mbed device driver for HC-SR04 ultrasonic range finder
Dependents: ROBot Ultrasonic Ksen Core1000_SmartFarm ... more
HCSR04.cpp
00001 /* File: HCSR04.h 00002 * Author: Robert Abad Copyright (c) 2013 00003 * 00004 * Desc: driver for HCSR04 Ultrasonic Range Finder. See header file, 00005 * HCSR04.h, for more details. 00006 */ 00007 00008 #include "mbed.h" 00009 #include "HCSR04.h" 00010 00011 #define SPEED_OF_SOUND (343.2f) // meters/sec 00012 00013 // HCSR04 TRIGGER pin 00014 #define SIGNAL_HIGH (1) 00015 #define SIGNAL_LOW (0) 00016 00017 #define TRIGGER_TIME (10) // microseconds 00018 00019 00020 // Name: HCSR04 00021 // Desc: HCSR04 constructor 00022 // Inputs: PinName - pin used for trigger signal 00023 // PinName - pin used for echo signal 00024 // Outputs: none 00025 // 00026 HCSR04::HCSR04( PinName pinTrigger, PinName pinEcho ) : 00027 trigger(pinTrigger), 00028 echo(pinEcho), 00029 measTimeStart_us(0), 00030 measTimeStop_us(0) 00031 { 00032 echo.rise( this, &HCSR04::ISR_echoRising ); 00033 echo.fall( this, &HCSR04::ISR_echoFalling ); 00034 echoTimer.start(); 00035 } 00036 00037 // Name: startMeas 00038 // Desc: initiates range measurement driving the trigger signal HIGH then sets 00039 // a timer to keep trigger HIGH for the duration of TRIGGER_TIME 00040 // Inputs: none 00041 // Outputs: none 00042 // 00043 void HCSR04::startMeas(void) 00044 { 00045 trigger = SIGNAL_HIGH; 00046 echoTimer.reset(); 00047 triggerTicker.attach_us(this, &HCSR04::triggerTicker_cb, TRIGGER_TIME); 00048 } 00049 00050 // Name: getMeas 00051 // Desc: returns range measurement in meters 00052 // Inputs: float & - reference to range measurement 00053 // Outputs: etHCSR04 - RANGE_MEAS_VALID or RANGE_MEAS_INVALID 00054 // 00055 etHCSR04_RANGE_STATUS HCSR04::getMeas(float &rRangeMeters) 00056 { 00057 unsigned long dTime_us; 00058 if ( !measTimeStart_us || !measTimeStop_us ) 00059 { 00060 return RANGE_MEAS_INVALID; 00061 } 00062 00063 dTime_us = measTimeStop_us - measTimeStart_us; 00064 rRangeMeters = (float)dTime_us / 1000000. * SPEED_OF_SOUND / 2; 00065 measTimeStart_us = 0; 00066 measTimeStop_us = 0; 00067 return ( RANGE_MEAS_VALID ); 00068 } 00069 00070 // Name: triggerTicker_cb 00071 // Desc: Timer ticker callback function used to drive trigger signal LOW 00072 // Inputs: none 00073 // Outputs: none 00074 // 00075 void HCSR04::triggerTicker_cb(void) 00076 { 00077 trigger = SIGNAL_LOW; 00078 } 00079 00080 00081 // Name: ISR_echoRising 00082 // Desc: ISR for rising edge of HCSR04 ECHO signal 00083 // Inputs: none 00084 // Outputs: none 00085 // 00086 void HCSR04::ISR_echoRising(void) 00087 { 00088 measTimeStart_us = echoTimer.read_us(); 00089 } 00090 00091 // Name: ISR_echoFalling 00092 // Desc: ISR for falling edge of HCSR04 ECHO signal 00093 // Inputs: none 00094 // Outputs: none 00095 // 00096 void HCSR04::ISR_echoFalling(void) 00097 { 00098 measTimeStop_us = echoTimer.read_us(); 00099 } 00100
Generated on Wed Jul 13 2022 07:00:09 by
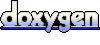