
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
HeapBlockDevice.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "HeapBlockDevice.h" 00018 00019 00020 HeapBlockDevice::HeapBlockDevice(bd_size_t size, bd_size_t block) 00021 : _read_size(block), _program_size(block), _erase_size(block) 00022 , _count(size / block), _blocks(0) 00023 { 00024 MBED_ASSERT(_count * _erase_size == size); 00025 } 00026 00027 HeapBlockDevice::HeapBlockDevice(bd_size_t size, bd_size_t read, bd_size_t program, bd_size_t erase) 00028 : _read_size(read), _program_size(program), _erase_size(erase) 00029 , _count(size / erase), _blocks(0) 00030 { 00031 MBED_ASSERT(_count * _erase_size == size); 00032 } 00033 00034 HeapBlockDevice::~HeapBlockDevice() 00035 { 00036 if (_blocks) { 00037 for (size_t i = 0; i < _count; i++) { 00038 free(_blocks[i]); 00039 } 00040 00041 delete[] _blocks; 00042 _blocks = 0; 00043 } 00044 } 00045 00046 int HeapBlockDevice::init() 00047 { 00048 if (!_blocks) { 00049 _blocks = new uint8_t*[_count]; 00050 for (size_t i = 0; i < _count; i++) { 00051 _blocks[i] = 0; 00052 } 00053 } 00054 00055 return BD_ERROR_OK; 00056 } 00057 00058 int HeapBlockDevice::deinit() 00059 { 00060 MBED_ASSERT(_blocks != NULL); 00061 // Memory is lazily cleaned up in destructor to allow 00062 // data to live across de/reinitialization 00063 return BD_ERROR_OK; 00064 } 00065 00066 bd_size_t HeapBlockDevice::get_read_size() const 00067 { 00068 MBED_ASSERT(_blocks != NULL); 00069 return _read_size; 00070 } 00071 00072 bd_size_t HeapBlockDevice::get_program_size() const 00073 { 00074 MBED_ASSERT(_blocks != NULL); 00075 return _program_size; 00076 } 00077 00078 bd_size_t HeapBlockDevice::get_erase_size() const 00079 { 00080 MBED_ASSERT(_blocks != NULL); 00081 return _erase_size; 00082 } 00083 00084 bd_size_t HeapBlockDevice::size() const 00085 { 00086 MBED_ASSERT(_blocks != NULL); 00087 return _count * _erase_size; 00088 } 00089 00090 int HeapBlockDevice::read(void *b, bd_addr_t addr, bd_size_t size) 00091 { 00092 MBED_ASSERT(_blocks != NULL); 00093 MBED_ASSERT(is_valid_read(addr, size)); 00094 uint8_t *buffer = static_cast<uint8_t*>(b); 00095 00096 while (size > 0) { 00097 bd_addr_t hi = addr / _erase_size; 00098 bd_addr_t lo = addr % _erase_size; 00099 00100 if (_blocks[hi]) { 00101 memcpy(buffer, &_blocks[hi][lo], _read_size); 00102 } else { 00103 memset(buffer, 0, _read_size); 00104 } 00105 00106 buffer += _read_size; 00107 addr += _read_size; 00108 size -= _read_size; 00109 } 00110 00111 return 0; 00112 } 00113 00114 int HeapBlockDevice::program(const void *b, bd_addr_t addr, bd_size_t size) 00115 { 00116 MBED_ASSERT(_blocks != NULL); 00117 MBED_ASSERT(is_valid_program(addr, size)); 00118 const uint8_t *buffer = static_cast<const uint8_t*>(b); 00119 00120 while (size > 0) { 00121 bd_addr_t hi = addr / _erase_size; 00122 bd_addr_t lo = addr % _erase_size; 00123 00124 if (!_blocks[hi]) { 00125 _blocks[hi] = (uint8_t*)malloc(_erase_size); 00126 if (!_blocks[hi]) { 00127 return BD_ERROR_DEVICE_ERROR; 00128 } 00129 } 00130 00131 memcpy(&_blocks[hi][lo], buffer, _program_size); 00132 00133 buffer += _program_size; 00134 addr += _program_size; 00135 size -= _program_size; 00136 } 00137 00138 return 0; 00139 } 00140 00141 int HeapBlockDevice::erase(bd_addr_t addr, bd_size_t size) 00142 { 00143 MBED_ASSERT(_blocks != NULL); 00144 MBED_ASSERT(is_valid_erase(addr, size)); 00145 // TODO assert on programming unerased blocks 00146 00147 return 0; 00148 } 00149
Generated on Tue Jul 12 2022 14:48:48 by
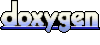