
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
platform.h
00001 /** 00002 * \file platform.h 00003 * 00004 * \brief mbed TLS Platform abstraction layer 00005 * 00006 * Copyright (C) 2006-2016, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_PLATFORM_H 00024 #define MBEDTLS_PLATFORM_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #if defined(MBEDTLS_HAVE_TIME) 00033 #include "mbedtls/platform_time.h" 00034 #endif 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** 00041 * \name SECTION: Module settings 00042 * 00043 * The configuration options you can set for this module are in this section. 00044 * Either change them in config.h or define them on the compiler command line. 00045 * \{ 00046 */ 00047 00048 #if !defined(MBEDTLS_PLATFORM_NO_STD_FUNCTIONS) 00049 #include <stdio.h> 00050 #include <stdlib.h> 00051 #include <time.h> 00052 #if !defined(MBEDTLS_PLATFORM_STD_SNPRINTF) 00053 #if defined(_WIN32) 00054 #define MBEDTLS_PLATFORM_STD_SNPRINTF mbedtls_platform_win32_snprintf /**< Default snprintf to use */ 00055 #else 00056 #define MBEDTLS_PLATFORM_STD_SNPRINTF snprintf /**< Default snprintf to use */ 00057 #endif 00058 #endif 00059 #if !defined(MBEDTLS_PLATFORM_STD_PRINTF) 00060 #define MBEDTLS_PLATFORM_STD_PRINTF printf /**< Default printf to use */ 00061 #endif 00062 #if !defined(MBEDTLS_PLATFORM_STD_FPRINTF) 00063 #define MBEDTLS_PLATFORM_STD_FPRINTF fprintf /**< Default fprintf to use */ 00064 #endif 00065 #if !defined(MBEDTLS_PLATFORM_STD_CALLOC) 00066 #define MBEDTLS_PLATFORM_STD_CALLOC calloc /**< Default allocator to use */ 00067 #endif 00068 #if !defined(MBEDTLS_PLATFORM_STD_FREE) 00069 #define MBEDTLS_PLATFORM_STD_FREE free /**< Default free to use */ 00070 #endif 00071 #if !defined(MBEDTLS_PLATFORM_STD_EXIT) 00072 #define MBEDTLS_PLATFORM_STD_EXIT exit /**< Default exit to use */ 00073 #endif 00074 #if !defined(MBEDTLS_PLATFORM_STD_TIME) 00075 #define MBEDTLS_PLATFORM_STD_TIME time /**< Default time to use */ 00076 #endif 00077 #if !defined(MBEDTLS_PLATFORM_STD_EXIT_SUCCESS) 00078 #define MBEDTLS_PLATFORM_STD_EXIT_SUCCESS EXIT_SUCCESS /**< Default exit value to use */ 00079 #endif 00080 #if !defined(MBEDTLS_PLATFORM_STD_EXIT_FAILURE) 00081 #define MBEDTLS_PLATFORM_STD_EXIT_FAILURE EXIT_FAILURE /**< Default exit value to use */ 00082 #endif 00083 #if defined(MBEDTLS_FS_IO) 00084 #if !defined(MBEDTLS_PLATFORM_STD_NV_SEED_READ) 00085 #define MBEDTLS_PLATFORM_STD_NV_SEED_READ mbedtls_platform_std_nv_seed_read 00086 #endif 00087 #if !defined(MBEDTLS_PLATFORM_STD_NV_SEED_WRITE) 00088 #define MBEDTLS_PLATFORM_STD_NV_SEED_WRITE mbedtls_platform_std_nv_seed_write 00089 #endif 00090 #if !defined(MBEDTLS_PLATFORM_STD_NV_SEED_FILE) 00091 #define MBEDTLS_PLATFORM_STD_NV_SEED_FILE "seedfile" 00092 #endif 00093 #endif /* MBEDTLS_FS_IO */ 00094 #else /* MBEDTLS_PLATFORM_NO_STD_FUNCTIONS */ 00095 #if defined(MBEDTLS_PLATFORM_STD_MEM_HDR) 00096 #include MBEDTLS_PLATFORM_STD_MEM_HDR 00097 #endif 00098 #endif /* MBEDTLS_PLATFORM_NO_STD_FUNCTIONS */ 00099 00100 00101 /* \} name SECTION: Module settings */ 00102 00103 /* 00104 * The function pointers for calloc and free 00105 */ 00106 #if defined(MBEDTLS_PLATFORM_MEMORY) 00107 #if defined(MBEDTLS_PLATFORM_FREE_MACRO) && \ 00108 defined(MBEDTLS_PLATFORM_CALLOC_MACRO) 00109 #define mbedtls_free MBEDTLS_PLATFORM_FREE_MACRO 00110 #define mbedtls_calloc MBEDTLS_PLATFORM_CALLOC_MACRO 00111 #else 00112 /* For size_t */ 00113 #include <stddef.h> 00114 extern void * (*mbedtls_calloc)( size_t n, size_t size ); 00115 extern void (*mbedtls_free)( void *ptr ); 00116 00117 /** 00118 * \brief Set your own memory implementation function pointers 00119 * 00120 * \param calloc_func the calloc function implementation 00121 * \param free_func the free function implementation 00122 * 00123 * \return 0 if successful 00124 */ 00125 int mbedtls_platform_set_calloc_free( void * (*calloc_func)( size_t, size_t ), 00126 void (*free_func)( void * ) ); 00127 #endif /* MBEDTLS_PLATFORM_FREE_MACRO && MBEDTLS_PLATFORM_CALLOC_MACRO */ 00128 #else /* !MBEDTLS_PLATFORM_MEMORY */ 00129 #define mbedtls_free free 00130 #define mbedtls_calloc calloc 00131 #endif /* MBEDTLS_PLATFORM_MEMORY && !MBEDTLS_PLATFORM_{FREE,CALLOC}_MACRO */ 00132 00133 /* 00134 * The function pointers for fprintf 00135 */ 00136 #if defined(MBEDTLS_PLATFORM_FPRINTF_ALT) 00137 /* We need FILE * */ 00138 #include <stdio.h> 00139 extern int (*mbedtls_fprintf)( FILE *stream, const char *format, ... ); 00140 00141 /** 00142 * \brief Set your own fprintf function pointer 00143 * 00144 * \param fprintf_func the fprintf function implementation 00145 * 00146 * \return 0 00147 */ 00148 int mbedtls_platform_set_fprintf( int (*fprintf_func)( FILE *stream, const char *, 00149 ... ) ); 00150 #else 00151 #if defined(MBEDTLS_PLATFORM_FPRINTF_MACRO) 00152 #define mbedtls_fprintf MBEDTLS_PLATFORM_FPRINTF_MACRO 00153 #else 00154 #define mbedtls_fprintf fprintf 00155 #endif /* MBEDTLS_PLATFORM_FPRINTF_MACRO */ 00156 #endif /* MBEDTLS_PLATFORM_FPRINTF_ALT */ 00157 00158 /* 00159 * The function pointers for printf 00160 */ 00161 #if defined(MBEDTLS_PLATFORM_PRINTF_ALT) 00162 extern int (*mbedtls_printf)( const char *format, ... ); 00163 00164 /** 00165 * \brief Set your own printf function pointer 00166 * 00167 * \param printf_func the printf function implementation 00168 * 00169 * \return 0 00170 */ 00171 int mbedtls_platform_set_printf( int (*printf_func)( const char *, ... ) ); 00172 #else /* !MBEDTLS_PLATFORM_PRINTF_ALT */ 00173 #if defined(MBEDTLS_PLATFORM_PRINTF_MACRO) 00174 #define mbedtls_printf MBEDTLS_PLATFORM_PRINTF_MACRO 00175 #else 00176 #define mbedtls_printf printf 00177 #endif /* MBEDTLS_PLATFORM_PRINTF_MACRO */ 00178 #endif /* MBEDTLS_PLATFORM_PRINTF_ALT */ 00179 00180 /* 00181 * The function pointers for snprintf 00182 * 00183 * The snprintf implementation should conform to C99: 00184 * - it *must* always correctly zero-terminate the buffer 00185 * (except when n == 0, then it must leave the buffer untouched) 00186 * - however it is acceptable to return -1 instead of the required length when 00187 * the destination buffer is too short. 