
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
AttClientToGattClientAdapter.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_PAL_ATTCLIENTTOGATTCLIENTADAPTER_H_ 00018 #define BLE_PAL_ATTCLIENTTOGATTCLIENTADAPTER_H_ 00019 00020 #include "AttClient.h" 00021 #include "PalGattClient.h" 00022 00023 namespace ble { 00024 namespace pal { 00025 00026 /** 00027 * Adapt a pal::AttClient into a pal::GattClient. 00028 * 00029 * This class let vendors define their abstraction layer in term of an AttClient 00030 * and adapt any AttClient into a GattClient. 00031 */ 00032 class AttClientToGattClientAdapter : public GattClient { 00033 00034 public: 00035 static const uint16_t END_ATTRIBUTE_HANDLE = 0xFFFF; 00036 static const uint16_t SERVICE_TYPE_UUID = 0x2800; 00037 static const uint16_t INCLUDE_TYPE_UUID = 0x2802; 00038 static const uint16_t CHARACTERISTIC_TYPE_UUID = 0x2803; 00039 00040 /** 00041 * Construct an instance of GattClient from an instance of AttClient. 00042 * @param client The client to adapt. 00043 */ 00044 AttClientToGattClientAdapter(AttClient& client) : 00045 GattClient(), _client(client) { 00046 _client.when_server_message_received( 00047 mbed::callback(this, &AttClientToGattClientAdapter::on_server_event) 00048 ); 00049 _client.when_transaction_timeout( 00050 mbed::callback( 00051 this, &AttClientToGattClientAdapter::on_transaction_timeout 00052 ) 00053 ); 00054 } 00055 00056 /** 00057 * @see ble::pal::GattClient::exchange_mtu 00058 */ 00059 virtual ble_error_t exchange_mtu (connection_handle_t connection) { 00060 return _client.exchange_mtu_request(connection); 00061 } 00062 00063 /** 00064 * @see ble::pal::GattClient::get_mtu_size 00065 */ 00066 virtual ble_error_t get_mtu_size ( 00067 connection_handle_t connection_handle, 00068 uint16_t& mtu_size 00069 ) { 00070 return _client.get_mtu_size(connection_handle, mtu_size); 00071 } 00072 00073 /** 00074 * @see ble::pal::GattClient::discover_primary_service 00075 */ 00076 virtual ble_error_t discover_primary_service ( 00077 connection_handle_t connection, 00078 attribute_handle_t discovery_range_begining 00079 ) { 00080 return _client.read_by_group_type_request( 00081 connection, 00082 attribute_handle_range(discovery_range_begining, END_ATTRIBUTE_HANDLE), 00083 SERVICE_TYPE_UUID 00084 ); 00085 } 00086 00087 /** 00088 * @see ble::pal::GattClient::discover_primary_service_by_service_uuid 00089 */ 00090 virtual ble_error_t discover_primary_service_by_service_uuid ( 00091 connection_handle_t connection_handle, 00092 attribute_handle_t discovery_range_begining, 00093 const UUID& uuid 00094 ) { 00095 return _client.find_by_type_value_request( 00096 connection_handle, 00097 attribute_handle_range(discovery_range_begining, END_ATTRIBUTE_HANDLE), 00098 SERVICE_TYPE_UUID, 00099 ArrayView<const uint8_t> ( 00100 uuid.getBaseUUID(), 00101 (uuid.shortOrLong() == UUID::UUID_TYPE_SHORT) ? 2 : UUID::LENGTH_OF_LONG_UUID 00102 ) 00103 ); 00104 } 00105 00106 /** 00107 * @see ble::pal::GattClient::find_included_service 00108 */ 00109 virtual ble_error_t find_included_service ( 00110 connection_handle_t connection_handle, 00111 attribute_handle_range_t service_range 00112 ) { 00113 return _client.read_by_type_request( 00114 connection_handle, 00115 service_range, 00116 INCLUDE_TYPE_UUID 00117 ); 00118 } 00119 00120 /** 00121 * @see ble::pal::GattClient::discover_characteristics_of_a_service 00122 */ 00123 virtual ble_error_t discover_characteristics_of_a_service ( 00124 connection_handle_t connection_handle, 00125 attribute_handle_range_t discovery_range 00126 ) { 00127 return _client.read_by_type_request( 00128 connection_handle, 00129 discovery_range, 00130 CHARACTERISTIC_TYPE_UUID 00131 ); 00132 } 00133 00134 /** 00135 * @see ble::pal::GattClient::discover_characteristics_descriptors 00136 */ 00137 virtual ble_error_t discover_characteristics_descriptors ( 00138 connection_handle_t connection_handle, 00139 attribute_handle_range_t descriptors_discovery_range 00140 ) { 00141 return _client.find_information_request( 00142 connection_handle, 00143 