
A program that sends a image saved to the sd card to a server through a tcp socket connection
Dependencies: EthernetInterface SDFileSystem mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "SDFileSystem.h" 00004 00005 const char* SERVER_ADDRESS = ""; 00006 const int SERVER_PORT = 5005; 00007 SDFileSystem fs(p5, p6, p7, p8, "sd"); 00008 //LocalFileSystem local("local"); // Create the local filesystem under the name "local" 00009 00010 00011 int main() { 00012 EthernetInterface eth; 00013 eth.init(); //Use DHCP 00014 eth.connect(); 00015 printf("IP Address is %s\n\r", eth.getIPAddress()); 00016 00017 TCPSocketConnection socket; 00018 while (socket.connect(SERVER_ADDRESS, SERVER_PORT) < 0) { 00019 printf("Unable to connect to (%s) on port (%d)\n\r", SERVER_ADDRESS, SERVER_PORT); 00020 wait(1); 00021 } 00022 00023 FILE *file; 00024 char *buffer; 00025 unsigned long fileLen; 00026 00027 //Open file 00028 file = fopen("/sd/IMG_0001.jpg", "rb"); 00029 if (!file) 00030 { 00031 fprintf(stderr, "Unable to open file"); 00032 exit(0); 00033 } 00034 00035 //Get file length 00036 int size; 00037 00038 fseek(file, 0, SEEK_END); 00039 size=ftell(file); 00040 fseek(file, 0, SEEK_SET); 00041 00042 char send_buffer[size]; 00043 while(!feof(file)) { 00044 fread(send_buffer, 1, sizeof(send_buffer), file); 00045 socket.send(send_buffer, sizeof(send_buffer)); 00046 } 00047 00048 free(buffer); 00049 printf("Done Sending\n\r"); 00050 00051 00052 socket.close(); 00053 eth.disconnect(); 00054 00055 while(true) {} 00056 }
Generated on Fri Jul 22 2022 12:05:34 by
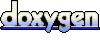