Ethernet for Nucleo and Disco board STM32F746 works with gcc and arm. IAC is untested
Fork of F7_Ethernet by
Network_defines_lwip.h
00001 //////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00002 // Copyright (c) Microsoft Corporation. All rights reserved. 00003 //////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00004 00005 #ifndef _DRIVERS_NETWORK_DEFINES_LWIP_H_ 00006 #define _DRIVERS_NETWORK_DEFINES_LWIP_H_ 1 00007 00008 /* Pick min, default or max configuration based on platform */ 00009 #include <lwip_selector.h> 00010 00011 #if !defined(NETWORK_MEMORY_PROFILE_LWIP__small) && !defined(NETWORK_MEMORY_PROFILE_LWIP__medium) && !defined(NETWORK_MEMORY_PROFILE_LWIP__large) && !defined(NETWORK_MEMORY_PROFILE_LWIP__custom) 00012 #error You must define a NETWORK_MEMORY_PROFILE_LWIP_xxx for this platform 00013 #endif 00014 00015 #define TCPIP_LWIP 1 00016 00017 /* min, default, max configuration for lwIP. Values have initially 00018 been taken from lwiopts.small.h, lwipopts.h and lwipopts.big.h */ 00019 00020 /* MEM_SIZE: the size of the heap memory. If the application will send 00021 a lot of data that needs to be copied, this should be set high. */ 00022 #define MEM_SIZE__min (16*1024) 00023 #define MEM_SIZE__default (64*1024) 00024 #define MEM_SIZE__max (1024*1024) // TODO - this seems a bit extreme 00025 00026 /* MEMP_NUM_PBUF: the number of memp struct pbufs. If the application 00027 sends a lot of data out of ROM (or other static memory), this 00028 should be set high. */ 00029 #define MEMP_NUM_PBUF__min 16 00030 #define MEMP_NUM_PBUF__default 32 00031 #define MEMP_NUM_PBUF__max 32 00032 00033 /* MEMP_NUM_UDP_PCB: the number of UDP protocol control blocks. One 00034 per active UDP "connection". */ 00035 #define MEMP_NUM_UDP_PCB__min 6 00036 #define MEMP_NUM_UDP_PCB__default 8 00037 #define MEMP_NUM_UDP_PCB__max 16 00038 00039 /* MEMP_NUM_TCP_PCB: the number of simulatenously active TCP 00040 connections. */ 00041 #define MEMP_NUM_TCP_PCB__min 8 00042 #define MEMP_NUM_TCP_PCB__default 16 00043 #define MEMP_NUM_TCP_PCB__max 32 00044 00045 /* MEMP_NUM_TCP_PCB_LISTEN: the number of listening TCP 00046 connections. */ 00047 #define MEMP_NUM_TCP_PCB_LISTEN__min 4 00048 #define MEMP_NUM_TCP_PCB_LISTEN__default 8 00049 #define MEMP_NUM_TCP_PCB_LISTEN__max 12 00050 00051 /* MEMP_NUM_TCP_SEG: the number of simultaneously queued TCP 00052 segments. */ 00053 #define MEMP_NUM_TCP_SEG__min 32 00054 #define MEMP_NUM_TCP_SEG__default 64 00055 #define MEMP_NUM_TCP_SEG__max 128 00056 00057 /* MEMP_NUM_SYS_TIMEOUT: the number of simulateously active 00058 timeouts. */ 00059 #define MEMP_NUM_SYS_TIMEOUT__min 8 // set to 3 in lwiopts.small.h but didn't compile 00060 #define MEMP_NUM_SYS_TIMEOUT__default 12 00061 #define MEMP_NUM_SYS_TIMEOUT__max 16 00062 00063 /* MEMP_NUM_NETBUF: the number of struct netbufs. */ 00064 #define MEMP_NUM_NETBUF__min 8 00065 #define MEMP_NUM_NETBUF__default 16 00066 #define MEMP_NUM_NETBUF__max 32 00067 00068 /* MEMP_NUM_NETCONN: the number of struct netconns. */ 00069 #define MEMP_NUM_NETCONN__min 10 00070 #define MEMP_NUM_NETCONN__default 20 00071 #define MEMP_NUM_NETCONN__max 40 00072 00073 /* PBUF_POOL_SIZE: the number of buffers in the pbuf pool. */ 00074 #define PBUF_POOL_SIZE__min 40 00075 #define PBUF_POOL_SIZE__default 128 00076 #define PBUF_POOL_SIZE__max 256 00077 00078 /* PBUF_POOL_BUFSIZE: the size of each pbuf in the pbuf pool. */ 00079 #define PBUF_POOL_BUFSIZE__min 512 00080 #define PBUF_POOL_BUFSIZE__default 1024 00081 #define PBUF_POOL_BUFSIZE__max 2048 00082 00083 /* TCP Maximum segment size. */ 00084 #define TCP_MSS__min 536 00085 #define TCP_MSS__default 1460 00086 #define TCP_MSS__max 1460 00087 00088 /* TCP sender buffer space (bytes). */ 00089 #define TCP_SND_BUF__min (2*TCP_MSS) 00090 #define TCP_SND_BUF__default (4*TCP_MSS) 00091 #define TCP_SND_BUF__max (8*TCP_MSS) 00092 00093 /* TCP sender buffer space (pbufs). This must be at least = 2 * 00094 TCP_SND_BUF/TCP_MSS for things to work. */ 00095 #define TCP_SND_QUEUELEN__min ((2*TCP_SND_BUF)/TCP_MSS) 00096 #define TCP_SND_QUEUELEN__default ((4*TCP_SND_BUF)/TCP_MSS) 00097 #define TCP_SND_QUEUELEN__max ((8*TCP_SND_BUF)/TCP_MSS) 00098 00099 /* TCP receive window. */ 00100 #define TCP_WND__min (2*1024) 00101 #define TCP_WND__default (8*1024) 00102 #define TCP_WND__max (32*1024) 00103 00104 /* TCP writable space (bytes). This must be less than or equal 00105 to TCP_SND_BUF. It is the amount of space which must be 00106 available in the tcp snd_buf for select to return writable */ 00107 #define TCP_SNDLOWAT__min (TCP_SND_BUF/2) 00108 #define TCP_SNDLOWAT__default (TCP_SND_BUF/2) 00109 #define TCP_SNDLOWAT__max (TCP_SND_BUF/32) 00110 00111 00112 //--// RAM Profiles 00113 00114 #ifdef NETWORK_MEMORY_PROFILE_LWIP__small 00115 #define PLATFORM_DEPENDENT__MEM_SIZE MEM_SIZE__min 00116 #define PLATFORM_DEPENDENT__MEMP_NUM_PBUF MEMP_NUM_PBUF__min 00117 #define PLATFORM_DEPENDENT__MEMP_NUM_UDP_PCB MEMP_NUM_UDP_PCB__min 00118 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB MEMP_NUM_TCP_PCB__min 00119 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB_LISTEN MEMP_NUM_TCP_PCB_LISTEN__min 00120 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_SEG MEMP_NUM_TCP_SEG__min 00121 #define PLATFORM_DEPENDENT__MEMP_NUM_SYS_TIMEOUT MEMP_NUM_SYS_TIMEOUT__min 00122 #define PLATFORM_DEPENDENT__MEMP_NUM_NETBUF MEMP_NUM_NETBUF__min 00123 #define PLATFORM_DEPENDENT__MEMP_NUM_NETCONN MEMP_NUM_NETCONN__min 00124 #define PLATFORM_DEPENDENT__PBUF_POOL_SIZE PBUF_POOL_SIZE__min 00125 #define PLATFORM_DEPENDENT__PBUF_POOL_BUFSIZE PBUF_POOL_BUFSIZE__min 00126 #define PLATFORM_DEPENDENT__TCP_MSS TCP_MSS__min 00127 #define PLATFORM_DEPENDENT__TCP_SND_BUF TCP_SND_BUF__min 00128 #define PLATFORM_DEPENDENT__TCP_SND_QUEUELEN TCP_SND_QUEUELEN__min 00129 #define PLATFORM_DEPENDENT__TCP_WND TCP_WND__min 00130 #define PLATFORM_DEPENDENT__TCP_SNDLOWAT TCP_SNDLOWAT__min 00131 #endif 00132 00133 #ifdef NETWORK_MEMORY_PROFILE_LWIP__medium 00134 #define PLATFORM_DEPENDENT__MEM_SIZE MEM_SIZE__default 00135 #define PLATFORM_DEPENDENT__MEMP_NUM_PBUF MEMP_NUM_PBUF__default 00136 #define PLATFORM_DEPENDENT__MEMP_NUM_UDP_PCB MEMP_NUM_UDP_PCB__default 00137 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB MEMP_NUM_TCP_PCB__default 00138 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB_LISTEN MEMP_NUM_TCP_PCB_LISTEN__default 00139 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_SEG MEMP_NUM_TCP_SEG__default 00140 #define PLATFORM_DEPENDENT__MEMP_NUM_SYS_TIMEOUT MEMP_NUM_SYS_TIMEOUT__default 00141 #define PLATFORM_DEPENDENT__MEMP_NUM_NETBUF