
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
GattAttribute.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_GATT_ATTRIBUTE_H__ 00018 #define MBED_GATT_ATTRIBUTE_H__ 00019 00020 #include "UUID.h " 00021 #include "BLETypes.h" 00022 00023 /** 00024 * @addtogroup ble 00025 * @{ 00026 * @addtogroup gatt 00027 * @{ 00028 * @addtogroup server 00029 * @{ 00030 */ 00031 00032 /** 00033 * Representation of a GattServer attribute. 00034 * 00035 * Attributes are the building block of GATT servers: services are attributes, 00036 * characteristics are groups of attributes and characteristic descriptors are 00037 * attributes, too. 00038 * 00039 * @par Typed values 00040 * 00041 * Attributes are typed values composed of a type and its associated value. The 00042 * attribute type identifies the attribute purpose. A UUID read by the client 00043 * during the discovery of the GATT server models the attribute type. The value of the 00044 * attribute is an array of bytes; its length may be fixed or variable. 00045 * 00046 * As an example, a primary service is declared by an attribute with the type 00047 * 0x2800, and the value of the attribute is the UUID of the service. 00048 * 00049 * @par Attribute Access 00050 * 00051 * The GATT server is an array of attributes in which a unique index identifies 00052 * each of the attributes within the array. That index is called the attribute 00053 * handle, and clients use it to access to attributes within the server. 00054 * 00055 * @note Attributes do not contain information related to their permissions, 00056 * grouping or semantic. Higher level specifications define these concepts. 00057 */ 00058 class GattAttribute { 00059 public: 00060 /** 00061 * Representation of an attribute handle. 00062 * 00063 * Each attribute in a GattServer has a unique handle that clients can use 00064 * to identify the attribute. The underlying BLE stack usually 00065 * generates and assigns handles to attributes. 00066 */ 00067 typedef ble::attribute_handle_t Handle_t; 00068 00069 /** 00070 * Invalid attribute handle. 00071 */ 00072 static const Handle_t INVALID_HANDLE = 0x0000; 00073 00074 public: 00075 /** 00076 * Construct an attribute. 00077 * 00078 * Application code uses attributes to model characteristic descriptors and 00079 * characteristics values. 00080 * 00081 * @param[in] uuid The type of the attribute. 00082 * @param[in] valuePtr Pointer to the memory buffer, which contains the 00083 * initial value of the attribute. The constructor does not make a copy of 00084 * the attribute buffer; as a consequence, the memory buffer must remain 00085 * valid during the lifetime of the attribute. 00086 * @param[in] len The length in bytes of this attribute's value. 00087 * @param[in] maxLen The length in bytes of the memory buffer containing the 00088 * attribute value. It must be greater than or equal to @p len. 00089 * @param[in] hasVariableLen Flag that indicates whether the attribute's value 00090 * length can change throughout time. 00091 * 00092 * @par Example 00093 * 00094 * @code 00095 * // declare a value of 2 bytes within a 10 bytes buffer 00096 * const uint8_t attribute_value[10] = { 10, 50 }; 00097 * GattAttribute attr = GattAttribute( 00098 * 0x2A19, // attribute type 00099 * attribute_value, 00100 * 2, // length of the current value 00101 * sizeof(attribute_value), // length of the buffer containing the value 00102 * true // variable length 00103 * ); 00104 * @endcode 00105 */ 00106 GattAttribute( 00107 const UUID &uuid, 00108 uint8_t *valuePtr = NULL, 00109 uint16_t len = 0, 00110 uint16_t maxLen = 0, 00111 bool hasVariableLen = true 00112 ) : _uuid(uuid), 00113 _valuePtr(valuePtr), 00114 _lenMax(maxLen), 00115 _len(len), 00116 _hasVariableLen(hasVariableLen), 00117 _handle() { 00118 } 00119 00120 public: 00121 /** 00122 * Get the attribute's handle in the ATT table. 00123 * 00124 * @note The GattServer sets the attribute's handle when services are 00125 * inserted. 00126 * 00127 * @return The attribute's handle. 00128 */ 00129 Handle_t getHandle(void) const 00130 { 00131 return _handle; 00132 } 00133 00134 /** 00135 * Get the UUID of the attribute. 00136 * 00137 * The UUID identifies the type of the attribute. 00138 * 00139 * @return The attribute. 00140 */ 00141 const UUID &getUUID(void) const 00142 { 00143 return _uuid; 00144 } 00145 00146 /** 00147 * Get the current length of the attribute value. 00148 * 00149 * @return The current length of the attribute value. 00150 */ 00151 uint16_t getLength(void) const 00152 { 00153 return _len; 00154 } 00155 00156 /** 00157 * Get the maximum length of the attribute value. 00158 * 00159 * The maximum length of the attribute value. 00160 */ 00161 uint16_t getMaxLength(void) const 00162 { 00163 return _lenMax; 00164 } 00165 00166 /** 00167 * Get a pointer to the current length of the attribute value. 00168 * 00169 * @important note Do not use this function. 00170 * 00171 * @return A pointer to the current length of the attribute value. 00172 */ 00173 uint16_t *getLengthPtr(void) 00174 { 00175 return &_len; 00176 } 00177 00178 /** 00179 * Set the attribute handle. 00180 * 00181 * @important The GattServer uses this function internally. 00182 * Application code must not use it. 00183 * 00184 * @param[in] id The new attribute handle. 00185 */ 00186 void setHandle(Handle_t id) 00187 { 00188 _handle = id; 00189 } 00190 00191 /** 00192 * Get a pointer to the attribute value. 00193 * 00194 * @return A pointer to the attribute value. 00195 */ 00196 uint8_t *getValuePtr(void) 00197 { 00198 return _valuePtr; 00199 } 00200 00201 /** 00202 * Check whether the length of the attribute's value can change throughout time. 00203 * 00204 * @return true if the attribute value has a variable length and false 00205 * otherwise. 00206 */ 00207 bool hasVariableLength(void) const 00208 { 00209 return _hasVariableLen; 00210 } 00211 00212 private: 00213 /** 00214 * Characteristic's UUID. 00215 */ 00216 UUID _uuid; 00217 00218 /** 00219 * Pointer to the attribute's value. 00220 */ 00221 uint8_t *_valuePtr; 00222 00223 /** 00224 * Length in byte of the buffer containing the attribute value. 00225 */ 00226 uint16_t _lenMax; 00227 00228 /** 00229 * Current length of the value pointed to by GattAttribute::_valuePtr. 00230 */ 00231 uint16_t _len; 00232 00233 /** 00234 * Whether the length of the value can change throughout time. 00235 */ 00236 bool _hasVariableLen; 00237 00238 /** 00239 * The attribute's handle in the ATT table. 00240 */ 00241 Handle_t _handle; 00242 00243 private: 00244 /* Disallow copy and assignment. */ 00245 GattAttribute(const GattAttribute &); 00246 GattAttribute& operator=(const GattAttribute &); 00247 }; 00248 00249 /** 00250 * @} 00251 * @} 00252 * @} 00253 */ 00254 00255 #endif /* ifndef MBED_GATT_ATTRIBUTE_H__ */
Generated on Tue Jul 12 2022 14:48:47 by
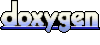