
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
fhss_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file fhss_api.h 00017 * \brief 00018 */ 00019 00020 #ifndef FHSS_API_H 00021 #define FHSS_API_H 00022 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 typedef struct fhss_api fhss_api_t; 00029 typedef struct fhss_callback fhss_callback_t; 00030 00031 /** 00032 * @brief FHSS frame types. 00033 */ 00034 #define FHSS_SYNCH_FRAME 0 /**< FHSS synchronization frame */ 00035 #define FHSS_SYNCH_REQUEST_FRAME 1 /**< FHSS synchronization request frame */ 00036 #define FHSS_DATA_FRAME 2 /**< FHSS data frame */ 00037 00038 /** 00039 * @brief FHSS synchronization info length. 00040 */ 00041 #define FHSS_SYNCH_INFO_LENGTH 21 00042 00043 /** 00044 * @brief FHSS states. 00045 */ 00046 typedef enum 00047 { 00048 FHSS_UNSYNCHRONIZED, 00049 FHSS_SYNCHRONIZED, 00050 } fhss_states; 00051 00052 /** 00053 * @brief FHSS is broadcast channel. Checks if current channel is broadcast channel. 00054 * @param api FHSS instance. 00055 * @return false if unicast channel, true if broadcast channel. 00056 */ 00057 typedef bool fhss_is_broadcast_channel(const fhss_api_t *api); 00058 00059 /** 00060 * @brief FHSS queue check. Checks if broadcast queue must be used instead of unicast queue. 00061 * @param api FHSS instance. 00062 * @param is_broadcast_addr Destination address type of packet (true if broadcast address). 00063 * @param frame_type Frame type of packet (Frames types are defined by FHSS api). 00064 * @return false if unicast queue, true if broadcast queue. 00065 */ 00066 typedef bool fhss_use_broadcast_queue(const fhss_api_t *api, bool is_broadcast_addr, int frame_type); 00067 00068 /** 00069 * @brief FHSS TX handle. Set destination channel and write synchronization info. 00070 * @param api FHSS instance. 00071 * @param is_broadcast_addr Destination address type of packet (true if broadcast address). 00072 * @param destination_address Destination MAC address. 00073 * @param frame_type Frame type of packet (Frames types are defined by FHSS api). 00074 * @param synch_info Pointer to where FHSS synchronization info is written (if synch frame). 00075 * @param frame_length MSDU length of the frame. 00076 * @param phy_header_length PHY header length. 00077 * @param phy_tail_length PHY tail length. 00078 * @return 0 Success. 00079 * @return -1 Transmission of the packet is currently not allowed, try again. 00080 * @return -2 Invalid api. 00081 * @return -3 Broadcast packet on Unicast channel (not allowed), push packet back to queue. 00082 * @return -4 Synchronization info missing. 00083 */ 00084 typedef int fhss_tx_handle(const fhss_api_t *api, bool is_broadcast_addr, uint8_t *destination_address, int frame_type, uint8_t *synch_info, uint16_t frame_length, uint8_t phy_header_length, uint8_t phy_tail_length); 00085 00086 /** 00087 * @brief Check TX permission. 00088 * @param api FHSS instance. 00089 * @param is_broadcast_addr Destination address type of packet (true if broadcast address). 00090 * @param handle Handle of the data request. 00091 * @param frame_type Frame type of packet (Frames types are defined by FHSS api). 00092 * @param frame_length MSDU length of the frame. 00093 * @param phy_header_length PHY header length. 00094 * @param phy_tail_length PHY tail length. 00095 * @return false if transmission is denied, true if transmission is allowed. 00096 */ 00097 typedef bool fhss_check_tx_conditions(const fhss_api_t *api, bool is_broadcast_addr, uint8_t handle, int frame_type, uint16_t frame_length, uint8_t phy_header_length, uint8_t phy_tail_length); 00098 00099 /** 00100 * @brief Notification of received FHSS synch or synch request frame. 00101 * @param api FHSS instance. 