
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
TCPSocket.h
00001 00002 /** \addtogroup netsocket */ 00003 /** @{*/ 00004 /* TCPSocket 00005 * Copyright (c) 2015 ARM Limited 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 00020 #ifndef TCPSOCKET_H 00021 #define TCPSOCKET_H 00022 00023 #include "netsocket/Socket.h" 00024 #include "netsocket/NetworkStack.h" 00025 #include "netsocket/NetworkInterface.h" 00026 #include "rtos/EventFlags.h" 00027 00028 00029 /** TCP socket connection 00030 */ 00031 class TCPSocket : public Socket { 00032 public: 00033 /** Create an uninitialized socket 00034 * 00035 * Must call open to initialize the socket on a network stack. 00036 */ 00037 TCPSocket(); 00038 00039 /** Create a socket on a network interface 00040 * 00041 * Creates and opens a socket on the network stack of the given 00042 * network interface. 00043 * 00044 * @param stack Network stack as target for socket 00045 */ 00046 template <typename S> 00047 TCPSocket(S *stack) 00048 : _pending(0), _event_flag(0), 00049 _read_in_progress(false), _write_in_progress(false) 00050 { 00051 open(stack); 00052 } 00053 00054 /** Destroy a socket 00055 * 00056 * Closes socket if the socket is still open 00057 */ 00058 virtual ~TCPSocket(); 00059 00060 /** Override multicast functions to return error for TCP 00061 * 00062 */ 00063 int join_multicast_group(const SocketAddress &address) { return NSAPI_ERROR_UNSUPPORTED ; } 00064 00065 /** Connects TCP socket to a remote host 00066 * 00067 * Initiates a connection to a remote server specified by either 00068 * a domain name or an IP address and a port. 00069 * 00070 * @param host Hostname of the remote host 00071 * @param port Port of the remote host 00072 * @return 0 on success, negative error code on failure 00073 */ 00074 nsapi_error_t connect(const char *host, uint16_t port); 00075 00076 /** Connects TCP socket to a remote host 00077 * 00078 * Initiates a connection to a remote server specified by the 00079 * indicated address. 00080 * 00081 * @param address The SocketAddress of the remote host 00082 * @return 0 on success, negative error code on failure 00083 */ 00084 nsapi_error_t connect(const SocketAddress &address); 00085 00086 /** Send data over a TCP socket 00087 * 00088 * The socket must be connected to a remote host. Returns the number of 00089 * bytes sent from the buffer. 00090 * 00091 * By default, send blocks until data is sent. If socket is set to 00092 * non-blocking or times out, NSAPI_ERROR_WOULD_BLOCK is returned 00093 * immediately. 00094 * 00095 * @param data Buffer of data to send to the host 00096 * @param size Size of the buffer in bytes 00097 * @return Number of sent bytes on success, negative error 00098 * code on failure 00099 */ 00100 nsapi_size_or_error_t send(const void *data, nsapi_size_t size); 00101 00102 /** Receive data over a TCP socket 00103 * 00104 * The socket must be connected to a remote host. Returns the number of 00105 * bytes received into the buffer. 00106 * 00107 * By default, recv blocks until data is sent. If socket is set to 00108 * non-blocking or times out, NSAPI_ERROR_WOULD_BLOCK is returned 00109 * immediately. 00110 * 00111 * @param data Destination buffer for data received from the host 00112 * @param size Size of the buffer in bytes 00113 * @return Number of received bytes on success, negative error 00114 * code on failure 00115 */ 00116 nsapi_size_or_error_t recv(void *data, nsapi_size_t size); 00117 00118 protected: 00119 friend class TCPServer; 00120 00121 virtual nsapi_protocol_t get_proto(); 00122 virtual void event(); 00123 00124 volatile unsigned _pending; 00125 rtos::EventFlags _event_flag; 00126 bool _read_in_progress; 00127 bool _write_in_progress; 00128 }; 00129 00130 00131 #endif 00132 00133 /** @}*/
Generated on Tue Jul 12 2022 14:49:04 by
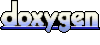