
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
Dir.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "Dir.h" 00018 #include "mbed.h" 00019 #include <errno.h> 00020 00021 00022 Dir::Dir() 00023 : _fs(0), _dir(0) 00024 { 00025 } 00026 00027 Dir::Dir(FileSystem *fs, const char *path) 00028 : _fs(0), _dir(0) 00029 { 00030 open(fs, path); 00031 } 00032 00033 Dir::~Dir() 00034 { 00035 if (_fs) { 00036 close(); 00037 } 00038 } 00039 00040 int Dir::open(FileSystem *fs, const char *path) 00041 { 00042 if (_fs) { 00043 return -EINVAL; 00044 } 00045 00046 int err = fs->dir_open(&_dir, path); 00047 if (!err) { 00048 _fs = fs; 00049 } 00050 00051 return err; 00052 } 00053 00054 int Dir::close() 00055 { 00056 if (!_fs) { 00057 return -EINVAL; 00058 } 00059 00060 int err = _fs->dir_close(_dir); 00061 _fs = 0; 00062 return err; 00063 } 00064 00065 ssize_t Dir::read(struct dirent *ent) 00066 { 00067 MBED_ASSERT(_fs); 00068 memset(ent, 0, sizeof(struct dirent)); 00069 return _fs->dir_read(_dir, ent); 00070 } 00071 00072 void Dir::seek(off_t offset) 00073 { 00074 MBED_ASSERT(_fs); 00075 return _fs->dir_seek(_dir, offset); 00076 } 00077 00078 off_t Dir::tell() 00079 { 00080 MBED_ASSERT(_fs); 00081 return _fs->dir_tell(_dir); 00082 } 00083 00084 void Dir::rewind() 00085 { 00086 MBED_ASSERT(_fs); 00087 return _fs->dir_rewind(_dir); 00088 } 00089 00090 size_t Dir::size() 00091 { 00092 MBED_ASSERT(_fs); 00093 return _fs->dir_size(_dir); 00094 }
Generated on Tue Jul 12 2022 14:48:42 by
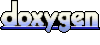