
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
ecp.h
00001 /** 00002 * \file ecp.h 00003 * 00004 * \brief Elliptic curves over GF(p) 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ECP_H 00024 #define MBEDTLS_ECP_H 00025 00026 #include "bignum.h" 00027 00028 /* 00029 * ECP error codes 00030 */ 00031 #define MBEDTLS_ERR_ECP_BAD_INPUT_DATA -0x4F80 /**< Bad input parameters to function. */ 00032 #define MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL -0x4F00 /**< The buffer is too small to write to. */ 00033 #define MBEDTLS_ERR_ECP_FEATURE_UNAVAILABLE -0x4E80 /**< Requested curve not available. */ 00034 #define MBEDTLS_ERR_ECP_VERIFY_FAILED -0x4E00 /**< The signature is not valid. */ 00035 #define MBEDTLS_ERR_ECP_ALLOC_FAILED -0x4D80 /**< Memory allocation failed. */ 00036 #define MBEDTLS_ERR_ECP_RANDOM_FAILED -0x4D00 /**< Generation of random value, such as (ephemeral) key, failed. */ 00037 #define MBEDTLS_ERR_ECP_INVALID_KEY -0x4C80 /**< Invalid private or public key. */ 00038 #define MBEDTLS_ERR_ECP_SIG_LEN_MISMATCH -0x4C00 /**< Signature is valid but shorter than the user-supplied length. */ 00039 00040 #if !defined(MBEDTLS_ECP_ALT) 00041 /* 00042 * default mbed TLS elliptic curve arithmetic implementation 00043 * 00044 * (in case MBEDTLS_ECP_ALT is defined then the developer has to provide an 00045 * alternative implementation for the whole module and it will replace this 00046 * one.) 00047 */ 00048 00049 #ifdef __cplusplus 00050 extern "C" { 00051 #endif 00052 00053 /** 00054 * Domain parameters (curve, subgroup and generator) identifiers. 00055 * 00056 * Only curves over prime fields are supported. 00057 * 00058 * \warning This library does not support validation of arbitrary domain 00059 * parameters. Therefore, only well-known domain parameters from trusted 00060 * sources should be used. See mbedtls_ecp_group_load(). 00061 */ 00062 typedef enum 00063 { 00064 MBEDTLS_ECP_DP_NONE = 0, 00065 MBEDTLS_ECP_DP_SECP192R1, /*!< 192-bits NIST curve */ 00066 MBEDTLS_ECP_DP_SECP224R1, /*!< 224-bits NIST curve */ 00067 MBEDTLS_ECP_DP_SECP256R1, /*!< 256-bits NIST curve */ 00068 MBEDTLS_ECP_DP_SECP384R1, /*!< 384-bits NIST curve */ 00069 MBEDTLS_ECP_DP_SECP521R1, /*!< 521-bits NIST curve */ 00070 MBEDTLS_ECP_DP_BP256R1, /*!< 256-bits Brainpool curve */ 00071 MBEDTLS_ECP_DP_BP384R1, /*!< 384-bits Brainpool curve */ 00072 MBEDTLS_ECP_DP_BP512R1, /*!< 512-bits Brainpool curve */ 00073 MBEDTLS_ECP_DP_CURVE25519, /*!< Curve25519 */ 00074 MBEDTLS_ECP_DP_SECP192K1, /*!< 192-bits "Koblitz" curve */ 00075 MBEDTLS_ECP_DP_SECP224K1, /*!< 224-bits "Koblitz" curve */ 00076 MBEDTLS_ECP_DP_SECP256K1, /*!< 256-bits "Koblitz" curve */ 00077 } mbedtls_ecp_group_id; 00078 00079 /** 00080 * Number of supported curves (plus one for NONE). 00081 * 00082 * (Montgomery curves excluded for now.) 00083 */ 00084 #define MBEDTLS_ECP_DP_MAX 12 00085 00086 /** 00087 * Curve information for use by other modules 00088 */ 00089 typedef struct 00090 { 00091 mbedtls_ecp_group_id grp_id ; /*!< Internal identifier */ 00092 uint16_t tls_id ; /*!< TLS NamedCurve identifier */ 00093 uint16_t bit_size ; /*!< Curve size in bits */ 00094 const char *name ; /*!< Human-friendly name */ 00095 } mbedtls_ecp_curve_info; 00096 00097 /** 00098 * \brief ECP point structure (jacobian coordinates) 00099 * 00100 * \note All functions expect and return points satisfying 00101 * the following condition: Z == 0 or Z == 1. (Other 00102 * values of Z are used by internal functions only.) 00103 * The point is zero, or "at infinity", if Z == 0. 