
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
mbed_stats.c
00001 #include "mbed_stats.h" 00002 #include <string.h> 00003 #include <stdlib.h> 00004 #include "mbed_assert.h" 00005 00006 #if MBED_CONF_RTOS_PRESENT 00007 #include "cmsis_os2.h" 00008 #endif 00009 00010 // note: mbed_stats_heap_get defined in mbed_alloc_wrappers.cpp 00011 00012 void mbed_stats_stack_get(mbed_stats_stack_t *stats) 00013 { 00014 memset(stats, 0, sizeof(mbed_stats_stack_t)); 00015 00016 #if MBED_STACK_STATS_ENABLED && MBED_CONF_RTOS_PRESENT 00017 uint32_t thread_n = osThreadGetCount(); 00018 unsigned i; 00019 osThreadId_t *threads; 00020 00021 threads = malloc(sizeof(osThreadId_t) * thread_n); 00022 MBED_ASSERT(threads != NULL); 00023 00024 osKernelLock(); 00025 thread_n = osThreadEnumerate(threads, thread_n); 00026 00027 for(i = 0; i < thread_n; i++) { 00028 uint32_t stack_size = osThreadGetStackSize(threads[i]); 00029 stats->max_size += stack_size - osThreadGetStackSpace(threads[i]); 00030 stats->reserved_size += stack_size; 00031 stats->stack_cnt++; 00032 } 00033 osKernelUnlock(); 00034 00035 free(threads); 00036 #endif 00037 } 00038 00039 size_t mbed_stats_stack_get_each(mbed_stats_stack_t *stats, size_t count) 00040 { 00041 memset(stats, 0, count*sizeof(mbed_stats_stack_t)); 00042 size_t i = 0; 00043 00044 #if MBED_STACK_STATS_ENABLED && MBED_CONF_RTOS_PRESENT 00045 osThreadId_t *threads; 00046 00047 threads = malloc(sizeof(osThreadId_t) * count); 00048 MBED_ASSERT(threads != NULL); 00049 00050 osKernelLock(); 00051 count = osThreadEnumerate(threads, count); 00052 00053 for(i = 0; i < count; i++) { 00054 uint32_t stack_size = osThreadGetStackSize(threads[i]); 00055 stats[i].max_size = stack_size - osThreadGetStackSpace(threads[i]); 00056 stats[i].reserved_size = stack_size; 00057 stats[i].thread_id = (uint32_t)threads[i]; 00058 stats[i].stack_cnt = 1; 00059 } 00060 osKernelUnlock(); 00061 00062 free(threads); 00063 #endif 00064 00065 return i; 00066 } 00067 00068 #if MBED_STACK_STATS_ENABLED && !MBED_CONF_RTOS_PRESENT 00069 #warning Stack statistics are currently not supported without the rtos. 00070 #endif
Generated on Tue Jul 12 2022 14:48:53 by
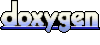