
STM32F7 Ethernet interface for nucleo STM32F767
Embed:
(wiki syntax)
Show/hide line numbers
ATCmdParser.h
00001 /* Copyright (c) 2017 ARM Limited 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 * 00015 * @section DESCRIPTION 00016 * 00017 * Parser for the AT command syntax 00018 * 00019 */ 00020 #ifndef MBED_ATCMDPARSER_H 00021 #define MBED_ATCMDPARSER_H 00022 00023 #include "mbed.h" 00024 #include <cstdarg> 00025 #include "Callback.h" 00026 00027 namespace mbed { 00028 00029 /** \addtogroup platform */ 00030 /** @{*/ 00031 /** 00032 * \defgroup platform_ATCmdParser ATCmdParser class 00033 * @{ 00034 */ 00035 00036 /** 00037 * Parser class for parsing AT commands 00038 * 00039 * Here are some examples: 00040 * @code 00041 * UARTSerial serial = UARTSerial(D1, D0); 00042 * ATCmdParser at = ATCmdParser(&serial, "\r\n"); 00043 * int value; 00044 * char buffer[100]; 00045 * 00046 * at.send("AT") && at.recv("OK"); 00047 * at.send("AT+CWMODE=%d", 3) && at.recv("OK"); 00048 * at.send("AT+CWMODE?") && at.recv("+CWMODE:%d\r\nOK", &value); 00049 * at.recv("+IPD,%d:", &value); 00050 * at.read(buffer, value); 00051 * at.recv("OK"); 00052 * @endcode 00053 */ 00054 00055 class ATCmdParser : private NonCopyable<ATCmdParser> 00056 { 00057 private: 00058 // File handle 00059 // Not owned by ATCmdParser 00060 FileHandle *_fh; 00061 00062 int _buffer_size; 00063 char *_buffer; 00064 int _timeout; 00065 00066 // Parsing information 00067 const char *_output_delimiter; 00068 int _output_delim_size; 00069 char _in_prev; 00070 bool _dbg_on; 00071 bool _aborted; 00072 00073 struct oob { 00074 unsigned len; 00075 const char *prefix; 00076 mbed::Callback<void()> cb; 00077 oob *next; 00078 }; 00079 oob *_oobs; 00080 00081 public: 00082 00083 /** 00084 * Constructor 00085 * 00086 * @param fh A FileHandle to a digital interface to use for AT commands 00087 * @param output_delimiter end of command line termination 00088 * @param buffer_size size of internal buffer for transaction 00089 * @param timeout timeout of the connection 00090 * @param debug turns on/off debug output for AT commands 00091 */ 00092 ATCmdParser(FileHandle *fh, const char *output_delimiter = "\r", 00093 int buffer_size = 256, int timeout = 8000, bool debug = false) 00094 : _fh(fh), _buffer_size(buffer_size), _in_prev(0), _oobs(NULL) 00095 { 00096 _buffer = new char[buffer_size]; 00097 set_timeout(timeout); 00098 set_delimiter(output_delimiter); 00099 debug_on(debug); 00100 } 00101 00102 /** 00103 * Destructor 00104 */ 00105 ~ATCmdParser() 00106 { 00107 while (_oobs) { 00108 struct oob *oob = _oobs; 00109 _oobs = oob->next; 00110 delete oob; 00111 } 00112 delete[] _buffer; 00113 } 00114 00115 /** 00116 * Allows timeout to be changed between commands 00117 * 00118 * @param timeout timeout of the connection 00119 */ 00120 void set_timeout(int timeout) 00121 { 00122 _timeout = timeout; 00123 } 00124 00125 /** 00126 * For backwards compatibility. 00127 * 00128 * Please use set_timeout(int) API only from now on. 00129 * Allows timeout to be changed between commands 00130 * 00131 * @param timeout timeout of the connection 00132 */ 00133 MBED_DEPRECATED_SINCE("mbed-os-5.5.0", "Replaced with set_timeout for consistency") 00134 void setTimeout(int timeout) 00135 { 00136 set_timeout(timeout); 00137 } 00138 00139 /** 00140 * Sets string of characters to use as line delimiters 00141 * 00142 * @param output_delimiter string of characters to use as line delimiters 00143 */ 00144 void set_delimiter(const char *output_delimiter) 00145 { 00146 _output_delimiter = output_delimiter; 00147 _output_delim_size = strlen(output_delimiter); 00148 } 00149 00150 /** 00151 * For backwards compatibility. 00152 * 00153 * Please use set_delimiter(const char *) API only from now on. 00154 * Sets string of characters to use as line delimiters 00155 * 00156 * @param output_delimiter string of characters to use as line delimiters 00157 */ 00158 MBED_DEPRECATED_SINCE("mbed-os-5.5.0", "Replaced with set_delimiter for consistency") 00159 void setDelimiter(const char *output_delimiter) 00160 { 00161 set_delimiter(output_delimiter); 00162 } 00163 00164 /** 00165 * Allows traces from modem to be turned on or off 00166 * 00167 * @param on set as 1 to turn on traces and vice versa. 00168 */ 00169 void debug_on(uint8_t on) 00170 { 00171 _dbg_on = (on) ? 