
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
BLEProtocol.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_BLE_PROTOCOL_H__ 00018 #define MBED_BLE_PROTOCOL_H__ 00019 00020 #include <stddef.h> 00021 #include <stdint.h> 00022 #include <algorithm> 00023 00024 /** 00025 * @addtogroup ble 00026 * @{ 00027 * @addtogroup common 00028 * @{ 00029 */ 00030 00031 /** 00032 * Common namespace for types and constants used everywhere in BLE API. 00033 */ 00034 namespace BLEProtocol { 00035 00036 /** 00037 * Container for the enumeration of BLE address types. 00038 * 00039 * @note Adding a struct to encapsulate the contained enumeration prevents 00040 * polluting the BLEProtocol namespace with the enumerated values. It also 00041 * allows type-aliases for the enumeration while retaining the enumerated 00042 * values. i.e. doing: 00043 * 00044 * @code 00045 * typedef AddressType AliasedType; 00046 * @endcode 00047 * 00048 * would allow the use of AliasedType::PUBLIC in code. 00049 * 00050 * @note see Bluetooth Standard version 4.2 [Vol 6, Part B] section 1.3 . 00051 */ 00052 struct AddressType { 00053 /** 00054 * Address-types for Protocol addresses. 00055 */ 00056 enum Type { 00057 /** 00058 * Public device address. 00059 */ 00060 PUBLIC = 0, 00061 00062 /** 00063 * Random static device address. 00064 */ 00065 RANDOM_STATIC, 00066 00067 /** 00068 * Private resolvable device address. 00069 */ 00070 RANDOM_PRIVATE_RESOLVABLE, 00071 00072 /** 00073 * Private non-resolvable device address. 00074 */ 00075 RANDOM_PRIVATE_NON_RESOLVABLE 00076 }; 00077 }; 00078 00079 /** 00080 * Alias for AddressType::Type 00081 */ 00082 typedef AddressType::Type AddressType_t; 00083 00084 /** 00085 * Length (in octets) of the BLE MAC address. 00086 */ 00087 static const size_t ADDR_LEN = 6; 00088 00089 /** 00090 * 48-bit address, in LSB format. 00091 */ 00092 typedef uint8_t AddressBytes_t[ADDR_LEN]; 00093 00094 /** 00095 * BLE address representation. 00096 * 00097 * It contains an address-type (::AddressType_t) and the address value 00098 * (::AddressBytes_t). 00099 */ 00100 struct Address_t { 00101 /** 00102 * Construct an Address_t object with the supplied type and address. 00103 * 00104 * @param[in] typeIn The BLE address type. 00105 * @param[in] addressIn The BLE address. 00106 * 00107 * @post type is equal to typeIn and address is equal to the content 00108 * present in addressIn. 00109 */ 00110 Address_t(AddressType_t typeIn, const AddressBytes_t &addressIn) : 00111 type(typeIn) { 00112 std::copy(addressIn, addressIn + ADDR_LEN, address); 00113 } 00114 00115 /** 00116 * Empty constructor. 00117 * 00118 * @note The address constructed with the empty constructor is not 00119 * valid. 00120 * 00121 * @post type is equal to PUBLIC and the address value is equal to 00122 * 00:00:00:00:00:00 00123 */ 00124 Address_t(void) : type(), address() { } 00125 00126 /** 00127 * Type of the BLE device address. 00128 */ 00129 AddressType_t type; 00130 00131 /** 00132 * Value of the device address. 00133 */ 00134 AddressBytes_t address; 00135 }; 00136 }; 00137 00138 /** 00139 * @} 00140 * @} 00141 */ 00142 00143 #endif /* MBED_BLE_PROTOCOL_H__ */
Generated on Tue Jul 12 2022 14:48:38 by
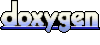