00104 * Otherwise, X and Y are its standard (affine) coordinates. 00105 */ 00106 typedef struct 00107 { 00108 mbedtls_mpi X ; /*!< the point's X coordinate */ 00109 mbedtls_mpi Y ; /*!< the point's Y coordinate */ 00110 mbedtls_mpi Z ; /*!< the point's Z coordinate */ 00111 } 00112 mbedtls_ecp_point; 00113 00114 /** 00115 * \brief ECP group structure 00116 * 00117 * We consider two types of curves equations: 00118 * 1. Short Weierstrass y^2 = x^3 + A x + B mod P (SEC1 + RFC 4492) 00119 * 2. Montgomery, y^2 = x^3 + A x^2 + x mod P (Curve25519 + draft) 00120 * In both cases, a generator G for a prime-order subgroup is fixed. In the 00121 * short weierstrass, this subgroup is actually the whole curve, and its 00122 * cardinal is denoted by N. 00123 * 00124 * In the case of Short Weierstrass curves, our code requires that N is an odd 00125 * prime. (Use odd in mbedtls_ecp_mul() and prime in mbedtls_ecdsa_sign() for blinding.) 00126 * 00127 * In the case of Montgomery curves, we don't store A but (A + 2) / 4 which is 00128 * the quantity actually used in the formulas. Also, nbits is not the size of N 00129 * but the required size for private keys. 00130 * 00131 * If modp is NULL, reduction modulo P is done using a generic algorithm. 00132 * Otherwise, it must point to a function that takes an mbedtls_mpi in the range 00133 * 0..2^(2*pbits)-1 and transforms it in-place in an integer of little more 00134 * than pbits, so that the integer may be efficiently brought in the 0..P-1 00135 * range by a few additions or substractions. It must return 0 on success and 00136 * non-zero on failure. 00137 */ 00138 typedef struct 00139 { 00140 mbedtls_ecp_group_id id ; /*!< internal group identifier */ 00141 mbedtls_mpi P ; /*!< prime modulus of the base field */ 00142 mbedtls_mpi A ; /*!< 1. A in the equation, or 2. (A + 2) / 4 */ 00143 mbedtls_mpi B ; /*!< 1. B in the equation, or 2. unused */ 00144 mbedtls_ecp_point G ; /*!< generator of the (sub)group used */ 00145 mbedtls_mpi N ; /*!< 1. the order of G, or 2. unused */ 00146 size_t pbits ; /*!< number of bits in P */ 00147 size_t nbits ; /*!< number of bits in 1. P, or 2. private keys */ 00148 unsigned int h ; /*!< internal: 1 if the constants are static */ 00149 int (*modp)(mbedtls_mpi *); /*!< function for fast reduction mod P */ 00150 int (*t_pre)(mbedtls_ecp_point *, void *); /*!< unused */ 00151 int (*t_post)(mbedtls_ecp_point *, void *); /*!< unused */ 00152 void *t_data ; /*!< unused */ 00153 mbedtls_ecp_point *T ; /*!< pre-computed points for ecp_mul_comb() */ 00154 size_t T_size ; /*!< number for pre-computed points */ 00155 } 00156 mbedtls_ecp_group; 00157 00158 /** 00159 * \brief ECP key pair structure 00160 * 00161 * A generic key pair that could be used for ECDSA, fixed ECDH, etc. 00162 * 00163 * \note Members purposefully in the same order as struc mbedtls_ecdsa_context. 00164 */ 00165 typedef struct 00166 { 00167 mbedtls_ecp_group grp ; /*!< Elliptic curve and base point */ 00168 mbedtls_mpi d ; /*!< our secret value */ 00169 mbedtls_ecp_point Q ; /*!< our public value */ 00170 } 00171 mbedtls_ecp_keypair; 00172 00173 /** 00174 * \name SECTION: Module settings 00175 * 00176 * The configuration options you can set for this module are in this section. 00177 * Either change them in config.h or define them on the compiler command line. 00178 * \{ 00179 */ 00180 00181 #if !defined(MBEDTLS_ECP_MAX_BITS) 00182 /** 00183 * Maximum size of the groups (that is, of N and P) 00184 */ 00185 #define MBEDTLS_ECP_MAX_BITS 521 /**< Maximum bit size of groups */ 00186 #endif 00187 00188 #define MBEDTLS_ECP_MAX_BYTES ( ( MBEDTLS_ECP_MAX_BITS + 7 ) / 8 ) 00189 #define MBEDTLS_ECP_MAX_PT_LEN ( 2 * MBEDTLS_ECP_MAX_BYTES + 1 ) 00190 00191 #if !defined(MBEDTLS_ECP_WINDOW_SIZE) 00192 /* 00193 * Maximum "window" size used for point multiplication. 