
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
arm_hal_phy.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file arm_hal_phy.h 00017 * \brief PHY device driver API. 00018 */ 00019 00020 #ifndef ARM_HAL_PHY_H_ 00021 #define ARM_HAL_PHY_H_ 00022 00023 #include "ns_types.h" 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 /** Interface states */ 00030 typedef enum { 00031 PHY_INTERFACE_RESET, /**< Reset PHY driver and set to idle. */ 00032 PHY_INTERFACE_DOWN, /**< Disable PHY interface driver (RF radio disable). */ 00033 PHY_INTERFACE_UP, /**< Enable PHY interface driver (RF radio receiver ON). */ 00034 PHY_INTERFACE_RX_ENERGY_STATE, /**< Enable wireless interface ED scan mode. */ 00035 PHY_INTERFACE_SNIFFER_STATE /**< Enable sniffer mode. */ 00036 } phy_interface_state_e; 00037 00038 /** TX process return codes */ 00039 typedef enum { 00040 PHY_LINK_TX_DONE, /**< TX process ready and ACK RX. */ 00041 PHY_LINK_TX_DONE_PENDING, /**< TX process OK with ACK pending flag. */ 00042 PHY_LINK_TX_SUCCESS, /**< MAC TX complete. MAC will a make decision to enter wait ACK or TX done state. */ 00043 PHY_LINK_TX_FAIL, /**< Link TX process fail. */ 00044 PHY_LINK_CCA_FAIL, /**< RF link CCA process fail. */ 00045 } phy_link_tx_status_e; 00046 00047 /** Extension types */ 00048 typedef enum { 00049 PHY_EXTENSION_CTRL_PENDING_BIT, /**< Control MAC pending bit for indirect data. */ 00050 PHY_EXTENSION_READ_LAST_ACK_PENDING_STATUS, /**< Read status if the last ACK is still pending. */ 00051 PHY_EXTENSION_SET_CHANNEL, /**< Net library channel set. */ 00052 PHY_EXTENSION_READ_CHANNEL_ENERGY, /**< RF interface ED scan energy read. */ 00053 PHY_EXTENSION_READ_LINK_STATUS, /**< Net library could read link status. */ 00054 PHY_EXTENSION_CONVERT_SIGNAL_INFO, /**< Convert signal info. */ 00055 PHY_EXTENSION_ACCEPT_ANY_BEACON, /**< Set boolean true or false for accept beacon from other Pan-ID than configured. Default value should be false */ 00056 } phy_extension_type_e; 00057 00058 /** Address types */ 00059 typedef enum { 00060 PHY_MAC_48BIT, /**< IPv4/IPv6/BLE link layer address for Ethernet. This is optional. */ 00061 PHY_MAC_64BIT, /**< RF/PLC link layer address. */ 00062 PHY_MAC_16BIT, /**< RF interface short address. */ 00063 PHY_MAC_PANID, /**< RF interface 16-Bit PAN-ID. */ 00064 } phy_address_type_e; 00065 00066 /** PHY types */ 00067 typedef enum phy_link_type_e { 00068 PHY_LINK_ETHERNET_TYPE, /**< Standard IEEE 802 Ethernet. */ 00069 PHY_LINK_15_4_2_4GHZ_TYPE, /**< Standard 802.15.4 2.4GHz radio. */ 00070 PHY_LINK_15_4_SUBGHZ_TYPE, /**< Standard 802.15.4 subGHz radio 868 /915MHz. */ 00071 PHY_LINK_TUN, /**< Tunnel interface for Linux TUN, RF network driver over serial bus or just basic application to application data flow. */ 00072 PHY_LINK_SLIP, /**< Generic SLIP driver which just forward SLIP payload */ 00073 } phy_link_type_e; 00074 00075 /** Data layers */ 00076 typedef enum data_protocol_e { 00077 LOCAL_SOCKET_DATA = 0, /**< 6LoWPAN library local socket data. */ 00078 INTERFACE_DATA = 1, /**< 6LoWPAN library interface internal used protocol. */ 00079 PHY_LAYER_PAYLOAD = 2, /**< PHY layer data selection or handler. */ 00080 IPV6_DATAGRAM = 3, /**< IP layer data or TUN driver request data. */ 00081 UNKNOWN_PROTOCOL = 4 /**< Non-supported protocol ID. */ 00082 } data_protocol_e; 00083 00084 /** Requested data layer */ 00085 typedef enum driver_data_request_e { 00086 PHY_LAYER_PAYLOAD_DATA_FLOW, /**< PHY layer data. */ 00087 IPV6_DATAGRAMS_DATA_FLOW, /**< IP layer data or TUN driver request data. */ 00088 } driver_data_request_e; 00089 00090 /** \brief Signal info types. 