
This code allows you to use the TD Loader application to update the firmware on the Telecom Design module serially.
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut LED_0 (PB_6); 00004 00005 //Virtual serial port over USB 00006 Serial pc(USBTX, USBRX); 00007 Serial modem(PA_9, PA_10); 00008 00009 bool once = true; 00010 00011 int main() { 00012 pc.baud(9600); 00013 modem.baud(9600); 00014 char c; 00015 00016 LED_0 = 1; 00017 00018 char responsebuffer[3]; 00019 00020 while(1) { 00021 if(modem.readable()) { 00022 pc.putc(modem.getc()); 00023 } 00024 00025 if(pc.readable()) { 00026 c = pc.getc(); 00027 responsebuffer[0] = responsebuffer[1]; 00028 responsebuffer[1] = responsebuffer[2]; 00029 responsebuffer[2] = c; 00030 if( once && responsebuffer[0] == 'A' && responsebuffer[1] == 'T' && responsebuffer[2] == 'Z' ) 00031 { 00032 LED_0 = 0; 00033 wait(3); 00034 modem.printf("ATZ\n\rATZ\n\r"); 00035 wait(0.1); 00036 modem.baud(115200); 00037 pc.baud(115200); 00038 wait(0.1); 00039 once = false; 00040 } 00041 modem.putc(c); 00042 } 00043 } 00044 }
Generated on Thu Jul 14 2022 18:02:25 by
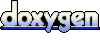