00194 * Default: 6. 00195 * Minimum value: 2. Maximum value: 7. 00196 * 00197 * Result is an array of at most ( 1 << ( MBEDTLS_ECP_WINDOW_SIZE - 1 ) ) 00198 * points used for point multiplication. This value is directly tied to EC 00199 * peak memory usage, so decreasing it by one should roughly cut memory usage 00200 * by two (if large curves are in use). 00201 * 00202 * Reduction in size may reduce speed, but larger curves are impacted first. 00203 * Sample performances (in ECDHE handshakes/s, with FIXED_POINT_OPTIM = 1): 00204 * w-size: 6 5 4 3 2 00205 * 521 145 141 135 120 97 00206 * 384 214 209 198 177 146 00207 * 256 320 320 303 262 226 00208 00209 * 224 475 475 453 398 342 00210 * 192 640 640 633 587 476 00211 */ 00212 #define MBEDTLS_ECP_WINDOW_SIZE 6 /**< Maximum window size used */ 00213 #endif /* MBEDTLS_ECP_WINDOW_SIZE */ 00214 00215 #if !defined(MBEDTLS_ECP_FIXED_POINT_OPTIM) 00216 /* 00217 * Trade memory for speed on fixed-point multiplication. 00218 * 00219 * This speeds up repeated multiplication of the generator (that is, the 00220 * multiplication in ECDSA signatures, and half of the multiplications in 00221 * ECDSA verification and ECDHE) by a factor roughly 3 to 4. 00222 * 00223 * The cost is increasing EC peak memory usage by a factor roughly 2. 00224 * 00225 * Change this value to 0 to reduce peak memory usage. 00226 */ 00227 #define MBEDTLS_ECP_FIXED_POINT_OPTIM 1 /**< Enable fixed-point speed-up */ 00228 #endif /* MBEDTLS_ECP_FIXED_POINT_OPTIM */ 00229 00230 /* \} name SECTION: Module settings */ 00231 00232 /* 00233 * Point formats, from RFC 4492's enum ECPointFormat 00234 */ 00235 #define MBEDTLS_ECP_PF_UNCOMPRESSED 0 /**< Uncompressed point format */ 00236 #define MBEDTLS_ECP_PF_COMPRESSED 1 /**< Compressed point format */ 00237 00238 /* 00239 * Some other constants from RFC 4492 00240 */ 00241 #define MBEDTLS_ECP_TLS_NAMED_CURVE 3 /**< ECCurveType's named_curve */ 00242 00243 /** 00244 * \brief Get the list of supported curves in order of preferrence 00245 * (full information) 00246 * 00247 * \return A statically allocated array, the last entry is 0. 00248 */ 00249 const mbedtls_ecp_curve_info *mbedtls_ecp_curve_list( void ); 00250 00251 /** 00252 * \brief Get the list of supported curves in order of preferrence 00253 * (grp_id only) 00254 * 00255 * \return A statically allocated array, 00256 * terminated with MBEDTLS_ECP_DP_NONE. 00257 */ 00258 const mbedtls_ecp_group_id *mbedtls_ecp_grp_id_list( void ); 00259 00260 /** 00261 * \brief Get curve information from an internal group identifier 00262 * 00263 * \param grp_id A MBEDTLS_ECP_DP_XXX value 00264 * 00265 * \return The associated curve information or NULL 00266 */ 00267 const mbedtls_ecp_curve_info *mbedtls_ecp_curve_info_from_grp_id( mbedtls_ecp_group_id grp_id ); 00268 00269 /** 00270 * \brief Get curve information from a TLS NamedCurve value 00271 * 00272 * \param tls_id A MBEDTLS_ECP_DP_XXX value 00273 * 00274 * \return The associated curve information or NULL 00275 */ 00276 const mbedtls_ecp_curve_info *mbedtls_ecp_curve_info_from_tls_id( uint16_t tls_id ); 00277 00278 /** 00279 * \brief Get curve information from a human-readable name 00280 * 00281 * \param name The name 00282 * 00283 * \return The associated curve information or NULL 00284 */ 00285 const mbedtls_ecp_curve_info *mbedtls_ecp_curve_info_from_name( const char *name ); 00286 00287 /** 00288 * \brief Initialize a point (as zero) 00289 */ 00290 void mbedtls_ecp_point_init( mbedtls_ecp_point *pt ); 00291 00292 /** 00293 * \brief Initialize a group (to something meaningless) 00294 */ 00295 void mbedtls_ecp_group_init( mbedtls_ecp_group *grp ); 00296 00297 /** 00298 * \brief Initialize a key pair (as an invalid one) 00299 */ 00300 void mbedtls_ecp_keypair_init( mbedtls_ecp_keypair *key ); 00301 00302 /** 00303 * \brief Free the components of a point 00304 */ 00305 void mbedtls_ecp_point_free( mbedtls_ecp_point *pt ); 00306 00307 /** 00308 * \brief Free the components of an ECP group 00309 */ 00310 void mbedtls_ecp_group_free( mbedtls_ecp_group *grp ); 00311 00312 /** 00313 * \brief Free the components of a key pair 00314 */ 00315 void mbedtls_ecp_keypair_free( mbedtls_ecp_keypair *key ); 00316 00317 /** 00318 * \brief Copy the contents of point Q into P 00319 * 00320 * \param P Destination point 00321 * \param Q Source point 00322 * 00323 * \return 0 if successful, 00324 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00325 */ 00326 int mbedtls_ecp_copy( mbedtls_ecp_point *P, const mbedtls_ecp_point *Q ); 00327 00328 /** 00329 * \brief Copy the contents of a group object 00330 * 00331 * \param dst Destination group 00332 * \param src Source group 00333 * 00334 * \return 0 if successful, 00335 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00336 */ 00337 int mbedtls_ecp_group_copy( mbedtls_ecp_group *dst, const mbedtls_ecp_group *src ); 00338 00339 /** 00340 * \brief Set a point to zero 00341 * 00342 * \param pt Destination point 00343 * 00344 * \return 0 if successful, 00345 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00346 */ 00347 int mbedtls_ecp_set_zero( mbedtls_ecp_point *pt ); 00348 00349 /** 00350 * \brief Tell if a point is zero 00351 * 00352 * \param pt Point to test 00353 * 00354 * \return 1 if point is zero, 0 otherwise 00355 */ 00356 int mbedtls_ecp_is_zero( mbedtls_ecp_point *pt ); 00357 00358 /** 00359 * \brief Compare two points 00360 * 00361 * \note This assumes the points are normalized. Otherwise, 00362 * they may compare as "not equal" even if they are. 00363 * 00364 * \param P First point to compare 00365 * \param Q Second point to compare 00366 * 00367 * \return 0 if the points are equal, 00368 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA otherwise 00369 */ 00370 int mbedtls_ecp_point_cmp( const mbedtls_ecp_point *P, 00371 const mbedtls_ecp_point *Q ); 00372 00373 /** 00374 * \brief Import a non-zero point from two ASCII strings 00375 * 00376 * \param P Destination point 00377 * \param radix Input numeric base 00378 * \param x First affine coordinate as a null-terminated string 00379 * \param y Second affine coordinate as a null-terminated string 00380 * 00381 * \return 0 if successful, or a MBEDTLS_ERR_MPI_XXX error code 00382 */ 00383 int mbedtls_ecp_point_read_string( mbedtls_ecp_point *P, int radix, 00384 const char *x, const char *y ); 00385 00386 /** 00387 * \brief Export a point into unsigned binary data 00388 * 00389 * \param grp Group to which the point should belong 00390 * \param P Point to export 00391 * \param format Point format, should be a MBEDTLS_ECP_PF_XXX macro 00392 * \param olen Length of the actual output 00393 * \param buf Output buffer 00394 * \param buflen Length of the output buffer 00395 * 00396 * \return 0 if successful, 00397 * or MBEDTLS_ERR_ECP_BAD_INPUT_DATA 00398 * or MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL 00399 */ 00400 int