
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
arm_hal_aes.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file arm_hal_aes.h 00017 * \brief AES Platform API 00018 */ 00019 00020 #ifndef ARM_HAL_AES_H_ 00021 #define ARM_HAL_AES_H_ 00022 00023 /* The network library requires a simple AES implementation for its 00024 * IEEE 802.15.4 security. The expectation is that 802.15.4 devices will have 00025 * some hardware acceleration, which should be made available through this API. 00026 * 00027 * If no hardware acceleration is available, the API can be easily hooked up to 00028 * an external software library such as mbed TLS. 00029 * 00030 * To simplify porting, the API is used single-threaded, so that no special care 00031 * is needed to maintain global context or state in the hardware accelerator. 00032 */ 00033 00034 #include "ns_types.h" 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** 00041 * \brief Set the AES key 00042 * 00043 * This function sets the 128-bit AES key that will be used for future 00044 * calls to arm_aes_encrypt(). The key must be copied by the function. 00045 * 00046 * \param key pointer to 128-bit AES key 00047 */ 00048 void arm_aes_start(const uint8_t key[__static 16]); 00049 00050 /** 00051 * \brief This function performs dst=E[preset key,src] (Simple ECB block). 00052 * 00053 * This function performs a single-block AES encryption, using the preset key. 00054 * It is called between arm_aes_start() and arm_aes_finish(). 00055 * Note that src and dst pointers may be equal. 00056 * 00057 * \param src pointer to 128-bit plaintext in 00058 * \param dst pointer for 128-bit ciphertext out 00059 */ 00060 extern void arm_aes_encrypt( 00061 const uint8_t src[__static 16], 00062 uint8_t dst[__static 16]); 00063 00064 /** 00065 * \brief Finish AES operations 00066 * 00067 * This function is called to terminate a series of AES operations. 00068 * It may be a no-op, or it may disable AES hardware. Use of the preset key is 00069 * no longer valid after this call. 00070 */ 00071 void arm_aes_finish(void); 00072 00073 #ifdef __cplusplus 00074 } 00075 #endif 00076 #endif /* ARM_HAL_AES_H_ */
Generated on Tue Jul 12 2022 14:48:38 by
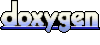