Library for the HMC5883L with 5 pins
Fork of QMC5883L by
Embed:
(wiki syntax)
Show/hide line numbers
QMC5883L.cpp
00001 /* QMC5883L Digital Compass Library 00002 * 00003 * @author: Baser Kandehir 00004 * @date: August 5, 2015 00005 * @license: MIT license 00006 * 00007 * Copyright (c) 2015, Baser Kandehir, baser.kandehir@ieee.metu.edu.tr 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining a copy 00010 * of this software and associated documentation files (the "Software"), to deal 00011 * in the Software without restriction, including without limitation the rights 00012 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00013 * copies of the Software, and to permit persons to whom the Software is 00014 * furnished to do so, subject to the following conditions: 00015 * 00016 * The above copyright notice and this permission notice shall be included in 00017 * all copies or substantial portions of the Software. 00018 * 00019 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00020 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00021 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00022 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00023 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00024 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00025 * THE SOFTWARE. 00026 * 00027 */ 00028 00029 // Some part of the code is adapted from Adafruit HMC5883 library 00030 00031 #include "QMC5883L.h" 00032 00033 QMC5883L::QMC5883L(PinName sda, PinName scl): QMC5883L_i2c(sda, scl) 00034 { 00035 } 00036 00037 float QMC5883L::setMagRange(MagScale Mscale) 00038 { 00039 float mRes; // Varies with gain 00040 00041 switch(Mscale) 00042 { 00043 case MagScale_2G: 00044 mRes = 1.0/12000; //LSB/G 00045 break; 00046 case MagScale_8G: 00047 mRes = 1.0/3000; 00048 break; 00049 } 00050 return mRes; 00051 } 00052 00053 void QMC5883L::QMC5883L_WriteByte(uint8_t QMC5883L_reg, uint8_t QMC5883L_data) 00054 { 00055 char data_out[2]; 00056 data_out[0]=QMC5883L_reg; 00057 data_out[1]=QMC5883L_data; 00058 this->QMC5883L_i2c.write(QMC5883L_ADDRESS, data_out, 2, 0); 00059 } 00060 00061 uint8_t QMC5883L::QMC5883L_ReadByte(uint8_t QMC5883L_reg) 00062 { 00063 char data_out[1], data_in[1]; 00064 data_out[0] = QMC5883L_reg; 00065 this->QMC5883L_i2c.write(QMC5883L_ADDRESS, data_out, 1, 1); 00066 this->QMC5883L_i2c.read(QMC5883L_ADDRESS, data_in, 1, 0); 00067 return (data_in[0]); 00068 } 00069 00070 void QMC5883L::ChipID() 00071 { 00072 uint8_t ChipID = QMC5883L_ReadByte(CHIP_ID); // Should return 0x68 00073 } 00074 00075 void QMC5883L::init() 00076 { 00077 setMagRange(MagScale_8G); 00078 QMC5883L_WriteByte(CONTROL_A, 0x0D | MagScale_8G); // Range: 8G, ODR: 200 Hz, mode:Continuous-Measurement 00079 QMC5883L_WriteByte(SET_RESET, 0x01); 00080 wait_ms(10); 00081 } 00082 00083 int16_t QMC5883L::getMagXvalue() 00084 { 00085 uint8_t LoByte, HiByte; 00086 LoByte = QMC5883L_ReadByte(OUT_X_LSB); // read Accelerometer X_Low value 00087 HiByte = QMC5883L_ReadByte(OUT_X_MSB); // read Accelerometer X_High value 00088 return((HiByte<<8) | LoByte); 00089 } 00090 00091 int16_t QMC5883L::getMagYvalue() 00092 { 00093 uint8_t LoByte, HiByte; 00094 LoByte = QMC5883L_ReadByte(OUT_Y_LSB); // read Accelerometer X_Low value 00095 HiByte = QMC5883L_ReadByte(OUT_Y_MSB); // read Accelerometer X_High value 00096 return ((HiByte<<8) | LoByte); 00097 } 00098 00099 int16_t QMC5883L::getMagZvalue() 00100 { 00101 uint8_t LoByte, HiByte; 00102 LoByte = QMC5883L_ReadByte(OUT_Z_LSB); // read Accelerometer X_Low value 00103 HiByte = QMC5883L_ReadByte(OUT_Z_MSB); // read Accelerometer X_High value 00104 return ((HiByte<<8) | LoByte); 00105 } 00106 00107 int16_t QMC5883L::getMagTemp() 00108 { 00109 uint8_t LoByte, HiByte; 00110 LoByte = QMC5883L_ReadByte(TEMP_LSB); // read Accelerometer X_Low value 00111 HiByte = QMC5883L_ReadByte(TEMP_MSB); // read Accelerometer X_High value 00112 return ((HiByte<<8) | LoByte); 00113 }
Generated on Sun Jul 31 2022 13:20:13 by
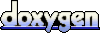