mbedtls_ecp_point_write_binary( const mbedtls_ecp_group *grp, const mbedtls_ecp_point *P, 00401 int format, size_t *olen, 00402 unsigned char *buf, size_t buflen ); 00403 00404 /** 00405 * \brief Import a point from unsigned binary data 00406 * 00407 * \param grp Group to which the point should belong 00408 * \param P Point to import 00409 * \param buf Input buffer 00410 * \param ilen Actual length of input 00411 * 00412 * \return 0 if successful, 00413 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid, 00414 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00415 * MBEDTLS_ERR_ECP_FEATURE_UNAVAILABLE if the point format 00416 * is not implemented. 00417 * 00418 * \note This function does NOT check that the point actually 00419 * belongs to the given group, see mbedtls_ecp_check_pubkey() for 00420 * that. 00421 */ 00422 int mbedtls_ecp_point_read_binary( const mbedtls_ecp_group *grp, mbedtls_ecp_point *P, 00423 const unsigned char *buf, size_t ilen ); 00424 00425 /** 00426 * \brief Import a point from a TLS ECPoint record 00427 * 00428 * \param grp ECP group used 00429 * \param pt Destination point 00430 * \param buf $(Start of input buffer) 00431 * \param len Buffer length 00432 * 00433 * \note buf is updated to point right after the ECPoint on exit 00434 * 00435 * \return 0 if successful, 00436 * MBEDTLS_ERR_MPI_XXX if initialization failed 00437 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid 00438 */ 00439 int mbedtls_ecp_tls_read_point( const mbedtls_ecp_group *grp, mbedtls_ecp_point *pt, 00440 const unsigned char **buf, size_t len ); 00441 00442 /** 00443 * \brief Export a point as a TLS ECPoint record 00444 * 00445 * \param grp ECP group used 00446 * \param pt Point to export 00447 * \param format Export format 00448 * \param olen length of data written 00449 * \param buf Buffer to write to 00450 * \param blen Buffer length 00451 * 00452 * \return 0 if successful, 00453 * or MBEDTLS_ERR_ECP_BAD_INPUT_DATA 00454 * or MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL 00455 */ 00456 int mbedtls_ecp_tls_write_point( const mbedtls_ecp_group *grp, const mbedtls_ecp_point *pt, 00457 int format, size_t *olen, 00458 unsigned char *buf, size_t blen ); 00459 00460 /** 00461 * \brief Set a group using well-known domain parameters 00462 * 00463 * \param grp Destination group 00464 * \param id Index in the list of well-known domain parameters 00465 * 00466 * \return 0 if successful, 00467 * MBEDTLS_ERR_MPI_XXX if initialization failed 00468 * MBEDTLS_ERR_ECP_FEATURE_UNAVAILABLE for unkownn groups 00469 * 00470 * \note Index should be a value of RFC 4492's enum NamedCurve, 00471 * usually in the form of a MBEDTLS_ECP_DP_XXX macro. 00472 */ 00473 int mbedtls_ecp_group_load( mbedtls_ecp_group *grp, mbedtls_ecp_group_id id ); 00474 00475 /** 00476 * \brief Set a group from a TLS ECParameters record 00477 * 00478 * \param grp Destination group 00479 * \param buf &(Start of input buffer) 00480 * \param len Buffer length 00481 * 00482 * \note buf is updated to point right after ECParameters on exit 00483 * 00484 * \return 0 if successful, 00485 * MBEDTLS_ERR_MPI_XXX if initialization failed 00486 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid 00487 */ 00488 int mbedtls_ecp_tls_read_group( mbedtls_ecp_group *grp, const unsigned char **buf, size_t len ); 00489 00490 /** 00491 * \brief Write the TLS ECParameters record for a group 00492 * 00493 * \param grp ECP group used 00494 * \param olen Number of bytes actually written 00495 * \param buf Buffer to write to 00496 * \param blen Buffer length 