
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
des.h
00001 /** 00002 * \file des.h 00003 * 00004 * \brief DES block cipher 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_DES_H 00024 #define MBEDTLS_DES_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 #include <stdint.h> 00034 00035 #define MBEDTLS_DES_ENCRYPT 1 00036 #define MBEDTLS_DES_DECRYPT 0 00037 00038 #define MBEDTLS_ERR_DES_INVALID_INPUT_LENGTH -0x0032 /**< The data input has an invalid length. */ 00039 00040 #define MBEDTLS_DES_KEY_SIZE 8 00041 00042 #if !defined(MBEDTLS_DES_ALT) 00043 // Regular implementation 00044 // 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /** 00051 * \brief DES context structure 00052 */ 00053 typedef struct 00054 { 00055 uint32_t sk[32]; /*!< DES subkeys */ 00056 } 00057 mbedtls_des_context; 00058 00059 /** 00060 * \brief Triple-DES context structure 00061 */ 00062 typedef struct 00063 { 00064 uint32_t sk[96]; /*!< 3DES subkeys */ 00065 } 00066 mbedtls_des3_context; 00067 00068 /** 00069 * \brief Initialize DES context 00070 * 00071 * \param ctx DES context to be initialized 00072 */ 00073 void mbedtls_des_init( mbedtls_des_context *ctx ); 00074 00075 /** 00076 * \brief Clear DES context 00077 * 00078 * \param ctx DES context to be cleared 00079 */ 00080 void mbedtls_des_free( mbedtls_des_context *ctx ); 00081 00082 /** 00083 * \brief Initialize Triple-DES context 00084 * 00085 * \param ctx DES3 context to be initialized 00086 */ 00087 void mbedtls_des3_init( mbedtls_des3_context *ctx ); 00088 00089 /** 00090 * \brief Clear Triple-DES context 00091 * 00092 * \param ctx DES3 context to be cleared 00093 */ 00094 void mbedtls_des3_free( mbedtls_des3_context *ctx ); 00095 00096 /** 00097 * \brief Set key parity on the given key to odd. 00098 * 00099 * DES keys are 56 bits long, but each byte is padded with 00100 * a parity bit to allow verification. 00101 * 00102 * \param key 8-byte secret key 00103 */ 00104 void mbedtls_des_key_set_parity( unsigned char key[MBEDTLS_DES_KEY_SIZE] ); 00105 00106 /** 00107 * \brief Check that key parity on the given key is odd. 00108 * 00109 * DES keys are 56 bits long, but each byte is padded with 00110 * a parity bit to allow verification. 00111 * 00112 * \param key 8-byte secret key 00113 * 00114 * \return 0 is parity was ok, 1 if parity was not correct. 00115 */ 00116 int mbedtls_des_key_check_key_parity( const unsigned char key[MBEDTLS_DES_KEY_SIZE] ); 00117 00118 /** 00119 * \brief Check that key is not a weak or semi-weak DES key 00120 * 00121 * \param key 8-byte secret key 00122 * 00123 * \return 0 if no weak key was found, 1 if a weak key was identified. 00124 */ 00125 int mbedtls_des_key_check_weak( const unsigned char key[MBEDTLS_DES_KEY_SIZE] ); 00126 00127 /** 00128 * \brief DES key schedule (56-bit, encryption) 00129 * 00130 * \param ctx DES context to be initialized 00131 * \param key 8-byte secret key 00132 * 00133 * \return 0 00134 */ 00135 int mbedtls_des_setkey_enc( mbedtls_des_context *ctx, const unsigned char key[MBEDTLS_DES_KEY_SIZE] ); 00136 00137 /** 00138 * \brief DES key schedule (56-bit, decryption) 00139 * 00140 * \param ctx DES context to be initialized 00141 * \param key 8-byte secret key 00142 * 00143 * \return 0 00144 */ 00145 int mbedtls_des_setkey_dec( mbedtls_des_context *ctx, const unsigned char key[MBEDTLS_DES_KEY_SIZE] ); 00146 00147 /** 00148 * \brief Triple-DES key schedule (112-bit, encryption) 00149 * 00150 * \param ctx 3DES context to be initialized 00151 * \param key 16-byte secret key 00152 * 00153 * \return 0 00154 */ 00155 int mbedtls_des3_set2key_enc( mbedtls_des3_context *ctx, 00156 const unsigned char key[MBEDTLS_DES_KEY_SIZE * 2] ); 00157 00158 /** 00159 * \brief Triple-DES key schedule (112-bit, decryption) 00160 * 00161 * \param ctx 3DES context to be initialized 00162 * \param key 16-byte secret key 00163 * 00164 * \return 0 00165 */ 00166 int mbedtls_des3_set2key_dec( mbedtls_des3_context *ctx, 00167 const unsigned char key[MBEDTLS_DES_KEY_SIZE * 2] ); 00168 00169 /** 00170 * \brief Triple-DES key schedule (168-bit, encryption) 00171 * 00172 * \param ctx 3DES context to be initialized 00173 * \param key 24-byte secret key 00174 * 00175 * \return 0 00176 */ 00177 int mbedtls_des3_set3key_enc( mbedtls_des3_context *ctx, 00178 const unsigned