
STM32F103 WEB USB DFU 固件
Dependencies: mbed mbed-STM32F103C8T6 USBDevice_STM32F103
main.cpp
00001 #include "stm32f103c8t6.h" 00002 #include "mbed.h" 00003 #include "WebUSBDFU.h" 00004 00005 DigitalOut myled(LED1); 00006 00007 bool detached = false; 00008 void onDetachRequested() { 00009 detached = true; 00010 } 00011 00012 void resetIntoBootloader() { 00013 // Turn on write access to the backup registers 00014 __PWR_CLK_ENABLE(); 00015 HAL_PWR_EnableBkUpAccess(); 00016 00017 // Write the magic value to force the bootloader to run 00018 BKP->DR2 = 0x544F; 00019 BKP->DR1 = 0x4F42; 00020 00021 HAL_PWR_DisableBkUpAccess(); 00022 00023 // Reset and let the bootloader run 00024 NVIC_SystemReset(); 00025 } 00026 00027 int main() 00028 { 00029 confSysClock(); //Configure system clock (72MHz HSE clock, 48MHz USB clock) 00030 00031 /* Note: 1209:0001 is a test VID/PID pair - it should be changed before using in 00032 * a real application */ 00033 WebUSBDFU usbDFU(0x1209, 0x0001, 0x0001, false); 00034 usbDFU.attach(onDetachRequested); 00035 00036 while(1) { 00037 // Check the DFU status 00038 if (!usbDFU.configured()) { 00039 usbDFU.connect(false); 00040 } 00041 if (detached) { 00042 for (int i=0; i < 3; i++) { 00043 myled = 1; 00044 wait_ms(100); 00045 myled = 0; 00046 wait_ms(100); 00047 } 00048 resetIntoBootloader(); 00049 } 00050 00051 // Do normal stuff 00052 myled = !myled; 00053 wait_ms(500); 00054 } 00055 } 00056
Generated on Fri Jul 22 2022 00:33:17 by
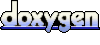