Adapted to Lora Semtech + Nucleo
Dependents: LoRaWAN-lmic-app LoRaWAN-lmic-app LoRaWAN-test-10secs LoRaPersonalizedDeviceForEverynet ... more
Fork of lwip_ppp_ethernet by
cc.h
00001 /* cc.h */ 00002 /* 00003 Copyright (C) 2012 ARM Limited. 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of 00006 this software and associated documentation files (the "Software"), to deal in 00007 the Software without restriction, including without limitation the rights to 00008 use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies 00009 of the Software, and to permit persons to whom the Software is furnished to do 00010 so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in all 00013 copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00021 SOFTWARE. 00022 */ 00023 00024 #ifndef CC_H_ 00025 #define CC_H_ 00026 00027 #include <stdint.h> 00028 00029 //This file was written according to http://lwip.wikia.com/wiki/Porting_for_an_OS and http://git.savannah.gnu.org/cgit/lwip.git/tree/doc/sys_arch.txt 00030 00031 //Data types 00032 typedef uint8_t u8_t; 00033 typedef uint16_t u16_t; 00034 typedef uint32_t u32_t; 00035 00036 typedef int8_t s8_t; 00037 typedef int16_t s16_t; 00038 typedef int32_t s32_t; 00039 00040 typedef uint32_t mem_ptr_t; 00041 00042 //Debug 00043 //__DEBUG__ must be defined to enable the macros 00044 #ifndef __DEBUG__ 00045 #define __DEBUG__ 4 //Maximum verbosity 00046 #endif 00047 #ifndef __MODULE__ 00048 #define __MODULE__ "LwIP" 00049 #endif 00050 #include "dbg.h" 00051 00052 #define LWIP_PLATFORM_DIAG(...) DBG(__VA_ARGS__) 00053 //#define LWIP_PLATFORM_ASSERT(x) do{ ERR(x); } while(0) 00054 #define LWIP_PLATFORM_ASSERT(...) ERR(__VA_ARGS__) 00055 00056 //Printf formatters 00057 #define U16_F "hu" 00058 #define S16_F "d" 00059 #define X16_F "hx" 00060 #define U32_F "u" 00061 #define S32_F "d" 00062 #define X32_F "x" 00063 #define SZT_F "uz" 00064 00065 //Endianness 00066 #define BYTE_ORDER LITTLE_ENDIAN 00067 00068 #define LWIP_PLATFORM_BYTESWAP 1 00069 /* 00070 #define LWIP_PLATFORM_HTONS(x) ( (((u16_t)(x))>>8) | (((x)&0xFF)<<8) ) 00071 #define LWIP_PLATFORM_HTONL(x) ( (((u32_t)(x))>>24) | (((x)&0xFF0000)>>8) \ 00072 | (((x)&0xFF00)<<8) | (((x)&0xFF)<<24) ) 00073 */ 00074 00075 #include "cmsis.h" 00076 #define LWIP_PLATFORM_HTONS(x) __REV16(x) 00077 #define LWIP_PLATFORM_HTONL(x) __REV(x) 00078 00079 //Checksums 00080 /* 00081 IP protocols use checksums (see RFC 1071). LwIP gives you a choice of 3 algorithms: 00082 load byte by byte, construct 16 bits word and add: not efficient for most platforms 00083 load first byte if odd address, loop processing 16 bits words, add last byte. 00084 load first byte and word if not 4 byte aligned, loop processing 32 bits words, add last word/byte. 00085 */ 00086 #define LWIP_CHKSUM_ALGORITHM 3 00087 00088 //Structure packing 00089 #if defined ( __CC_ARM ) 00090 00091 //This will work with ARMCC 00092 #define PACK_STRUCT_FIELD(x) x//__packed x 00093 #define PACK_STRUCT_STRUCT 00094 #define PACK_STRUCT_BEGIN __packed 00095 #define PACK_STRUCT_END 00096 #define ALIGNED(n) __align(n) 00097 00098 #elif defined ( __GNUC__ ) 00099 00100 //This will work with GCC and ARMCC in GCC-compatible mode 00101 #define PACK_STRUCT_FIELD(x) x __attribute__((packed)) 00102 #define PACK_STRUCT_STRUCT __attribute__((packed)) 00103 #define PACK_STRUCT_BEGIN 00104 #define PACK_STRUCT_END 00105 #define ALIGNED(n) __attribute__((aligned (n))) 00106 00107 #else 00108 #error "This toolchain is not currently supported for LwIP support (you can FIXME)" 00109 #endif 00110 00111 #define LWIP_PROVIDE_ERRNO 00112 00113 #define SYS_LIGHTWEIGHT_PROT 1 00114 /* 00115 sys.h will provide default definitions (as of version 1.3.0) of these macros as: 00116 #define SYS_ARCH_DECL_PROTECT(lev) sys_prot_t lev 00117 #define SYS_ARCH_PROTECT(lev) lev = sys_arch_protect() 00118 #define SYS_ARCH_UNPROTECT(lev) sys_arch_unprotect(lev) 00119 */ 00120 00121 #endif /* CC_H_ */
Generated on Tue Jul 12 2022 15:12:14 by
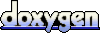