## name 00066 00067 #if MEMP_MEM_MALLOC 00068 00069 #define LWIP_MEMPOOL_DECLARE(name,num,size,desc) \ 00070 LWIP_MEMPOOL_DECLARE_STATS_INSTANCE(memp_stats_ ## name) \ 00071 const struct memp_desc memp_ ## name = { \ 00072 DECLARE_LWIP_MEMPOOL_DESC(desc) \ 00073 LWIP_MEMPOOL_DECLARE_STATS_REFERENCE(memp_stats_ ## name) \ 00074 LWIP_MEM_ALIGN_SIZE(size) \ 00075 }; 00076 00077 #else /* MEMP_MEM_MALLOC */ 00078 00079 /** 00080 * @ingroup mempool 00081 * Declare a private memory pool 00082 * Private mempools example: 00083 * .h: only when pool is used in multiple .c files: LWIP_MEMPOOL_PROTOTYPE(my_private_pool); 00084 * .c: 00085 * - in global variables section: LWIP_MEMPOOL_DECLARE(my_private_pool, 10, sizeof(foo), "Some description") 00086 * - call ONCE before using pool (e.g. in some init() function): LWIP_MEMPOOL_INIT(my_private_pool); 00087 * - allocate: void* my_new_mem = LWIP_MEMPOOL_ALLOC(my_private_pool); 00088 * - free: LWIP_MEMPOOL_FREE(my_private_pool, my_new_mem); 00089 * 00090 * To relocate a pool, declare it as extern in cc.h. Example for GCC: 00091 * extern u8_t __attribute__((section(".onchip_mem"))) memp_memory_my_private_pool[]; 00092 */ 00093 #define LWIP_MEMPOOL_DECLARE(name,num,size,desc) \ 00094 LWIP_DECLARE_MEMORY_ALIGNED(memp_memory_ ## name ## _base, ((num) * (MEMP_SIZE + MEMP_ALIGN_SIZE(size)))); \ 00095 \ 00096 LWIP_MEMPOOL_DECLARE_STATS_INSTANCE(memp_stats_ ## name) \ 00097 \ 00098 static struct memp *memp_tab_ ## name; \ 00099 \ 00100 const struct memp_desc memp_ ## name = { \ 00101 DECLARE_LWIP_MEMPOOL_DESC(desc) \ 00102 LWIP_MEMPOOL_DECLARE_STATS_REFERENCE(memp_stats_ ## name) \ 00103 LWIP_MEM_ALIGN_SIZE(size), \ 00104 (num), \ 00105 memp_memory_ ## name ## _base, \ 00106 &memp_tab_ ## name \ 00107 }; 00108 00109 #endif /* MEMP_MEM_MALLOC */ 00110 00111 /** 00112 * @ingroup mempool 00113 * Initialize a private memory pool 00114 */ 00115 #define LWIP_MEMPOOL_INIT(name) memp_init_pool(&memp_ ## name) 00116 /** 00117 * @ingroup mempool 00118 * Allocate from a private memory pool 00119 */ 00120 #define LWIP_MEMPOOL_ALLOC(name) memp_malloc_pool(&memp_ ## name) 00121 /** 00122 * @ingroup mempool 00123 * Free element from a private memory pool 00124 */ 00125 #define LWIP_MEMPOOL_FREE(name, x) memp_free_pool(&memp_ ## name, (x)) 00126 00127 #if MEM_USE_POOLS 00128 /** This structure is used to save the pool one element came from. 00129 * This has to be defined here as it is required for pool size calculation. */ 00130 struct memp_malloc_helper 00131 { 00132 memp_t poolnr; 00133 #if MEMP_OVERFLOW_CHECK || (LWIP_STATS && MEM_STATS) 00134 u16_t size; 00135 #endif /* MEMP_OVERFLOW_CHECK || (LWIP_STATS && MEM_STATS) */ 00136 }; 00137 #endif /* MEM_USE_POOLS */ 00138 00139 void memp_init(void); 00140 00141 #if MEMP_OVERFLOW_CHECK 00142 void *memp_malloc_fn(memp_t type, const char* file, const int line); 00143 #define memp_malloc(t) memp_malloc_fn((t), __FILE__, __LINE__) 00144 #else 00145 void *memp_malloc(memp_t type); 00146 #endif 00147 void memp_free(memp_t type, void *mem); 00148 00149 #ifdef __cplusplus 00150 } 00151 #endif 00152 00153 #endif /* LWIP_HDR_MEMP_H */
Generated on Tue Jul 12 2022 14:48:54 by
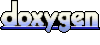