
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
UARTSerial.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_UARTSERIAL_H 00018 #define MBED_UARTSERIAL_H 00019 00020 #include "platform/platform.h" 00021 00022 #if (DEVICE_SERIAL && DEVICE_INTERRUPTIN) || defined(DOXYGEN_ONLY) 00023 00024 #include "FileHandle.h" 00025 #include "SerialBase.h" 00026 #include "InterruptIn.h" 00027 #include "PlatformMutex.h" 00028 #include "serial_api.h" 00029 #include "CircularBuffer.h" 00030 #include "platform/NonCopyable.h" 00031 00032 #ifndef MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE 00033 #define MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE 256 00034 #endif 00035 00036 #ifndef MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE 00037 #define MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE 256 00038 #endif 00039 00040 namespace mbed { 00041 00042 /** \addtogroup drivers */ 00043 00044 /** Class providing buffered UART communication functionality using separate circular buffer for send and receive channels 00045 * 00046 * @ingroup drivers 00047 */ 00048 00049 class UARTSerial : private SerialBase, public FileHandle, private NonCopyable<UARTSerial> { 00050 00051 public: 00052 00053 /** Create a UARTSerial port, connected to the specified transmit and receive pins, with a particular baud rate. 00054 * @param tx Transmit pin 00055 * @param rx Receive pin 00056 * @param baud The baud rate of the serial port (optional, defaults to MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE) 00057 */ 00058 UARTSerial(PinName tx, PinName rx, int baud = MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE); 00059 virtual ~UARTSerial(); 00060 00061 /** Equivalent to POSIX poll(). Derived from FileHandle. 00062 * Provides a mechanism to multiplex input/output over a set of file handles. 00063 */ 00064 virtual short poll(short events) const; 00065 00066 /* Resolve ambiguities versus our private SerialBase 00067 * (for writable, spelling differs, but just in case) 00068 */ 00069 using FileHandle::readable; 00070 using FileHandle::writable; 00071 00072 /** Write the contents of a buffer to a file 00073 * 00074 * @param buffer The buffer to write from 00075 * @param length The number of bytes to write 00076 * @return The number of bytes written, negative error on failure 00077 */ 00078 virtual ssize_t write(const void* buffer, size_t length); 00079 00080 /** Read the contents of a file into a buffer 00081 * 00082 * Follows POSIX semantics: 00083 * 00084 * * if no data is available, and non-blocking set return -EAGAIN 00085 * * if no data is available, and blocking set, wait until data is available 00086 * * If any data is available, call returns immediately 00087 * 00088 * @param buffer The buffer to read in to 00089 * @param length The number of bytes to read 00090 * @return The number of bytes read, 0 at end of file, negative error on failure 00091 */ 00092 virtual ssize_t read(void* buffer, size_t length); 00093 00094 /** Close a file 00095 * 00096 * @return 0 on success, negative error code on failure 00097 */ 00098 virtual int close(); 00099 00100 /** Check if the file in an interactive terminal device 00101 * 00102 * @return True if the file is a terminal 00103 * @return False if the file is not a terminal 00104 * @return Negative error code on failure 00105 */ 00106 virtual int isatty(); 00107 00108 /** Move the file position to a given offset from from a given location 00109 * 00110 * Not valid for a device type FileHandle like UARTSerial. 00111 * In case of UARTSerial, returns ESPIPE 00112 * 00113 * @param offset The offset from whence to move to 00114 * @param whence The start of where to seek 00115 * SEEK_SET to start from beginning of file, 00116 * SEEK_CUR to start from current position in file, 00117 * SEEK_END to start from end of file 00118 * @return The new offset of the file, negative error code on failure 00119 */ 00120 virtual off_t seek(off_t offset, int whence); 00121 00122 /** Flush any buffers associated with the file 00123 * 00124 * @return 0 on success, negative error code on failure 00125 */ 00126 virtual int sync(); 00127 00128 /** Set blocking or non-blocking mode 00129 * The default is blocking. 