00091 * 00092 * Types of signal quality indication desired by various link protocols. Some are 00093 * really statistical, but a driver should ideally be able to create an estimate 00094 * based on its LQI/DBM numbers, for example to bootstrap a statistic calculation. 00095 */ 00096 typedef enum phy_signal_info_type_e { 00097 PHY_SIGNAL_INFO_ETX, /**< Expected transmissions, unsigned 16-bit fixed-point ETX*128 [1..512], for example Zigbee IP + RFC 6719. */ 00098 PHY_SIGNAL_INFO_IDR, /**< Inverse Delivery Ratio, unsigned 16-bit fixed-point IDR*32*256 [1..8], for example MLE draft 06. */ 00099 PHY_SIGNAL_INFO_LINK_MARGIN, /**< Link margin, unsigned 16-bit fixed-point dB*256, [0..255], for example Thread routing draft. */ 00100 } phy_signal_info_type_e; 00101 00102 /** Signal level info */ 00103 typedef struct phy_signal_info_s { 00104 phy_signal_info_type_e type; /**< Signal info type desired. */ 00105 uint8_t lqi; /**< Quality passed to arm_net_phy_rx. */ 00106 int8_t dbm; /**< Strength passed to arm_net_phy_rx. */ 00107 uint16_t result; /**< Resulting signal information. */ 00108 } phy_signal_info_s; 00109 00110 /** PHY modulation scheme */ 00111 typedef enum phy_modulation_e 00112 { 00113 M_OFDM, ///< QFDM 00114 M_OQPSK, ///< OQPSK 00115 M_BPSK, ///< BPSK 00116 M_GFSK, ///< GFSK 00117 M_UNDEFINED ///< UNDEFINED 00118 } phy_modulation_e; 00119 00120 /** Channel page numbers */ 00121 typedef enum 00122 { 00123 CHANNEL_PAGE_0 = 0, ///< Page 0 00124 CHANNEL_PAGE_1 = 1, ///< Page 1 00125 CHANNEL_PAGE_2 = 2, ///< Page 2 00126 CHANNEL_PAGE_3 = 3, ///< Page 3 00127 CHANNEL_PAGE_4 = 4, ///< Page 4 00128 CHANNEL_PAGE_5 = 5, ///< Page 5 00129 CHANNEL_PAGE_6 = 6, ///< Page 6 00130 CHANNEL_PAGE_9 = 9, ///< Page 9 00131 CHANNEL_PAGE_10 = 10 ///< Page 10 00132 } channel_page_e; 00133 00134 /** Channel configuration */ 00135 typedef struct phy_rf_channel_configuration_s 00136 { 00137 uint32_t channel_0_center_frequency; ///< Center frequency 00138 uint32_t channel_spacing; ///< Channel spacing 00139 uint32_t datarate; ///< Data rate 00140 uint16_t number_of_channels; ///< Number of channels 00141 phy_modulation_e modulation; ///< Modulation scheme 00142 } phy_rf_channel_configuration_s; 00143 00144 /** Channel page configuration */ 00145 typedef struct phy_device_channel_page_s 00146 { 00147 channel_page_e channel_page; ///< Channel page 00148 const phy_rf_channel_configuration_s *rf_channel_configuration; ///< Pointer to channel configuration 00149 } phy_device_channel_page_s; 00150 00151 /** Virtual data request */ 00152 typedef struct virtual_data_req_s { 00153 uint16_t parameter_length; /**< Length of user specified header. Can be zero. */ 00154 uint8_t *parameters; /**< Pointer to user specified header. Optional */ 00155 uint16_t msduLength; /**< MSDU Length */ 00156 const uint8_t *msdu; /**< MSDU */ 00157 } virtual_data_req_t; 00158 00159 /** 00160 * @brief arm_net_phy_rx RX callback set by upper layer. Called when data is received 00161 * @param data_ptr Data received 00162 * @param data_len Length of the data received 00163 * @param link_quality Link quality 00164 * @param dbm Power ratio in decibels 00165 * @param driver_id ID of driver which received data 00166 * @return 0 if success, error otherwise 00167 */ 00168 typedef int8_t arm_net_phy_rx_fn(const uint8_t *data_ptr, uint16_t