
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
mac_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file mac_api.h 00017 * \brief A API class to support different MACs from multiple vendors. 00018 * Vendor must implement a function which fills supported callback functions which Upper layer will use. 00019 */ 00020 00021 #ifndef MAC_API_H 00022 #define MAC_API_H 00023 00024 #include <inttypes.h> 00025 #include "mlme.h" 00026 #include "mac_mcps.h" 00027 00028 #ifdef __cplusplus 00029 extern "C" { 00030 #endif 00031 00032 typedef struct mac_api_s mac_api_t; 00033 00034 /** 00035 * Every MAC adapting to Upper layer must implement a function which creates mac_api_t pointer, e.g 'mac_api_t* create_mac_api();' 00036 * In the function external Mac needs to fill necessary function pointers so that Upper layer can use it. 00037 * For Nanostack to work, following (mlme/mcps) request functions are mandatory: 00038 * - mcps-data 00039 * - scan 00040 * - start 00041 * - poll 00042 * - get 00043 * - set 00044 * - reset 00045 * - (purge) 00046 * Also indication and confirm callbacks for above are needed plus 00047 * - beacon notify 00048 * - comm status 00049 * 00050 * \return mac_api_t Ownership of newly created object 00051 */ 00052 00053 /*! 00054 * \enum mlme_primitive 00055 * \brief Enum for MLME primitive types. 00056 */ 00057 typedef enum { 00058 MLME_ASSOCIATE, 00059 MLME_DISASSOCIATE, 00060 MLME_BEACON_NOTIFY, 00061 MLME_GET, 00062 MLME_GTS, 00063 MLME_ORPHAN, 00064 MLME_RESET, 00065 MLME_RX_ENABLE, 00066 MLME_SCAN, 00067 MLME_COMM_STATUS, 00068 MLME_SET, 00069 MLME_START, 00070 MLME_SYNC, 00071 MLME_SYNC_LOSS, 00072 MLME_POLL 00073 } mlme_primitive; 00074 00075 /** 00076 * \struct mac_description_storage_size_t 00077 * \brief Container for MAC storage sizes. 00078 */ 00079 typedef struct mac_description_storage_size_s { 00080 uint8_t device_decription_table_size; /**< MAC Device description list size */ 00081 uint8_t key_description_table_size; /**< MAC Key description list size */ 00082 uint8_t key_lookup_size; /**< Key description key lookup list size */ 00083 uint8_t key_usage_size; /**< Key description key usage list size */ 00084 } mac_description_storage_size_t; 00085 00086 /*! 00087 * \enum mac_extended_address_type 00088 * \brief Enum for MAC extended address types. 00089 */ 00090 typedef enum mac_extended_address_type { 00091 MAC_EXTENDED_READ_ONLY, /** EUID64 which is unique */ 00092 MAC_EXTENDED_DYNAMIC /** Configured MAC 64-bit address to RAM and Radio */ 00093 }mac_extended_address_type; 00094 //External MAC functions 00095 00096 /** 00097 * @brief mlme_associate_response 00098 * @param api API to handle the request 00099 * @param data MLME-ASSOCIATE.response specific values 00100 */ 00101 //typedef void mlme_associate_response(const mac_api_t* api, mlme_associate_resp_t *data); 00102 00103 //typedef void mlme_orphan_response(const mac_api_t* api, mlme_orphan_resp_t *data); 00104 00105 /** 00106 * @brief mlme_request 00107 * @param api API to handle the request 00108 * @param id The identifier of the MLME primitive 00109 * @param data Primitive specific data (\see mlme.h) 00110 */ 00111 typedef void mlme_request(const mac_api_t* api, mlme_primitive id, const void *data); 00112 00113 /** 00114 * @brief mcps_request MCPS_DATA request call 00115 * @param api API to handle the request 00116 * @param data MCPS-DATA.request specific values 00117 */ 00118 typedef void mcps_data_request(const mac_api_t* api, const mcps_data_req_t *data); 00119 00120 /** 00121 * @brief mcps_purge_request MCPS_PURGE request call 00122 * @param api API to handle the request 00123 * @param data MCPS-PURGE.request specific values 00124 */ 00125 typedef void mcps_purge_request(const mac_api_t* api, const mcps_purge_t *data); 00126 00127 //Upper layer specific callback functions (will also be set by Upper layer after mac_api_t has been created and given to it) 00128 00129 /** 00130 * @brief mcps_data_confirm MCPS-DATA confirm is called as a response to MCPS-DATA request 00131 * @param api The API which handled the response 00132 * @param data MCPS-DATA.confirm specific values 00133 */ 00134 typedef void mcps_data_confirm(const mac_api_t* api, const mcps_data_conf_t *data); 00135 00136 /** 00137 * @brief mcps_data_indication MCPS-DATA indication is called when MAC layer has received data 00138 * @param api The API which handled the response 00139 * @param data MCPS-DATA.indication specific values 00140 */ 00141 typedef void mcps_data_indication(const mac_api_t* api, const mcps_data_ind_t *data); 00142 00143 /** 00144 * @brief mcps_purge_confirm