
adding resources firmware and 1/0/8
Dependencies: Beep C12832_lcd EthernetInterface EthernetNetIf HTTPClient LM75B MMA7660 mbed-rtos mbed nsdl_lib
Fork of LWM2M_NanoService_Ethernet by
firmware_status.cpp
00001 // Firmware status resource implementation 00002 00003 #include "mbed.h" 00004 #include "rtos.h" 00005 #include "LM75B.h" 00006 #include "nsdl_support.h" 00007 #include "firmware_status.h" 00008 #include "firmware_result.h" 00009 00010 #define FIRMWARE_UPD_STATUS_RES_ID "5/0/2" 00011 00012 extern Serial pc; 00013 extern "C" void mbed_reset(); 00014 00015 /** 00016 * Cleanup all bin files. 00017 */ 00018 int cleanupAllBinFiles(void) { 00019 struct dirent *p; 00020 DIR *dir = opendir("/local"); 00021 if (dir == NULL) { 00022 return -1; 00023 } 00024 while ((p = readdir(dir)) != NULL) { 00025 char *str = p->d_name; 00026 00027 if ((strstr(str, ".bin") != NULL) || (strstr(str, ".BIN") != NULL)) { 00028 char buf[BUFSIZ]; 00029 snprintf(buf, sizeof(buf) - 1, "/local/%s", str); 00030 if (remove(buf) == 0) { 00031 pc.printf("INFO: Deleted '%s'.\r\n", buf); 00032 } else { 00033 pc.printf("ERR : Delete '%s' failed.\r\n", buf); 00034 } 00035 } 00036 } 00037 closedir(dir); 00038 return 0; 00039 } 00040 00041 /** fcopy: Copies a file 00042 * Checks to ensure destination file was created. 00043 * Returns -1 = error; 0 = success 00044 */ 00045 int fcopy (const char *src, const char *dst) { 00046 FILE *fpsrc = fopen(src, "r"); 00047 00048 if (fpsrc == NULL) 00049 return 0; 00050 00051 FILE *fpdst = fopen(dst, "w"); 00052 00053 if (fpdst == NULL) 00054 return 0; 00055 00056 int ch = fgetc(fpsrc); 00057 while (ch != EOF) { 00058 fputc(ch, fpdst); 00059 ch = fgetc(fpsrc); 00060 } 00061 fclose(fpsrc); 00062 fclose(fpdst); 00063 int retval = 0; 00064 fpdst = fopen(dst, "r"); 00065 if (fpdst == NULL) { 00066 retval = 0; 00067 } else { 00068 fclose(fpdst); 00069 retval = 1; 00070 } 00071 return retval; 00072 } 00073 00074 /* Only GET, POST method allowed */ 00075 static uint8_t firmware_resource_status_cb(sn_coap_hdr_s *received_coap_ptr, sn_nsdl_addr_s *address, sn_proto_info_s * proto) 00076 { 00077 sn_coap_hdr_s *coap_res_ptr = 0; 00078 char firmware_upd_status[16]; 00079 00080 pc.printf("firmware status callback\r\n"); 00081 00082 if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_POST) 00083 { 00084 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CHANGED); 00085 sn_nsdl_send_coap_message(address, coap_res_ptr); 00086 wait(2); 00087 FILE *fpsrc = fopen("/local/OUT.B__", "r"); 00088 00089 if (fpsrc == NULL) 00090 { 00091 pc.printf("Tmp file not found...\r\n"); 00092 setResult(7); 00093 send_firmware_result_observation(7); 00094 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00095 return 0; 00096 } 00097 00098 if (cleanupAllBinFiles() == -1) 00099 { 00100 pc.printf("Cleaning files failed...\r\n"); 00101 setResult(2); 00102 send_firmware_result_observation(2); 00103 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00104 return 0; 00105 } 00106 00107 pc.printf("Copying NEW_FW...\r\n"); 00108 if (fcopy("/local/OUT.B__","/local/NEW_FW.BIN") == 0) 00109 { 00110 setResult(2); 00111 send_firmware_result_observation(2); 00112 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00113 return 0; 00114 } 00115 pc.printf("Deleting tmp file...\r\n"); 00116 if (remove("/local/out.b__") != 0) 00117 { 00118 /*setResult(0); 00119 send_firmware_result_observation(0); 00120 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00121 return 0;*/ 00122 } 00123 pc.printf("Resetting...\r\n"); 00124 wait(3); 00125 setResult(1); 00126 mbed_reset(); 00127 00128 } 00129 00130 if(received_coap_ptr->msg_code == COAP_MSG_CODE_REQUEST_GET) 00131 { 00132 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CONTENT); 00133 00134 sprintf(firmware_upd_status, "%d", 1); 00135 00136 coap_res_ptr->payload_len = strlen(firmware_upd_status); 00137 coap_res_ptr->payload_ptr = (uint8_t*)firmware_upd_status; 00138 sn_nsdl_send_coap_message(address, coap_res_ptr); 00139 } 00140 00141 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00142 return 0; 00143 } 00144 00145 int create_firmware_status_resource(sn_nsdl_resource_info_s *resource_ptr) 00146 { 00147 nsdl_create_dynamic_resource(resource_ptr, sizeof(FIRMWARE_UPD_STATUS_RES_ID)-1, (uint8_t*) FIRMWARE_UPD_STATUS_RES_ID, 0, 0, 0, &firmware_resource_status_cb, SN_GRS_GET_ALLOWED | SN_GRS_POST_ALLOWED); 00148 return 0; 00149 } 00150 00151
Generated on Sat Jul 16 2022 02:03:09 by
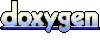