
Connecting a Multi-Tech Systems Dragonfly™ to Twilio's Sync for IoT Quickstart. Blink a dev board LED.
Dependencies: MQTT MbedJSONValue mbed mtsas
Fork of DragonflyMQTT by
MTSCellularManager.cpp
00001 #include "MTSCellularManager.hpp" 00002 00003 MTSCellularManager::MTSCellularManager( 00004 const char* apn_ 00005 ) : apn(apn_), 00006 io(NULL), 00007 radio(NULL) 00008 {} 00009 00010 00011 MTSCellularManager::~MTSCellularManager() { 00012 delete radio; 00013 delete io; 00014 } 00015 00016 bool MTSCellularManager::init() { 00017 logInfo("Initializing Cellular Radio"); 00018 00019 io = new mts::MTSSerialFlowControl( 00020 RADIO_TX, 00021 RADIO_RX, 00022 RADIO_RTS, 00023 RADIO_CTS 00024 ); 00025 00026 /* Radio default baud rate is 115200 */ 00027 io->baud(115200); 00028 if (! io) 00029 return false; 00030 00031 radio = mts::CellularFactory::create(io); 00032 radio2 = mts::CellularFactory::create(io); 00033 if (! radio) 00034 return false; 00035 00036 /* 00037 * Transport must be set properly before any TCPSocketConnection or 00038 * UDPSocket objects are created 00039 */ 00040 Transport::setTransport(radio); 00041 00042 logInfo("setting APN"); 00043 if (radio->setApn(apn) != MTS_SUCCESS) { 00044 logError("failed to set APN to \"%s\"", apn); 00045 return false; 00046 } 00047 00048 logInfo("bringing up the link"); 00049 if (! radio->connect()) { 00050 logError("failed to bring up the link"); 00051 return false; 00052 } else { 00053 return true; 00054 } 00055 } 00056 00057 void MTSCellularManager::uninit() { 00058 logInfo("finished - bringing down link"); 00059 radio->disconnect(); 00060 delete radio; 00061 delete io; 00062 }
Generated on Tue Jul 12 2022 21:09:00 by
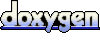