
Code for Player 1 of Rock Paper Scissors Game
Dependencies: 4DGL-uLCD-SE PinDetect SDFileSystem mbed wave_player
main.cpp
00001 //ECE 4180 Mini Project 00002 //Prachi Kulkarni and Kendra Dodson 00003 00004 // This code is for Player 1 00005 00006 #include "mbed.h" 00007 #include "uLCD_4DGL.h" 00008 #include "SDFileSystem.h" 00009 #include "wave_player.h" 00010 00011 //Leds to show status of sending and receiving messages 00012 DigitalOut myled(LED1); 00013 DigitalOut myled2(LED2); 00014 DigitalOut myled3(LED3); 00015 00016 //SD Card Reader 00017 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00018 AnalogOut DACout(p18); 00019 wave_player waver(&DACout); // Wave Player 00020 00021 uLCD_4DGL lcd(p28, p27, p29); //uLCD 00022 DigitalIn pb1(p17); //Pushbutton 1 - Rock 00023 DigitalIn pb2(p19); //Pushbutton 2 - Paper 00024 DigitalIn pb3(p20); //Pushbutton 3 - Scissors 00025 00026 //Shiftbrite 00027 DigitalOut latch(p15); 00028 DigitalOut enable(p16); 00029 SPI spi(p11, p12, p13); 00030 00031 //Choice 00032 //Choice = 1 for rock 00033 //Choice = 2 for paper 00034 //Choice = 3 for scissors 00035 char choice1; //Player 1's choice 00036 char choice2; //Player 2's choice 00037 00038 //Points 00039 int p1 = 0; //Player 1's points 00040 int p2 = 0; //Player 2's points 00041 00042 //RGB function for Shiftbrite 00043 void RGB_LED(int red, int green, int blue); 00044 void RGB_LED(int red, int green, int blue) 00045 { 00046 unsigned int low_color=0; 00047 unsigned int high_color=0; 00048 high_color=(blue<<4)|((red&0x3C0)>>6); 00049 low_color=(((red&0x3F)<<10)|(green)); 00050 spi.write(high_color); 00051 spi.write(low_color); 00052 latch=1; 00053 latch=0; 00054 } 00055 int i; 00056 00057 //Serial 00058 Serial player1(p9,p10); 00059 00060 int main() { 00061 00062 //Serial 00063 player1.baud(9600); 00064 spi.format(16,0); 00065 spi.frequency(500000); 00066 enable=0; 00067 latch=0; 00068 pb1.mode(PullUp); 00069 wait(0.001); 00070 pb2.mode(PullUp); 00071 wait(0.001); 00072 pb3.mode(PullUp); 00073 wait(0.001); 00074 00075 //Display 00076 lcd.locate(1,2); 00077 //Screen 1 shows the name of the game 00078 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00079 lcd.color(BLUE); //Set font color to blue 00080 lcd.text_bold(ON); //Bold the text 00081 lcd.text_width(2); 00082 lcd.text_height(2); 00083 lcd.printf(" Rock\n"); 00084 lcd.printf(" Paper\n\n"); 00085 lcd.printf(" Scissor\n\n"); 00086 wait(5); 00087 00088 //Screen 2 shows pushbutton options 00089 L1: 00090 lcd.cls(); 00091 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00092 lcd.color(GREEN); //Set font color to green 00093 lcd.locate(0,2); 00094 lcd.printf(" Button Options\n\n"); 00095 lcd.locate(0,5); 00096 lcd.text_underline(OFF); 00097 lcd.printf(" 1 for Rocks"); 00098 lcd.locate(0,7); 00099 lcd.printf(" 2 for Paper"); 00100 lcd.locate(0,9); 00101 lcd.printf(" 3 for Scissors\n\n\n"); 00102 lcd.text_bold(ON); //Bold the text 00103 lcd.printf(" Press 1 to start!"); 00104 wait(1); 00105 00106 while(1){ 00107 //If push button 1 is pressed, start the game! 00108 if(pb1 != 1){ 00109 00110 //Play button select 00111 FILE *wave_file; 00112 printf("\n\n\nHello, wave world!\n"); 00113 wave_file=fopen("/sd/select.wav","r"); 00114 waver.play(wave_file); 00115 wait(1); 00116 printf("ok!!"); 00117 fclose(wave_file); 00118 00119 L3: 00120 lcd.cls(); //Clear screen 00121 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00122 //Tell player 1 that she/he has 5 seconds to chose between rock,paper and scissors 00123 lcd.locate(0,4); 00124 lcd.color(BLUE); //Set font color to yellow 00125 lcd.printf(" Player 1 \n\n\n"); 00126 lcd.locate(0,6); 00127 lcd.color(GREEN); //Set font color to green 00128 lcd.printf("You have 5 seconds"); 00129 lcd.printf(" to choose\n"); 00130 lcd.printf(" an option!