00102 * @param pan_id Source PAN id of the received frame (FHSS_SYNCH_FRAME only). 00103 * @param source_address Source address of the received frame (FHSS_SYNCH_FRAME only). 00104 * @param timestamp Timestamp of reception (FHSS_SYNCH_FRAME only). 00105 * @param synch_info Pointer to synchronization info (FHSS_SYNCH_FRAME only). 00106 * @param frame_type Frame type of packet (Frames types are defined by FHSS api). 00107 */ 00108 typedef void fhss_receive_frame(const fhss_api_t *api, uint16_t pan_id, uint8_t *source_address, uint32_t timestamp, uint8_t *synch_info, int frame_type); 00109 00110 /** 00111 * @brief Data TX done callback. 00112 * @param api FHSS instance. 00113 * @param waiting_ack MAC is waiting Acknowledgement for this frame. 00114 * @param tx_completed TX completed (Ack received or no more retries left (if unicast frame)). 00115 * @param handle Handle of the data request. 00116 */ 00117 typedef void fhss_data_tx_done(const fhss_api_t *api, bool waiting_ack, bool tx_completed, uint8_t handle); 00118 00119 /** 00120 * @brief Data TX or CCA failed callback. 00121 * @param api FHSS instance. 00122 * @param handle Handle of the data request. 00123 * @return true if frame has to be queued for retransmission, false otherwise. 00124 */ 00125 typedef bool fhss_data_tx_fail(const fhss_api_t *api, uint8_t handle); 00126 00127 /** 00128 * @brief Change synchronization state. 00129 * @param api FHSS instance. 00130 * @param fhss_state FHSS state (FHSS states are defined by FHSS api). 00131 * @param pan_id PAN id of the network FHSS synchronizes with. 00132 */ 00133 typedef void fhss_synch_state_set(const fhss_api_t *api, fhss_states fhss_state, uint16_t pan_id); 00134 00135 /** 00136 * @brief Read timestamp. 00137 * @param api FHSS instance. 00138 * @return Timestamp to be written in received frame. 00139 */ 00140 typedef uint32_t fhss_read_timestamp(const fhss_api_t *api); 00141 00142 /** 00143 * @brief Get retransmission period. FHSS uses different retry periods for different destinations. 00144 * @param api FHSS instance. 00145 * @param destination_address Destination MAC address. 00146 * @param phy_mtu PHY MTU size. 00147 * @return Retransmission period. 00148 */ 00149 typedef uint16_t fhss_get_retry_period(const fhss_api_t *api, uint8_t *destination_address, uint16_t phy_mtu); 00150 00151 /** 00152 * @brief Initialize MAC functions. 00153 * @param api FHSS instance. 00154 * @param callbacks MAC functions to be called from FHSS. 00155 * @return 0 Success. 00156 * @return -1 Invalid parameters. 00157 */ 00158 typedef int fhss_init_callbacks(const fhss_api_t *api, fhss_callback_t *callbacks); 00159 00160 /** 00161 * \brief Struct fhss_api defines interface between software MAC and FHSS. 00162 * Application creates fhss_api_s object by calling FHSS creator function. 00163 * Then object is passed to software MAC which then initialises it's own callback functions. 00164 */ 00165 struct fhss_api { 00166 fhss_is_broadcast_channel *is_broadcast_channel; /**< FHSS is broadcast channel. */ 00167 fhss_use_broadcast_queue *use_broadcast_queue; /**< FHSS queue check. */ 00168 fhss_tx_handle *tx_handle; /**< FHSS TX handle. */ 00169 fhss_check_tx_conditions *check_tx_conditions; /**< Check TX permission. */ 00170 fhss_receive_frame *receive_frame; /**< Notification of received FHSS synch or synch request frame. */ 00171 fhss_data_tx_done *data_tx_done; /**< Data TX done callback. */ 00172 fhss_data_tx_fail *data_tx_fail; /**< Data TX or CCA failed callback. */ 00173 fhss_synch_state_set *synch_state_set; /**< Change synchronization state. */ 00174 fhss_read_timestamp *read_timestamp; /**< Read timestamp. */ 00175 fhss_get_retry_period *get_retry_period; /**< Get retransmission period. */ 00176 fhss_init_callbacks *init_callbacks; /**< Initialize MAC functions. */ 00177 }; 00178 00179 /** 00180 * @brief Read MAC TX queue size. 00181 * @param fhss_api FHSS instance. 