
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
md_internal.h
Go to the documentation of this file.
00001 /** 00002 * \file md_internal.h 00003 * 00004 * \brief Message digest wrappers. 00005 * 00006 * \warning This in an internal header. Do not include directly. 00007 * 00008 * \author Adriaan de Jong <dejong@fox-it.com> 00009 * 00010 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00011 * SPDX-License-Identifier: Apache-2.0 00012 * 00013 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00014 * not use this file except in compliance with the License. 00015 * You may obtain a copy of the License at 00016 * 00017 * http://www.apache.org/licenses/LICENSE-2.0 00018 * 00019 * Unless required by applicable law or agreed to in writing, software 00020 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00021 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00022 * See the License for the specific language governing permissions and 00023 * limitations under the License. 00024 * 00025 * This file is part of mbed TLS (https://tls.mbed.org) 00026 */ 00027 #ifndef MBEDTLS_MD_WRAP_H 00028 #define MBEDTLS_MD_WRAP_H 00029 00030 #if !defined(MBEDTLS_CONFIG_FILE) 00031 #include "config.h" 00032 #else 00033 #include MBEDTLS_CONFIG_FILE 00034 #endif 00035 00036 #include "md.h" 00037 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif 00041 00042 /** 00043 * Message digest information. 00044 * Allows message digest functions to be called in a generic way. 00045 */ 00046 struct mbedtls_md_info_t 00047 { 00048 /** Digest identifier */ 00049 mbedtls_md_type_t type; 00050 00051 /** Name of the message digest */ 00052 const char * name; 00053 00054 /** Output length of the digest function in bytes */ 00055 int size; 00056 00057 /** Block length of the digest function in bytes */ 00058 int block_size; 00059 00060 /** Digest initialisation function */ 00061 void (*starts_func)( void *ctx ); 00062 00063 /** Digest update function */ 00064 void (*update_func)( void *ctx, const unsigned char *input, size_t ilen ); 00065 00066 /** Digest finalisation function */ 00067 void (*finish_func)( void *ctx, unsigned char *output ); 00068 00069 /** Generic digest function */ 00070 void (*digest_func)( const unsigned char *input, size_t ilen, 00071 unsigned char *output ); 00072 00073 /** Allocate a new context */ 00074 void * (*ctx_alloc_func)( void ); 00075 00076 /** Free the given context */ 00077 void (*ctx_free_func)( void *ctx ); 00078 00079 /** Clone state from a context */ 00080 void (*clone_func)( void *dst, const void *src ); 00081 00082 /** Internal use only */ 00083 void (*process_func)( void *ctx, const unsigned char *input ); 00084 }; 00085 00086 #if defined(MBEDTLS_MD2_C) 00087 extern const mbedtls_md_info_t mbedtls_md2_info; 00088 #endif 00089 #if defined(MBEDTLS_MD4_C) 00090 extern const mbedtls_md_info_t mbedtls_md4_info; 00091 #endif 00092 #if defined(MBEDTLS_MD5_C) 00093 extern const mbedtls_md_info_t mbedtls_md5_info; 00094 #endif 00095 #if defined(MBEDTLS_RIPEMD160_C) 00096 extern const mbedtls_md_info_t mbedtls_ripemd160_info; 00097 #endif 00098 #if defined(MBEDTLS_SHA1_C) 00099 extern const mbedtls_md_info_t mbedtls_sha1_info; 00100 #endif 00101 #if defined(MBEDTLS_SHA256_C) 00102 extern const mbedtls_md_info_t mbedtls_sha224_info; 00103 extern const mbedtls_md_info_t mbedtls_sha256_info; 00104 #endif 00105 #if defined(MBEDTLS_SHA512_C) 00106 extern const mbedtls_md_info_t mbedtls_sha384_info; 00107 extern const mbedtls_md_info_t mbedtls_sha512_info; 00108 #endif 00109 00110 #ifdef __cplusplus 00111 } 00112 #endif 00113 00114 #endif /* MBEDTLS_MD_WRAP_H */
Generated on Tue Jul 12 2022 14:48:53 by
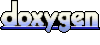