descriptors_discovery_range 00144 ); 00145 } 00146 00147 /** 00148 * @see ble::pal::GattClient::read_attribute_value 00149 */ 00150 virtual ble_error_t read_attribute_value ( 00151 connection_handle_t connection_handle, 00152 attribute_handle_t attribute_handle 00153 ) { 00154 return _client.read_request( 00155 connection_handle, 00156 attribute_handle 00157 ); 00158 } 00159 00160 /** 00161 * @see ble::pal::GattClient::read_using_characteristic_uuid 00162 */ 00163 virtual ble_error_t read_using_characteristic_uuid ( 00164 connection_handle_t connection_handle, 00165 attribute_handle_range_t read_range, 00166 const UUID& uuid 00167 ) { 00168 return _client.read_by_type_request( 00169 connection_handle, 00170 read_range, 00171 uuid 00172 ); 00173 } 00174 00175 /** 00176 * @see ble::pal::GattClient::read_attribute_blob 00177 */ 00178 virtual ble_error_t read_attribute_blob ( 00179 connection_handle_t connection_handle, 00180 attribute_handle_t attribute_handle, 00181 uint16_t offset 00182 ) { 00183 return _client.read_blob_request( 00184 connection_handle, 00185 attribute_handle, 00186 offset 00187 ); 00188 } 00189 00190 /** 00191 * @see ble::pal::GattClient::read_multiple_characteristic_values 00192 */ 00193 virtual ble_error_t read_multiple_characteristic_values ( 00194 connection_handle_t connection_handle, 00195 const ArrayView<const attribute_handle_t>& characteristic_value_handles 00196 ) { 00197 return _client.read_multiple_request( 00198 connection_handle, 00199 characteristic_value_handles 00200 ); 00201 } 00202 00203 /** 00204 * @see ble::pal::GattClient::write_without_response 00205 */ 00206 virtual ble_error_t write_without_response ( 00207 connection_handle_t connection_handle, 00208 attribute_handle_t characteristic_value_handle, 00209 const ArrayView<const uint8_t> & value 00210 ) { 00211 return _client.write_command( 00212 connection_handle, 00213 characteristic_value_handle, 00214 value 00215 ); 00216 } 00217 00218 /** 00219 * @see ble::pal::GattClient::signed_write_without_response 00220 */ 00221 virtual ble_error_t signed_write_without_response ( 00222 connection_handle_t connection_handle, 00223 attribute_handle_t characteristic_value_handle, 00224 const ArrayView<const uint8_t> & value 00225 ) { 00226 return _client.signed_write_command( 00227 connection_handle, 00228 characteristic_value_handle, 00229 value 00230 ); 00231 } 00232 00233 /** 00234 * @see ble::pal::GattClient::write_attribute 00235 */ 00236 virtual ble_error_t write_attribute ( 00237 connection_handle_t connection_handle, 00238 attribute_handle_t attribute_handle, 00239 const ArrayView<const uint8_t> & value 00240 ) { 00241 return _client.write_request( 00242 connection_handle, 00243 attribute_handle, 00244 value 00245 ); 00246 } 00247 00248 /** 00249 * @see ble::pal::GattClient::queue_prepare_write 00250 */ 00251 virtual ble_error_t queue_prepare_write ( 00252 connection_handle_t connection_handle, 00253 attribute_handle_t characteristic_value_handle, 00254 const ArrayView<const uint8_t> & value, 00255 uint16_t offset 00256 ) { 00257 return _client.prepare_write_request( 00258 connection_handle, 00259 characteristic_value_handle, 00260 offset, 00261 value 00262 ); 00263 } 00264 00265 /** 00266 * @see ble::pal::GattClient::execute_write_queue 00267 */ 00268 virtual ble_error_t execute_write_queue ( 00269 connection_handle_t connection_handle, 00270 bool execute 00271 ) { 00272 return _client.execute_write_request(connection_handle, execute); 00273 } 00274 00275 /** 00276 * @see ble::pal::GattClient::initialize 00277 */ 00278 virtual ble_error_t initialize () { 00279 return _client.initialize(); 00280 } 00281 00282 /** 00283 * @see ble::pal::GattClient::terminate 00284 */ 00285 virtual ble_error_t terminate () { 00286 return _client.initialize(); 00287 } 00288 00289 private: 00290 AttClient& _client; 00291 }; 00292 00293 } // namespace pal 00294 } // namespace ble 00295 00296 00297 #endif /* BLE_PAL_ATTCLIENTTOGATTCLIENTADAPTER_H_ */
Generated on Tue Jul 12 2022 14:48:38 by
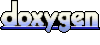