
after pin config implementation
Fork of BAE_FRDM by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "HK.h" 00004 #include "slave.h" 00005 #include "beacon.h" 00006 #include "ACS.h" 00007 #include "fault.h" 00008 #include "slave.h" 00009 00010 Serial pc(USBTX, USBRX); 00011 00012 InterruptIn interrupt(D9); 00013 InterruptIn master_reset(D11); 00014 00015 00016 Timer t; //To know the time of execution each thread 00017 Timer t1; 00018 //To know the time of entering of each thread 00019 00020 Thread *ptr_t_hk_acq; 00021 Thread *ptr_t_acs; 00022 Thread *ptr_t_acs_write2flash; 00023 Thread *ptr_t_bea; 00024 Thread *ptr_t_bea_telecommand; 00025 Thread *ptr_t_fault; 00026 Thread *ptr_t_i2c; 00027 00028 //-------------------------------------------------------------------------------------------------------------------------------------------------- 00029 //TASK 2 : HK 00030 //-------------------------------------------------------------------------------------------------------------------------------------------------- 00031 00032 00033 00034 void t_hk_acq(void const *args) 00035 { 00036 00037 while(1) 00038 { 00039 Thread::signal_wait(0x2); 00040 00041 printf("\nTHIS IS HK %f\n",t1.read()); 00042 t.start(); 00043 00044 FUNC_HK_MAIN(); //Collecting HK data 00045 //thread_2.signal_set(0x4); 00046 //FUNC_I2C_SLAVE_MAIN(24); //Sending to CDMS via I2C 00047 00048 t.stop(); 00049 printf("The time to execute hk_acq is %f seconds\n",t.read()); 00050 t.reset(); 00051 } 00052 } 00053 00054 //--------------------------------------------------------------------------------------------------------------------------------------- 00055 //TASK 1 : ACS 00056 //--------------------------------------------------------------------------------------------------------------------------------------- 00057 typedef struct { 00058 float mag_field; 00059 float omega; 00060 } sensor_data; 00061 00062 Mail <sensor_data, 16> q_acs; 00063 00064 void func_acs_readdata(sensor_data *ptr) 00065 { 00066 printf("Reading the data\n"); 00067 ptr -> mag_field = 10; 00068 ptr -> omega = 3; 00069 } 00070 00071 void func_acs_ctrlalgo() 00072 { 00073 printf("Executing control algo\n"); 00074 } 00075 00076 00077 00078 void func_acs_write2flash(sensor_data *ptr2) 00079 { 00080 printf("The magnetic field is %.2f T\n\r",ptr2->mag_field); 00081 printf("The angular velocity is %.2f rad/s\n\r",ptr2->omega); 00082 } 00083 00084 int acs_pflag = 1; 00085 void t_acs(void const *args) 00086 { 00087 while(1) 00088 { 00089 Thread::signal_wait(0x1); 00090 printf("\nTHIS IS ACS %f\n",t1.read()); 00091 t.start(); 00092 sensor_data *ptr = q_acs.alloc(); 00093 00094 func_acs_readdata(ptr); 00095 //printf("\ngdhd\n"); 00096 q_acs.put(ptr); 00097 if(acs_pflag == 1) 00098 { 00099 func_acs_ctrlalgo(); 00100 FUNC_ACS_GENPWM(); //Generating PWM signal. 00101 } 00102 00103 t.reset(); 00104 } 00105 } 00106 00107 void t_acs_write2flash(void const *args) 00108 { 00109 while(1) 00110 { 00111 //printf("Writing in the flash\n"); 00112 osEvent evt = q_acs.get(); 00113 if(evt.status == osEventMail) 00114 { 00115 sensor_data *ptr = (sensor_data*)evt.value.p; 00116 func_acs_write2flash(ptr); 00117 q_acs.free(ptr); 00118 } 00119 printf("Writing acs data in the flash\n"); 00120 } 00121 } 00122 00123 00124 //---------------------------------------------------BEACON-------------------------------------------------------------------------------------------- 00125 00126 int beac_flag=0; //To receive telecommand from ground. 