char key[MBEDTLS_DES_KEY_SIZE * 3] ); 00179 00180 /** 00181 * \brief Triple-DES key schedule (168-bit, decryption) 00182 * 00183 * \param ctx 3DES context to be initialized 00184 * \param key 24-byte secret key 00185 * 00186 * \return 0 00187 */ 00188 int mbedtls_des3_set3key_dec( mbedtls_des3_context *ctx, 00189 const unsigned char key[MBEDTLS_DES_KEY_SIZE * 3] ); 00190 00191 /** 00192 * \brief DES-ECB block encryption/decryption 00193 * 00194 * \param ctx DES context 00195 * \param input 64-bit input block 00196 * \param output 64-bit output block 00197 * 00198 * \return 0 if successful 00199 */ 00200 int mbedtls_des_crypt_ecb( mbedtls_des_context *ctx, 00201 const unsigned char input[8], 00202 unsigned char output[8] ); 00203 00204 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00205 /** 00206 * \brief DES-CBC buffer encryption/decryption 00207 * 00208 * \note Upon exit, the content of the IV is updated so that you can 00209 * call the function same function again on the following 00210 * block(s) of data and get the same result as if it was 00211 * encrypted in one call. This allows a "streaming" usage. 00212 * If on the other hand you need to retain the contents of the 00213 * IV, you should either save it manually or use the cipher 00214 * module instead. 00215 * 00216 * \param ctx DES context 00217 * \param mode MBEDTLS_DES_ENCRYPT or MBEDTLS_DES_DECRYPT 00218 * \param length length of the input data 00219 * \param iv initialization vector (updated after use) 00220 * \param input buffer holding the input data 00221 * \param output buffer holding the output data 00222 */ 00223 int mbedtls_des_crypt_cbc( mbedtls_des_context *ctx, 00224 int mode, 00225 size_t length, 00226 unsigned char iv[8], 00227 const unsigned char *input, 00228 unsigned char *output ); 00229 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00230 00231 /** 00232 * \brief 3DES-ECB block encryption/decryption 00233 * 00234 * \param ctx 3DES context 00235 * \param input 64-bit input block 00236 * \param output 64-bit output block 00237 * 00238 * \return 0 if successful 00239 */ 00240 int mbedtls_des3_crypt_ecb( mbedtls_des3_context *ctx, 00241 const unsigned char input[8], 00242 unsigned char output[8] ); 00243 00244 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00245 /** 00246 * \brief 3DES-CBC buffer encryption/decryption 00247 * 00248 * \note Upon exit, the content of the IV is updated so that you can 00249 * call the function same function again on the following 00250 * block(s) of data and get the same result as if it was 00251 * encrypted in one call. This allows a "streaming" usage. 00252 * If on the other hand you need to retain the contents of the 00253 * IV, you should either save it manually or use the cipher 00254 * module instead. 00255 * 00256 * \param ctx 3DES context 00257 * \param mode MBEDTLS_DES_ENCRYPT or MBEDTLS_DES_DECRYPT 00258 * \param length length of the input data 00259 * \param iv initialization vector (updated after use) 00260 * \param input buffer holding the input data 00261 * \param output buffer holding the output data 00262 * 00263 * \return 0 if successful, or MBEDTLS_ERR_DES_INVALID_INPUT_LENGTH 00264 */ 00265 int mbedtls_des3_crypt_cbc( mbedtls_des3_context *ctx, 00266 int mode, 00267 size_t length, 00268 unsigned char iv[8], 00269 const unsigned char *input, 00270 unsigned char *output ); 00271 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00272 00273 /** 00274 * \brief Internal function for key expansion. 00275 * (Only exposed to allow overriding it, 00276 * see MBEDTLS_DES_SETKEY_ALT) 00277 * 00278 * \param SK Round keys 00279 * \param key Base key 00280 */ 00281 void mbedtls_des_setkey( uint32_t SK[32], 00282 const unsigned char key[MBEDTLS_DES_KEY_SIZE] ); 00283 #ifdef __cplusplus 00284 } 00285 #endif 00286 00287 #else /* MBEDTLS_DES_ALT */ 00288 #include "des_alt.h" 00289 #endif /* MBEDTLS_DES_ALT */ 00290 00291 #ifdef __cplusplus 00292 extern "C" { 00293 #endif 00294 00295 /** 00296 * \brief Checkup routine 00297 * 00298 * \return 0 if successful, or 1 if the test failed 00299 */ 00300 int mbedtls_des_self_test( int verbose ); 00301 00302 #ifdef __cplusplus 00303 } 00304 #endif 00305 00306 #endif /* des.h */
Generated on Tue Jul 12 2022 14:48:42 by
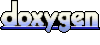