
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
coap_connection_handler.h
00001 /* 00002 * Copyright (c) 2015-2017 ARM Limited. All Rights Reserved. 00003 * 00004 * SPDX-License-Identifier: Apache-2.0 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00007 * not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00014 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 */ 00018 00019 #ifndef __COAP_CONNECTION_HANDLER_H__ 00020 #define __COAP_CONNECTION_HANDLER_H__ 00021 00022 #include <inttypes.h> 00023 #include <stddef.h> 00024 #include <stdbool.h> 00025 #include "ns_address.h" 00026 #include "coap_service_api_internal.h" 00027 00028 #define MAX_SECURE_SESSION_COUNT 3 00029 #define CLOSED_SECURE_SESSION_TIMEOUT 3600 // Seconds 00030 #define OPEN_SECURE_SESSION_TIMEOUT 18000 // Seconds 00031 #define SECURE_SESSION_CLEAN_INTERVAL 60 // Seconds 00032 00033 struct internal_socket_s; 00034 00035 typedef int send_to_socket_cb(int8_t socket_id, const uint8_t address[static 16], uint16_t port, const void *, int); 00036 typedef int receive_from_socket_cb(int8_t socket_id, uint8_t src_address[static 16], uint16_t port, const uint8_t dst_address[static 16], unsigned char *, int); 00037 typedef int get_pw_cb(int8_t socket_id, uint8_t address[static 16], uint16_t port, uint8_t *pw_ptr, uint8_t *pw_len); 00038 typedef void security_done_cb(int8_t socket_id, uint8_t address[static 16], uint16_t port, uint8_t keyblock[static 40]); 00039 00040 typedef struct coap_conn_handler_s{ 00041 struct internal_socket_s *socket; 00042 00043 receive_from_socket_cb *_recv_cb; 00044 send_to_socket_cb *_send_cb; 00045 get_pw_cb *_get_password_cb; 00046 security_done_cb *_security_done_cb; 00047 int8_t socket_interface_selection; 00048 bool registered_to_multicast; 00049 } coap_conn_handler_t; 00050 00051 coap_conn_handler_t *connection_handler_create(receive_from_socket_cb *recv_from_cb, 00052 send_to_socket_cb *send_to_cb, 00053 get_pw_cb *pw_cb, 00054 security_done_cb *done_cb); 00055 00056 void connection_handler_destroy( coap_conn_handler_t *handler, bool multicast_group_leave); 00057 00058 void connection_handler_close_secure_connection( coap_conn_handler_t *handler, uint8_t destination_addr_ptr[static 16], uint16_t port ); 00059 00060 int coap_connection_handler_open_connection(coap_conn_handler_t *handler, uint16_t listen_port, bool use_ephemeral_port, bool is_secure, bool real_socket, bool bypassSec); 00061 00062 //If returns -2, it means security was started and data was not send 00063 int coap_connection_handler_send_data(coap_conn_handler_t *handler, const ns_address_t *dest_addr, const uint8_t src_address[static 16], uint8_t *data_ptr, uint16_t data_len, bool bypass_link_sec); 00064 00065 int coap_connection_handler_virtual_recv(coap_conn_handler_t *handler, uint8_t address[static 16], uint16_t port, uint8_t *data_ptr, uint16_t data_len); 00066 00067 bool coap_connection_handler_socket_belongs_to(coap_conn_handler_t *handler, int8_t socket_id); 00068 00069 int8_t coap_connection_handler_set_timeout(coap_conn_handler_t *handler, uint32_t min, uint32_t max); 00070 00071 void coap_connection_handler_exec(uint32_t time); 00072 00073 #endif
Generated on Tue Jul 12 2022 14:48:40 by
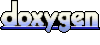