Very simple library for reading temperature from NTC thermistors
Dependents: lightweight-weather-station
thermistor.h
00001 /** Measuring temperature with thermistor 00002 * 00003 * Example of use: 00004 * @code 00005 * #include "mbed.h" 00006 * #include "thermistor.h" 00007 * 00008 * Serial pc(USBTX, USBRX); // serial communication 00009 * 00010 * int main() { 00011 * Thermistor my_thermistor(A5, 10000, 3950, 4700); // instantiate the sensor object (on pin A5, nominal resistance is 10kOhms, beta coefficient is 3950 and value of the sacond resistor is 4,7kOhms 00012 * while(1) { 00013 * pc.printf("Temperature: %5.1f celsius degrees\r", my_thermistor.temperature()); 00014 * pc.printf("Resistance: %5.1f ohms\r", my_thermistor.resistance()); 00015 * wait_ms(1000); 00016 * } 00017 * } 00018 * @endcode 00019 */ 00020 class Thermistor 00021 { 00022 00023 public: 00024 00025 /** 00026 * @param thermistorpin mbed analog pin to which the thermistor is connected to 00027 * @param nominalres resistance of thermistor at 25 celsius degrees 00028 * @param beta beta coefficient of thermistor 00029 * @param seriesresistor value of the resistor connecter with thermistor 00030 */ 00031 Thermistor (PinName thermistorpin, int nominalres, int beta, int seriesresistor); 00032 00033 /** 00034 * @returns temperature in celsius degrees 00035 */ 00036 float temperature (); 00037 void get_temperature(); 00038 /** 00039 * @returns resistance of thermistor 00040 */ 00041 float resistance (); 00042 void init(); 00043 00044 private: 00045 00046 int temperaturenominal; 00047 AnalogIn thermistorpin; 00048 int nominalres; 00049 int beta; 00050 int seriesresistor; 00051 float temp; 00052 float res; 00053 float steinhart; 00054 int a; 00055 00056 };
Generated on Sun Jul 17 2022 23:03:46 by
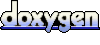