Since things didn't work out between me and mbedtls, here are the basic encryption algorithms.
Embed:
(wiki syntax)
Show/hide line numbers
rsa-small-numbers.cpp
00001 #include<iostream> 00002 #include<math.h> 00003 00004 int gcd(int a, int h) 00005 { 00006 int temp; 00007 while (1) 00008 { 00009 temp = a%h; 00010 if (temp == 0) 00011 return h; 00012 a = h; 00013 h = temp; 00014 } 00015 } 00016 00017 int main() 00018 { 00019 double p = 3; 00020 double q = 7; 00021 double n = p*q; 00022 double e = 2; 00023 double phi = (p-1)*(q-1); 00024 while (e < phi) 00025 { 00026 00027 if (gcd(e, phi)==1) 00028 break; 00029 else 00030 e++; 00031 } 00032 00033 int k = 2; 00034 double d = (1 + (k*phi))/e; 00035 00036 double msg = 20; 00037 00038 printf("Message data = %lf", msg); 00039 double c = pow(msg, e); 00040 c = fmod(c, n); 00041 printf("\nEncrypted data = %lf", c); 00042 00043 double m = pow(c, d); 00044 m = fmod(m, n); 00045 printf("\nOriginal Message Sent = %lf", m); 00046 00047 return 0; 00048 }
Generated on Thu Jul 21 2022 11:53:31 by
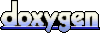