00130 * 00131 * @param blocking true for blocking mode, false for non-blocking mode. 00132 */ 00133 virtual int set_blocking(bool blocking) 00134 { 00135 _blocking = blocking; 00136 return 0; 00137 } 00138 00139 /** Register a callback on state change of the file. 00140 * 00141 * The specified callback will be called on state changes such as when 00142 * the file can be written to or read from. 00143 * 00144 * The callback may be called in an interrupt context and should not 00145 * perform expensive operations. 00146 * 00147 * Note! This is not intended as an attach-like asynchronous api, but rather 00148 * as a building block for constructing such functionality. 00149 * 00150 * The exact timing of when the registered function 00151 * is called is not guaranteed and susceptible to change. It should be used 00152 * as a cue to make read/write/poll calls to find the current state. 00153 * 00154 * @param func Function to call on state change 00155 */ 00156 virtual void sigio(Callback<void()> func); 00157 00158 /** Setup interrupt handler for DCD line 00159 * 00160 * If DCD line is connected, an IRQ handler will be setup. 00161 * Does nothing if DCD is NC, i.e., not connected. 00162 * 00163 * @param dcd_pin Pin-name for DCD 00164 * @param active_high a boolean set to true if DCD polarity is active low 00165 */ 00166 void set_data_carrier_detect(PinName dcd_pin, bool active_high = false); 00167 00168 /** Set the baud rate 00169 * 00170 * @param baud The baud rate 00171 */ 00172 void set_baud(int baud); 00173 00174 // Expose private SerialBase::Parity as UARTSerial::Parity 00175 using SerialBase::Parity; 00176 // In C++11, we wouldn't need to also have using directives for each value 00177 using SerialBase::None; 00178 using SerialBase::Odd; 00179 using SerialBase::Even; 00180 using SerialBase::Forced1; 00181 using SerialBase::Forced0; 00182 00183 /** Set the transmission format used by the serial port 00184 * 00185 * @param bits The number of bits in a word (5-8; default = 8) 00186 * @param parity The parity used (None, Odd, Even, Forced1, Forced0; default = None) 00187 * @param stop_bits The number of stop bits (1 or 2; default = 1) 00188 */ 00189 void set_format(int bits=8, Parity parity=UARTSerial::None, int stop_bits=1); 00190 00191 #if DEVICE_SERIAL_FC 00192 // For now use the base enum - but in future we may have extra options 00193 // such as XON/XOFF or manual GPIO RTSCTS. 00194 using SerialBase::Flow; 00195 // In C++11, we wouldn't need to also have using directives for each value 00196 using SerialBase::Disabled; 00197 using SerialBase::RTS; 00198 using SerialBase::CTS; 00199 using SerialBase::RTSCTS; 00200 00201 /** Set the flow control type on the serial port 00202 * 00203 * @param type the flow control type (Disabled, RTS, CTS, RTSCTS) 00204 * @param flow1 the first flow control pin (RTS for RTS or RTSCTS, CTS for CTS) 00205 * @param flow2 the second flow control pin (CTS for RTSCTS) 00206 */ 00207 void set_flow_control(Flow type, PinName flow1=NC, PinName flow2=NC); 00208 #endif 00209 00210 private: 00211 00212 void wait_ms(uint32_t millisec); 00213 00214 /** SerialBase lock override */ 00215 virtual void lock(void); 00216 00217 /** SerialBase unlock override */ 00218 virtual void unlock(void); 00219 00220 /** Acquire mutex */ 00221 virtual void api_lock(void); 00222 00223 /** Release mutex */ 00224 virtual void api_unlock(void); 00225 00226 /** Software serial buffers 00227 * By default buffer size is 256 for TX and 256 for RX. Configurable through mbed_app.json 00228 */ 00229 CircularBuffer<char, MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE> _rxbuf; 00230 CircularBuffer<char, MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE> _txbuf; 00231 00232 PlatformMutex _mutex; 00233 00234 Callback<void()> _sigio_cb; 00235 00236 bool _blocking; 00237 bool _tx_irq_enabled; 00238 bool _rx_irq_enabled; 00239 InterruptIn *_dcd_irq; 00240 00241 /** Device Hanged up 00242 * Determines if the device hanged up on us. 00243 * 00244 * @return True, if hanged up 00245 */ 00246 bool hup() const; 00247 00248 /** ISRs for serial 00249 * Routines to handle interrupts on serial pins. 00250 * Copies data into Circular Buffer. 00251 * Reports the state change to File handle. 00252 */ 00253 void tx_irq(void); 00254 void rx_irq(void); 00255 00256 void wake(void); 00257 00258 void dcd_irq(void); 00259 00260 }; 00261 } //namespace mbed 00262 00263 #endif //(DEVICE_SERIAL && DEVICE_INTERRUPTIN) || defined(DOXYGEN_ONLY) 00264 #endif //MBED_UARTSERIAL_H
Generated on Tue Jul 12 2022 14:49:09 by
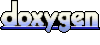