data_len, uint8_t link_quality, int8_t dbm, int8_t driver_id); 00169 00170 /** 00171 * @brief arm_net_phy_tx_done TX done callback set by upper layer. Called when tx sent by upper layer has been handled 00172 * @param driver_id Id of the driver which handled TX request 00173 * @param tx_handle Handle of the TX 00174 * @param status Status code of the TX handling result 00175 * @param cca_retry Number of CCA retries done during handling 00176 * @param tx_retry Number of TX retries done during handling 00177 * @return 0 if success, error otherwise 00178 */ 00179 typedef int8_t arm_net_phy_tx_done_fn(int8_t driver_id, uint8_t tx_handle, phy_link_tx_status_e status, uint8_t cca_retry, uint8_t tx_retry); 00180 00181 /** 00182 * @brief arm_net_virtual_rx RX callback set by user of serial MAC. Called when virtual RF has received data. 00183 * @param data_ptr Data received 00184 * @param data_len Length of the data received 00185 * @param driver_id ID of driver which received data 00186 * @return 0 if success, error otherwise 00187 */ 00188 typedef int8_t arm_net_virtual_rx_fn(const uint8_t *data_ptr, uint16_t data_len,int8_t driver_id); 00189 00190 /** 00191 * @brief arm_net_virtual_tx TX callback set by serial MAC. Used to send data. 00192 * @param data_req Data to be sent 00193 * @param driver_id Id of the driver to be used. 00194 * @return 0 if success, error otherwise 00195 */ 00196 typedef int8_t arm_net_virtual_tx_fn(const virtual_data_req_t *data_req,int8_t driver_id); 00197 00198 /** 00199 * @brief arm_net_virtual_config Configuration receive callback set by upper layer. Used to receive internal configuration parameters. 00200 * @param driver_id Id of the driver to be used. 00201 * @param data Pointer to received configuration data. 00202 * @param length Length of the configuration data. 00203 * @return 0 if success, error otherwise 00204 */ 00205 typedef int8_t arm_net_virtual_config_rx_fn(int8_t driver_id, const uint8_t *data, uint16_t length); 00206 00207 /** 00208 * @brief arm_net_virtual_config Configuration send callback set by upper layer. Used to send internal configuration parameters. 00209 * @param driver_id Id of the driver to be used. 00210 * @param data Pointer to sent configuration data. 00211 * @param length Length of the configuration data. 00212 * @return 0 if success, error otherwise 00213 */ 00214 typedef int8_t arm_net_virtual_config_tx_fn(int8_t driver_id, const uint8_t *data, uint16_t length); 00215 00216 /** 00217 * @brief arm_net_virtual_confirmation Confirmation receive callback set by upper layer. Used to receive MLME confirmation data. 00218 * @param driver_id Id of the driver to be used. 00219 * @param data Pointer to received confirmation data. 00220 * @param length Length of the confirmation data. 00221 * @return 0 if success, error otherwise 00222 */ 00223 typedef int8_t arm_net_virtual_confirmation_rx_fn(int8_t driver_id, const uint8_t *data, uint16_t length); 00224 00225 /** Device driver structure */ 00226 typedef struct phy_device_driver_s 00227 { 00228 phy_link_type_e link_type; /**< Define driver types. */ 00229 driver_data_request_e data_request_layer; /**< Define interface data OUT protocol. */ 00230 uint8_t *PHY_MAC; /**< Pointer to 64-bit or 48-bit MAC address. */ 00231 uint16_t phy_MTU; /**< Define MAX PHY layer MTU size. */ 00232 char *driver_description; /**< Short driver platform description. Needs to end with zero. */ 00233 uint8_t phy_tail_length; /**< Define PHY