
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
MeshInterfaceNanostack.cpp
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "MeshInterfaceNanostack.h" 00018 #include "NanostackInterface.h" 00019 #include "mesh_system.h" 00020 00021 MeshInterfaceNanostack::MeshInterfaceNanostack() 00022 : phy(NULL), _network_interface_id(-1), _device_id(-1), eui64(), 00023 ip_addr_str(), mac_addr_str(), connect_semaphore(0) 00024 { 00025 // Nothing to do 00026 } 00027 00028 MeshInterfaceNanostack::MeshInterfaceNanostack(NanostackPhy *phy) 00029 : phy(phy), _network_interface_id(-1), _device_id(-1), connect_semaphore(0) 00030 { 00031 // Nothing to do 00032 } 00033 00034 nsapi_error_t MeshInterfaceNanostack::initialize(NanostackPhy *phy) 00035 { 00036 mesh_system_init(); 00037 if (this->phy != NULL) { 00038 error("Phy already set"); 00039 } 00040 this->phy = phy; 00041 return 0; 00042 } 00043 00044 void MeshInterfaceNanostack::mesh_network_handler(mesh_connection_status_t status) 00045 { 00046 nanostack_lock(); 00047 00048 if (status == MESH_CONNECTED) { 00049 connect_semaphore.release(); 00050 } 00051 00052 nanostack_unlock(); 00053 } 00054 00055 nsapi_error_t MeshInterfaceNanostack::register_phy() 00056 { 00057 nanostack_lock(); 00058 00059 _device_id = phy->phy_register(); 00060 if (_device_id < 0) { 00061 nanostack_unlock(); 00062 return -1; 00063 } 00064 // Read mac address after registering the device. 00065 phy->get_mac_address(eui64); 00066 sprintf(mac_addr_str, "%02x:%02x:%02x:%02x:%02x:%02x:%02x:%02x", eui64[0], eui64[1], eui64[2], eui64[3], eui64[4], eui64[5], eui64[6], eui64[7]); 00067 00068 nanostack_unlock(); 00069 00070 return 0; 00071 } 00072 00073 NetworkStack * MeshInterfaceNanostack::get_stack() 00074 { 00075 return NanostackInterface::get_stack(); 00076 } 00077 00078 const char *MeshInterfaceNanostack::get_ip_address() 00079 { 00080 nanostack_lock(); 00081 00082 const char *ret = NULL; 00083 if (getOwnIpAddress(ip_addr_str, sizeof ip_addr_str)) { 00084 ret = ip_addr_str; 00085 } 00086 00087 nanostack_unlock(); 00088 00089 return ret; 00090 } 00091 00092 const char *MeshInterfaceNanostack::get_mac_address() 00093 { 00094 return mac_addr_str; 00095 }
Generated on Tue Jul 12 2022 14:48:54 by
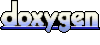