00127 00128 00129 void t_bea_telecommand(void const *args) 00130 { 00131 char c = pc.getc(); 00132 if(c=='a') 00133 { 00134 printf("Telecommand detected\n"); 00135 beac_flag=1; 00136 } 00137 } 00138 00139 void t_bea(void const *args) 00140 { 00141 00142 while(1) 00143 { 00144 Thread::signal_wait(0x3); 00145 printf("\nTHIS IS BEACON %f\n",t1.read()); 00146 t.start(); 00147 00148 00149 00150 FUNC_BEA(); 00151 00152 00153 if(beac_flag==1) 00154 { 00155 Thread::wait(600000); 00156 beac_flag = 0; 00157 } 00158 00159 printf("The time to execute beacon thread is %f seconds\n",t.read()); 00160 t.reset(); 00161 } 00162 } 00163 00164 //--------------------------------------------------------------------------------------------------------------------------------------------------- 00165 //TASK 4 : FAULT MANAGEMENT 00166 //--------------------------------------------------------------------------------------------------------------------------------------------------- 00167 //Dummy fault rectifier functions 00168 00169 /*Mail<int,16> faults; 00170 00171 void FUNC_FAULT_FUNCT1() 00172 { 00173 printf("\nFault 1 detected... \n"); 00174 } 00175 00176 void FUNC_FAULT_FUNCT2() 00177 { 00178 printf("\nFault 2 detected...\n"); 00179 } 00180 00181 void T_FAULT(void const *args) 00182 { 00183 while(1) 00184 { 00185 osEvent evt = faults.get(); 00186 if(evt.status==osEventMail) 00187 { 00188 int *fault_id= (int *)evt.value.p; 00189 switch(*fault_id) 00190 { 00191 case 1: FUNC_FAULT_FUNCT1(); 00192 break; 00193 case 2: FUNC_FAULT_FUNCT2(); 00194 break; 00195 } 00196 faults.free(fault_id); 00197 } 00198 } 00199 }*/ 00200 00201 extern SensorData Sensor; 00202 00203 00204 void T_FAULT(void const *args) 00205 { 00206 Sensor.power_mode='0'; 00207 while(1) 00208 { 00209 Thread :: signal_wait(0x2); 00210 FAULTS(); 00211 POWER(Sensor.power_mode); 00212 //Sensor.power_mode++; //testing ... should be removed 00213 } 00214 } 00215 00216 //--------------------------------------------------------------------------------------------------------------------------------------------------- 00217 //TASK 5 : i2c data 00218 //--------------------------------------------------------------------------------------------------------------------------------------------------- 00219 00220 00221 void C_FUNC_INT() 00222 { 00223 FUNC_INT(); 00224 ptr_t_i2c->signal_set(0x4); 00225 00226 } 00227 00228 void C_FUNC_RESET() 00229 { 00230 FUNC_RESET(); 00231 } 00232 00233 00234 void C_T_I2C_BAE(void const * args) 00235 { 00236 //char data_send,data_receive; 00237 while(1) 00238 { 00239 Thread::signal_wait(0x4); 00240 T_I2C_BAE(); 00241 //i2c_status = temp; 00242 //wait(0.5); 00243 /*printf("\n entered thread\n\r"); 00244 if(i2c_status == 0 && reset !=1) 00245 { 00246 00247 FUNC_I2C_WRITE2CDMS(&data_receive); 00248 i2c_data * i2c_data_r = i2c_data_receive.alloc(); 00249 i2c_data_r->data = data_receive; 00250 i2c_data_r->length = 1; 00251 i2c_data_receive.put(i2c_data_r); 00252 printf("\n Data received from CDMS is %c\n\r",data_receive); 00253 i2c_data_receive.free(i2c_data_r); // This has to be done from a differen thread 00254 00255 } 00256 else if(i2c_status ==1 && reset !