Library of menu structure
Embed:
(wiki syntax)
Show/hide line numbers
Navigator.cpp
00001 #include "Navigator.h" 00002 00003 Navigator::Navigator(Menu *root, RPG &rpg, TextLCD *lcd) : activeMenu(root), rpg(rpg), lcd(lcd) 00004 { 00005 bottom = root->selections.size(); 00006 cursorPos = 0; 00007 cursorLine = 1; 00008 button = 0; 00009 lastButton = 0; 00010 00011 printMenu(); 00012 printCursor(); 00013 } 00014 00015 void Navigator::printMenu() 00016 { 00017 lcd->cls(); 00018 if(bottom == 1){ // the current Menu only has one selection 00019 lcd->printf("%s\n", activeMenu->selections[0].selText); 00020 } else { 00021 if(cursorLine == 2){ // if we're at the bottom 00022 lcd->printf("%s\n", activeMenu->selections[cursorPos-1].selText); 00023 lcd->printf("%s\n", activeMenu->selections[cursorPos].selText); 00024 } else { 00025 lcd->printf("%s\n", activeMenu->selections[cursorPos].selText); 00026 lcd->printf("%s\n", activeMenu->selections[cursorPos+1].selText); 00027 } 00028 } 00029 } 00030 00031 void Navigator::printCursor() 00032 { 00033 if(activeMenu->selections[cursorPos].childMenu == NULL) printf("No child menu\n"); 00034 else printf("child menu: %s\n", activeMenu->selections[cursorPos].childMenu->menuID); 00035 00036 lcd->locate(0,0); 00037 if(cursorLine == 1){ 00038 lcd->putc('>'); 00039 lcd->locate(0,1); 00040 lcd->putc(' '); 00041 } else if(cursorLine == 2){ 00042 lcd->putc(' '); 00043 lcd->locate(0,1); 00044 lcd->putc('>'); 00045 } 00046 } 00047 00048 void Navigator::poll() 00049 { 00050 if((direction = rpg.dir())!=0){ //Get Dir 00051 wait(0.2); 00052 if(direction == 1) moveDown(); 00053 else if(direction == -1) moveUp(); 00054 } 00055 00056 if ((button = rpg.pb()) && !lastButton){ //prevents multiple selections when button is held down 00057 wait(0.2); 00058 if(activeMenu->selections[cursorPos].fun != NULL){ 00059 (activeMenu->selections[cursorPos].fun)(); 00060 } 00061 if(activeMenu->selections[cursorPos].childMenu != NULL){ 00062 activeMenu = activeMenu->selections[cursorPos].childMenu; 00063 bottom = activeMenu->selections.size(); 00064 cursorPos = 0; 00065 cursorLine = 1; 00066 printMenu(); 00067 printCursor(); 00068 } 00069 } 00070 lastButton = button; 00071 } 00072 00073 void Navigator::moveUp() 00074 { 00075 if(cursorLine == 1){ 00076 printMenu(); 00077 } else if(cursorLine == 2){ 00078 cursorLine = 1; 00079 } 00080 00081 if(cursorPos != 0){ 00082 cursorPos--; 00083 printMenu(); 00084 } 00085 printCursor(); 00086 } 00087 00088 void Navigator::moveDown() 00089 { 00090 if(cursorLine == 1){ 00091 cursorLine = 2; 00092 } else if(cursorLine == 2){ 00093 printMenu(); 00094 } 00095 00096 if(cursorPos != (bottom-1)){ 00097 cursorPos++; 00098 printMenu(); 00099 } 00100 printCursor(); 00101 }
Generated on Wed Jul 13 2022 18:45:29 by
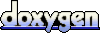