A library for Silicon Sensing DMU02
Embed:
(wiki syntax)
Show/hide line numbers
DMU.h
00001 #ifndef DMU_H 00002 #define DMU_H 00003 00004 00005 #include "mbed.h" 00006 /** 00007 * A DMU interface for communicating with the Silicon Sensing DMU02 Dynamics Measurement Unit 00008 * 00009 */ 00010 class DMU { 00011 public: 00012 00013 00014 /** Creates a DMU interface for using regular mbed pins 00015 * 00016 * @param MOSI SPI MOSI line 00017 * @param MISO SPI MISO line 00018 * @param SCLK SPI SCLK line 00019 * @param S0-S2 Select lines for X, Y and Z axi 00020 * 00021 */ 00022 DMU(PinName MOSI, PinName MISO, PinName SCLK, PinName S0, PinName S1, PinName S2); 00023 00024 00025 /** Return the acceleration in the direction of the selected axis 00026 * 00027 * @param axis The axis you wish to query: x, y or z. 00028 */ 00029 float acceleration(char axis); 00030 00031 00032 /** Return the roll 00033 * 00034 */ 00035 float roll(); 00036 00037 00038 /** Return the pitch 00039 * 00040 */ 00041 float pitch(); 00042 00043 /** Return the yaw 00044 * 00045 */ 00046 float yaw(); 00047 00048 00049 /** forces the device to perform the built-in test 00050 * 00051 */ 00052 void test(); 00053 00054 00055 protected: 00056 00057 //Device Initialization 00058 void _init(); 00059 00060 //used to set selected axis for query, active low 00061 void _selectAxis(char axis); 00062 00063 //deselects all axis, inactive high 00064 void _deselectAxis(); 00065 00066 //writes control byte to device, populates data bytes, returns bool of if data was received correctly 00067 bool _write(char controlByte); 00068 00069 bool _correctChecksumReceived(char statusByte, char chkSum); 00070 00071 00072 // Regular mbed pins to drive chip, a SPI bus and S0-S2 which function as slave selects, active low 00073 SPI *_spi; 00074 BusOut *_cs; 00075 00076 // Received data bytes 00077 char data0; 00078 char data1; 00079 char data2; 00080 char data3; 00081 00082 00083 // Timer used to ensure only 10 Hz total reads 00084 Timer _repClock; 00085 }; 00086 00087 #endif
Generated on Tue Jul 12 2022 22:29:20 by
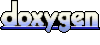