
adding resources firmware and 1/0/8
Dependencies: Beep C12832_lcd EthernetInterface EthernetNetIf HTTPClient LM75B MMA7660 mbed-rtos mbed nsdl_lib
Fork of LWM2M_NanoService_Ethernet by
register_upd_trigger.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "nsdl_support.h" 00004 #include "register_upd_trigger.h" 00005 00006 #define REGISTER_UPD_TRIGGER_ID "1/0/8" 00007 00008 extern char endpoint_name[16]; 00009 extern uint8_t ep_type[]; 00010 extern uint8_t lifetime_ptr[]; 00011 extern Serial pc; 00012 00013 /* Only POST and PUT method allowed */ 00014 static uint8_t register_upd_trigger_resource_cb(sn_coap_hdr_s *received_coap_ptr, sn_nsdl_addr_s *address, sn_proto_info_s * proto) 00015 { 00016 sn_coap_hdr_s *coap_res_ptr = 0; 00017 00018 strcpy((char*) ep_type, "mbed_lpc1768_appboard"); 00019 strcpy((char*) lifetime_ptr, "60"); 00020 pc.printf("Register Update Trigger callback\r\n"); 00021 00022 if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_POST) 00023 { 00024 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CHANGED); 00025 sn_nsdl_send_coap_message(address, coap_res_ptr); 00026 00027 sn_nsdl_ep_parameters_s *endpoint_ptr = NULL; 00028 endpoint_ptr = nsdl_init_register_endpoint(endpoint_ptr, (uint8_t*)endpoint_name, ep_type, lifetime_ptr); 00029 00030 sn_nsdl_update_registration(endpoint_ptr); 00031 } 00032 else if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_PUT) 00033 { 00034 00035 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CHANGED); 00036 sn_nsdl_send_coap_message(address, coap_res_ptr); 00037 00038 sn_nsdl_ep_parameters_s *endpoint_ptr = NULL; 00039 endpoint_ptr = nsdl_init_register_endpoint(endpoint_ptr, (uint8_t*)endpoint_name, (uint8_t*)ep_type, (uint8_t*)lifetime_ptr); 00040 00041 sn_nsdl_update_registration(endpoint_ptr); 00042 } 00043 00044 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00045 return 0; 00046 } 00047 00048 int create_register_upd_trigger_resource(sn_nsdl_resource_info_s *resource_ptr) 00049 { 00050 nsdl_create_dynamic_resource(resource_ptr, strlen(REGISTER_UPD_TRIGGER_ID), (uint8_t*)REGISTER_UPD_TRIGGER_ID, 0, 0, 0, ®ister_upd_trigger_resource_cb, (SN_GRS_POST_ALLOWED | SN_GRS_PUT_ALLOWED)); 00051 return 0; 00052 }
Generated on Sat Jul 16 2022 02:03:09 by
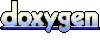