
12Oct2012MBEDLab3Project
Dependencies: DebounceIn EthernetNetIf FatFileSystem HTTPClient Motor SDFileSystem TextLCD mbed
main.cpp
00001 #include "mbed.h" 00002 #include "VS1002.h" 00003 #include "TextLCD.h" 00004 #include "EthernetNetIf.h" 00005 #include "HTTPClient.h" 00006 #include "DebounceIn.h" 00007 #include "Motor.h" 00008 #include <string> 00009 #include<stdlib.h> 00010 #define PI 3.14 00011 #define DIAMETER 0.076 00012 #define MINIMUM_FARE 11 00013 #define FARE_PER_KM 10 00014 00015 TextLCD fareDisplay(p10, p18, p24, p23, p22, p21 ); 00016 VS1002 audioDriver(p11, p12, p13, p8, "sd", 00017 p5, p6, p7, p14, p15, 00018 p16, p17, p20); 00019 EthernetNetIf ethernet; 00020 HTTPClient http; 00021 AnalogIn sensorReading(p19); 00022 DebounceIn setup(p9); 00023 DebounceIn startMeter(p20); 00024 DebounceIn stopMeter(p30); 00025 DebounceIn playFare(p29); 00026 Motor m(p25, p26, p27); 00027 00028 void initializeAudioDriver() 00029 { 00030 #ifndef FS_ONLY 00031 audioDriver._RST = 1; 00032 audioDriver.cs_high(); 00033 audioDriver.sci_initialise(); 00034 audioDriver.sci_write(0x00,(SM_SDINEW+SM_STREAM+SM_DIFF)); 00035 audioDriver.sci_write(0x03, 0x9800); 00036 audioDriver.sdi_initialise(); 00037 #endif 00038 } 00039 00040 float calculateFare(int noOfRevolutions) 00041 { 00042 float distanceinKM; 00043 distanceinKM = noOfRevolutions*PI*DIAMETER;//For Demo purposes we do not divide by 1000 as would be in real scenario. 00044 float fare; 00045 if(distanceinKM>1) 00046 fare = MINIMUM_FARE + FARE_PER_KM*(distanceinKM - 1); // Formula can be changed as per required or can be taken dynamically from a web server 00047 else 00048 fare = MINIMUM_FARE; 00049 return fare; 00050 } 00051 00052 00053 void convertFareToSpeech(float fare) 00054 { 00055 string ttsURL=" http://translate.google.com/translate_tts?tl=en&q=Your+Fare+is+;+"; 00056 char strFare[100]; 00057 sprintf(strFare,"%d",(int)fare); 00058 ttsURL+=strFare; 00059 ttsURL+="+Rupees+.+Thank+you+for+the+ride+.+Have+a+Nice+Day+."; 00060 //printf("%s\n",ttsURL); 00061 HTTPFile audioOutput("/sd/Fare.mp3"); 00062 HTTPResult result = http.get(ttsURL.c_str(),&audioOutput); 00063 if(result!=HTTP_OK) 00064 { 00065 printf("Error during speech convrsion!! Error Number : %d\n", result); 00066 } 00067 else 00068 printf("Done\n"); 00069 audioDriver.play_song("/sd/Fare.mp3"); 00070 00071 } 00072 00073 void initialize_system() 00074 { 00075 setup.mode(PullUp); 00076 wait(0.001); 00077 startMeter.mode(PullUp); 00078 wait(0.001); 00079 stopMeter.mode(PullUp); 00080 wait(0.001); 00081 playFare.mode(PullUp); 00082 wait(0.001); 00083 wait(2); 00084 fareDisplay.printf("PRESS SETUP"); 00085 printf("Press setup to continue.....\n"); 00086 } 00087 00088 int main() 00089 { 00090 bool flag = false; 00091 int noOfRevolutions =0; 00092 float fare; 00093 bool isMeterStopped=false; 00094 00095 initialize_system(); 00096 00097 while(setup==1){wait(0.1);} 00098 while(setup == 0){wait(0.1);} 00099 fareDisplay.cls(); 00100 fareDisplay.printf("INITIALIZING..."); 00101 00102 initializeAudioDriver(); 00103 EthernetErr error = ethernet.setup(); 00104 if(error) 00105 { 00106 printf("Error in correction. Error number: %d.\n", error); 00107 return -1; 00108 } 00109 printf(" Connection established\n"); 00110 00111 while(1) 00112 { 00113 fareDisplay.cls(); 00114 fareDisplay.printf("START METER"); 00115 printf("Press start to continue.....\n"); 00116 while(startMeter==1){wait(0.1);} 00117 while(startMeter==0){wait(0.1);} 00118 int new_val = 0; 00119 int old_val =0; 00120 fareDisplay.cls(); 00121 fareDisplay.printf("METER STARTED"); 00122 { 00123 m.speed(0.15); 00124 } 00125 while(!isMeterStopped) 00126 { 00127 if(sensorReading>0.5) 00128 { 00129 flag=true; 00130 wait(0.1); 00131 } 00132 if(flag) 00133 { 00134 if(sensorReading<0.4) 00135 { 00136 flag=false; 00137 noOfRevolutions++; 00138 } 00139 } 00140 new_val = stopMeter; 00141 if ((new_val==0) && (old_val==1)) 00142 { 00143 isMeterStopped = true; 00144 fareDisplay.cls(); 00145 fareDisplay.printf("METER STOPPED !!"); 00146 m.speed(0); 00147 } 00148 old_val=new_val; 00149 wait(0.1); 00150 } 00151 00152 if(isMeterStopped) 00153 { 00154 isMeterStopped = false; 00155 fare = calculateFare(noOfRevolutions); 00156 fareDisplay.cls(); 00157 fareDisplay.printf("The Fare is: %d",(int)fare); 00158 printf("The no of revolutions is: %d\n; The Fare is: %d\n",(int)noOfRevolutions,(int)fare); 00159 convertFareToSpeech(fare); 00160 } 00161 bool isExit =false; 00162 while(1) 00163 { 00164 while(playFare==1) 00165 { 00166 new_val = stopMeter; 00167 if ((new_val==0) && (old_val==1)) 00168 { 00169 isExit = true; 00170 break; 00171 } 00172 old_val=new_val; 00173 wait(0.2); 00174 } 00175 while(playFare==0){wait(0.1);} 00176 if(isExit) 00177 break; 00178 audioDriver.play_song("/sd/Fare.mp3"); 00179 00180 } 00181 } 00182 }
Generated on Thu Jul 21 2022 13:54:34 by
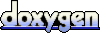