
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
Timer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_TIMER_H 00017 #define MBED_TIMER_H 00018 00019 #include "platform/platform.h" 00020 #include "hal/ticker_api.h" 00021 #include "platform/NonCopyable.h" 00022 #include "platform/mbed_sleep.h" 00023 00024 namespace mbed { 00025 /** \addtogroup drivers */ 00026 00027 /** A general purpose timer 00028 * 00029 * @note Synchronization level: Interrupt safe 00030 * 00031 * Example: 00032 * @code 00033 * // Count the time to toggle a LED 00034 * 00035 * #include "mbed.h" 00036 * 00037 * Timer timer; 00038 * DigitalOut led(LED1); 00039 * int begin, end; 00040 * 00041 * int main() { 00042 * timer.start(); 00043 * begin = timer.read_us(); 00044 * led = !led; 00045 * end = timer.read_us(); 00046 * printf("Toggle the led takes %d us", end - begin); 00047 * } 00048 * @endcode 00049 * @ingroup drivers 00050 */ 00051 class Timer : private NonCopyable<Timer> { 00052 00053 public: 00054 Timer(); 00055 Timer(const ticker_data_t *data); 00056 ~Timer(); 00057 00058 /** Start the timer 00059 */ 00060 void start(); 00061 00062 /** Stop the timer 00063 */ 00064 void stop(); 00065 00066 /** Reset the timer to 0. 00067 * 00068 * If it was already counting, it will continue 00069 */ 00070 void reset(); 00071 00072 /** Get the time passed in seconds 00073 * 00074 * @returns Time passed in seconds 00075 */ 00076 float read(); 00077 00078 /** Get the time passed in milli-seconds 00079 * 00080 * @returns Time passed in milli seconds 00081 */ 00082 int read_ms(); 00083 00084 /** Get the time passed in micro-seconds 00085 * 00086 * @returns Time passed in micro seconds 00087 */ 00088 int read_us(); 00089 00090 /** An operator shorthand for read() 00091 */ 00092 operator float(); 00093 00094 /** Get in a high resolution type the time passed in micro-seconds. 00095 */ 00096 us_timestamp_t read_high_resolution_us(); 00097 00098 protected: 00099 us_timestamp_t slicetime(); 00100 int _running; // whether the timer is running 00101 us_timestamp_t _start; // the start time of the latest slice 00102 us_timestamp_t _time; // any accumulated time from previous slices 00103 const ticker_data_t *_ticker_data; 00104 bool _lock_deepsleep; // flag which indicates if deep-sleep should be disabled 00105 }; 00106 00107 } // namespace mbed 00108 00109 #endif
Generated on Tue Jul 12 2022 14:49:08 by
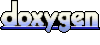