00497 * 00498 * \return 0 if successful, 00499 * or MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL 00500 */ 00501 int mbedtls_ecp_tls_write_group( const mbedtls_ecp_group *grp, size_t *olen, 00502 unsigned char *buf, size_t blen ); 00503 00504 /** 00505 * \brief Multiplication by an integer: R = m * P 00506 * (Not thread-safe to use same group in multiple threads) 00507 * 00508 * \note In order to prevent timing attacks, this function 00509 * executes the exact same sequence of (base field) 00510 * operations for any valid m. It avoids any if-branch or 00511 * array index depending on the value of m. 00512 * 00513 * \note If f_rng is not NULL, it is used to randomize intermediate 00514 * results in order to prevent potential timing attacks 00515 * targeting these results. It is recommended to always 00516 * provide a non-NULL f_rng (the overhead is negligible). 00517 * 00518 * \param grp ECP group 00519 * \param R Destination point 00520 * \param m Integer by which to multiply 00521 * \param P Point to multiply 00522 * \param f_rng RNG function (see notes) 00523 * \param p_rng RNG parameter 00524 * 00525 * \return 0 if successful, 00526 * MBEDTLS_ERR_ECP_INVALID_KEY if m is not a valid privkey 00527 * or P is not a valid pubkey, 00528 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00529 */ 00530 int mbedtls_ecp_mul( mbedtls_ecp_group *grp, mbedtls_ecp_point *R, 00531 const mbedtls_mpi *m, const mbedtls_ecp_point *P, 00532 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00533 00534 /** 00535 * \brief Multiplication and addition of two points by integers: 00536 * R = m * P + n * Q 00537 * (Not thread-safe to use same group in multiple threads) 00538 * 00539 * \note In contrast to mbedtls_ecp_mul(), this function does not guarantee 00540 * a constant execution flow and timing. 00541 * 00542 * \param grp ECP group 00543 * \param R Destination point 00544 * \param m Integer by which to multiply P 00545 * \param P Point to multiply by m 00546 * \param n Integer by which to multiply Q 00547 * \param Q Point to be multiplied by n 00548 * 00549 * \return 0 if successful, 00550 * MBEDTLS_ERR_ECP_INVALID_KEY if m or n is not a valid privkey 00551 * or P or Q is not a valid pubkey, 00552 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00553 */ 00554 int mbedtls_ecp_muladd( mbedtls_ecp_group *grp, mbedtls_ecp_point *R, 00555 const mbedtls_mpi *m, const mbedtls_ecp_point *P, 00556 const mbedtls_mpi *n, const mbedtls_ecp_point *Q ); 00557 00558 /** 00559 * \brief Check that a point is a valid public key on this curve 00560 * 00561 * \param grp Curve/group the point should belong to 00562 * \param pt Point to check 00563 * 00564 * \return 0 if point is a valid public key, 00565 * MBEDTLS_ERR_ECP_INVALID_KEY otherwise. 00566 * 00567 * \note This function only checks the point is non-zero, has valid 00568 * coordinates and lies on the curve, but not that it is 00569 * indeed a multiple of G. This is additional check is more 00570 * expensive, isn't required by standards, and shouldn't be 00571 * necessary if the group used has a small cofactor. In 00572 * particular, it is useless for the NIST groups which all 00573 * have a cofactor of 1. 00574 * 00575 * \note Uses bare components rather than an mbedtls_ecp_keypair structure 00576 * in order to ease use with other structures such as 00577 * mbedtls_ecdh_context of mbedtls_ecdsa_context. 