MCPS-PURGE confirm is called as a response to MCPS-PURGE request 00145 * @param api The API which handled the request 00146 * @param data MCPS-PURGE.confirm specific values 00147 */ 00148 typedef void mcps_purge_confirm( const mac_api_t* api, mcps_purge_conf_t *data ); 00149 00150 /** 00151 * @brief mlme_confirm One of the MLME primitive confirm callbacks 00152 * @param api API which handled the response 00153 * @param id The identifier of the MLME primitive 00154 * @param data Primitive specific data (\see mlme.h) 00155 */ 00156 typedef void mlme_confirm(const mac_api_t* api, mlme_primitive id, const void *data); 00157 00158 /** 00159 * @brief mlme_indication One of the 00160 * @param api API which handled the response 00161 * @param id The identifier of the MLME primitive 00162 * @param data Primitive specific data (\see mlme.h) 00163 */ 00164 typedef void mlme_indication(const mac_api_t* api, mlme_primitive id, const void *data); 00165 00166 /** 00167 * @brief Set extended address from MAC 00168 * @param api API to handle the request 00169 * @param mac64 pointer 00170 */ 00171 typedef int8_t mac_ext_mac64_address_set(const mac_api_t* api, const uint8_t *mac64); 00172 00173 /** 00174 * @brief Read extended address from MAC 00175 * @param api API to handle the request 00176 * @param mac64_buf Pointer where mac extended address can be written 00177 */ 00178 typedef int8_t mac_ext_mac64_address_get(const mac_api_t* api, mac_extended_address_type type, uint8_t *mac64_buf); 00179 00180 /** 00181 * @brief Read MAC security description storage sizes from MAC 00182 * @param api API to handle the request 00183 * @param buffer Pointer where supported sizes can be written 00184 */ 00185 typedef int8_t mac_storage_decription_sizes_get(const mac_api_t* api, mac_description_storage_size_t *buffer); 00186 00187 /** 00188 * @brief mac_api_initialize Initialises MAC layer into use, callbacks must be non-NULL. 00189 * @param api mac_api_t pointer, which is created by application. 00190 * @param data_conf_cb Upper layer function to handle MCPS confirmations 00191 * @param data_ind_cb Upper layer function to handle MCPS indications 00192 * @param mlme_conf_cb Upper layer function to handle MLME confirmations 00193 * @param mlme_ind_cb Upper layer function to handle MLME indications 00194 * @param parent_id Upper layer id, which is used in confirmation and indication callbacks 00195 * @return -1 if error, -2 if OOM, 0 otherwise 00196 */ 00197 typedef int8_t mac_api_initialize(mac_api_t *api, mcps_data_confirm *data_conf_cb, 00198 mcps_data_indication *data_ind_cb, mcps_purge_confirm *purge_conf_cb, mlme_confirm *mlme_conf_cb, 00199 mlme_indication *mlme_ind_cb, int8_t parent_id); 00200 00201 /** 00202 * \brief Struct mac_api_s defines functions for two-way communications between external MAC and Upper layer. 00203 * Application creates mac_api_t object by calling external MAC's creator function. 00204 * Then object is passed to Upper layer which then initializes it's own callback functions. 00205 * Then MAC is operated by Upper layer by calling MLME or MCPS primitive functions. 00206 */ 00207 struct mac_api_s { 00208 mac_api_initialize *mac_initialize; /**< MAC initialize function to use */ 00209 //External MAC callbacks 00210 mlme_request *mlme_req; /**< MAC MLME request function to use */ 00211 mcps_data_request *mcps_data_req; /**< MAC MCPS data request function to use */ 00212 mcps_purge_request *mcps_purge_req; /**< MAC MCPS purge request function to use */ 00213 00214 //Upper layer callbacksMLME_ASSOCIATE 00215 mcps_data_confirm *data_conf_cb; /**< MAC MCPS data confirm callback function */ 00216 mcps_data_indication *data_ind_cb; /**< MAC MCPS data indication callback function */ 00217 mcps_purge_confirm *purge_conf_cb; /**< MAC MCPS purge confirm callback function */ 00218 mlme_confirm *mlme_conf_cb; /**< MAC MLME confirm callback function */ 00219 mlme_indication *mlme_ind_cb; /**< MAC MLME indication callback function */ 00220 mac_ext_mac64_address_set *mac64_set; /**< MAC extension function to set mac64 address */ 00221 mac_ext_mac64_address_get *mac64_get; /**< MAC extension function to get mac64 address */ 00222 mac_storage_decription_sizes_get *mac_storage_sizes_get; /**< Getter function to query data storage sizes from MAC */ 00223 00224 int8_t parent_id; /**< Upper layer id */ 00225 uint16_t phyMTU; /**< Maximum Transmission Unit(MTU) used by MAC*/ 00226 }; 00227 00228 #ifdef __cplusplus 00229 } 00230 #endif 00231 00232 #endif // MAC_API_H
Generated on Tue Jul 12 2022 14:48:52 by
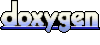