\n"); 00131 wait(5); 00132 00133 00134 //Start timer for 5 seconds 00135 i = 5;; 00136 int red = 1; 00137 //for(i = 5; i > -1 ; i--){ 00138 while(i > -1){ 00139 00140 lcd.cls(); 00141 lcd.locate(4,4); //Place text in the center of the screen 00142 lcd.text_width(8); //Set tet width 00143 lcd.text_height(8); //Set text height 00144 lcd.text_bold(ON); //Set text style to 'bold' 00145 RGB_LED(red*25,0,0);//Increase intensity of light on shiftbrite as time decreases 00146 red = red + 5; 00147 lcd.printf("%d",i); //Display time left on LCD 00148 wait(1); 00149 00150 //Press pushbutton 1 to choose rock 00151 if(pb1 != 1) { 00152 00153 lcd.cls(); 00154 choice1 = 'R'; 00155 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00156 lcd.locate(0,1); 00157 lcd.printf(" You chose rock"); 00158 00159 //Play button select 00160 FILE *wave_file; 00161 printf("\n\n\nHello, wave world!\n"); 00162 wave_file=fopen("/sd/mydir/select.wav","r"); 00163 waver.play(wave_file); 00164 wait(1); 00165 printf("ok!!"); 00166 fclose(wave_file); 00167 00168 lcd.circle(60, 60, 30,WHITE); 00169 wait(3); 00170 lcd.cls(); 00171 break; 00172 } 00173 //Press pushbutton 2 to choose paper 00174 else if(pb2 != 1) { 00175 lcd.cls(); 00176 choice1 = 'P'; 00177 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00178 00179 lcd.locate(0,1); 00180 lcd.printf(" You chose paper"); 00181 00182 //Play button select 00183 FILE *wave_file; 00184 printf("\n\n\nHello, wave world!\n"); 00185 wave_file=fopen("/sd/mydir/select.wav","r"); 00186 waver.play(wave_file); 00187 00188 printf("ok!!"); 00189 fclose(wave_file); 00190 00191 lcd.filled_rectangle(50, 45, 80,90,WHITE); 00192 wait(3); 00193 lcd.cls(); 00194 break; 00195 } 00196 //Press pushbutton 3 to choose scissor 00197 else if(pb3 != 1) { 00198 lcd.cls(); 00199 choice1 = 'S'; 00200 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00201 lcd.locate(0,1); 00202 lcd.printf("You chose scissors"); 00203 00204 //Play button select 00205 FILE *wave_file; 00206 printf("\n\n\nHello, wave world!\n"); 00207 wave_file=fopen("/sd/mydir/select.wav","r"); 00208 waver.play(wave_file); 00209 wait(1); 00210 printf("ok!!"); 00211 fclose(wave_file); 00212 lcd.circle(50, 60, 10,WHITE); 00213 lcd.circle(50, 80, 10,WHITE); 00214 lcd.line(62, 61, 90, 85, WHITE); 00215 lcd.line(62, 81, 90, 55, WHITE); 00216 wait(3); 00217 lcd.cls(); 00218 break; 00219 } 00220 i--; 00221 00222 } 00223 00224 RGB_LED(0,0,0); // Reset shiftbrite color 00225 00226 //------------------------------------------------------------------------------------------------------------------------------------- 00227 // TIME-OUT 00228 //------------------------------------------------------------------------------------------------------------------------------------ 00229 00230 //If user doesn't choose an option, show "Time Out" message and restart game 00231 if(i == -1){ 00232 lcd.cls(); 00233 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00234 lcd.locate(0,5); 00235 lcd.color(RED); 00236 lcd.printf(" Your time is up!"); 00237 lcd.color(GREEN); 00238 //Play time up tune 00239 FILE *wave_file; 00240 printf("\n\n\nHello, wave world!