driver needed TAIL Length. */ 00234 uint8_t phy_header_length; /**< Define PHY driver needed header length before PDU. */ 00235 int8_t (*state_control)(phy_interface_state_e, uint8_t); /**< Function pointer for control PHY driver state. */ 00236 int8_t (*tx)(uint8_t *, uint16_t, uint8_t, data_protocol_e); /**< Function pointer for PHY driver write operation. */ 00237 int8_t (*address_write)(phy_address_type_e , uint8_t *); /**< Function pointer for PHY driver address write. */ 00238 int8_t (*extension)(phy_extension_type_e, uint8_t *); /**< Function pointer for PHY driver extension control. */ 00239 const phy_device_channel_page_s *phy_channel_pages; /**< Pointer to channel page list */ 00240 00241 //Upper layer callbacks, set with arm_net_phy_register(); 00242 arm_net_phy_rx_fn *phy_rx_cb; /**< PHY RX callback. Initialized by \ref arm_net_phy_register(). */ 00243 arm_net_phy_tx_done_fn *phy_tx_done_cb; /**< Transmission done callback. Initialized by \ref arm_net_phy_register(). */ 00244 //Virtual upper data rx 00245 arm_net_virtual_rx_fn *arm_net_virtual_rx_cb; /**< Virtual RX callback. Initialized by \ref arm_net_phy_register(). */ 00246 arm_net_virtual_tx_fn *arm_net_virtual_tx_cb; /**< Virtual TX callback. Initialized by \ref arm_net_phy_register(). */ 00247 arm_net_virtual_config_rx_fn *virtual_config_rx_cb; /**< Virtual config receive callback. Initialized by \ref arm_net_phy_register(). */ 00248 arm_net_virtual_config_tx_fn *virtual_config_tx_cb; /**< Virtual config send callback. Initialized by \ref arm_net_phy_register(). */ 00249 arm_net_virtual_confirmation_rx_fn *virtual_confirmation_rx_cb; /**< Virtual confirmation receive callback. Initialized by \ref arm_net_phy_register(). */ 00250 uint16_t tunnel_type; /**< Tun driver type. */ 00251 } phy_device_driver_s; 00252 00253 00254 /** 00255 * \brief This function registers the device driver to stack. 00256 * 00257 * \param phy_driver A pointer to device driver structure. 00258 * 00259 * \return >= 0 Device driver ID. 00260 * \return < 0 Means register fail. 00261 * 00262 */ 00263 extern int8_t arm_net_phy_register(phy_device_driver_s *phy_driver); 00264 00265 00266 /** 00267 * \brief Set driver mac64 address. 00268 * 00269 * \param MAC A pointer to new mac64 address which is copied to old one. 00270 * \param id driver id 00271 * 00272 * \return >= 0 SET OK. 00273 * \return < 0 Means register fail. 00274 * 00275 */ 00276 extern int8_t arm_net_phy_mac64_set(uint8_t *MAC, int8_t id); 00277 00278 /** 00279 * \brief Get driver mac64 address. 00280 * 00281 * \param id driver id 00282 * 00283 * \return > 0 Return pointer to MAC. 00284 * \return NULL. 00285 * 00286 */ 00287 extern uint8_t *arm_net_phy_mac64_get(int8_t id); 00288 00289 /** 00290 * \brief Get driver link type. 00291 * 00292 * \param id driver id 00293 * 00294 * \return driver link type. 00295 * 00296 */ 00297 extern int arm_net_phy_rf_type(int8_t id); 00298 00299 /** 00300 * \brief Get driver link type MTU size. 00301 * 00302 * \param id driver id 00303 * 00304 * \return size of MTU. 00305 * 00306 */ 00307 extern uint16_t arm_net_phy_mtu_size(int8_t id); 00308 00309 /** 00310 * \brief Unregister the driver from storage. 00311 * 00312 * \param driver_id driver id 00313 * 00314 */ 00315 extern void arm_net_phy_unregister(int8_t driver_id); 00316 00317 #ifdef __cplusplus 00318 } 00319 #endif 00320 #endif /* ARM_HAL_PHY_H_ */
Generated on Tue Jul 12 2022 14:48:38 by
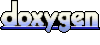