=1) 00257 { 00258 osEvent evt = i2c_data_send.get(); 00259 if (evt.status == osEventMail) 00260 { 00261 i2c_data *i2c_data_s = (i2c_data*)evt.value.p; 00262 data_send = i2c_data_s -> data; 00263 FUNC_I2C_WRITE2CDMS(&data_send); 00264 printf("\nData sent to CDMS is %c\n\r",data_send); 00265 i2c_data_send.free(i2c_data_s); 00266 i2c_status = 0; 00267 //temp = i2c_status; 00268 } 00269 } 00270 */ 00271 } 00272 } 00273 00274 00275 00276 00277 00278 00279 //------------------------------------------------------------------------------------------------------------------------------------------------ 00280 //SCHEDULER 00281 //------------------------------------------------------------------------------------------------------------------------------------------------ 00282 int beacon_sc = 3; 00283 uint16_t schedcount=1; 00284 void t_sc(void const *args) 00285 { 00286 00287 printf("The value of i in scheduler is %d\n",schedcount); 00288 if(schedcount == 65532) //to reset the counter 00289 { 00290 schedcount = 0; 00291 } 00292 00293 if(schedcount%1==0) 00294 { 00295 ptr_t_acs -> signal_set(0x1); 00296 } 00297 if(schedcount%2==0) 00298 { 00299 ptr_t_fault -> signal_set(0x2); 00300 ptr_t_hk_acq -> signal_set(0x2); 00301 00302 } 00303 if(schedcount%beacon_sc==0) 00304 { 00305 if(beac_flag==0) 00306 { 00307 00308 ptr_t_bea -> signal_set(0x3); 00309 } 00310 } 00311 schedcount++; 00312 } 00313 00314 //--------------------------------------------------------------------------------------------------------------------------------------------- 00315 00316 int main() 00317 { 00318 t1.start(); 00319 00320 ptr_t_hk_acq = new Thread(t_hk_acq); 00321 ptr_t_acs = new Thread(t_acs); 00322 ptr_t_acs_write2flash = new Thread(t_acs_write2flash); 00323 ptr_t_bea = new Thread(t_bea); 00324 ptr_t_bea_telecommand = new Thread(t_bea_telecommand); 00325 ptr_t_fault = new Thread(T_FAULT); 00326 ptr_t_i2c = new Thread(C_T_I2C_BAE); 00327 //ptr_t_sc = new Thread(t_sc); 00328 00329 interrupt_fault(); 00330 00331 ptr_t_fault -> set_priority(osPriorityRealtime); 00332 ptr_t_acs->set_priority(osPriorityHigh); 00333 ptr_t_i2c->set_priority(osPriorityAboveNormal); 00334 ptr_t_hk_acq->set_priority(osPriorityNormal); 00335 ptr_t_acs_write2flash->set_priority(osPriorityBelowNormal); 00336 ptr_t_bea->set_priority(osPriorityAboveNormal); 00337 ptr_t_bea_telecommand->set_priority(osPriorityIdle); 00338 //ptr_t_sc->set_priority(osPriorityAboveNormal); 00339 00340 00341 // ---------------------------------------------------------------------------------------------- 00342 printf("\n T_FAULT priority is %d",ptr_t_fault->get_priority()); 00343 printf("\n T_ACS priority is %d",ptr_t_acs->get_priority()); 00344 printf("\n T_HK_ACQ priority is %d",ptr_t_hk_acq->get_priority()); 00345 printf("\n T_ACS_WRITE2FLASH priority is %d",ptr_t_acs_write2flash->get_priority()); 00346 printf("\n T_BEA priority is %d",ptr_t_bea->get_priority()); 00347 RtosTimer t_sc_timer(t_sc,osTimerPeriodic); 00348 t_sc_timer.start(10000); 00349 printf("\n%f\n",t1.read()); 00350 00351 00352 00353 master_reset.fall(&C_FUNC_RESET); 00354 interrupt.rise(&C_FUNC_INT); 00355 00356 while(1) 00357 { 00358 //Thread::wait(10000); 00359 ir2master(); 00360 } 00361 00362 }
Generated on Tue Jul 12 2022 22:08:21 by
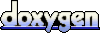