\n"); 00241 wave_file=fopen("/sd/mydir/timeup.wav","r"); 00242 waver.play(wave_file); 00243 00244 printf("ok!!"); 00245 fclose(wave_file); 00246 wait(3); 00247 goto L1; //Starts the game again 00248 } 00249 00250 //-------------------------------------------------------------------------------------------------------------------------------------- 00251 00252 //Send Player 1's choice to Player 2 00253 player1.printf("%c",choice1); 00254 myled2 = 1; 00255 wait(1); 00256 00257 //Receive Player 2's choice 00258 while(1){ 00259 lcd.locate(1,4); 00260 lcd.printf(" Waiting for \n the other player"); 00261 if(player1.readable()){ 00262 choice2 = player1.getc(); 00263 myled = 1; 00264 break; 00265 } 00266 } 00267 00268 00269 //------------------------------------------------------------------------------------------------------------------------------------- 00270 //Check to see who won 00271 //-------------------------------------------------------------------------------------------------------------------------- 00272 //Case 1 : Both players choose same option 00273 if(choice1 == 'R' && choice2 == 'R' || 00274 choice1== 'P' && choice2 == 'P' || 00275 choice1 == 'S' && choice2 == 'S' ) { 00276 lcd.cls(); 00277 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00278 lcd.locate(1,2); 00279 lcd.printf(" It's a draw!"); 00280 wait(4); 00281 00282 } 00283 //Case 2: 00284 //Player 1 : Rock 00285 //Player 2 : Paper 00286 else if(choice1 == 'R' && choice2 == 'P'){ 00287 00288 lcd.cls(); 00289 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00290 00291 lcd.locate(1,2); 00292 lcd.printf(" P1 chose Rock\n"); 00293 lcd.printf(" P2 chose Paper\n\n\n\n"); 00294 lcd.locate(1,5); 00295 lcd.printf(" Player 2 wins!"); 00296 lcd.filled_rectangle(50, 60, 80,90,WHITE); 00297 p2++; 00298 wait(4); 00299 00300 } 00301 //Case 3: 00302 //Player 1 : Paper 00303 //Player 2 : Rock 00304 else if(choice2== 'P' && choice1== 'R'){ 00305 00306 lcd.cls(); 00307 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00308 lcd.locate(1,2); 00309 lcd.printf("P1 chose Paper\n"); 00310 lcd.printf(" P2 chose Rock\n\n"); 00311 lcd.locate(1,5); 00312 lcd.printf("P1 wins!"); 00313 lcd.filled_rectangle(50, 60, 80,90,WHITE); 00314 p1++; 00315 wait(5); 00316 00317 } 00318 00319 //Case 4: 00320 //Player 1 : Paper 00321 //Player 2 : Scissor 00322 00323 else if(choice1 == 'P' && choice2 == 'S'){ 00324 00325 lcd.cls(); 00326 lcd.locate(1,1); 00327 lcd.printf("P1 chose Paper\n"); 00328 lcd.printf(" P2 chose Scissors\n\n"); 00329 lcd.locate(1,4); 00330 lcd.printf("P2 wins!"); 00331 p2++; 00332 lcd.circle(50, 60, 10,WHITE); 00333 lcd.circle(50, 80, 10,WHITE); 00334 lcd.line(62, 61, 90, 85, WHITE); 00335 lcd.line(62, 81, 90, 55, WHITE); 00336 wait(5); 00337 00338 } 00339 //Case 5: 00340 //Player 1 : Scissor 00341 //Player 2 : Paper 00342 else if(choice1 == 'S' && choice2 == 'P'){ 00343 00344 lcd.cls(); 00345 lcd.locate(1,1); 00346 lcd.printf("P1 chose Scissors\n"); 00347 lcd.printf(" P2 chose Paper\n\n"); 00348 lcd.locate(1,4); 00349 lcd.printf("P1 wins!"); 00350 p1++; 00351 lcd.circle(50, 60, 10,WHITE); 00352 lcd.circle(50, 80, 10,WHITE); 00353 lcd.line(62, 61, 90, 85, WHITE); 00354 lcd.line(62, 81, 90, 55, WHITE); 00355 wait(5); 00356 00357 } 00358 //Case 6: 00359 //Player 1 : Rock 00360 //Player 2 : Scissors 00361 else if(choice1 == 'R' && choice2 == 'S'){ 00362 00363 lcd.cls(); 00364 lcd.locate(1,1); 00365 lcd.printf("P1 chose Rock\n"); 00366 lcd.printf(" P2 chose Scissors\n\n"); 00367 lcd.locate(1,3); 00368 lcd.printf("P1 wins!"); 00369 p1++; 00370 lcd.circle(60, 70, 30,WHITE); 00371 wait(5); 00372 00373 } 00374 //Case 7: 00375 //Player 1 : Scissors 00376 //Player 2 : Rock 00377 00378 else if(choice1 == 'S' && choice2 == 'R'){ 00379 00380 lcd.cls(); 00381 lcd.locate(1,1); 00382 lcd.printf("P1 chose Scissors\n"); 00383 lcd.printf(" P2 chose Rock\n\n"); 00384 lcd.locate(1,3); 00385 lcd.printf("P2 wins!"); 00386 p2++; 00387 lcd.circle(60, 70, 30,WHITE); 00388 wait(5); 00389 00390 } 00391 00392 00393 lcd.cls(); 00394 lcd.locate(1,4); 00395 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00396 lcd.printf(" P1 Score : %d\n",p1); 00397 lcd.printf(" P2 Score : %d\n\n\n\n",p2); 00398 lcd.printf(" 1 to continue\n\n"); 00399 lcd.printf(" 2 to end\n\n"); 00400 00401 char continue1 = 'N'; 00402 char continue2; 00403 //char cont; 00404 00405 00406 while(1){ 00407 if(pb1 != 1) { 00408 lcd.cls(); 00409 continue1 = 'Y'; 00410 break; 00411 //goto L3; 00412 } 00413 else if(pb2 != 1) { 00414 lcd.cls(); 00415 continue1 = 'N'; 00416 break; 00417 } 00418 } 00419 00420 00421 player1.printf("%c",continue1); 00422 myled3 = 1; 00423 wait(1); 00424 00425 while(1){ 00426 lcd.locate(1,4); 00427 lcd.printf(" Waiting for \n the other player"); 00428 if(player1.readable()){ 00429 continue2 = player1.getc(); 00430 myled = 1; 00431 break; 00432 } 00433 } 00434 00435 //If both say continue then you need to go back to L3 00436 if(continue1 == 'Y' && continue2 == 'Y') goto L3; 00437 else goto L2; 00438 }} 00439 00440 00441 00442 00443 00444 //If game ends : 00445 L2: 00446 lcd.cls(); 00447 //Create animation of two bouncing balls 00448 float fx=50.0,fy=21.0,vx=5.0,vy=0.4,vx2=2,fx2=40.0; 00449 int x=50,y=21,radius=4; 00450 int x2 = 30, y2 = 10; 00451 00452 //draw walls 00453 wait(2); 00454 //If Player 1's score is higher than Player 2's score 00455 if(p1 > p2) 00456 //lcd.printf("Player 1 wins!"); 00457 lcd.text_string("Player 1 Wins!", 2, 4, FONT_7X8, WHITE); 00458 //If Player 1's score is equal to Player 2's score 00459 else if(p1 == p2) 00460 //lcd.printf("It's a draw!"); 00461 lcd.text_string("It's a draw!", 2, 4, FONT_7X8, WHITE); 00462 //If Player 2's score is higher than Player 1's score 00463 else if(p2 > p1) 00464 //lcd.printf("Player 2 wins!"); 00465 lcd.text_string("Player 2 Wins!", 2, 4, FONT_7X8, WHITE); 00466 00467 // Play winning tune 00468 FILE *wave_file; 00469 printf("\n\n\nHello, wave world!\n"); 00470 wave_file=fopen("/sd/mydir/win.wav","r"); 00471 waver.play(wave_file); 00472 00473 printf("ok!!"); 00474 fclose(wave_file); 00475 00476 for (int i=0; i<100; i++) { 00477 //draw ball 00478 //lcd.cls(); 00479 if(p1 > p2) 00480 lcd.text_string("Player 1 Wins!", 2, 4, FONT_7X8, WHITE); 00481 00482 else if(p1 == p2) 00483 lcd.text_string("It's a draw!", 2, 4, FONT_7X8, WHITE); 00484 00485 else if(p2 > p1) 00486 lcd.text_string("Player 2 Wins!", 2, 4, FONT_7X8, WHITE); 00487 00488 lcd.filled_circle(x, y, radius, RED); 00489 lcd.filled_circle(x2,y2,radius,BLUE); 00490 RGB_LED(x,y,x); 00491 //bounce off edge walls and slow down a bit 00492 if ((x<=radius+1) || (x>=126-radius)) vx = -.90*vx; 00493 if ((y<=radius+1) || (y>=126-radius)) vy = -.90*vy; 00494 if ((x2<=radius+1) || (x2>=126-radius)) vx2 = -.90*vx2; 00495 if ((y2<=radius+1) || (y2>=126-radius)) vy = -.90*vy; 00496 //erase old ball location 00497 lcd.filled_circle(x, y, radius, BLACK); 00498 lcd.filled_circle(x2, y2, radius, BLACK); 00499 //move ball 00500 fx=fx+vx; 00501 fx2=fx2+vx2; 00502 fy=fy+vy; 00503 x=(int)fx; 00504 y=(int)fy; 00505 x2=(int)fx2; 00506 y2=(int)fy; 00507 } 00508 lcd.cls(); 00509 00510 }
Generated on Sun Jul 17 2022 17:35:20 by
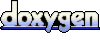