00182 * @param broadcast_queue Queue type to be read (true if broadcast queue). 00183 * @return Queue size (number of frames queued). 00184 */ 00185 typedef uint16_t mac_read_tx_queue_size(const fhss_api_t *fhss_api, bool broadcast_queue); 00186 00187 /** 00188 * @brief Read MAC address. 00189 * @param fhss_api FHSS instance. 00190 * @param mac_address MAC address pointer. 00191 * @return 0 Success. 00192 * @return -1 Invalid parameters. 00193 */ 00194 typedef int mac_read_mac_address(const fhss_api_t *fhss_api, uint8_t *mac_address); 00195 00196 /** 00197 * @brief Read PHY datarate. 00198 * @param fhss_api FHSS instance. 00199 * @return PHY datarate. 00200 */ 00201 typedef uint32_t mac_read_datarate(const fhss_api_t *fhss_api); 00202 00203 /** 00204 * @brief Change channel. 00205 * @param fhss_api FHSS instance. 00206 * @param channel_number Channel number. 00207 * @return 0 Success. 00208 * @return -1 Invalid parameters. 00209 */ 00210 typedef int mac_change_channel(const fhss_api_t *fhss_api, uint8_t channel_number); 00211 00212 /** 00213 * @brief Send FHSS frame. 00214 * @param fhss_api FHSS instance. 00215 * @param frame_type Frame type of packet (Frames types are defined by FHSS api). 00216 * @return 0 Success. 00217 * @return -1 Invalid parameters. 00218 */ 00219 typedef int mac_send_fhss_frame(const fhss_api_t *fhss_api, int frame_type); 00220 00221 /** 00222 * @brief Send notification when FHSS synchronization is lost. 00223 * @param fhss_api FHSS instance. 00224 * @return 0 Success. 00225 * @return -1 Invalid parameters. 00226 */ 00227 typedef int mac_synch_lost_notification(const fhss_api_t *fhss_api); 00228 00229 /** 00230 * @brief Poll TX queue. 00231 * @param fhss_api FHSS instance. 00232 * @return 0 Success. 00233 * @return -1 Invalid parameters. 00234 */ 00235 typedef int mac_tx_poll(const fhss_api_t *fhss_api); 00236 00237 /** 00238 * @brief Broadcast channel notification from FHSS. 00239 * @param fhss_api FHSS instance. 00240 * @param broadcast_time Remaining broadcast time. 00241 * @return 0 Success. 00242 * @return -1 Invalid parameters. 00243 */ 00244 typedef int mac_broadcast_notify(const fhss_api_t *fhss_api, uint32_t broadcast_time); 00245 00246 /** 00247 * @brief Read coordinator MAC address. 00248 * @param fhss_api FHSS instance. 00249 * @param mac_address MAC address pointer. 00250 * @return 0 Success. 00251 * @return -1 Invalid parameters. 00252 */ 00253 typedef int mac_read_coordinator_mac_address(const fhss_api_t *fhss_api, uint8_t *mac_address); 00254 00255 /** 00256 * \brief Struct fhss_callback defines functions that software MAC needs to implement. 00257 * Function pointers are passed to FHSS using fhss_init_callbacks function. 00258 */ 00259 struct fhss_callback { 00260 mac_read_tx_queue_size *read_tx_queue_size; /**< Read MAC TX queue size. */ 00261 mac_read_mac_address *read_mac_address; /**< Read MAC address. */ 00262 mac_read_datarate *read_datarate; /**< Read PHY datarate. */ 00263 mac_change_channel *change_channel; /**< Change channel. */ 00264 mac_send_fhss_frame *send_fhss_frame; /**< Send FHSS frame. */ 00265 mac_synch_lost_notification *synch_lost_notification; /**< Send notification when FHSS synchronization is lost. */ 00266 mac_tx_poll *tx_poll; /**< Poll TX queue. */ 00267 mac_broadcast_notify *broadcast_notify; /**< Broadcast channel notification from FHSS. */ 00268 mac_read_coordinator_mac_address *read_coord_mac_address; /**< Read coordinator MAC address. */ 00269 }; 00270 00271 #ifdef __cplusplus 00272 } 00273 #endif 00274 00275 #endif // FHSS_API_H
Generated on Tue Jul 12 2022 14:48:46 by
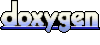