
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
LocalFileSystem.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_LOCALFILESYSTEM_H 00017 #define MBED_LOCALFILESYSTEM_H 00018 00019 #include "platform/platform.h" 00020 00021 #if DEVICE_LOCALFILESYSTEM 00022 00023 #include "platform/FileSystemLike.h" 00024 #include "platform/PlatformMutex.h" 00025 #include "platform/NonCopyable.h" 00026 00027 namespace mbed { 00028 /** \addtogroup platform */ 00029 /** @{*/ 00030 /** 00031 * \defgroup platform_LocalFileSystem LocalFileSystem functions 00032 * @{ 00033 */ 00034 00035 FILEHANDLE local_file_open(const char* name, int flags); 00036 00037 /** 00038 * @class LocalFileHandle 00039 * @ingroup platform 00040 */ 00041 class LocalFileHandle : public FileHandle, private NonCopyable<LocalFileHandle> { 00042 00043 public: 00044 LocalFileHandle(FILEHANDLE fh); 00045 00046 virtual int close(); 00047 00048 virtual ssize_t write(const void *buffer, size_t length); 00049 00050 virtual ssize_t read(void *buffer, size_t length); 00051 00052 virtual int isatty(); 00053 00054 virtual off_t seek(off_t position, int whence); 00055 00056 virtual int sync(); 00057 00058 virtual off_t size(); 00059 00060 protected: 00061 virtual void lock(); 00062 virtual void unlock(); 00063 FILEHANDLE _fh; 00064 int pos; 00065 PlatformMutex _mutex; 00066 }; 00067 00068 /** A filesystem for accessing the local mbed Microcontroller USB disk drive 00069 * 00070 * This allows programs to read and write files on the same disk drive that is used to program the 00071 * mbed Microcontroller. Once created, the standard C file access functions are used to open, 00072 * read and write files. 00073 * 00074 * @note Synchronization level: Thread safe 00075 * 00076 * Example: 00077 * @code 00078 * #include "mbed.h" 00079 * 00080 * LocalFileSystem local("local"); // Create the local filesystem under the name "local" 00081 * 00082 * int main() { 00083 * FILE *fp = fopen("/local/out.txt", "w"); // Open "out.txt" on the local file system for writing 00084 * fprintf(fp, "Hello World!"); 00085 * fclose(fp); 00086 * remove("/local/out.txt"); // Removes the file "out.txt" from the local file system 00087 * 00088 * DIR *d = opendir("/local"); // Opens the root directory of the local file system 00089 * struct dirent *p; 00090 * while((p = readdir(d)) != NULL) { // Print the names of the files in the local file system 00091 * printf("%s\n", p->d_name); // to stdout. 00092 * } 00093 * closedir(d); 00094 * } 00095 * @endcode 00096 * 00097 * @note 00098 * If the microcontroller program makes an access to the local drive, it will be marked as "removed" 00099 * on the Host computer. This means it is no longer accessible from the Host Computer. 00100 * 00101 * The drive will only re-appear when the microcontroller program exists. Note that if the program does 00102 * not exit, you will need to hold down reset on the mbed Microcontroller to be able to see the drive again! 00103 * @ingroup platform 00104 */ 00105 class LocalFileSystem : public FileSystemLike, private NonCopyable<LocalFileSystem> { 00106 // No modifiable state 00107 00108 public: 00109 LocalFileSystem(const char* n) : FileSystemLike(n) { 00110 00111 } 00112 00113 virtual int open(FileHandle **file, const char *path, int flags); 00114 virtual int open(DirHandle **dir, const char *name); 00115 virtual int remove(const char *filename); 00116 }; 00117 00118 /**@}*/ 00119 00120 /**@}*/ 00121 00122 } // namespace mbed 00123 00124 #endif 00125 00126 #endif 00127
Generated on Tue Jul 12 2022 14:48:49 by
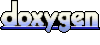