00188 */ 00189 #if defined(_WIN32) 00190 /* For Windows (inc. MSYS2), we provide our own fixed implementation */ 00191 int mbedtls_platform_win32_snprintf( char *s, size_t n, const char *fmt, ... ); 00192 #endif 00193 00194 #if defined(MBEDTLS_PLATFORM_SNPRINTF_ALT) 00195 extern int (*mbedtls_snprintf)( char * s, size_t n, const char * format, ... ); 00196 00197 /** 00198 * \brief Set your own snprintf function pointer 00199 * 00200 * \param snprintf_func the snprintf function implementation 00201 * 00202 * \return 0 00203 */ 00204 int mbedtls_platform_set_snprintf( int (*snprintf_func)( char * s, size_t n, 00205 const char * format, ... ) ); 00206 #else /* MBEDTLS_PLATFORM_SNPRINTF_ALT */ 00207 #if defined(MBEDTLS_PLATFORM_SNPRINTF_MACRO) 00208 #define mbedtls_snprintf MBEDTLS_PLATFORM_SNPRINTF_MACRO 00209 #else 00210 #define mbedtls_snprintf snprintf 00211 #endif /* MBEDTLS_PLATFORM_SNPRINTF_MACRO */ 00212 #endif /* MBEDTLS_PLATFORM_SNPRINTF_ALT */ 00213 00214 /* 00215 * The function pointers for exit 00216 */ 00217 #if defined(MBEDTLS_PLATFORM_EXIT_ALT) 00218 extern void (*mbedtls_exit)( int status ); 00219 00220 /** 00221 * \brief Set your own exit function pointer 00222 * 00223 * \param exit_func the exit function implementation 00224 * 00225 * \return 0 00226 */ 00227 int mbedtls_platform_set_exit( void (*exit_func)( int status ) ); 00228 #else 00229 #if defined(MBEDTLS_PLATFORM_EXIT_MACRO) 00230 #define mbedtls_exit MBEDTLS_PLATFORM_EXIT_MACRO 00231 #else 00232 #define mbedtls_exit exit 00233 #endif /* MBEDTLS_PLATFORM_EXIT_MACRO */ 00234 #endif /* MBEDTLS_PLATFORM_EXIT_ALT */ 00235 00236 /* 00237 * The default exit values 00238 */ 00239 #if defined(MBEDTLS_PLATFORM_STD_EXIT_SUCCESS) 00240 #define MBEDTLS_EXIT_SUCCESS MBEDTLS_PLATFORM_STD_EXIT_SUCCESS 00241 #else 00242 #define MBEDTLS_EXIT_SUCCESS 0 00243 #endif 00244 #if defined(MBEDTLS_PLATFORM_STD_EXIT_FAILURE) 00245 #define MBEDTLS_EXIT_FAILURE MBEDTLS_PLATFORM_STD_EXIT_FAILURE 00246 #else 00247 #define MBEDTLS_EXIT_FAILURE 1 00248 #endif 00249 00250 /* 00251 * The function pointers for reading from and writing a seed file to 00252 * Non-Volatile storage (NV) in a platform-independent way 00253 * 00254 * Only enabled when the NV seed entropy source is enabled 00255 */ 00256 #if defined(MBEDTLS_ENTROPY_NV_SEED) 00257 #if !defined(MBEDTLS_PLATFORM_NO_STD_FUNCTIONS) && defined(MBEDTLS_FS_IO) 00258 /* Internal standard platform definitions */ 00259 int mbedtls_platform_std_nv_seed_read( unsigned char *buf, size_t buf_len ); 00260 int mbedtls_platform_std_nv_seed_write( unsigned char *buf, size_t buf_len ); 00261 #endif 00262 00263 #if defined(MBEDTLS_PLATFORM_NV_SEED_ALT) 00264 extern int (*mbedtls_nv_seed_read)( unsigned char *buf, size_t buf_len ); 00265 extern int (*mbedtls_nv_seed_write)( unsigned char *buf, size_t buf_len ); 00266 00267 /** 00268 * \brief Set your own seed file writing/reading functions 00269 * 00270 * \param nv_seed_read_func the seed reading function implementation 00271 * \param nv_seed_write_func the seed writing function implementation 00272 * 00273 * \return 0 00274 */ 00275 int mbedtls_platform_set_nv_seed( 00276 int (*nv_seed_read_func)( unsigned char *buf, size_t buf_len ), 00277 int (*nv_seed_write_func)( unsigned char *buf, size_t buf_len ) 00278 ); 00279 #else 00280 #if defined(MBEDTLS_PLATFORM_NV_SEED_READ_MACRO) && \ 00281 defined(MBEDTLS_PLATFORM_NV_SEED_WRITE_MACRO) 00282 #define mbedtls_nv_seed_read MBEDTLS_PLATFORM_NV_SEED_READ_MACRO 00283 #define mbedtls_nv_seed_write MBEDTLS_PLATFORM_NV_SEED_WRITE_MACRO 00284 #else 00285 #define mbedtls_nv_seed_read mbedtls_platform_std_nv_seed_read 00286 #define mbedtls_nv_seed_write mbedtls_platform_std_nv_seed_write 00287 #endif 00288 #endif /* MBEDTLS_PLATFORM_NV_SEED_ALT */ 00289 #endif /* MBEDTLS_ENTROPY_NV_SEED */ 00290 00291 #if !defined(MBEDTLS_PLATFORM_SETUP_TEARDOWN_ALT) 00292 00293 /** 00294 * \brief Platform context structure 00295 * 00296 * \note This structure may be used to assist platform-specific 00297 * setup/teardown operations. 00298 */ 00299 typedef struct { 00300 char dummy; /**< Placeholder member as empty structs are not portable */ 00301 } 00302 mbedtls_platform_context; 00303 00304 #else 00305 #include "platform_alt.h" 00306 #endif /* !MBEDTLS_PLATFORM_SETUP_TEARDOWN_ALT */ 00307 00308 /** 00309 * \brief Perform any platform initialisation operations 00310 * 00311 * \param ctx mbed TLS context 00312 * 00313 * \return 0 if successful 00314 * 00315 * \note This function is intended to allow platform specific initialisation, 00316 * and should be called before any other library functions. Its 00317 * implementation is platform specific, and by default, unless platform 00318 * specific code is provided, it does nothing. 00319 * 00320 * Its use and whether its necessary to be called is dependent on the 00321 * platform. 00322 */ 00323 int mbedtls_platform_setup( mbedtls_platform_context *ctx ); 00324 /** 00325 * \brief Perform any platform teardown operations 00326 * 00327 * \param ctx mbed TLS context 00328 * 00329 * \note This function should be called after every other mbed TLS module has 00330 * been correctly freed using the appropriate free function. 00331 * Its implementation is platform specific, and by default, unless 00332 * platform specific code is provided, it does nothing. 00333 * 00334 * Its use and whether its necessary to be called is dependent on the 00335 * platform. 00336 */ 00337 void mbedtls_platform_teardown( mbedtls_platform_context *ctx ); 00338 00339 #ifdef __cplusplus 00340 } 00341 #endif 00342 00343 #endif /* platform.h */
Generated on Tue Jul 12 2022 14:48:56 by
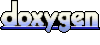