
Lab4 4180 photocell controlled cursor game
Dependencies: 4DGL-uLCD-SE SDFileSystem mbed-rtos mbed wave_player
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "uLCD_4DGL.h" 00004 #include "SDFileSystem.h" 00005 #include "wave_player.h" 00006 00007 // Mutex initialization 00008 Mutex lcdLock; 00009 Mutex speakerLock; 00010 Mutex hitLock; 00011 00012 // AnalogIn initialization 00013 AnalogIn photocell1(p15); 00014 AnalogIn photocell2(p16); 00015 00016 // Speaker initialization 00017 //PwmSpeaker speaker(p21); 00018 SDFileSystem sd(p5, p6, p7, p8, "sd"); //SD card 00019 FILE *wave_file; 00020 AnalogOut DACout(p18); 00021 wave_player waver(&DACout); 00022 00023 // uLCD initialization 00024 uLCD_4DGL uLCD(p28,p27,p26); // tx, rx, rst; 00025 00026 //Variable initializations 00027 int c1=0, c2=0, s1=0; //photocell variables 00028 int x=64, y=64, cursorRadius = 5; // cursor variables 00029 int xr=0,yr=0, hitRadius = 7; // beatmap variables 00030 int pnts = 0; int distAwaySqr = 100, distReqSqr = 101; int clearX=0, clearY=0; //handleHit variables 00031 static void handleHit() { 00032 Thread::wait(50); 00033 00034 hitLock.lock(); 00035 clearX = xr; 00036 clearY = yr; 00037 hitLock.unlock(); 00038 00039 distAwaySqr = (x-clearX)*(x-clearX) + (y-clearY)*(y-clearY); 00040 distReqSqr = (cursorRadius+hitRadius)*(cursorRadius+hitRadius); 00041 if ( distAwaySqr <= distReqSqr ) { 00042 pnts++; 00043 Thread::wait(50); 00044 00045 lcdLock.lock(); 00046 uLCD.circle(clearX,clearY,hitRadius+3,RED); 00047 uLCD.circle(clearX,clearY,hitRadius+5,RED); 00048 lcdLock.unlock(); 00049 Thread::wait(100); 00050 lcdLock.lock(); 00051 uLCD.filled_circle(clearX,clearY,hitRadius+5,BLACK); 00052 lcdLock.unlock(); 00053 00054 Thread::wait(50); 00055 00056 lcdLock.lock(); 00057 uLCD.printf("pnts: %d\r", pnts); 00058 lcdLock.unlock(); 00059 } 00060 Thread::wait(50); 00061 } 00062 00063 // Photocell thread 00064 void phc_thread(void const *args) { 00065 while(1){ 00066 c1 = (photocell1.read()- 0.06)*150; 00067 c1 = (c1>64) ? 64 : c1; //clamp below 64 00068 c1 = c1 - 32; // move between -32 and 31 00069 00070 c2 = (photocell2.read() - 0.06)*150; 00071 c2 = (c2>64) ? 64 : c2; //clamp below 64 00072 c2 = c2 - 32; // move between -32 and 31 00073 Thread::wait(50); 00074 } 00075 } 00076 00077 // player cursor thread 00078 void cursor_thread(void const *args) { 00079 while(1){ 00080 lcdLock.lock(); 00081 uLCD.filled_circle(x,y,cursorRadius,BLACK); 00082 lcdLock.unlock(); 00083 Thread::wait(50); 00084 00085 x = 64-c1; 00086 y = 64-c2; 00087 handleHit(); 00088 00089 lcdLock.lock(); 00090 uLCD.filled_circle(x,y,cursorRadius,GREEN); 00091 lcdLock.unlock(); 00092 Thread::wait(50); 00093 } 00094 } 00095 00096 // beatmap thread 00097 void bm_thread(void const *args) { 00098 while(1){ 00099 hitLock.lock(); 00100 xr = rand()%64 + 32; 00101 yr = rand()%64 + 32; 00102 hitLock.unlock(); 00103 00104 lcdLock.lock(); 00105 uLCD.filled_circle(xr,yr,hitRadius,0xFF00FF); 00106 lcdLock.unlock(); 00107 00108 Thread::wait(4000); 00109 00110 lcdLock.lock(); 00111 uLCD.filled_circle(xr,yr,hitRadius,BLACK); 00112 lcdLock.unlock(); 00113 Thread::wait(50); 00114 } 00115 } 00116 00117 // Sound thread 00118 void sound_thread(void const *args) { 00119 while(1){ 00120 wave_file=fopen("/sd/wavfiles/CaveStory.wav","r"); 00121 speakerLock.lock(); 00122 waver.play(wave_file); 00123 speakerLock.unlock(); 00124 fclose(wave_file); 00125 Thread::wait(30000); // wait 30s before playing again 00126 } 00127 00128 } 00129 00130 int main() { 00131 uLCD.printf("pnts: %d\n\n\n\n\n\n\n\n\n\n\n\n\n\n", pnts); 00132 uLCD.printf("Chase pink circle!"); 00133 uLCD.locate(0,0); 00134 Thread cursor(cursor_thread); 00135 Thread beatmap(bm_thread); 00136 Thread sound(sound_thread); 00137 Thread pcell(phc_thread); 00138 00139 while (true) { 00140 } 00141 }
Generated on Sat Jul 23 2022 07:03:02 by
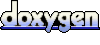