00578 */ 00579 int mbedtls_ecp_check_pubkey( const mbedtls_ecp_group *grp, const mbedtls_ecp_point *pt ); 00580 00581 /** 00582 * \brief Check that an mbedtls_mpi is a valid private key for this curve 00583 * 00584 * \param grp Group used 00585 * \param d Integer to check 00586 * 00587 * \return 0 if point is a valid private key, 00588 * MBEDTLS_ERR_ECP_INVALID_KEY otherwise. 00589 * 00590 * \note Uses bare components rather than an mbedtls_ecp_keypair structure 00591 * in order to ease use with other structures such as 00592 * mbedtls_ecdh_context of mbedtls_ecdsa_context. 00593 */ 00594 int mbedtls_ecp_check_privkey( const mbedtls_ecp_group *grp, const mbedtls_mpi *d ); 00595 00596 /** 00597 * \brief Generate a keypair with configurable base point 00598 * 00599 * \param grp ECP group 00600 * \param G Chosen base point 00601 * \param d Destination MPI (secret part) 00602 * \param Q Destination point (public part) 00603 * \param f_rng RNG function 00604 * \param p_rng RNG parameter 00605 * 00606 * \return 0 if successful, 00607 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00608 * 00609 * \note Uses bare components rather than an mbedtls_ecp_keypair structure 00610 * in order to ease use with other structures such as 00611 * mbedtls_ecdh_context of mbedtls_ecdsa_context. 00612 */ 00613 int mbedtls_ecp_gen_keypair_base( mbedtls_ecp_group *grp, 00614 const mbedtls_ecp_point *G, 00615 mbedtls_mpi *d, mbedtls_ecp_point *Q, 00616 int (*f_rng)(void *, unsigned char *, size_t), 00617 void *p_rng ); 00618 00619 /** 00620 * \brief Generate a keypair 00621 * 00622 * \param grp ECP group 00623 * \param d Destination MPI (secret part) 00624 * \param Q Destination point (public part) 00625 * \param f_rng RNG function 00626 * \param p_rng RNG parameter 00627 * 00628 * \return 0 if successful, 00629 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00630 * 00631 * \note Uses bare components rather than an mbedtls_ecp_keypair structure 00632 * in order to ease use with other structures such as 00633 * mbedtls_ecdh_context of mbedtls_ecdsa_context. 00634 */ 00635 int mbedtls_ecp_gen_keypair( mbedtls_ecp_group *grp, mbedtls_mpi *d, mbedtls_ecp_point *Q, 00636 int (*f_rng)(void *, unsigned char *, size_t), 00637 void *p_rng ); 00638 00639 /** 00640 * \brief Generate a keypair 00641 * 00642 * \param grp_id ECP group identifier 00643 * \param key Destination keypair 00644 * \param f_rng RNG function 00645 * \param p_rng RNG parameter 00646 * 00647 * \return 0 if successful, 00648 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00649 */ 00650 int mbedtls_ecp_gen_key( mbedtls_ecp_group_id grp_id, mbedtls_ecp_keypair *key, 00651 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00652 00653 /** 00654 * \brief Check a public-private key pair 00655 * 00656 * \param pub Keypair structure holding a public key 00657 * \param prv Keypair structure holding a private (plus public) key 00658 * 00659 * \return 0 if successful (keys are valid and match), or 00660 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA, or 00661 * a MBEDTLS_ERR_ECP_XXX or MBEDTLS_ERR_MPI_XXX code. 00662 */ 00663 int mbedtls_ecp_check_pub_priv( const mbedtls_ecp_keypair *pub, const mbedtls_ecp_keypair *prv ); 00664 00665 #if defined(MBEDTLS_SELF_TEST) 00666 00667 /** 00668 * \brief Checkup routine 00669 * 00670 * \return 0 if successful, or 1 if a test failed 00671 */ 00672 int mbedtls_ecp_self_test( int verbose ); 00673 00674 #endif /* MBEDTLS_SELF_TEST */ 00675 00676 #ifdef __cplusplus 00677 } 00678 #endif 00679 00680 #else /* MBEDTLS_ECP_ALT */ 00681 #include "ecp_alt.h" 00682 #endif /* MBEDTLS_ECP_ALT */ 00683 00684 #endif /* ecp.h */
Generated on Tue Jul 12 2022 14:48:43 by
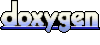