1 : 0; 00172 } 00173 00174 /** 00175 * For backwards compatibility. 00176 * 00177 * Allows traces from modem to be turned on or off 00178 * 00179 * @param on set as 1 to turn on traces and vice versa. 00180 */ 00181 MBED_DEPRECATED_SINCE("mbed-os-5.5.0", "Replaced with debug_on for consistency") 00182 void debugOn(uint8_t on) 00183 { 00184 debug_on(on); 00185 } 00186 00187 /** 00188 * Sends an AT command 00189 * 00190 * Sends a formatted command using printf style formatting 00191 * @see printf 00192 * 00193 * @param command printf-like format string of command to send which 00194 * is appended with a newline 00195 * @param ... all printf-like arguments to insert into command 00196 * @return true only if command is successfully sent 00197 */ 00198 bool send(const char *command, ...) MBED_PRINTF_METHOD(1,2); 00199 00200 bool vsend(const char *command, va_list args); 00201 00202 /** 00203 * Receive an AT response 00204 * 00205 * Receives a formatted response using scanf style formatting 00206 * @see scanf 00207 * 00208 * Responses are parsed line at a time. 00209 * Any received data that does not match the response is ignored until 00210 * a timeout occurs. 00211 * 00212 * @param response scanf-like format string of response to expect 00213 * @param ... all scanf-like arguments to extract from response 00214 * @return true only if response is successfully matched 00215 */ 00216 bool recv(const char *response, ...) MBED_SCANF_METHOD(1,2); 00217 00218 bool vrecv(const char *response, va_list args); 00219 00220 /** 00221 * Write a single byte to the underlying stream 00222 * 00223 * @param c The byte to write 00224 * @return The byte that was written or -1 during a timeout 00225 */ 00226 int putc(char c); 00227 00228 /** 00229 * Get a single byte from the underlying stream 00230 * 00231 * @return The byte that was read or -1 during a timeout 00232 */ 00233 int getc(); 00234 00235 /** 00236 * Write an array of bytes to the underlying stream 00237 * 00238 * @param data the array of bytes to write 00239 * @param size number of bytes to write 00240 * @return number of bytes written or -1 on failure 00241 */ 00242 int write(const char *data, int size); 00243 00244 /** 00245 * Read an array of bytes from the underlying stream 00246 * 00247 * @param data the destination for the read bytes 00248 * @param size number of bytes to read 00249 * @return number of bytes read or -1 on failure 00250 */ 00251 int read(char *data, int size); 00252 00253 /** 00254 * Direct printf to underlying stream 00255 * @see printf 00256 * 00257 * @param format format string to pass to printf 00258 * @param ... arguments to printf 00259 * @return number of bytes written or -1 on failure 00260 */ 00261 int printf(const char *format, ...) MBED_PRINTF_METHOD(1,2); 00262 00263 int vprintf(const char *format, va_list args); 00264 00265 /** 00266 * Direct scanf on underlying stream 00267 * @see scanf 00268 * 00269 * @param format format string to pass to scanf 00270 * @param ... arguments to scanf 00271 * @return number of bytes read or -1 on failure 00272 */ 00273 int scanf(const char *format, ...) MBED_SCANF_METHOD(1,2); 00274 00275 int vscanf(const char *format, va_list args); 00276 00277 /** 00278 * Attach a callback for out-of-band data 00279 * 00280 * @param prefix string on when to initiate callback 00281 * @param func callback to call when string is read 00282 * @note out-of-band data is only processed during a scanf call 00283 */ 00284 void oob(const char *prefix, mbed::Callback<void()> func); 00285 00286 /** 00287 * Flushes the underlying stream 00288 */ 00289 void flush(); 00290 00291 /** 00292 * Abort current recv 00293 * 00294 * Can be called from oob handler to interrupt the current 00295 * recv operation. 00296 */ 00297 void abort(); 00298 00299 /** 00300 * Process out-of-band data 00301 * 00302 * Process out-of-band data in the receive buffer. This function 00303 * returns immediately if there is no data to process. 00304 * 00305 * @return true if oob data processed, false otherwise 00306 */ 00307 bool process_oob(void); 00308 }; 00309 00310 /**@}*/ 00311 00312 /**@}*/ 00313 00314 } //namespace mbed 00315 00316 #endif //MBED_ATCMDPARSER_H
Generated on Tue Jul 12 2022 14:48:38 by
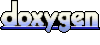