MEMP_NUM_NETBUF__default 00142 #define PLATFORM_DEPENDENT__MEMP_NUM_NETCONN MEMP_NUM_NETCONN__default 00143 #define PLATFORM_DEPENDENT__PBUF_POOL_SIZE PBUF_POOL_SIZE__default 00144 #define PLATFORM_DEPENDENT__PBUF_POOL_BUFSIZE PBUF_POOL_BUFSIZE__default 00145 #define PLATFORM_DEPENDENT__TCP_MSS TCP_MSS__default 00146 #define PLATFORM_DEPENDENT__TCP_SND_BUF TCP_SND_BUF__default 00147 #define PLATFORM_DEPENDENT__TCP_SND_QUEUELEN TCP_SND_QUEUELEN__default 00148 #define PLATFORM_DEPENDENT__TCP_WND TCP_WND__default 00149 #define PLATFORM_DEPENDENT__TCP_SNDLOWAT TCP_SNDLOWAT__default 00150 #endif 00151 00152 #ifdef NETWORK_MEMORY_PROFILE_LWIP__large 00153 #define PLATFORM_DEPENDENT__MEM_SIZE MEM_SIZE__max 00154 #define PLATFORM_DEPENDENT__MEMP_NUM_PBUF MEMP_NUM_PBUF__max 00155 #define PLATFORM_DEPENDENT__MEMP_NUM_UDP_PCB MEMP_NUM_UDP_PCB__max 00156 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB MEMP_NUM_TCP_PCB__max 00157 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB_LISTEN MEMP_NUM_TCP_PCB_LISTEN__max 00158 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_SEG MEMP_NUM_TCP_SEG__max 00159 #define PLATFORM_DEPENDENT__MEMP_NUM_SYS_TIMEOUT MEMP_NUM_SYS_TIMEOUT__max 00160 #define PLATFORM_DEPENDENT__MEMP_NUM_NETBUF MEMP_NUM_NETBUF__max 00161 #define PLATFORM_DEPENDENT__MEMP_NUM_NETCONN MEMP_NUM_NETCONN__max 00162 #define PLATFORM_DEPENDENT__PBUF_POOL_SIZE PBUF_POOL_SIZE__max 00163 #define PLATFORM_DEPENDENT__PBUF_POOL_BUFSIZE PBUF_POOL_BUFSIZE__max 00164 #define PLATFORM_DEPENDENT__TCP_MSS TCP_MSS__max 00165 #define PLATFORM_DEPENDENT__TCP_SND_BUF TCP_SND_BUF__max 00166 #define PLATFORM_DEPENDENT__TCP_SND_QUEUELEN TCP_SND_QUEUELEN__max 00167 #define PLATFORM_DEPENDENT__TCP_WND TCP_WND__max 00168 #define PLATFORM_DEPENDENT__TCP_SNDLOWAT TCP_SNDLOWAT__max 00169 #endif 00170 00171 #ifdef NETWORK_MEMORY_PROFILE_LWIP__custom 00172 #define PLATFORM_DEPENDENT__MEM_SIZE MEM_SIZE__custom 00173 #define PLATFORM_DEPENDENT__MEMP_NUM_PBUF MEMP_NUM_PBUF__custom 00174 #define PLATFORM_DEPENDENT__MEMP_NUM_UDP_PCB MEMP_NUM_UDP_PCB__custom 00175 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB MEMP_NUM_TCP_PCB__custom 00176 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB_LISTEN MEMP_NUM_TCP_PCB_LISTEN__custom 00177 #define PLATFORM_DEPENDENT__MEMP_NUM_TCP_SEG MEMP_NUM_TCP_SEG__custom 00178 #define PLATFORM_DEPENDENT__MEMP_NUM_SYS_TIMEOUT MEMP_NUM_SYS_TIMEOUT__custom 00179 #define PLATFORM_DEPENDENT__MEMP_NUM_NETBUF MEMP_NUM_NETBUF__custom 00180 #define PLATFORM_DEPENDENT__MEMP_NUM_NETCONN MEMP_NUM_NETCONN__custom 00181 #define PLATFORM_DEPENDENT__PBUF_POOL_SIZE PBUF_POOL_SIZE__custom 00182 #define PLATFORM_DEPENDENT__PBUF_POOL_BUFSIZE PBUF_POOL_BUFSIZE__custom 00183 #define PLATFORM_DEPENDENT__TCP_MSS TCP_MSS__custom 00184 #define PLATFORM_DEPENDENT__TCP_SND_BUF TCP_SND_BUF__custom 00185 #define PLATFORM_DEPENDENT__TCP_SND_QUEUELEN TCP_SND_QUEUELEN__custom 00186 #define PLATFORM_DEPENDENT__TCP_WND TCP_WND__custom 00187 #define PLATFORM_DEPENDENT__TCP_SNDLOWAT TCP_SNDLOWAT__custom 00188 #endif 00189 00190 #define PLATFORM_DEPENDENT__SOCKETS_MAX_COUNT (PLATFORM_DEPENDENT__MEMP_NUM_UDP_PCB + PLATFORM_DEPENDENT__MEMP_NUM_TCP_PCB) 00191 00192 #endif // _DRIVERS_NETWORK_DEFINES_LWIP_H_ 00193
Generated on Tue Jul 12 2022 21:19:02 by
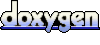