
Pulse consistency test using wait_us in a thread
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2018 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 #include <mbed.h> 00007 00008 #define CH_INIT 0 00009 #define CH_BOOST_START 1 00010 #define CH_BOOSTING 2 00011 #define CH_POST_BOOST 3 00012 #define CH_WAIT_200MS 4 00013 #define CH_WAIT_800MS 5 00014 00015 #define SAMPLE_FLAG1 (1UL << 0) 00016 00017 Serial pc(p6, p8); 00018 00019 DigitalOut led1(LED1); // Used to indicate boost state to user 00020 DigitalOut led2(LED2); // Used to indicate boost state to user 00021 00022 DigitalOut ch1(LED4); 00023 00024 Timeout chStateTimeout; 00025 00026 Timer t; 00027 00028 Thread thread; 00029 00030 //Semaphore sem(0); 00031 00032 EventFlags event_flags; 00033 00034 volatile bool ch_progress = false; 00035 volatile bool ch_boost_done = false; 00036 00037 // ----------------------------------------------------------------------------- 00038 void timeoutHandler() { 00039 ch_progress = true; 00040 } 00041 00042 // ----------------------------------------------------------------------------- 00043 void chBoostThread() { 00044 while (1) { 00045 //sem.wait(); 00046 //Thread::signal_wait(0x1); 00047 event_flags.wait_any(SAMPLE_FLAG1); 00048 00049 t.start(); 00050 ch1 = 1; 00051 wait_us(100); 00052 ch1 = 0; 00053 t.stop(); 00054 ch_boost_done = true; 00055 } 00056 } 00057 00058 // ----------------------------------------------------------------------------- 00059 int main() 00060 { 00061 uint8_t ch_state = CH_INIT; 00062 unsigned long dummy_count = 0, dummy_count_post = 0; 00063 00064 ch1 = 0; 00065 00066 thread.start(chBoostThread); 00067 00068 while(1) { 00069 switch (ch_state) { 00070 case CH_INIT: 00071 ch1 = 0; 00072 ch_progress = false; 00073 ch_boost_done = false; 00074 dummy_count = 0; 00075 dummy_count_post = 0; 00076 ch_state = CH_BOOST_START; 00077 break; 00078 case CH_BOOST_START: 00079 ch_state = CH_BOOSTING; 00080 led1 = 1; // Give visual indication at start of boost stage 00081 //sem.release(); 00082 //thread.signal_set(0x1); 00083 event_flags.set(SAMPLE_FLAG1); 00084 case CH_BOOSTING: 00085 dummy_count++; // measure response during 100us boost stage 00086 00087 if (ch_boost_done) { 00088 ch_state = CH_POST_BOOST; 00089 chStateTimeout.attach_us(&timeoutHandler, 1000); 00090 } 00091 else break; 00092 case CH_POST_BOOST: 00093 dummy_count_post++; // measuring response after boost stage for 1ms 00094 00095 if (ch_progress) { 00096 ch_progress = false; 00097 ch_state = CH_WAIT_200MS; 00098 chStateTimeout.attach(&timeoutHandler, 0.2f); 00099 } 00100 break; 00101 case CH_WAIT_200MS: 00102 if (ch_progress) { 00103 ch_progress = false; 00104 led1 = 0; // Turn off boost indication 00105 ch_state = CH_WAIT_800MS; 00106 chStateTimeout.attach(&timeoutHandler, 0.8f); 00107 } 00108 break; 00109 case CH_WAIT_800MS: 00110 if (ch_progress) { 00111 ch_progress = false; 00112 ch_state = CH_INIT; 00113 00114 pc.printf("CH_BOOSTING COUNT: %lu; CH_POST_BOOST COUNT: %lu; TIME TAKEN: %d\r\n", dummy_count, dummy_count_post, t.read_us()); // print measured response; 00115 t.reset(); 00116 } 00117 break; 00118 } 00119 00120 } 00121 }
Generated on Sun Jul 24 2022 08:02:40 by
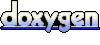