
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
coap_message_handler.h
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 * 00004 * SPDX-License-Identifier: Apache-2.0 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00007 * not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00014 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 00019 #ifndef __COAP_MESSAGE_HANDLER_H__ 00020 #define __COAP_MESSAGE_HANDLER_H__ 00021 00022 #include <inttypes.h> 00023 #include "mbed-coap/sn_coap_header.h" 00024 #include "ns_list.h" 00025 00026 #define TRANSACTION_LIFETIME 180 00027 /* Default value for CoAP duplicate message buffer (0 = disabled) */ 00028 #define DUPLICATE_MESSAGE_BUFFER_SIZE 0 00029 00030 /** 00031 * \brief Service message response receive callback. 00032 * 00033 * Function that handles CoAP service message receiving and parsing 00034 * 00035 * \param msg_id Id number of the current message. 00036 * \param response_ptr Pointer to CoAP header structure. 00037 * 00038 * \return 0 for success / -1 for failure 00039 */ 00040 typedef int coap_message_handler_response_recv(int8_t service_id, uint8_t source_address[static 16], uint16_t source_port, sn_coap_hdr_s *response_ptr); 00041 00042 typedef struct coap_msg_handler_s { 00043 void *(*sn_coap_service_malloc)(uint16_t); 00044 void (*sn_coap_service_free)(void *); 00045 00046 uint8_t (*sn_coap_tx_callback)(uint8_t *, uint16_t, sn_nsdl_addr_s *, void *); 00047 00048 struct coap_s *coap; 00049 } coap_msg_handler_t; 00050 00051 typedef struct coap_transaction { 00052 uint8_t remote_address[16]; 00053 uint8_t local_address[16]; 00054 uint8_t token[4]; 00055 uint32_t create_time; 00056 uint16_t remote_port; 00057 uint16_t msg_id; 00058 uint16_t data_len; 00059 int8_t service_id; 00060 uint8_t options; 00061 uint8_t *data_ptr; 00062 bool client_request: 1; 00063 00064 coap_message_handler_response_recv *resp_cb; 00065 ns_list_link_t link; 00066 } coap_transaction_t; 00067 00068 00069 extern coap_msg_handler_t *coap_message_handler_init(void *(*used_malloc_func_ptr)(uint16_t), void (*used_free_func_ptr)(void *), 00070 uint8_t (*used_tx_callback_ptr)(uint8_t *, uint16_t, sn_nsdl_addr_s *, void *)); 00071 00072 extern int8_t coap_message_handler_destroy(coap_msg_handler_t *handle); 00073 00074 extern coap_transaction_t *coap_message_handler_transaction_valid(coap_transaction_t *tr_ptr); 00075 00076 extern coap_transaction_t *coap_message_handler_find_transaction(uint8_t *address_ptr, uint16_t port); 00077 00078 extern int16_t coap_message_handler_coap_msg_process(coap_msg_handler_t *handle, int8_t socket_id, const uint8_t source_addr_ptr[static 16], uint16_t port, const uint8_t dst_addr_ptr[static 16], 00079 uint8_t *data_ptr, uint16_t data_len, int16_t (cb)(int8_t, sn_coap_hdr_s *, coap_transaction_t *)); 00080 00081 extern uint16_t coap_message_handler_request_send(coap_msg_handler_t *handle, int8_t service_id, uint8_t options, const uint8_t destination_addr[static 16], 00082 uint16_t destination_port, sn_coap_msg_type_e msg_type, sn_coap_msg_code_e msg_code, const char *uri, sn_coap_content_format_e cont_type, 00083 const uint8_t *payload_ptr, uint16_t payload_len, coap_message_handler_response_recv *request_response_cb); 00084 00085 extern int8_t coap_message_handler_response_send(coap_msg_handler_t *handle, int8_t service_id, uint8_t options, sn_coap_hdr_s *request_ptr, sn_coap_msg_code_e message_code, 00086 sn_coap_content_format_e content_type, const uint8_t *payload_ptr, uint16_t payload_len); 00087 00088 extern int8_t coap_message_handler_request_delete(coap_msg_handler_t *handle, int8_t service_id, uint16_t msg_id); 00089 00090 extern int8_t coap_message_handler_exec(coap_msg_handler_t *handle, uint32_t current_time); 00091 00092 extern void transaction_delete(coap_transaction_t *this); 00093 00094 extern void transactions_delete_all(uint8_t *address_ptr, uint16_t port); 00095 00096 #endif
Generated on Tue Jul 12 2022 14:48:40 by
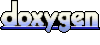