
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
lwip_pppcrypt.c
00001 /* 00002 * pppcrypt.c - PPP/DES linkage for MS-CHAP and EAP SRP-SHA1 00003 * 00004 * Extracted from chap_ms.c by James Carlson. 00005 * 00006 * Copyright (c) 1995 Eric Rosenquist. All rights reserved. 00007 * 00008 * Redistribution and use in source and binary forms, with or without 00009 * modification, are permitted provided that the following conditions 00010 * are met: 00011 * 00012 * 1. Redistributions of source code must retain the above copyright 00013 * notice, this list of conditions and the following disclaimer. 00014 * 00015 * 2. Redistributions in binary form must reproduce the above copyright 00016 * notice, this list of conditions and the following disclaimer in 00017 * the documentation and/or other materials provided with the 00018 * distribution. 00019 * 00020 * 3. The name(s) of the authors of this software must not be used to 00021 * endorse or promote products derived from this software without 00022 * prior written permission. 00023 * 00024 * THE AUTHORS OF THIS SOFTWARE DISCLAIM ALL WARRANTIES WITH REGARD TO 00025 * THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY 00026 * AND FITNESS, IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY 00027 * SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES 00028 * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN 00029 * AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING 00030 * OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00031 */ 00032 00033 #include "netif/ppp/ppp_opts.h" 00034 #if PPP_SUPPORT && MSCHAP_SUPPORT /* don't build if not necessary */ 00035 00036 #include "netif/ppp/ppp_impl.h" 00037 00038 #include "netif/ppp/pppcrypt.h" 00039 00040 00041 static u_char pppcrypt_get_7bits(u_char *input, int startBit) { 00042 unsigned int word; 00043 00044 word = (unsigned)input[startBit / 8] << 8; 00045 word |= (unsigned)input[startBit / 8 + 1]; 00046 00047 word >>= 15 - (startBit % 8 + 7); 00048 00049 return word & 0xFE; 00050 } 00051 00052 /* IN 56 bit DES key missing parity bits 00053 * OUT 64 bit DES key with parity bits added 00054 */ 00055 void pppcrypt_56_to_64_bit_key(u_char *key, u_char * des_key) { 00056 des_key[0] = pppcrypt_get_7bits(key, 0); 00057 des_key[1] = pppcrypt_get_7bits(key, 7); 00058 des_key[2] = pppcrypt_get_7bits(key, 14); 00059 des_key[3] = pppcrypt_get_7bits(key, 21); 00060 des_key[4] = pppcrypt_get_7bits(key, 28); 00061 des_key[5] = pppcrypt_get_7bits(key, 35); 00062 des_key[6] = pppcrypt_get_7bits(key, 42); 00063 des_key[7] = pppcrypt_get_7bits(key, 49); 00064 } 00065 00066 #endif /* PPP_SUPPORT && MSCHAP_SUPPORT */
Generated on Tue Jul 12 2022 14:48:51 by
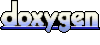